The command `git add --force` allows you to stage files that are otherwise ignored by Git due to rules specified in the `.gitignore` file.
git add --force path/to/ignored-file.txt
Understanding Git Add Force
What is Git?
Git is a distributed version control system that helps developers manage changes to their code while collaborating with others. It allows teams to track modifications, revert to previous stages, and branch off for experimental projects. By utilizing version control, developers can maintain the integrity and history of their codebase, facilitating a smoother workflow and enhanced teamwork.
The Basics of Git Add
The `git add` command is an essential part of the Git workflow. It allows you to stage changes in your project, preparing them for commit. When you execute `git add`, you’re essentially telling Git, “I want to include these changes in my next snapshot of the project.”
Staging changes is crucial because it allows you to select specific updates that you want to record without affecting other parts of your work. For example, you might have modifications in several files but only want to commit changes made to one file first.
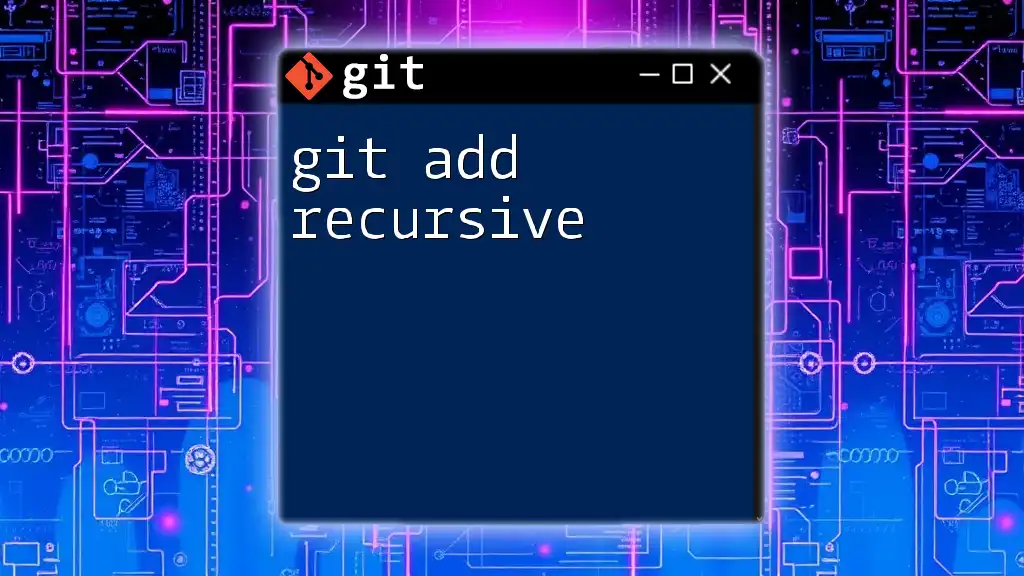
What is Git Add Force?
Definition of Git Add Force
The `git add --force` command is a powerful option that allows you to override Git's standard behavior regarding the staging of files. Typically, Git respects `.gitignore` files, which are used to specify files and directories that should not be tracked. Using the `--force` option, you instruct Git to ignore these rules and stage a file that would otherwise be ignored.
How to Use Git Add Force
The syntax for the `git add --force` command is straightforward:
git add --force <file>
You simply replace `<file>` with the actual file name you wish to stage, regardless of whether it is listed in your `.gitignore`.
Common Scenarios for Using Git Add Force
-
Overriding Files Without Git Tracking: There might be times when you need to include a file that was added to your `.gitignore` for some reason. Perhaps the file is essential for a temporary purpose, such as debugging or troubleshooting.
-
Dealing with .gitignore Files: If you are dealing with dynamically generated files or specific configurations that you initially decided to ignore, you can force Git to monitor these changes using `git add --force`.
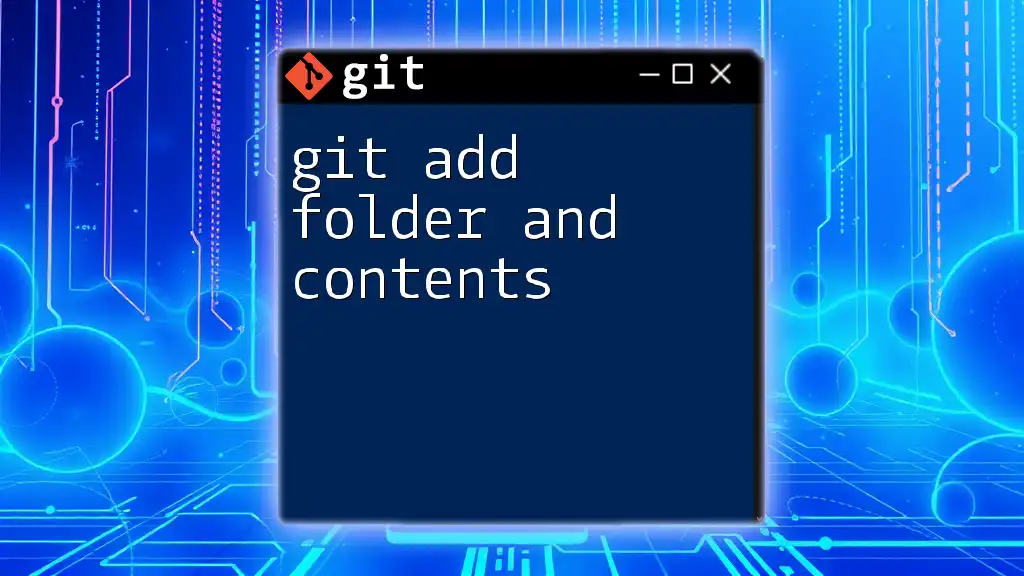
Risks and Considerations of Using Git Add Force
Potential Issues
While `git add --force` can be incredibly useful, it is essential to be cautious. Using this command carelessly can lead to unintended consequences, such as accidentally staging sensitive information or overwriting vital changes.
It’s crucial to understand the implications of using this command. Since it allows you to bypass Git’s tracking mechanisms, make sure you are aware of what you are including in your commits.
Error Handling
Encountering errors while using `git add --force` can happen. Some common error messages include:
- "fatal: pathspec '<file>' did not match any files" – This indicates that the specified file does not exist in the working directory.
- "fatal: unable to process path" – This suggests that there might be permission issues or the file is locked.
If you encounter these messages, double-check your spellings, ensure the files exist, and verify that you have the necessary permissions to access those files.
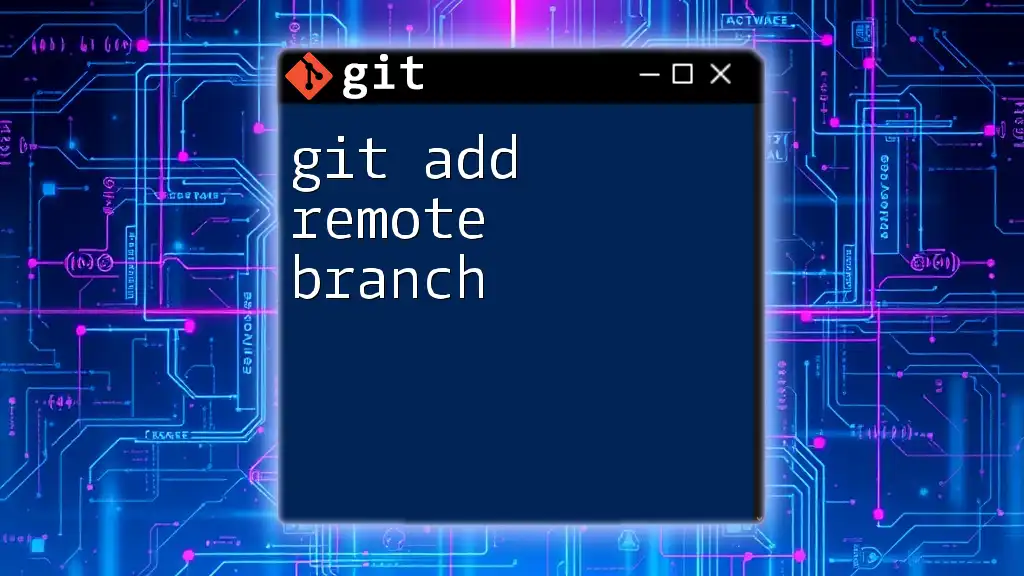
Practical Examples of Git Add Force
Example 1: Adding Ignored Files
To illustrate the application of `git add --force`, let's consider a situation where you want to add a file that is currently ignored by Git.
-
Create and Ignore a File: You created a file named `secret.txt` and added it to your `.gitignore` so it wouldn’t be tracked.
echo "secret" > secret.txt echo "secret.txt" >> .gitignore
-
Use `git add --force` to Add It: Now you decide you want to track this file after all.
git add --force secret.txt
By executing this command, you’ve overridden the `.gitignore` settings and staged `secret.txt`.
Example 2: Overwriting Local Changes
In another scenario, maybe you have modified a file, but you want to force certain changes to be staged.
-
Modify a File: You first save a change in `file.txt`.
echo "change 1" > file.txt git add file.txt
-
Alter the Same File Later: Later, you might append another change.
echo "change 2" >> file.txt
-
Use `git add --force` to Stage the New Change: To make sure this change gets included as well:
git add --force file.txt
By forcing the add, the new changes are prioritized and staged correctly.
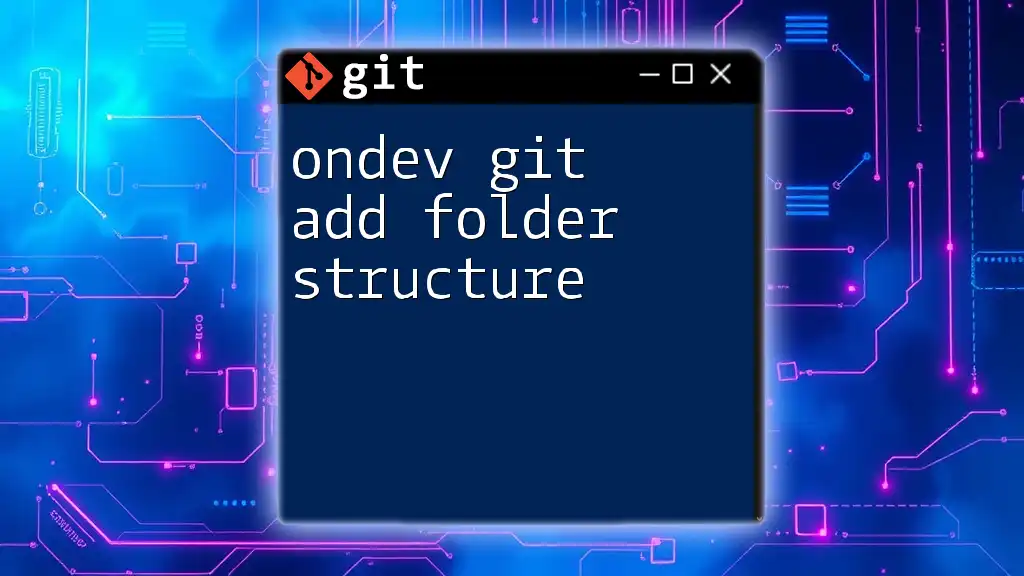
Best Practices When Using Git Add Force
When Not to Use Git Add Force
While the `git add --force` command can be handy, there are situations where it’s better not to use it. For example, if a file is in your `.gitignore` for a legitimate reason (like sensitive data or build artifacts), overriding Git’s ignore settings can lead to safety and privacy issues.
Combining Git Add Force with Other Commands
After staging files with `git add --force`, the next logical command is `git commit`, which saves your changes.
A typical workflow might look like this:
git commit -m "Added ignored file"
This command commits the changes staged, ensuring that any modifications — even those typically ignored — are now part of your repository history.
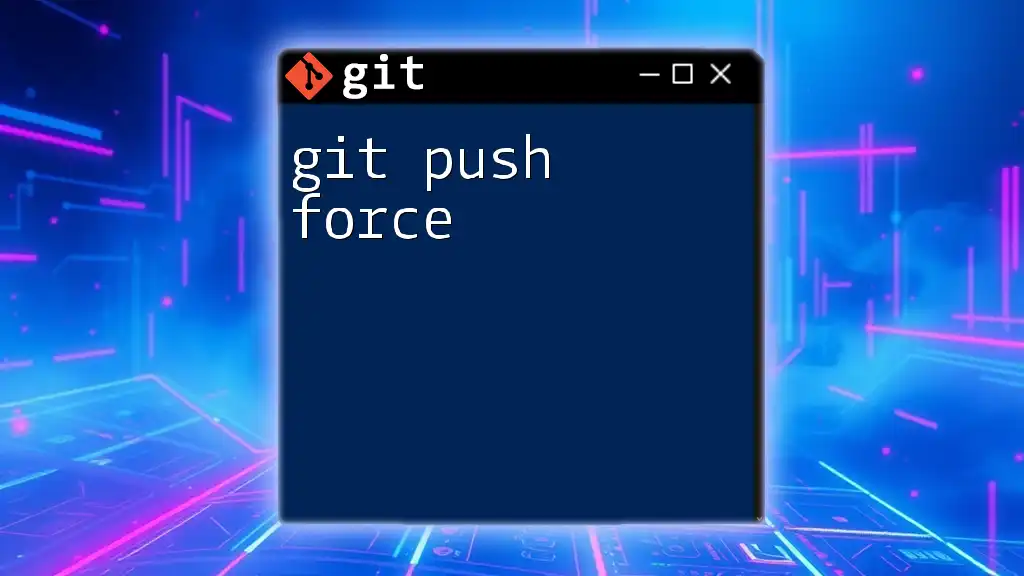
Conclusion
Summary of Key Points
Understanding the implications and use cases of the `git add --force` command is vital for effective Git management. While it grants flexibility, it also necessitates caution to prevent unintended consequences. Familiarizing yourself with this command enhances your Git skills, making it easier to navigate your version control tasks.
Additional Resources
For further exploration of Git and version control best practices, consider checking online tutorials, official documentation, and community forums. Mastery of these tools can significantly enhance your software development workflow.