To automatically retry a Git command when it fails, you can wrap it in a loop that checks for success, as shown in the following example:
for i in {1..5}; do git fetch && break || sleep 5; done
Understanding Auto Retry in Git
What is Auto Retry?
Auto retry refers to the capability of a system to automatically attempt a command again if the initial execution fails. In the context of Git, this functionality becomes crucial, especially in a landscape where network issues or server downtimes can disrupt workflows. By implementing auto retry, developers can save significant time and effort, ensuring that their code pushes or pulls are successful without manual intervention.
Why Use Auto Retry?
Using auto retry can mitigate common frustrations that arise during version control operations. Here are some important points to consider:
- Network Failures: Network connectivity issues can cause Git commands, particularly push and fetch, to fail intermittently.
- Large Repositories: For repositories containing a vast amount of data, operations may take longer, causing timeouts.
- Efficiency: On top of saving time, auto retry allows developers to focus on actual coding rather than repeatedly executing the same commands.
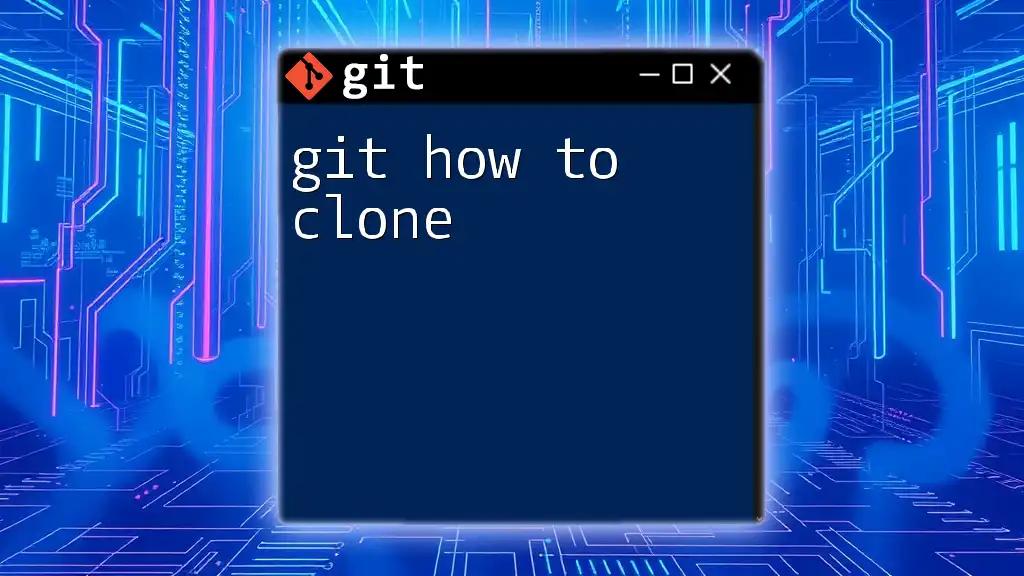
Getting Started with Git Auto Retry
Prerequisites
Before diving into auto retry configurations, ensure you have:
- Git installed on your system: You can download it from the official [Git website](https://git-scm.com/).
- Basic command-line knowledge to execute the necessary commands and scripts.
Configure Git for Auto Retry
Setting Up Your Environment
To leverage auto retry effectively, start by setting up your environment. Make sure your Git configuration is optimal for your workflow, particularly the remote repository settings and network configurations.
Implementing Auto Retry in Git Commands
Certain Git commands are more prone to failures and benefit greatly from an auto-retry mechanism. These include commands like:
- git push – for pushing changes to a remote repository.
- git fetch – for fetching updates from a remote repository.
Configuring these commands to retry automatically can significantly reduce workflow interruptions.
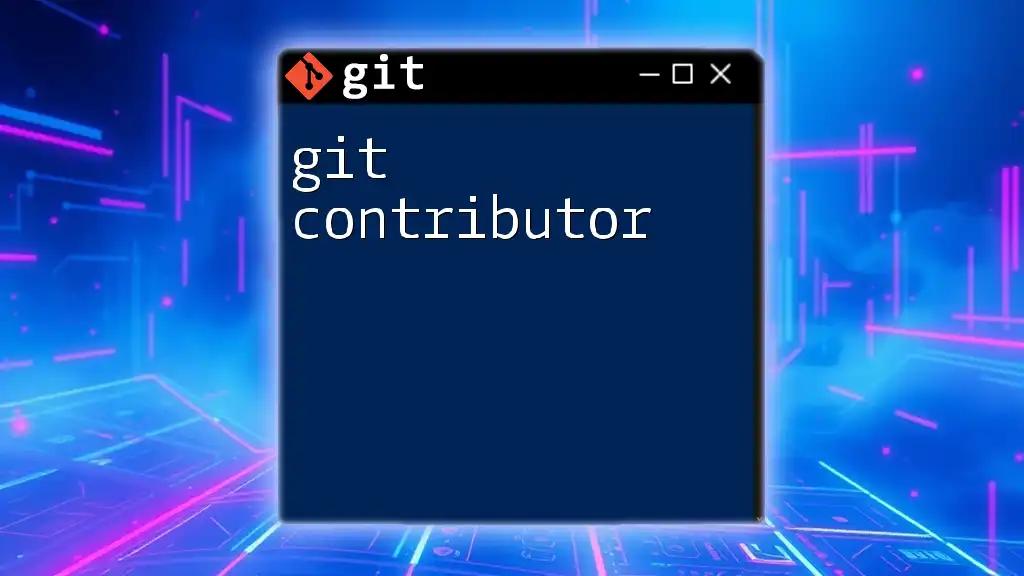
Auto Retry Mechanisms
Using Git's Built-In Auto Retry
While Git does not have an explicit built-in auto retry feature for all commands, it does allow retries in specific operations. For instance, when you configure a remote with SSH and use an internal retry mechanism via the `GIT_SSH_COMMAND` variable, you can achieve some level of auto retry.
Custom Auto Retry Scripts
Writing a Simple Bash Script
Creating a bash script can enable auto-retry functionality for any Git command. Below is a simple script that retries a `git push` command:
#!/bin/bash
max_retries=5
count=0
while true; do
git push && break
count=$((count + 1))
if [ "$count" -ge "$max_retries" ]; then
echo "Max retries reached. Exiting."
exit 1
fi
echo "Retrying... ($count)"
sleep 2
done
In this script, if the `git push` command fails, it will retry up to five times, waiting two seconds between each attempt. This script effectively reduces manual retries, making the process smoother.
Advanced Auto Retry with More Features
You can enhance the previous script to log each retry attempt. This not only helps in tracking which attempts succeeded or failed but also aids in debugging. Here’s a more advanced script:
#!/bin/bash
log_file="retry_log.txt"
max_retries=5
count=0
while true; do
git push && break
count=$((count + 1))
echo "$(date +'%Y-%m-%d %H:%M:%S') - Retry attempt $count" >> $log_file
if [ "$count" -ge "$max_retries" ]; then
echo "Max retries reached. Exiting."
exit 1
fi
sleep 2
done
In this script, each failure attempt is logged in a text file with a timestamp, allowing developers to review their push attempts later.
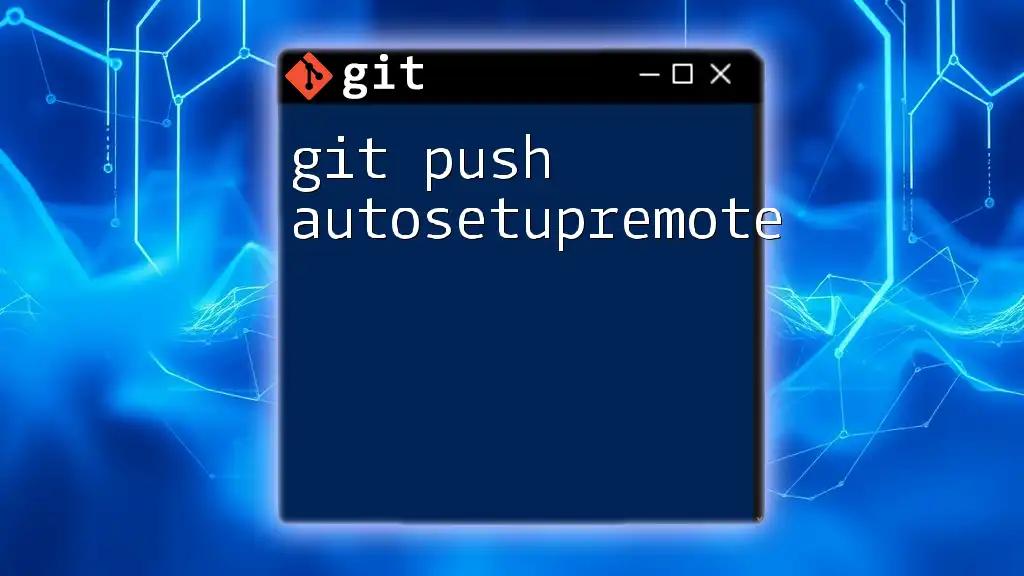
Best Practices for Using Auto Retry
Testing Your Scripts
It’s crucial to test your auto-retry scripts before deploying them in a production environment. This will ensure that they work as expected and handle errors gracefully. Consider setting up a small local Git repository where you can safely test different scenarios.
Maintaining Performance
While implementing auto-retry mechanisms can enhance productivity, it's important to be mindful of system performance. Here are some guidelines:
- Avoid excessively high retry limits and very short sleep intervals as they might lead to performance degradation.
- Ensure these scripts do not overwhelm your remote server or trigger rate limits.
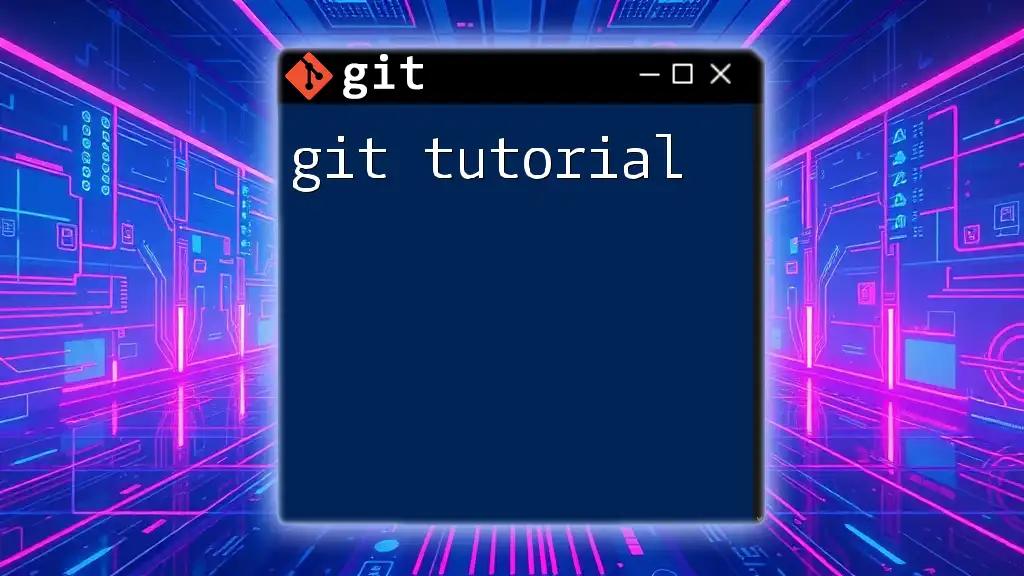
Troubleshooting Common Issues
Identifying Errors
In case your auto-retry mechanism fails to work as intended, you should first identify the error messages displayed in the console. Common errors might include authentication failures, network timeouts, or remote server issues.
Optimizing Retry Parameters
Optimizing your retry logic can make a substantial difference. Consider adjusting the max retries and the sleep duration based on your specific environment and network conditions. For example:
- If you're pushing large files, a longer sleep might be warranted to give the server processing time.
- Monitor your log files to determine if you need to alter your retry strategy based on patterns of failure.
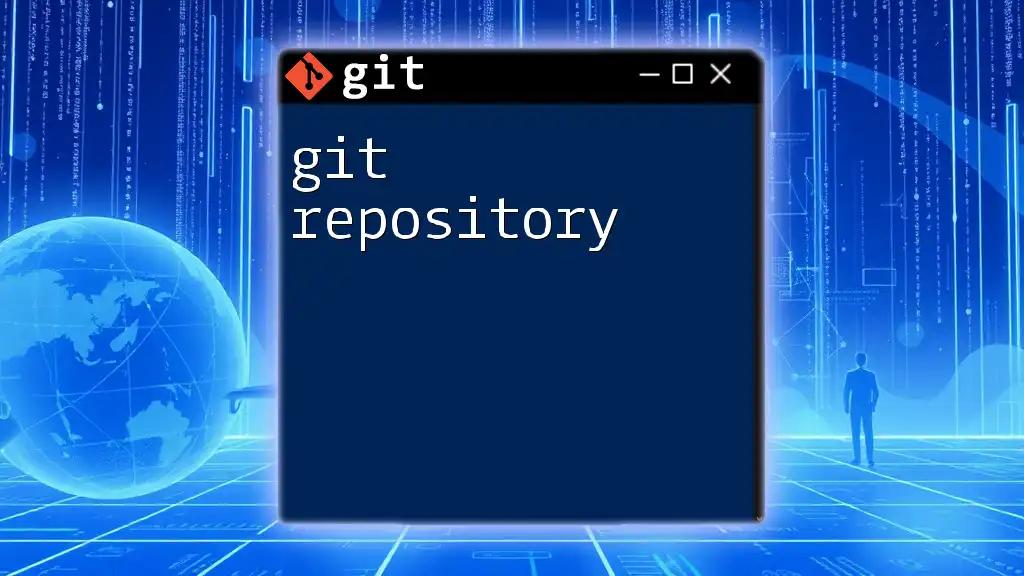
Conclusion
Implementing auto-retry functionality in Git not only smooths your workflow but also enhances efficiency and reliability. By experimenting with custom scripts and fine-tuning your settings, you can create a robust solution tailored to your team's needs. Don't hesitate to share your experiences or develop your version of auto-retry mechanisms, as each team's requirements may differ.