To create Git hooks, you can use a simple shell script that resides in the `.git/hooks` directory of your repository, allowing you to automate actions like pre-commit or post-merge.
Here’s an example of a pre-commit hook written in bash:
#!/bin/bash
# A simple pre-commit hook to prevent committing code with TODO comments
if git grep -q 'TODO'; then
echo "Commit aborted: You have TODO comments in your code."
exit 1
fi
Make sure to give execution permission to the script with `chmod +x .git/hooks/pre-commit` after saving it.
Understanding Git Hooks
What are Git Hooks?
Git hooks are scripts that Git executes before or after certain events, such as commits, merges, or push operations. They allow developers to automate tasks, ensuring adherence to required scripts or actions in the development workflow. These hooks can validate code, notify teams of changes, or enforce project standards, making them a valuable tool for any development team.
Common Git hook types include:
- Pre-commit: Executes right before a commit is finalized. Useful for performing checks and validations.
- Post-commit: Runs immediately after a commit operation. Ideal for notifications or logging.
- Pre-push: Activated before code is pushed to a remote repository. Great for testing to ensure code quality.
How Git Hooks Work
Git hooks are placed in the `.git/hooks` directory of your repository. Each hook script must be executable and can utilize various programming languages such as Shell, Python, Perl, or Ruby. When the triggering event occurs, Git automatically runs the corresponding hook script, which can perform specific tasks like checking for error conditions or executing tests.
For instance, during a commit, the `pre-commit` hook will run before finalizing your changes, allowing you to catch errors early in your workflow.
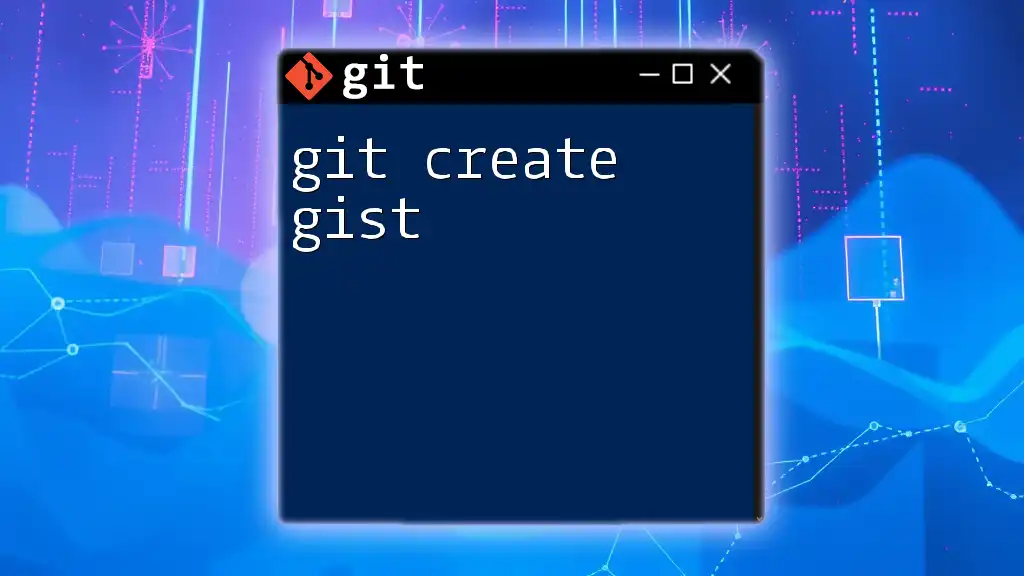
Getting Started with Git Hooks
Setting Up Your Environment
To effectively manage Git hooks, choose a suitable program to create and edit scripts. Reliable options include:
- Text Editors: Visual Studio Code, Sublime Text, Atom, or even built-in editors like Vim or Nano. Each provides syntax highlighting, which can make writing scripts more comfortable.
- Command Line Tools: You can also use the command line to create and modify hooks quickly.
Creating Your First Git Hook
Start by crafting a simple pre-commit hook that checks for whitespace errors. Here's how to do it step-by-step:
- Navigate to the hooks directory in your repository:
cd .git/hooks
- Create a new file named `pre-commit` and open it in your text editor:
touch pre-commit nano pre-commit
- Insert the following code snippet:
This script checks if there are any changes that consist only of whitespace before allowing the commit to proceed.#!/bin/sh if git diff --cached | grep -q '^[[:space:]]*$'; then echo 'Error: Commit contains only whitespace!' exit 1 fi
Making Your Hook Executable
For your hook to function correctly, it must be executable. Use the `chmod` command to adjust file permissions:
chmod +x .git/hooks/pre-commit
Now your pre-commit hook is ready to run whenever you make a commit!
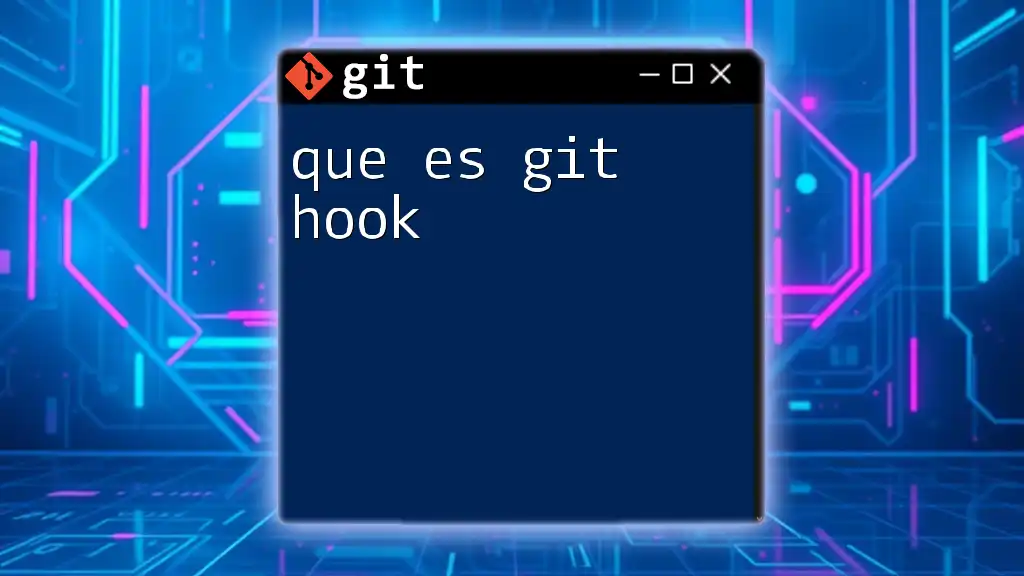
Advanced Git Hook Examples
Pre-commit Hooks
Elevate your pre-commit hook by adding a linter check. A code sample for running eslint could look like this:
#!/bin/sh
npm run lint
Implementing linter checks prevents poorly formatted code from being committed, which can save time during code reviews.
Post-commit Hooks
Post-commit hooks are useful for generating notifications or logging pertinent information. For example, to alert a team chat platform after a commit:
#!/bin/sh
curl -X POST -d "New commit pushed!" http://example.com/notify
This hook can ensure your team stays informed about changes, fostering better collaboration.
Pre-push Hooks
Run tests automatically before your code is pushed using a pre-push hook. Here’s a simple example:
#!/bin/sh
npm test
This ensures your code passes unit tests, aiding long-term stability and maintainability in your repository.
Customizing Hooks
You can customize your hooks further by integrating with additional scripts or command-line tools to enhance functionalities, including:
- Integrating CI/CD tools like Jenkins, Travis CI, or CircleCI to trigger builds or deployments
- Linking with code coverage tools to ensure quality before pushing
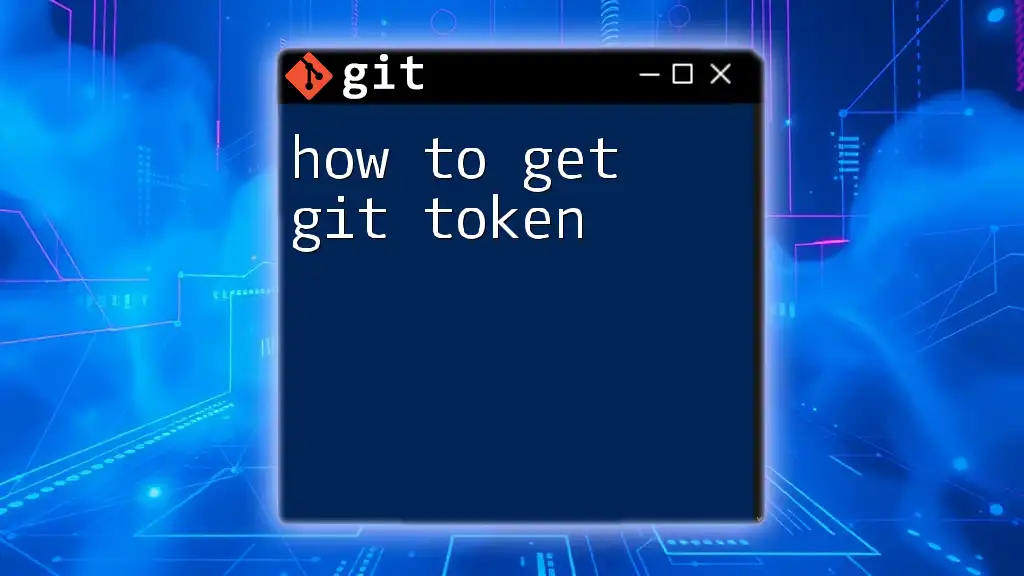
Debugging Git Hooks
Common Issues with Git Hooks
Sometimes, hooks may not trigger, which can be frustrating. Common issues include:
- Incorrect permissions on the hook file
- Syntax errors in the script
- Not being in the appropriate Git repository context
Tips for Effective Debugging
To debug hooks effectively:
- Use `echo` statements within your hooks to log outputs and help trace the execution flow.
- Check file permissions using:
ls -l .git/hooks/
- Ensure the correct hook is invoked during the appropriate Git operation.
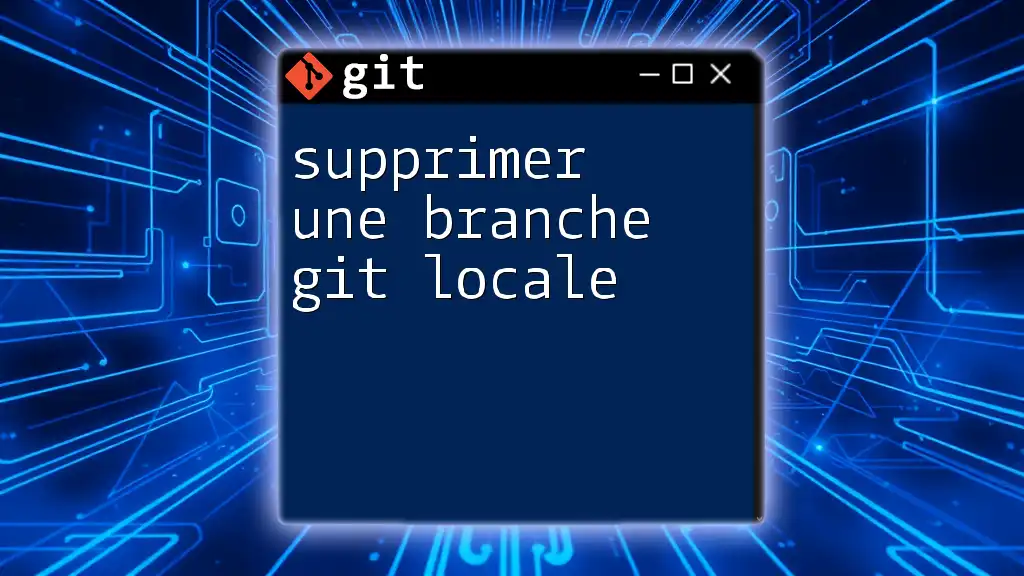
Tools and Frameworks for Managing Git Hooks
Pre-built Git Hook Frameworks
Managing hooks manually can be cumbersome, leading to mistakes or missed hooks. Using frameworks like:
- Husky: Facilitates the management of Git hooks in JavaScript projects.
- lefthook: A fast and efficient way to run hooks across multiple repositories.
These tools help simplify the process and reduce errors, making it easier to implement and maintain your Git hooks effectively.
Integrating Hooks with CI/CD
Git hooks can play a crucial role in your CI/CD workflows. For instance, you can set up a pre-push hook that triggers a CI pipeline, ensuring that all tests pass before code reaches the production environment. This adds an additional layer of verification that can help catch issues before they impact users.
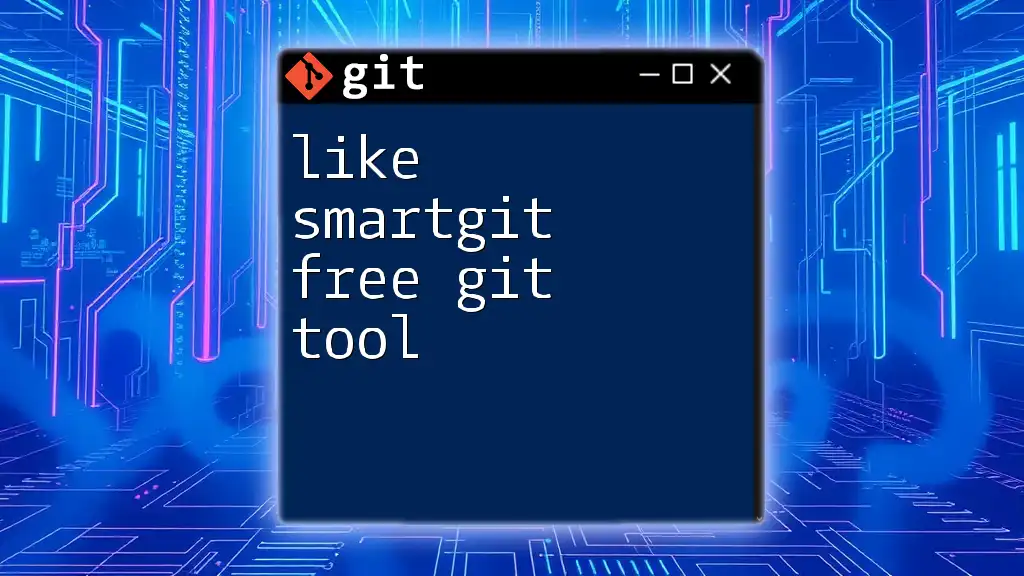
Best Practices for Using Git Hooks
Organizing Your Hooks
Maintain a clean setup by organizing your hooks logically. Group them according to their purpose and ensure names clearly indicate their function. This makes it easier to navigate and update them as necessary.
Avoiding Common Pitfalls
To avoid common mistakes:
- Test hooks thoroughly in a local environment before applying them across teams.
- Document the purpose and effects of each hook to ensure that other team members understand their use.
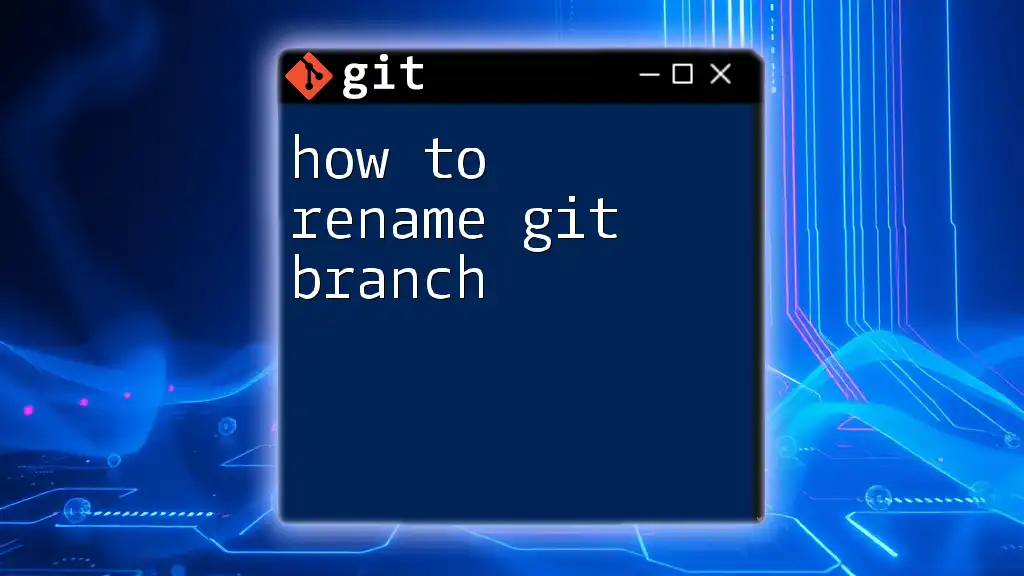
Conclusion
Git hooks are powerful tools that can significantly enhance your development workflow by automating repetitive tasks and ensuring code quality. Whether you're validating commits or automating deployment processes, understanding how to create and manage Git hooks effectively will lead you to a more efficient coding practice. Start experimenting with Git hooks today for improved automation and code standards.
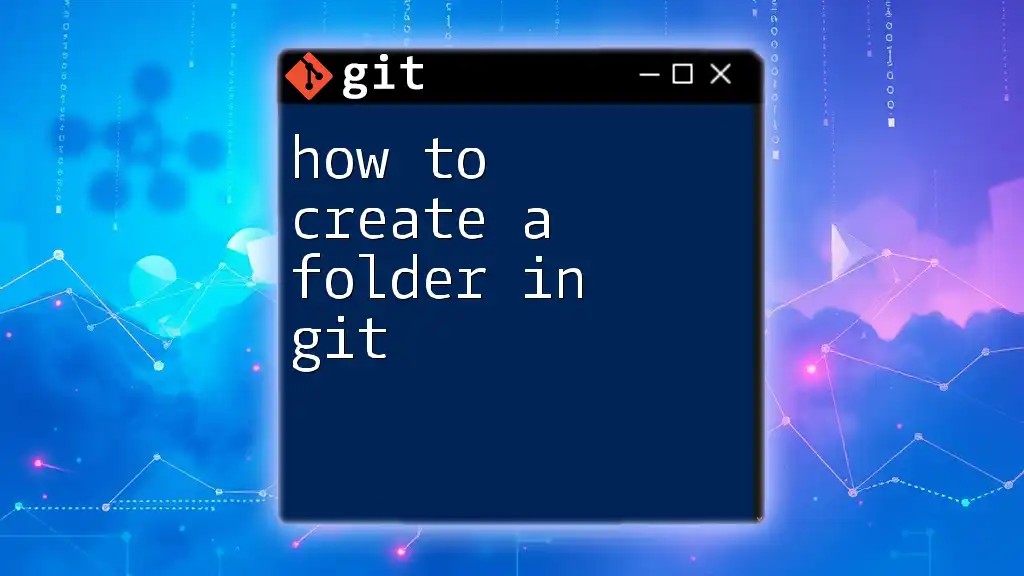
Additional Resources
For further exploration, refer to the official Git documentation on hooks, and consider diving into various tutorials and tool libraries that can amplify your understanding and use of Git hooks in your projects. Consider tools like Husky or Lefthook to elevate your Git workflows even further. With the right setup, you can harness the full power of Git hooks in your development process!