To add a folder structure to your Git repository, you can use the `git add` command followed by the path to the folder, which stages all the files within that directory for the next commit.
git add path/to/your/folder/
Understanding Git Basics
What is Git?
Git is a distributed version control system designed to track changes in source code during software development. Git allows multiple developers to work on a project simultaneously, manage versions, and coordinate their changes. Its importance lies in its ability to maintain history, enabling developers to review and revert to previous states if necessary.
The Role of 'git add'
The `git add` command is a crucial part of the Git workflow, responsible for adding changes from the working directory to the staging area. Staging your changes is essential because it allows you to prepare and review what will be committed to the repository. Without `git add`, changes would remain unrecorded and untracked.
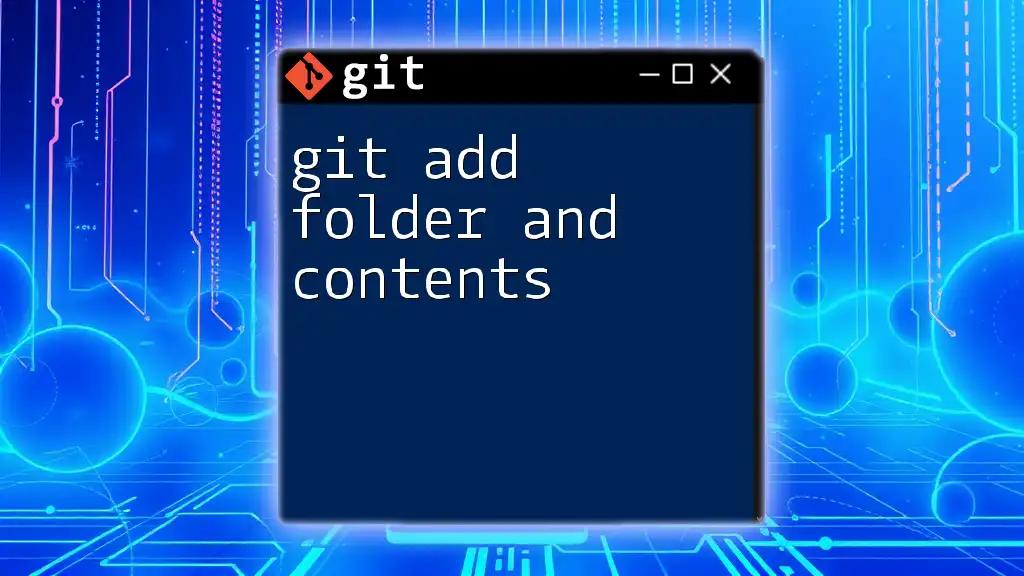
Setting Up Your Git Environment
Installing Git
To begin using Git, you first need to install it. The installation process varies depending on your operating system:
-
Windows: Download and run the installer from the [official Git website](https://git-scm.com/).
-
macOS: You can install Git via Homebrew using the command:
brew install git
-
Linux: Install via your package manager; for example, on Debian-based systems:
sudo apt-get install git
Ensure you check the [official Git documentation](https://git-scm.com/doc) for more detailed installation steps.
Initializing a Git Repository
Once Git is installed, you'll want to initialize a repository. This can be a local repository or linked to a remote server.
To create a new Git repository, navigate to your project directory and use the following command:
git init my-repo
This command creates a new hidden directory named `.git`, where all your version history and configurations will be stored.
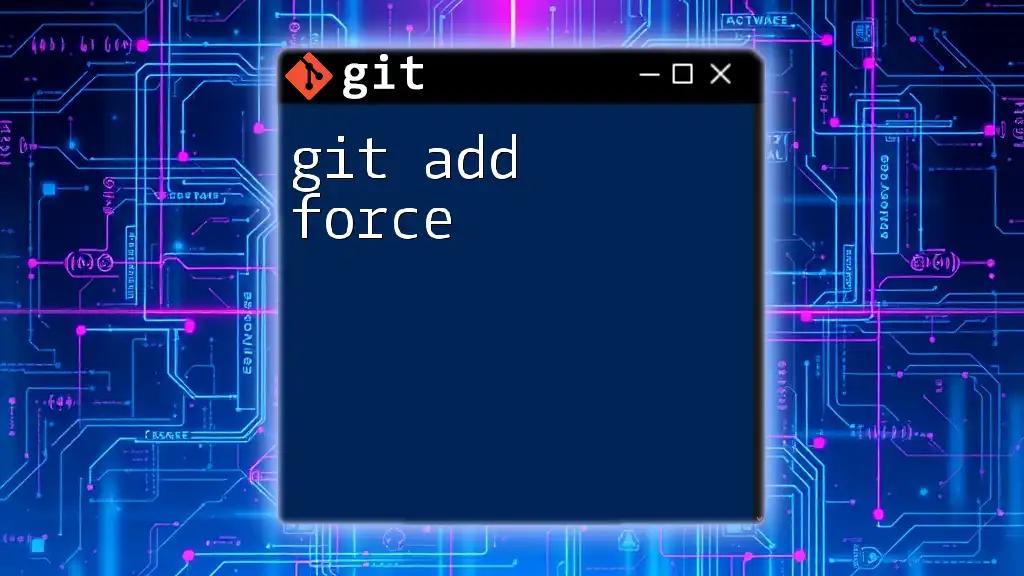
Creating Your Folder Structure
The Basics of a Folder Structure
A well-thought-out folder structure lays the groundwork for an organized project. An effective setup not only aids in clarity but also improves collaboration. A typical Git project structure might include:
- `/src`: for source files
- `/tests`: for test cases
- `/docs`: for documentation
This structure promotes separation of concerns, making it easier to manage various aspects of your projects.
Creating a Folder Structure
With your repository initialized, you can create the necessary folders directly from the command line. Here’s how:
mkdir src tests docs
Creating directories in this way helps maintain a clean and organized project from the outset. Establishing clear naming conventions during this phase will save time and avoid confusion later on.
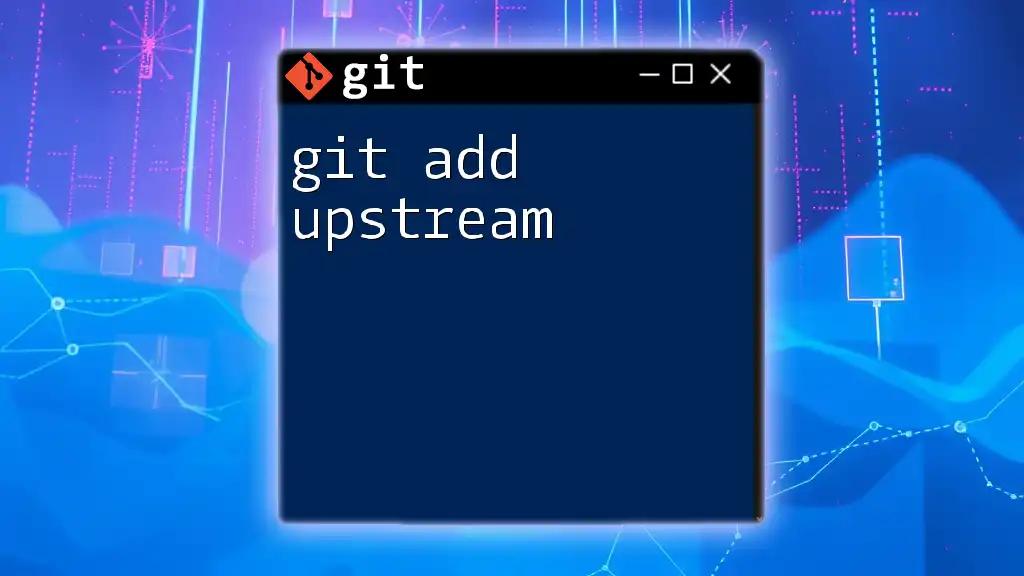
Adding the Folder Structure to Git
Using 'git add' for Entire Folders
Now that your folder structure is set up, it’s time to stage it with `git add`. To add an entire folder, simply use the following command:
git add src/
Using this command will stage all files within the `src` folder. Note that this includes any subfolders and files it contains, making it an efficient way to stage your changes.
Staging Specific Files within a Folder
Sometimes, you may want to stage specific files instead of the entire folder. You can do this using wildcards to match file types:
git add src/*.js
This command adds all JavaScript files from the `src` folder to the staging area. The asterisk (`*`) serves as a wildcard symbol that matches zero or more characters, thus enhancing your flexibility in staging files.
Verifying What You’ve Added
After staging your files, it’s crucial to check what has been added to the staging area. You can accomplish this using the `git status` command:
git status
This command provides an overview of your current repository state, highlighting both staged and unstaged files. It's an excellent tool for ensuring that you are ready to commit only the intended changes.
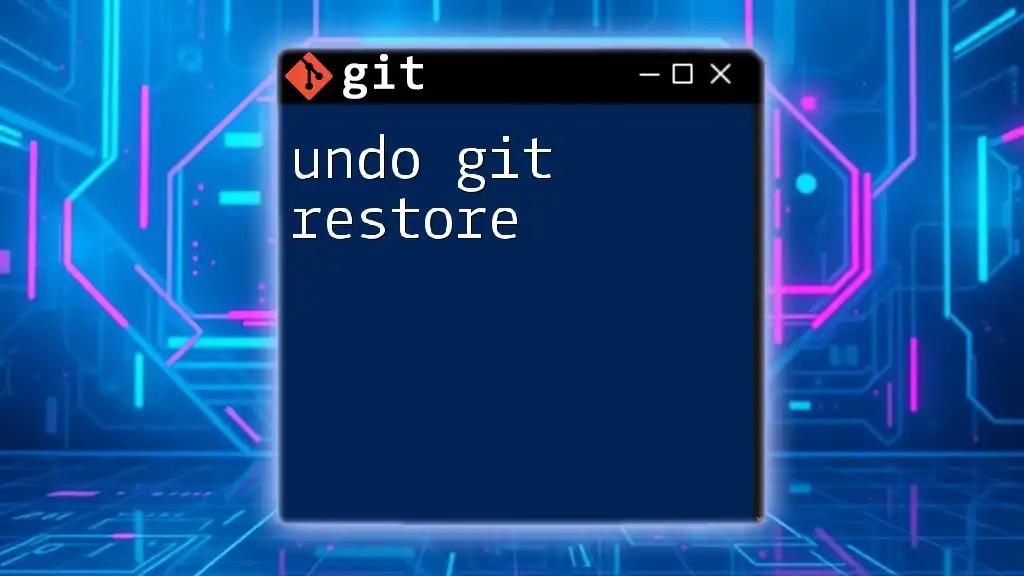
Ignoring Unwanted Files
Creating a .gitignore File
In nearly every project, there are files and directories you want Git to ignore, such as compiled files, logs, or sensitive information. To manage this, you can create a `.gitignore` file.
The `.gitignore` file allows you to specify patterns for files and directories that should be disregarded by Git. Here’s how you might set it up:
# Ignore node_modules
node_modules/
# Ignore log files
*.log
Creating and populating this file will help you keep your repository clean from unnecessary files.
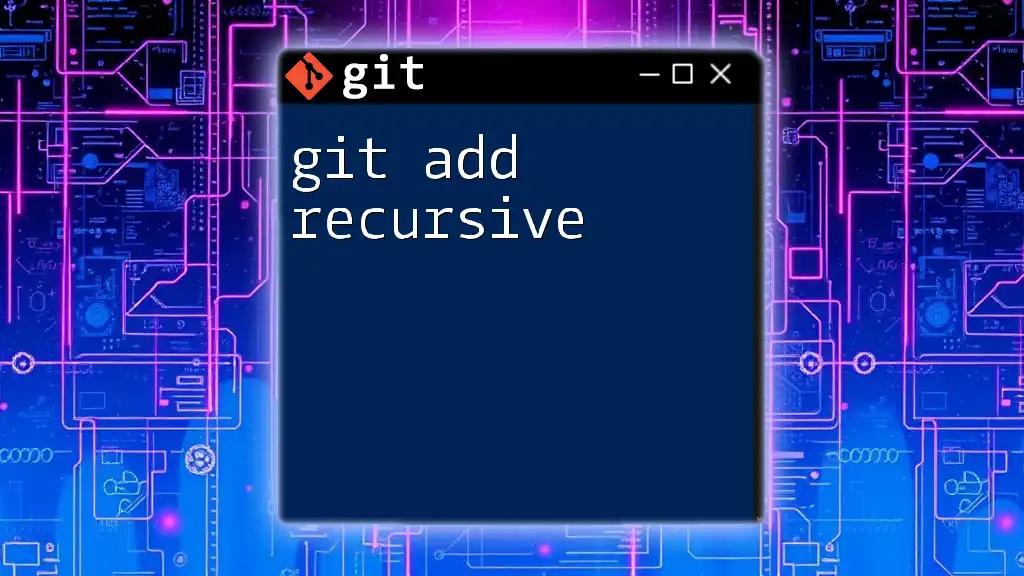
Committing Your Changes
The Importance of Committing
Committing is the process of saving changes in the version control system. It solidifies the changes made in the staging area to the repository’s history. This is crucial for tracking project evolution over time and collaborating with others.
How to Commit After Adding a Folder Structure
After ensuring your changes are staged, commit them to your repository with a clear message. Use the following command:
git commit -m "Added initial folder structure"
Writing meaningful commit messages is vital, as they provide context for the changes made, aiding both yourself and others in understanding the project history.
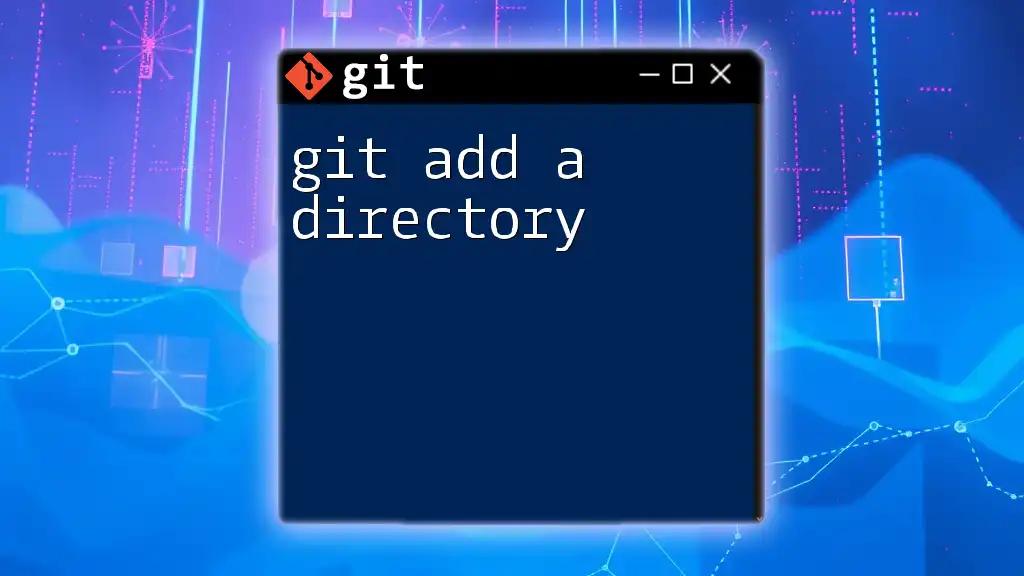
Best Practices for Folder Structures in Git
Consistency is Key
Consistency in naming and organizing your folder structure is essential. This practice not only enhances readability but also facilitates teamwork and minimizes errors during collaboration.
Keeping It Clean
A well-organized folder structure contributes significantly to project management. Here are some tips to maintain an efficient folder structure:
- Regularly review your folders and files to ensure they remain relevant.
- Group similar files together to simplify navigation.
- Document the folder structure in a README file to help guide new contributors.
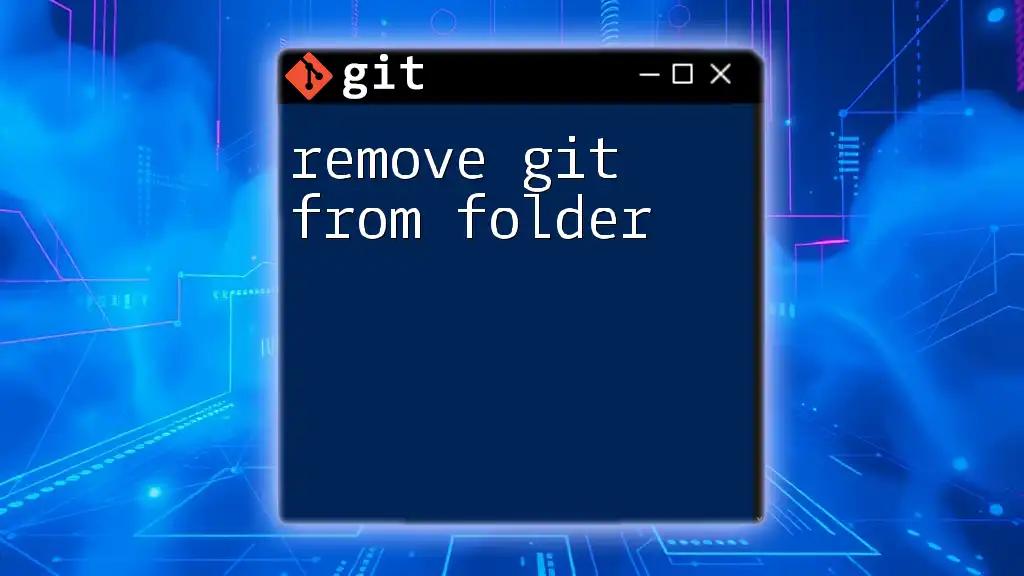
Advanced Techniques
Using Git Hooks
Git hooks are scripts that Git executes before or after events such as committing or merging. Although they may not directly relate to folder structure, they can automate tasks, improve workflows, and add layers of validation to your Git processes.
Automating the Folder Structure Creation
For larger projects, or when starting new ones, automating the creation of a folder structure can save time. Below is a simple script snippet to create a common folder structure quickly:
#!/bin/bash
mkdir -p src/{components,containers,utils} tests docs
Running this script would automatically create a predefined folder structure, promoting consistency across multiple projects.
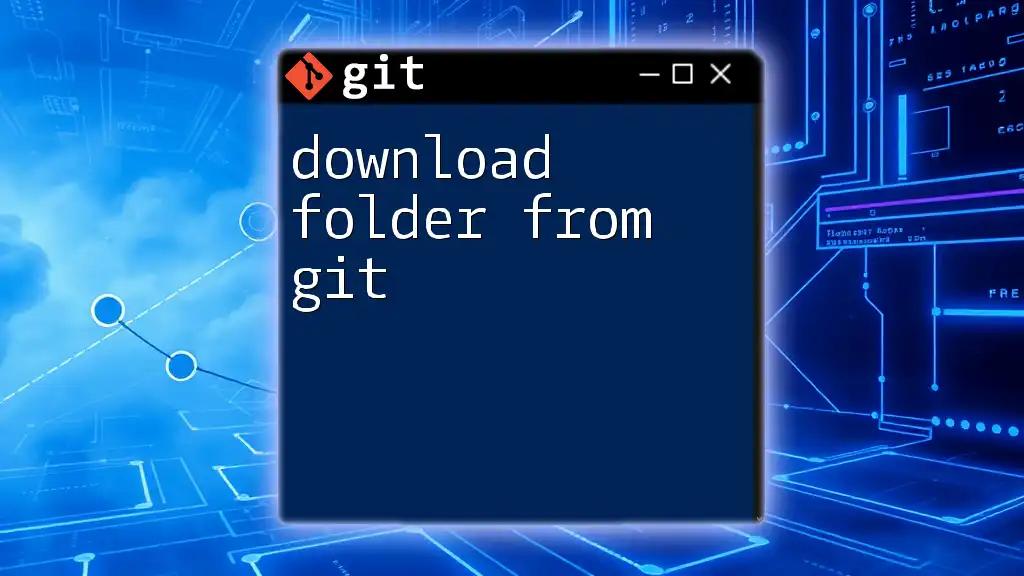
Conclusion
Understanding how to effectively use the `git add` command with your folder structure is pivotal in Git version control. By following the strategies outlined in this guide, you can enhance your project organization, improve collaboration, and ensure a smoother workflow. Whether you are a novice or an experienced developer, mastering these techniques will serve you well as you manage your projects.
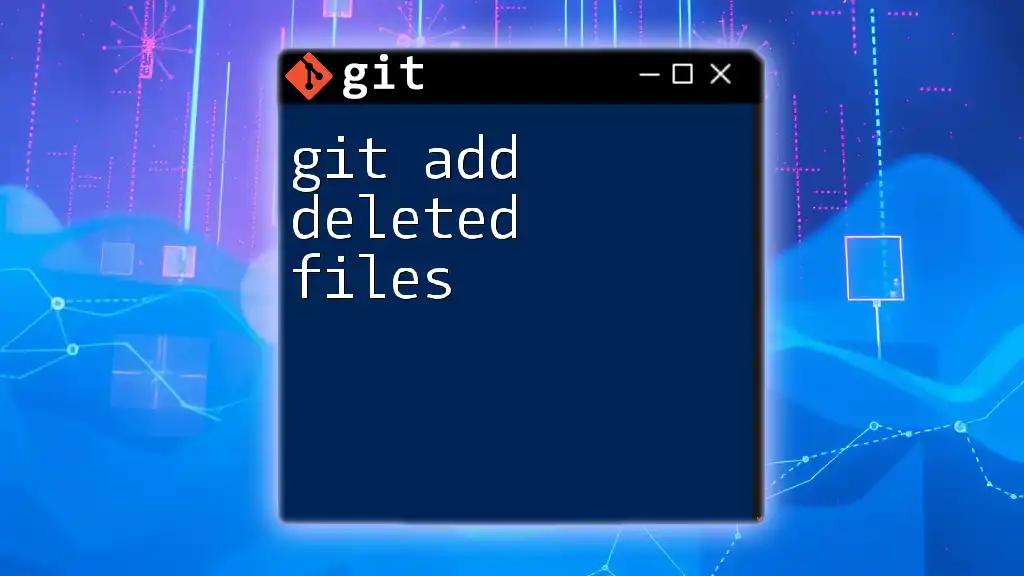
Additional Resources
For those looking to deepen their understanding of Git, consider exploring the [official Git documentation](https://git-scm.com/doc) and checking out recommended books or online courses. Engaging with community forums can also provide additional support as you learn.
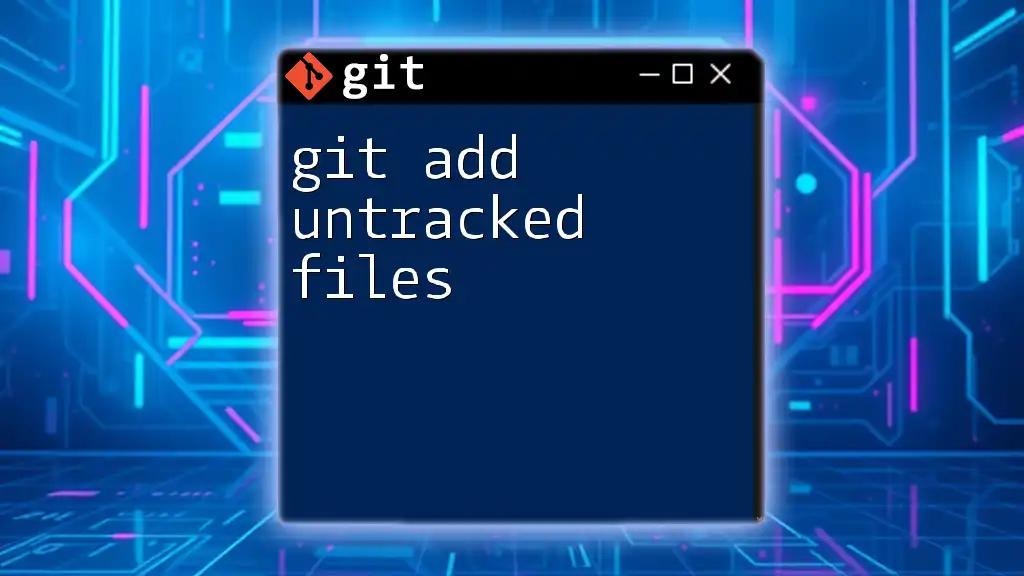
Call to Action
Practice applying the techniques covered in this article! Experiment with different folder structures using the `git add` command, and feel free to share your experiences or questions in the comments section. Happy coding!