To connect a Streamlit application to git for version control, you can initialize a git repository in your project directory with the following command:
git init
Understanding Git Basics
What is Git?
Git is a distributed version control system that allows multiple developers to work on a project simultaneously. It tracks changes, enabling users to revert to previous states or collaborate effectively, making it a crucial tool for managing code. Understanding version control is essential for maintaining the integrity and history of your projects.
Key Git Commands
Familiarizing yourself with essential Git commands will enhance your workflow with Streamlit applications. Here are some foundational commands to remember:
- `git init`: Initializes a new Git repository in your project directory.
- `git add`: Stages changes to be committed.
- `git commit`: Saves the staged changes along with a message.
- `git push`: Sends your committed changes to a remote repository.
These commands help manage your Streamlit code, ensuring that every iteration and feature enhancement is tracked.
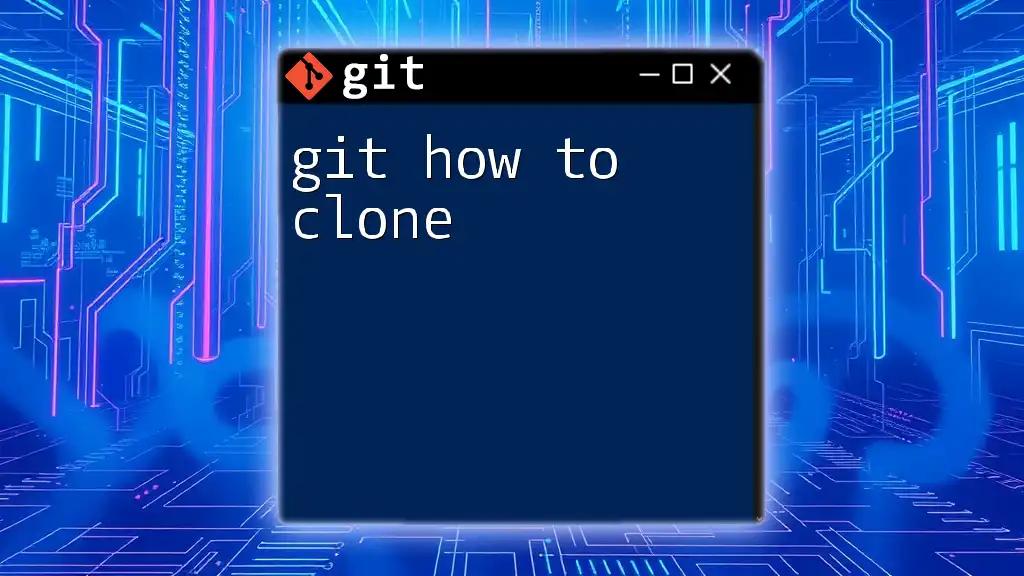
Setting Up Your Streamlit Project
Installing Streamlit
Before diving into Git, you need to have Streamlit installed. Use the following command to install Streamlit via pip:
pip install streamlit
This command fetches the Streamlit library and installs it in your Python environment. Once installed, you can start creating your Streamlit applications.
Creating a New Streamlit Project
To start your first project, create a new directory and initiate your Streamlit application. Here's a brief guide:
-
Create a new directory for your project:
mkdir my_streamlit_app cd my_streamlit_app
-
Start your first Streamlit app by creating a file called `app.py` and running:
streamlit run app.py
This command launches a local server where your Streamlit app will be visible.
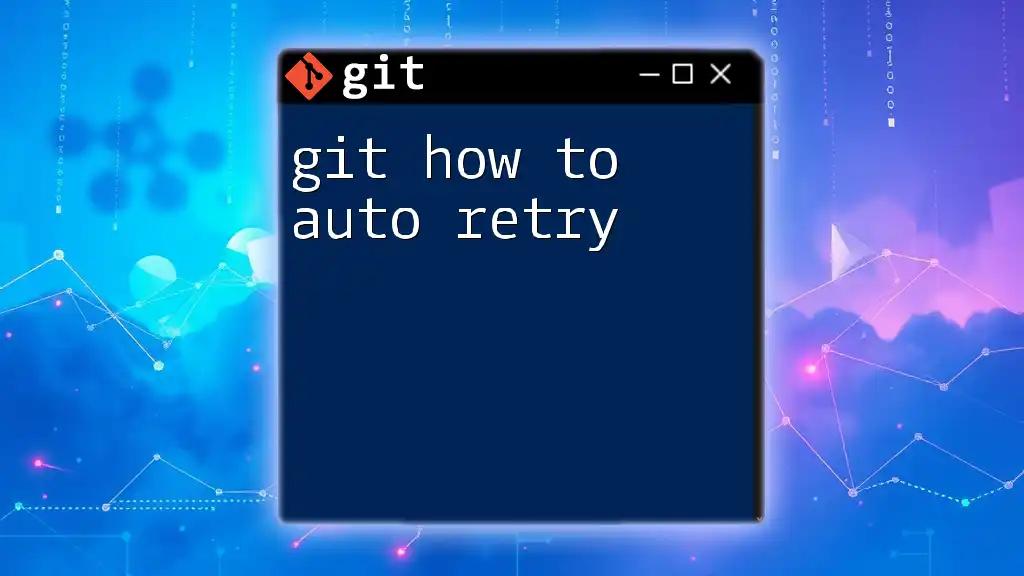
Initializing Git in Your Streamlit Project
Setting Up Git
Once your Streamlit project is ready, it's time to initialize Git. Navigate to your project directory and run:
git init
This command creates a new Git repository, transforming your project directory into a version-controlled environment.
Configuring Your Git Repository
Adding a .gitignore File
To avoid committing unnecessary files, you must configure a `.gitignore` file. This file ensures that specific files and directories are excluded from Git versioning. For a Streamlit project, consider the following entries:
__pycache__/
*.py[cod]
*.db
These entries prevent binary files and temporary cache directories from being tracked, keeping your repository clean.
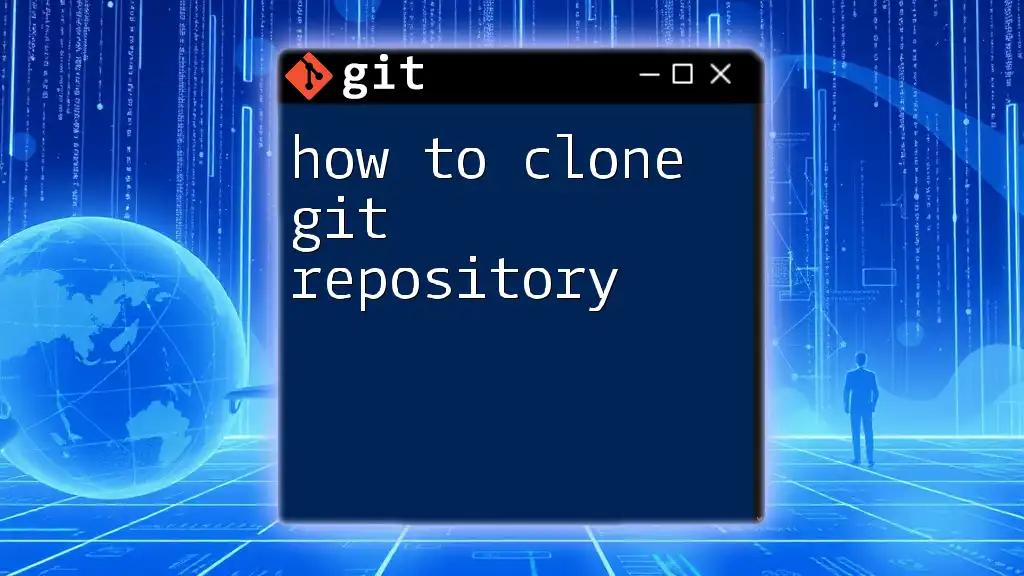
Committing Changes in Your Streamlit Project
Staging and Committing Files
After setting up your project, you will need to stage your changes before committing them. Use the following command to stage all modified files:
git add .
This command tells Git to track all changes in your project. Once staged, you can commit your changes with a descriptive message:
git commit -m "Initial commit"
Committing often with clear messages helps maintain a clean history of your project developments.
Creating Meaningful Commit Messages
Clear and descriptive commit messages are essential. They help your team (and your future self) understand the rationale behind changes. For example, instead of using a vague message like “update,” consider:
- Good: "Implement sidebar functionality in Streamlit app"
- Bad: "Fix stuff"
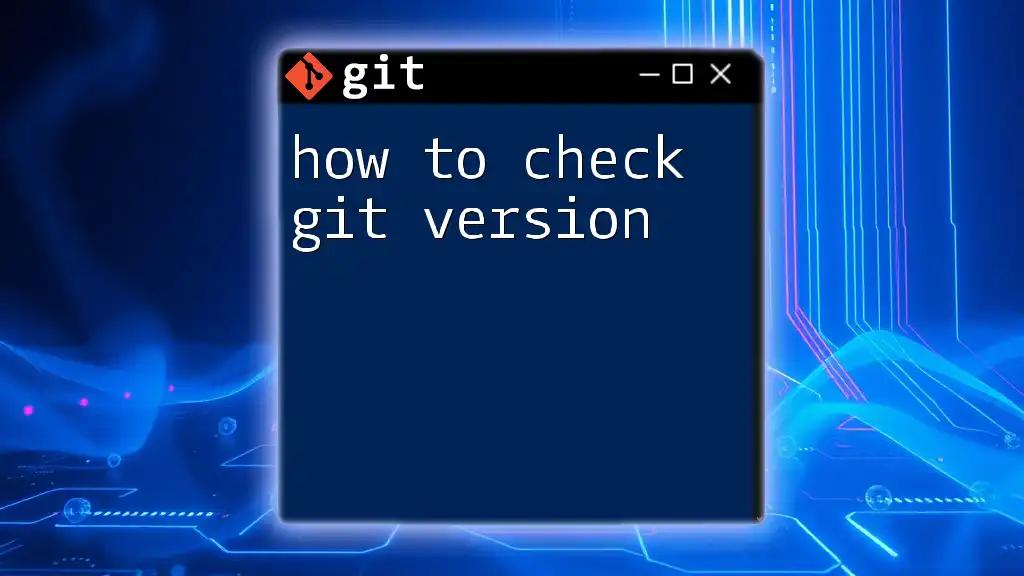
Pushing Your Streamlit Project to Remote Repository
Setting Up a Remote Repository
To collaborate on a Streamlit project, you’ll want to push your local repository to a remote service like GitHub. First, create a new repository on GitHub (do not initialize with a README). You can link your local project to this repository with:
git remote add origin https://github.com/username/repo_name.git
This command configures the remote repository, allowing for seamless pushing of local changes.
Pushing Code to GitHub
Once linked, you can push your initial commit to GitHub with:
git push -u origin master
This command uploads your commits to the `master` branch of your remote repository, making your work accessible online.

Collaborating on Streamlit Projects with Git
Pulling Changes from Remote Repository
When collaborating with others, you'll often need to pull the latest changes from your remote repository to keep your local copy up-to-date. Use the following command:
git pull origin master
This command merges any new changes from the remote branch into your local branch, ensuring you’re working with the latest updates.
Branching in Git
Branching allows multiple features or fixes to be developed simultaneously without interfering with the main codebase. To create and switch branches, use:
git checkout -b new-feature
This command creates a new branch named `new-feature` and switches to it, enabling you to work on new functionality independently.

Best Practices for Using Git with Streamlit
Consistent Commit Frequency
Commit your changes frequently throughout your development process. This practice minimizes data loss and allows you to revert to earlier versions if needed. Setting a personal rule, like committing after completing logical segments of your work, can help maintain consistency.
Writing Descriptive README Files
A well-structured README file serves as the first point of reference for anyone new to your project. It should provide an overview of the application, how to install dependencies, run it locally, and any additional documentation necessary for collaboration.
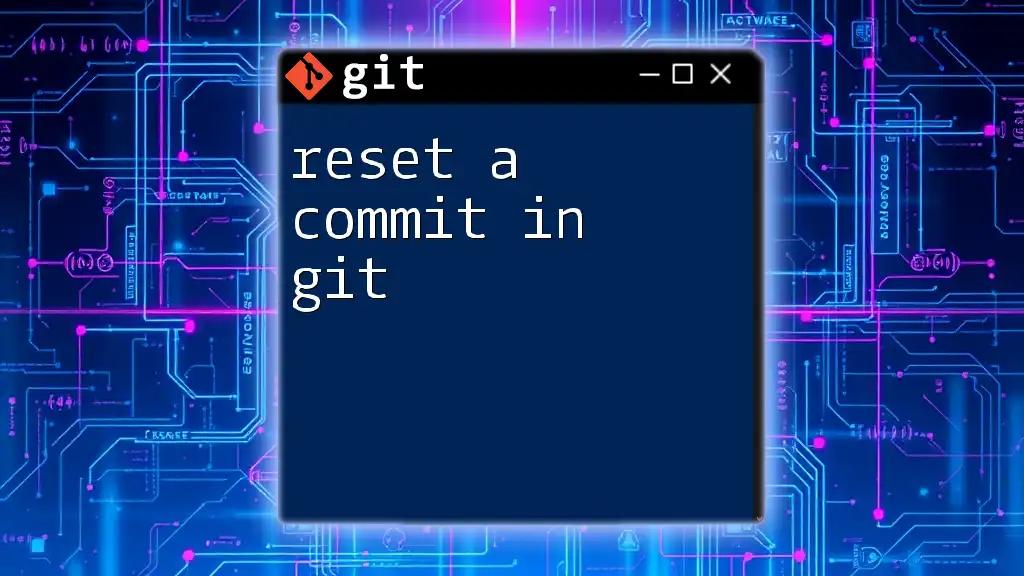
Conclusion
By mastering the connection between Streamlit and Git, you empower every project you undertake with the capability of effective version control. As you continue to develop your skills, leverage these tools to enhance your development workflow and collaborate efficiently with others.
Now that you’ve learned how to connect Streamlit to Git, it's time to put your knowledge into practice. Start your projects with Git, and unlock the potential of collaborative and version-controlled development!
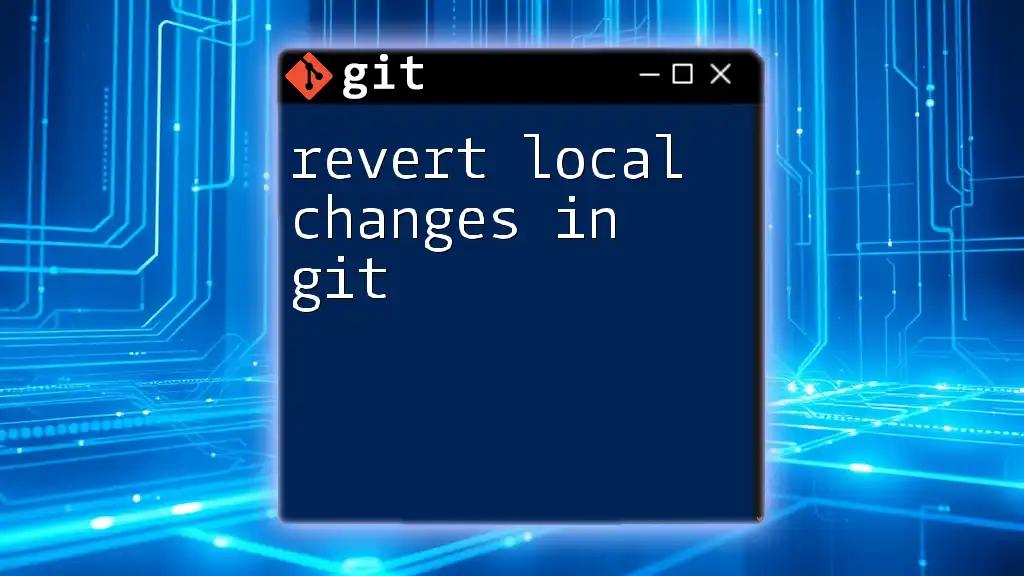
Additional Resources
For deeper dives into Git and Streamlit, consult the official documentation and explore additional Git tutorials for continuous learning.