To perform a Git pull operation on Azure without being prompted for a password, you can use a personal access token (PAT) and configure it in your Git remote URL.
Here's how you can set it up:
git remote set-url origin https://<username>:<personal_access_token>@dev.azure.com/<organization>/<project>/_git/<repository>
git pull
Make sure to replace `<username>`, `<personal_access_token>`, `<organization>`, `<project>`, and `<repository>` with your actual Azure DevOps account details.
Understanding Git Authentication Methods
The Role of Authentication in Git
Authentication is a fundamental aspect of using Git, particularly when interacting with remote repositories. It serves to verify your identity and ensure that only authorized users can access codebases. Without proper authentication, you may find yourself locked out of your repositories or continually prompted for your password.
Common Authentication Methods
Username and Password
Traditionally, the most straightforward method is using a username and password. While easy to set up, this method can be cumbersome and insecure, particularly when working with frequent Git operations like pulling changes.
SSH Keys
SSH keys provide a more secure and automated approach. With SSH, you can use a pair of cryptographic keys—one public and one private—to authenticate without transmitting a password.
Personal Access Tokens (PATs)
Personal Access Tokens are essentially passwords that Microsoft Azure DevOps allows you to generate for specific access. They are an excellent alternative to using your account password directly, especially for automated scripts.
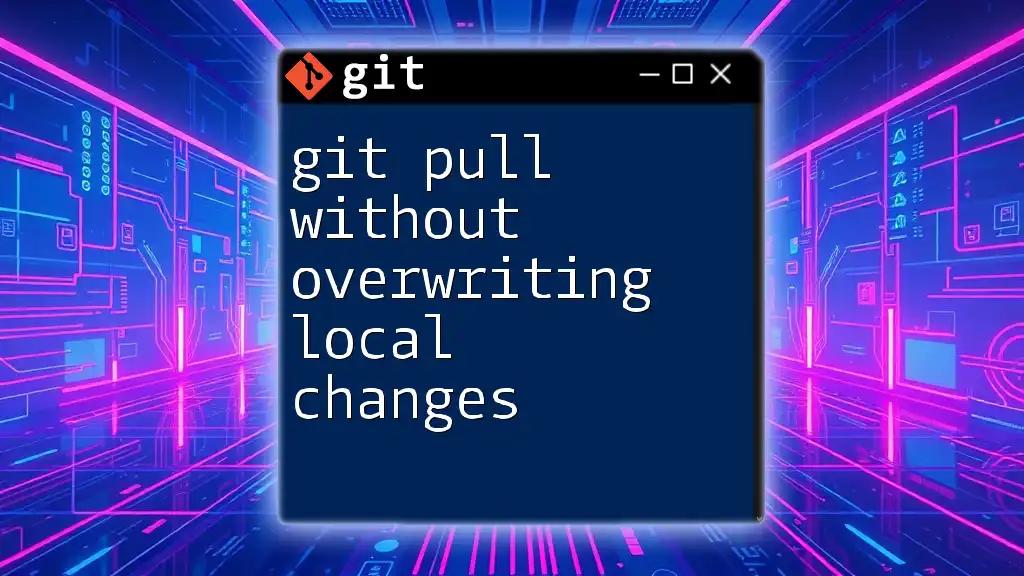
How to Set Up Azure for Password-less Git Pulls
Setting Up SSH Keys
What are SSH Keys?
SSH keys consist of a public key, which you can share, and a private key, which you keep secret. This asymmetric encryption method secures your Git operations and provides an easy way to authenticate without repeated password prompts.
Generating SSH Keys
To start using SSH keys, you first need to generate one. Below are the commands for various operating systems:
Linux/Mac:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Windows (using Git Bash):
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
During this process, you’ll be prompted to specify where to save the key and, optionally, a passphrase for additional security.
Adding SSH Key to Azure DevOps
After generating your SSH key, you need to add the public key to your Azure DevOps account.
-
Locate Your Public Key: You can view your public key using:
cat ~/.ssh/id_rsa.pub
-
Navigate to Azure DevOps: Go to the "User Settings" in Azure DevOps and select "SSH Public Keys."
-
Add New Key: Click on "Add" and paste the public key you just copied. Give it a recognizable title, and save the changes.
Using Personal Access Tokens (PAT)
What is a Personal Access Token?
A Personal Access Token (PAT) is a secure token you can use instead of a password when interacting with Azure DevOps services. It's particularly useful for accessing Azure DevOps APIs and can be generated with specific permissions.
Creating a Personal Access Token in Azure
To create a PAT, follow these simple steps:
- Navigate to User Settings: Click on your profile picture in Azure DevOps.
- Select Personal Access Tokens: Click on "Personal Access Tokens" and then "New Token."
- Configure Your Token: Set an expiration date and select the scopes necessary for your operations.
- Generate and Copy the Token: After creating the token, make sure to copy it immediately as you won't be able to see it again.
Using PATs in Git Commands
After generating your PAT, you can use it in your Git commands. Here’s an example:
git clone https://[USERNAME]:[PAT]@dev.azure.com/[ORG]/[PROJECT]/_git/[REPO]
Replace `[USERNAME]`, `[PAT]`, `[ORG]`, `[PROJECT]`, and `[REPO]` with your specific details. This approach provides a streamlined way to authenticate without entering your password each time.
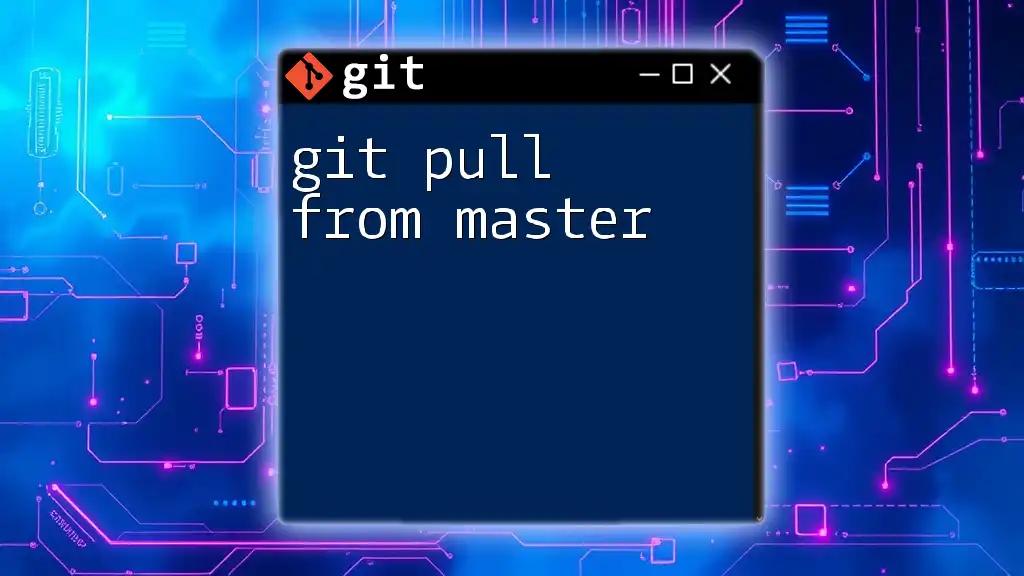
Configuring Your Local Git Repository
Storing Credentials Temporarily
If you prefer not to enter your credentials repeatedly but don't mind them being cached temporarily, you can store them for a limited time. Use the following command:
git config --global credential.helper cache
This command typically caches your credentials in memory for a period (15 minutes by default), which can be extended if needed.
Storing Credentials Permanently
If you want to avoid entering any credentials altogether, use the following command to store them permanently:
git config --global credential.helper store
With this, Git will save your credentials to your local machine.
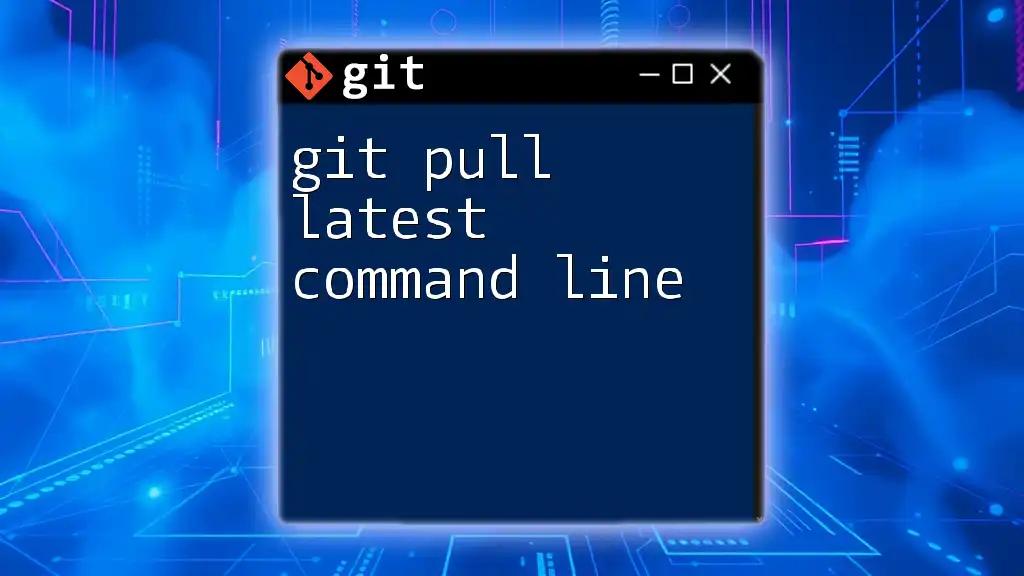
Executing Git Pull Without Password Prompt
Using SSH for Git Pull Command
Once you've set up SSH keys, you can use the `git pull` command without any password prompts. Here’s how you can execute it:
git pull git@dev.azure.com:[ORG]/[PROJECT]/_git/[REPO]
In this command, you simply replace `[ORG]`, `[PROJECT]`, and `[REPO]` with your respective Azure DevOps organization, project, and repository names.
Using PATs for Git Pull Command
If you opted for a Personal Access Token, your `git pull` command will look like this:
git pull https://[USERNAME]:[PAT]@dev.azure.com/[ORG]/[PROJECT]/_git/[REPO]
Again, ensure you replace all placeholders with your specific details. This approach allows for efficient code updates without annoying prompts.

Troubleshooting Common Issues
Issues with SSH Keys
If you encounter issues with SSH keys, ensure that:
- The public key was correctly added to your Azure DevOps account.
- The private key permissions are correctly set. On Linux, you can change permissions using:
chmod 600 ~/.ssh/id_rsa
Issues with Personal Access Tokens
Sometimes PATs can be problematic, particularly if they have not been granted the necessary scopes. Double-check permissions and ensure that your token is still valid.

Conclusion
In summary, utilizing azure git pull without prompt password is not only possible but also straightforward when you configure your authentication methods properly. Whether you choose SSH keys or Personal Access Tokens, either method streamlines your workflow, allowing you to concentrate more on coding than on repetitive password entry. Master these techniques, and you'll enhance your Git experience significantly.