To reauthenticate Git on a Mac, you can use the following command to clear your existing credentials and prompt for your username and password again.
git credential-osxkeychain erase
Understanding Git Authentication
What is Git Authentication?
Git authentication is the process through which Git verifies the identity of a user trying to perform operations on a repository. This is critical for maintaining the security and integrity of your code. By authenticating, you can ensure that only authorized users can make changes or access the repository.
Common Authentication Methods
SSH Keys
SSH (Secure Shell) keys provide a secure method for connecting to Git repositories without needing to enter a password every time. When you generate an SSH key pair, you create a public-private key pair that verifies your identity. Users can also limit access to specific machines, enhancing security further.
HTTPS Credentials
Using HTTPS for authentication involves providing a username and password when connecting to a Git repository. While HTTPS is straightforward and usually easy to set up, it requires entering credentials frequently unless stored.
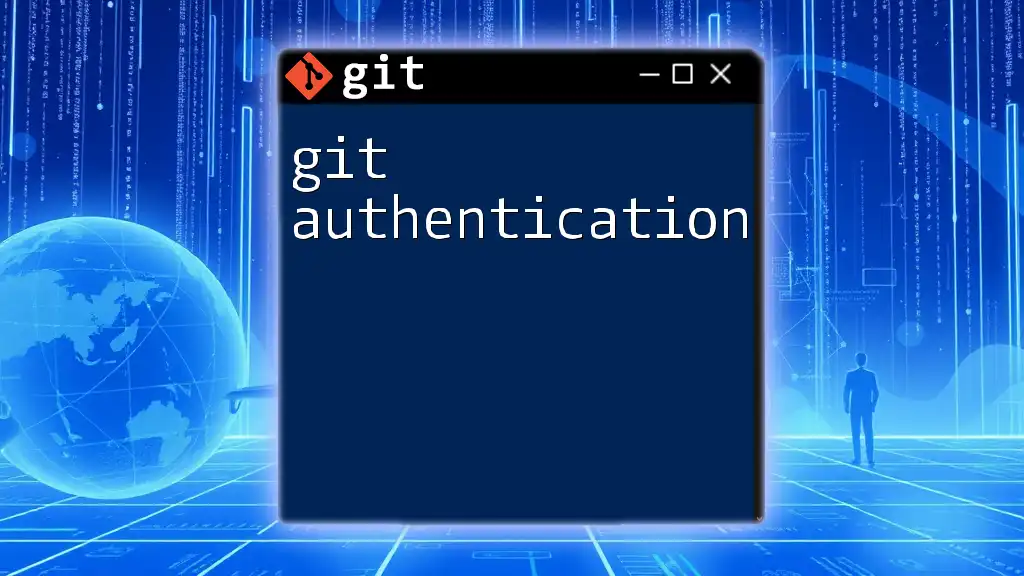
Reasons You May Need to Re-Authenticate
Expired Credentials
Credentials can have an expiration date, especially if your organization implements strict security policies. If your credentials expire, you will need to reauthenticate to continue using Git without interruptions.
Changes in Security Policies
Sometimes, your organization may change its security policies, requiring employees to update their authentication methods or credentials. Such changes could necessitate re-authentication in order to comply with new guidelines.
Key or Password Rotation
For security reasons, you may be required to rotate your keys or passwords periodically. If you have generated a new key or changed your password, you’ll need to re-authenticate before proceeding with Git operations.
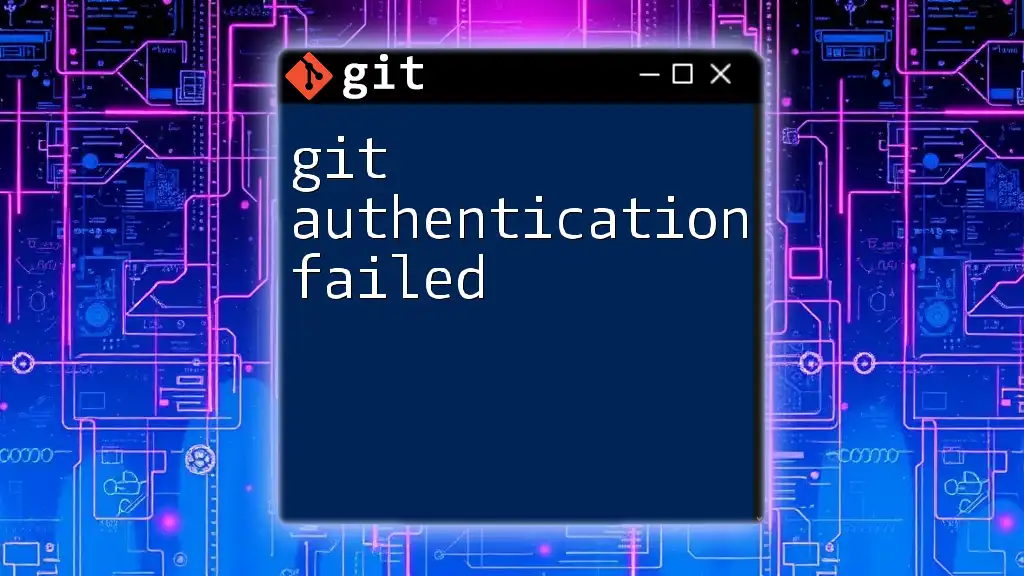
Steps to Re-Authenticate Git on Mac
Confirming Your Current Authentication Method
Before you start the reauthentication process, it’s important to know whether you are using SSH or HTTPS as your authentication method. You can check your current remote URL configuration with the following command:
git remote -v
This command will display the URLs associated with your repositories, helping you identify your authentication method.
Re-Authenticating for SSH Users
Generating a New SSH Key (If Needed)
If you need to create a new SSH key, follow these steps:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
In this command:
- `-t rsa` specifies the type of key to create.
- `-b 4096` indicates the number of bits in the key. (More bits make the key more secure.)
- `-C "your_email@example.com"` adds a label to the key.
You will be prompted to choose a file to save the key. Press Enter to accept the default location (`~/.ssh/id_rsa`) or specify a different path.
Adding SSH Key to the SSH Agent
To use the SSH key you just created, you must add it to the SSH agent. This allows your system to use the key securely without needing to enter it every time. Start the SSH agent with the following command:
eval "$(ssh-agent -s)"
Next, add your SSH private key:
ssh-add -K ~/.ssh/id_rsa
This command loads your key into the agent, allowing for seamless authentication when working with Git.
Updating Your SSH Key on Git Hosting Service
After generating a new SSH key, you need to update your key on your Git hosting service, such as GitHub, GitLab, or Bitbucket. Access your Git hosting account settings, navigate to the SSH keys section, and paste your new public key (found in `~/.ssh/id_rsa.pub`). Save your changes, and you’ll be reauthenticated via SSH.
Re-Authenticating for HTTPS Users
Clearing Cached Credentials
If you are using HTTPS and your credentials have changed, you may need to clear the old credentials stored in the macOS Keychain. Use the following command to erase old credentials:
git credential-osxkeychain erase
This command will prompt you to confirm the action to avoid accidental deletions of other stored credentials.
Entering New Credentials
After clearing the old credentials, perform an operation that requires authentication—such as pushing to a remote repository. When you run a command like:
git push origin main
You will be prompted to enter your username and password. Ensure that you enter the updated credentials.
Storing New Credentials
To store your new credentials in the macOS Keychain, run the following command:
git config --global credential.helper osxkeychain
By enabling this setup, Git will securely remember your credentials, minimizing the frequency with which you’re prompted to enter them.
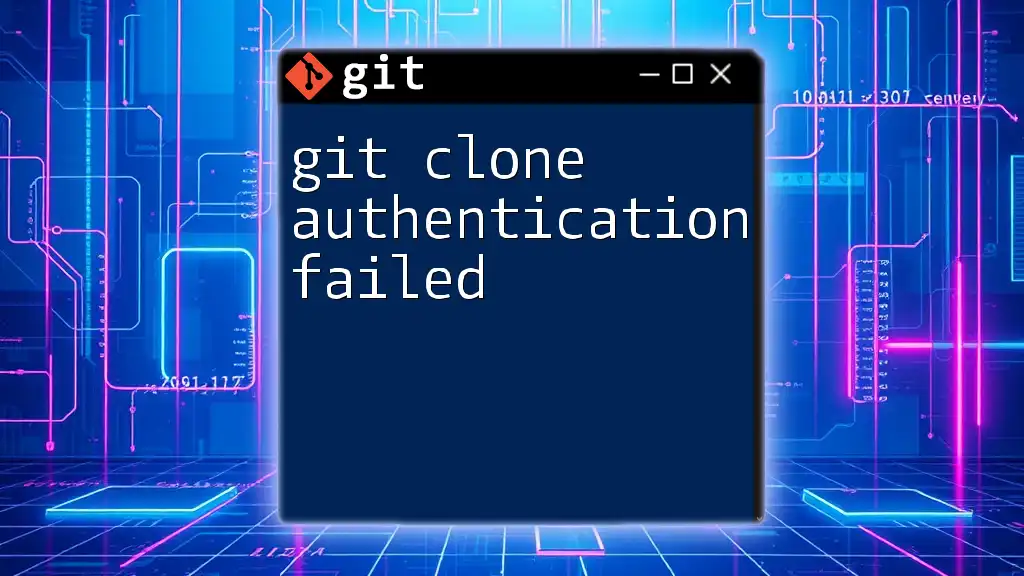
Troubleshooting Common Issues
Authentication Errors
If you encounter authentication errors after attempting to re-authenticate, take note of the specific message. Common reminders to pay attention to include "Permission denied" or "Authentication failed." Address these by ensuring you have the correct access and that your key or password is accurate.
Verifying Your Configuration
If you suspect that your Git configuration may be incorrect, verify it by running:
git config -l
This command will show you your current Git configuration settings for user details, remote URLs, and credential helpers, allowing you to confirm that everything is set up correctly.
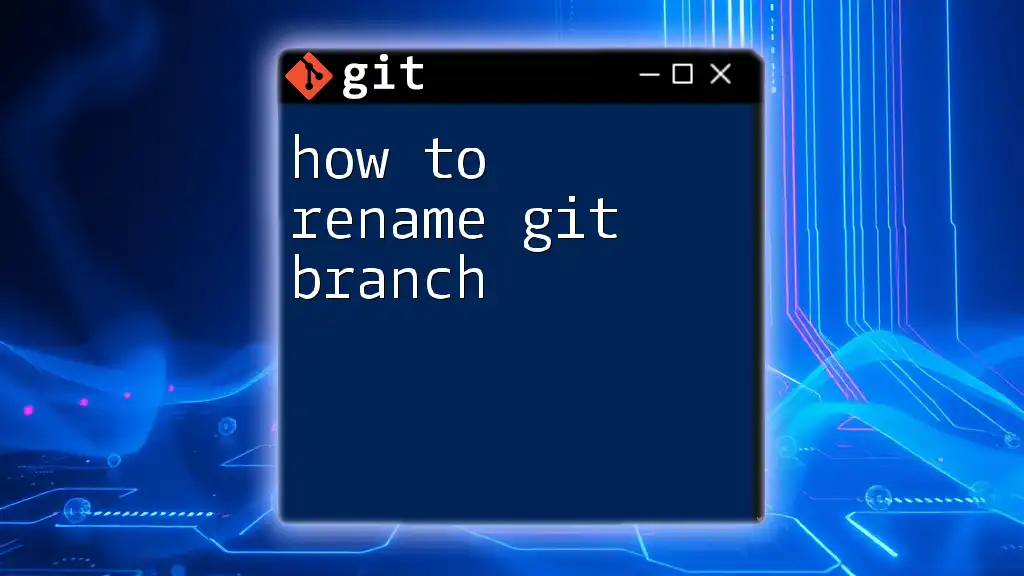
Best Practices for Managing Git Credentials
Using SSH vs. HTTPS
When deciding between SSH and HTTPS, consider your workflow and security requirements. SSH is generally recommended for its enhanced security and convenience. However, HTTPS may be necessary in restricted environments or when working with multiple users who may have varying SSH key access.
Keeping Your Credentials Secure
Avoid hardcoding credentials in your applications or scripts. Make use of secure password managers to store credentials and SSH keys safely. Regularly updating and reviewing your keys and passwords is another excellent practice to maintain account security.
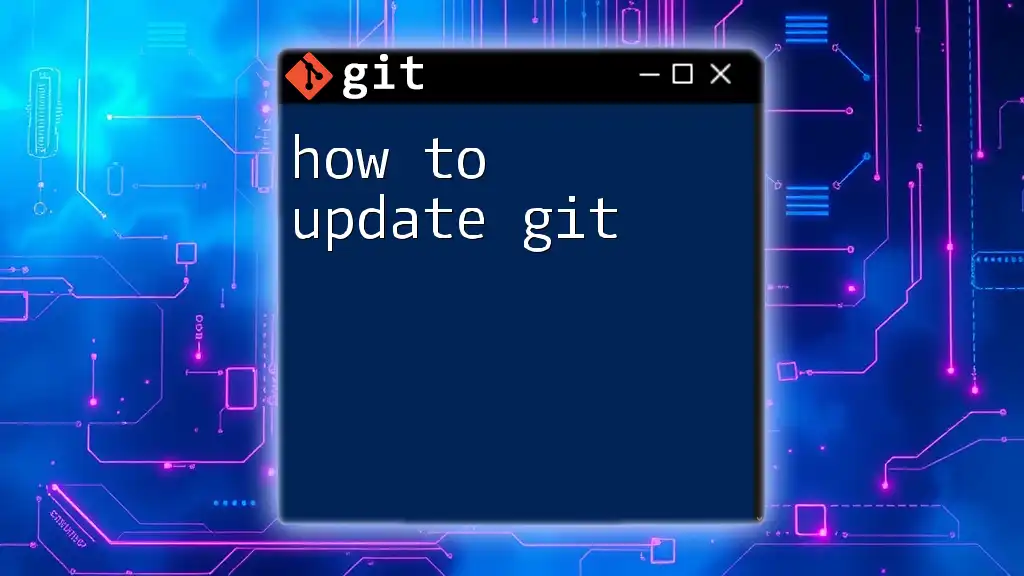
Conclusion
Re-authenticating Git on a Mac may initially seem challenging, but understanding your authentication method and the steps involved can simplify the process. By efficiently managing your Git credentials, you can maintain productivity while keeping your projects secure. Remember to stay updated on your organization's policies regarding credentials and security to avoid future access issues.
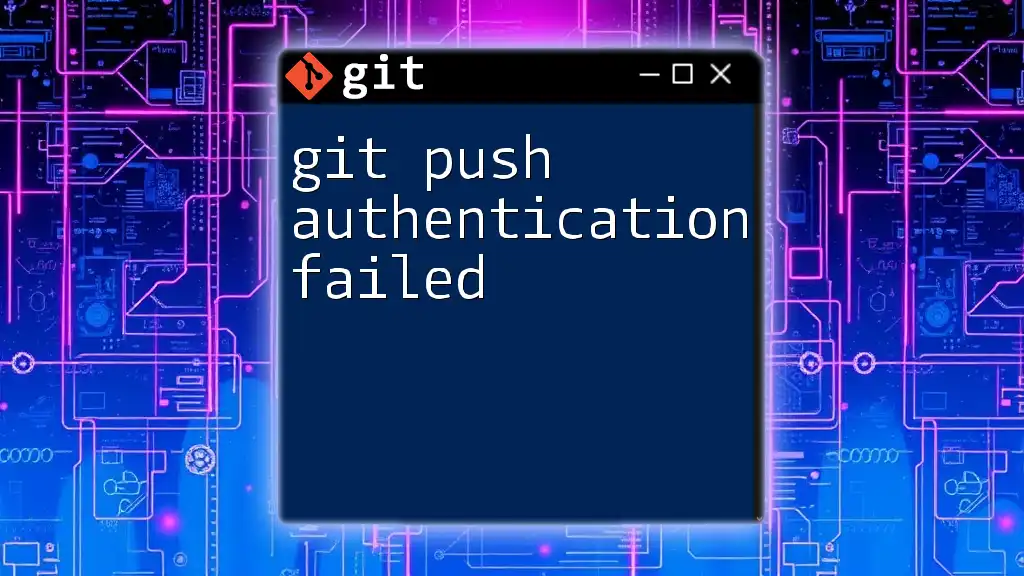
Additional Resources
For more in-depth tutorials and assistance, refer to:
- [Official Git documentation](https://git-scm.com/doc)
- [GitHub's guide to SSH](https://docs.github.com/en/authentication/connecting-to-github-with-ssh)
- [GitLab's documentation on SSH keys](https://docs.gitlab.com/ee/ssh/)