To add all changes in your Git repository except for one specific file, you can use the following command, which utilizes the `git add` command along with the `:` syntax to exclude the unwanted file.
git add . ':!filename.txt'
Understanding Git Staging Area
What is the Staging Area?
The staging area in Git, often referred to as the index, is a crucial part of the version control process. It acts as a middle ground between your working directory (where you make changes) and the repository (where your project's history is stored). When you modify files in your project, these changes reside in your working directory until you explicitly add them to the staging area using the `git add` command. Once staged, the changes are ready to be committed, allowing you to create a snapshot of your project at a specific point in time.
Why Selectively Add Files?
Selecting which files to stage can be incredibly beneficial for maintaining a clean commit history. There are various scenarios where you might want to exclude certain changes from being staged:
- Accidental modifications: You might inadvertently change files that should not be included in a particular commit.
- Separating concerns: Different features or bug fixes may be in progress simultaneously; keeping changes compartmentalized helps in understanding the commit history later.
- Collaboration clarity: A clear commit helps collaborators quickly understand the purpose behind a change.
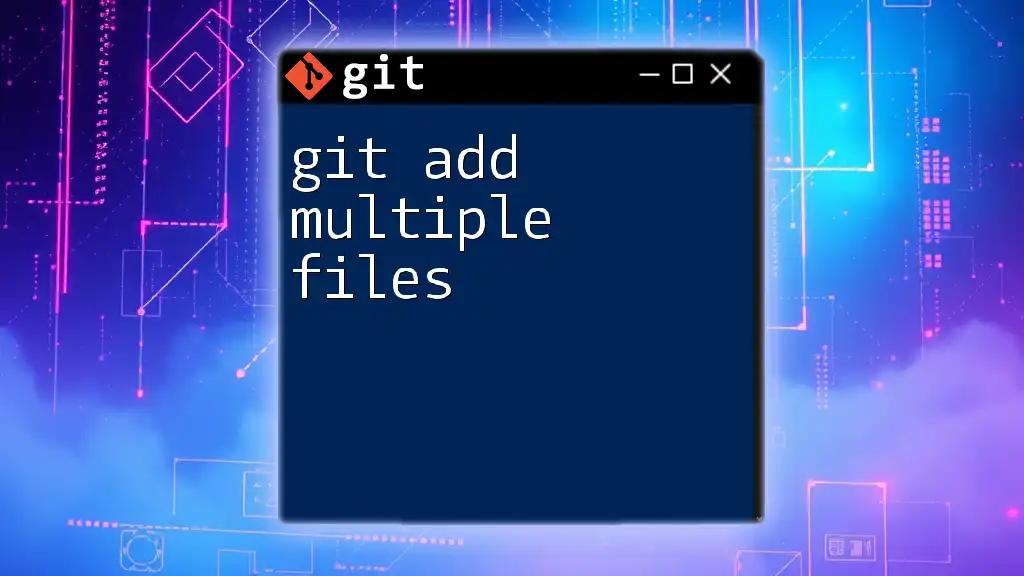
The Basic Git Add Command
Syntax of `git add`
The basic syntax of the `git add` command is straightforward:
git add <file-pattern>
This command tells Git to stage the specified files, preparing them for the next commit. A common use case is to stage everything at once using:
git add .
This command stages all modified files in the current directory and its subdirectories.
Example of Adding All Files
Using `git add .` is often the first command that new users learn, as it allows them to quickly include all changes. While this is convenient, it can lead to unwanted files being included in your commit if you're not careful about what changes you've made.
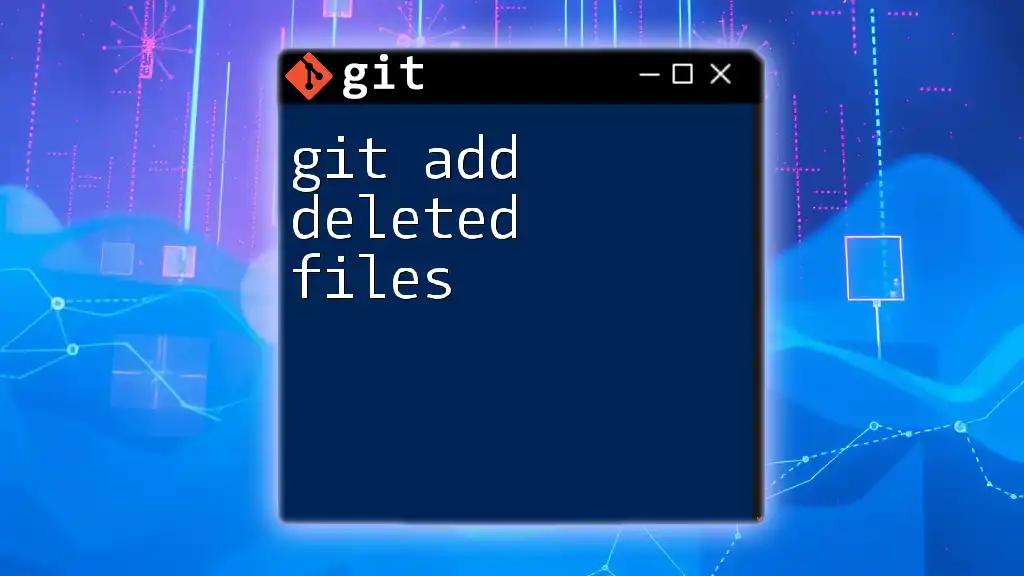
Excluding a Specific File
The Challenge of Excluding Files
Adding files selectively can sometimes be tricky. It’s easy to overlook files that should not be included in your commit, leading to unwanted noise in your project history. Thus, knowing how to exclude specific files becomes essential.
Utilizing Git’s Features
Fortunately, Git offers options for fine-tuning what gets added to the staging area, allowing you to include everything but certain files.
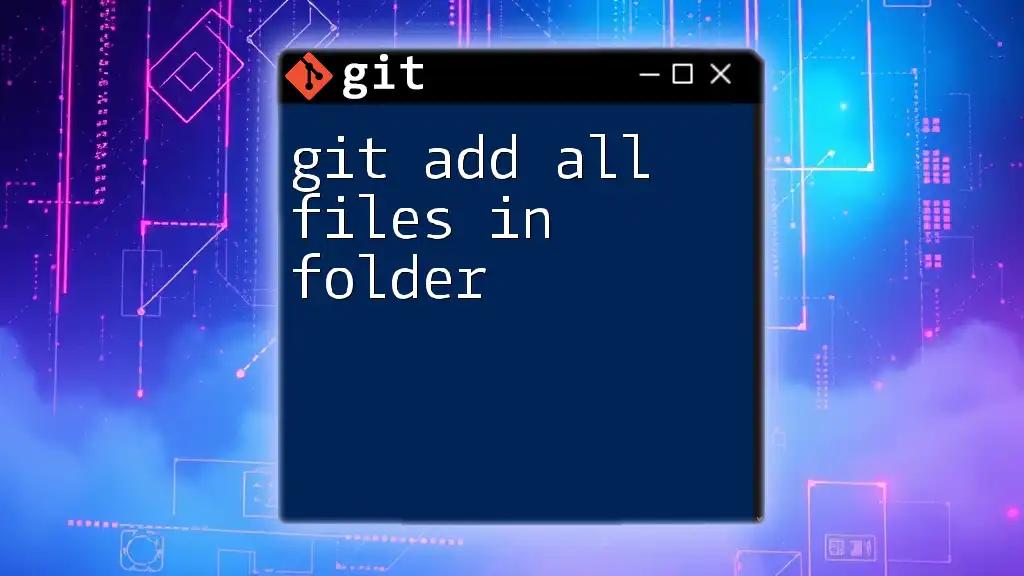
The Command to Add All Except One File
Using the `:` Option
To add all files while excluding one specific file, you can leverage the `:` notation. The command format looks like this:
git add --all :!unwanted-file.txt
In this example, all files are added to the staging area except for `unwanted-file.txt`. This method is incredibly useful when you need to stage your work but want to ensure certain files remain untouched for a particular commit.
Examples of Excluding Different File Types
Excluding a Specific File
Consider a situation where you’ve modified several files, but you do not want to include `config.json` in your commit. You can run:
git add --all :!config.json
This command allows all changes to be staged, excluding just the `config.json` file, ensuring that sensitive or environment-specific configurations are not accidentally shared.
Excluding a Directory
If you need to exclude an entire directory, the process is similarly straightforward. For instance, if there's a `logs/` directory you want to leave out, use:
git add --all :!logs/
This command stages all changes except those in the `logs/` directory, keeping your commit clean from log files.
Multiple Exclusions
You can exclude multiple files in one command as well. For example:
git add --all :!file1.txt :!file2.txt
This command will stage all changes while ensuring that both `file1.txt` and `file2.txt` remain untracked for that commit.
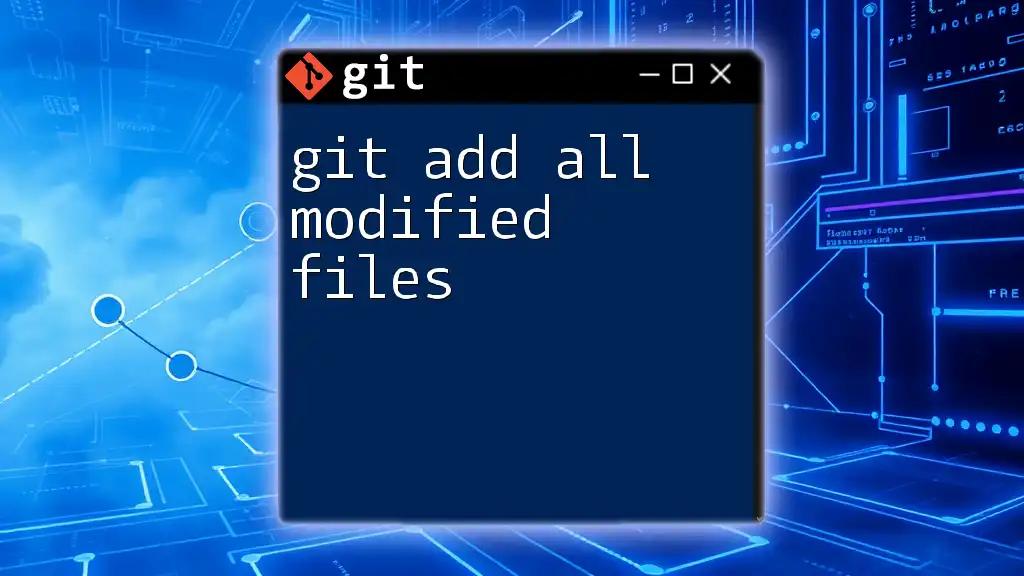
Alternative Methods for Excluding Files
Using `.gitignore`
Another approach for excluding files is through a `.gitignore` file. This file allows you to specify patterns of files that Git should ignore entirely. To add files to `.gitignore`, simply create or edit the file and include the file names or patterns you want to exclude:
# Ignore log files
logs/
# Ignore configuration files
config.json
Once these patterns are added to `.gitignore`, Git will never stage those files again unless explicitly told to do so.
Stashing Changes
If you have local modifications that you temporarily want to set aside, consider using the `git stash` command. This is especially helpful if you want a clean working directory without committing unfinished work. Use it as follows:
git stash
This command saves your modifications and allows you to return to a clean state and stage only what you need.
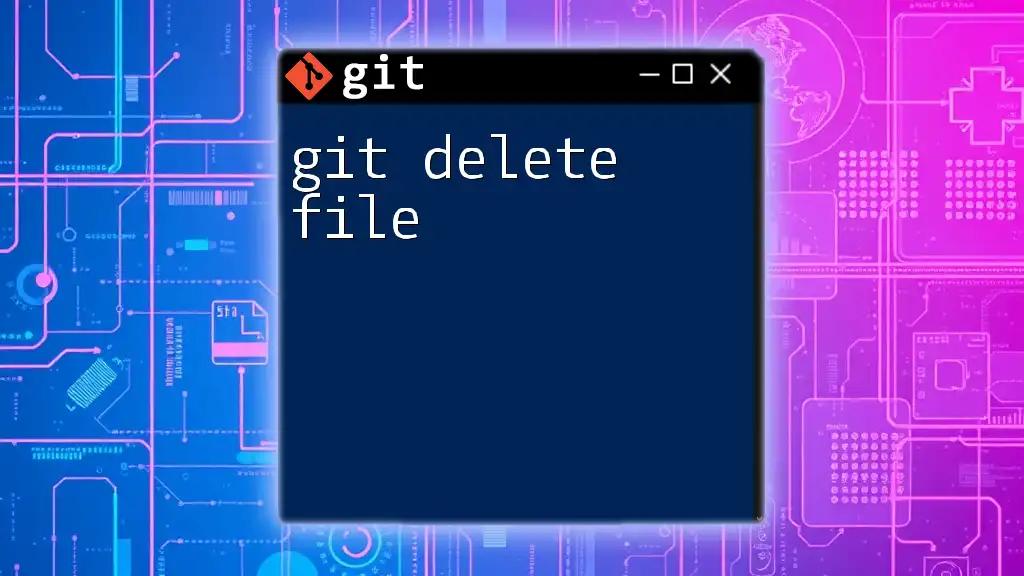
Real-World Scenarios
When to Use These Commands
Imagine you are developing a new feature while at the same time fixing bugs in another part of your project. In this case, you might make several changes across multiple files, but only a few are ready to be committed. Using the command:
git add --all :!unwanted-file.txt
becomes indispensable. You can easily add all necessary changes while leaving behind anything that isn’t ready for commit. This keeps your commit history organized and your project manageable.
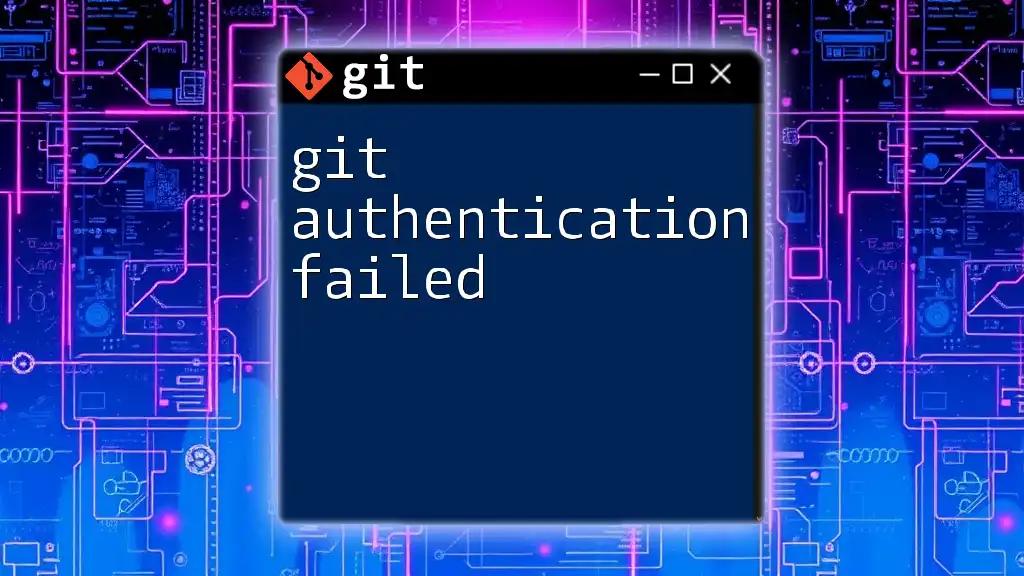
Conclusion
Being able to use the command git add all except one file effectively can significantly enhance your workflow in Git. By selectively staging changes, you maintain a cleaner, clearer project history, which is especially crucial in collaborative environments. Whether you opt for precise command-line methods or manage your exclusions through `.gitignore`, these practices will streamline your version control experience.
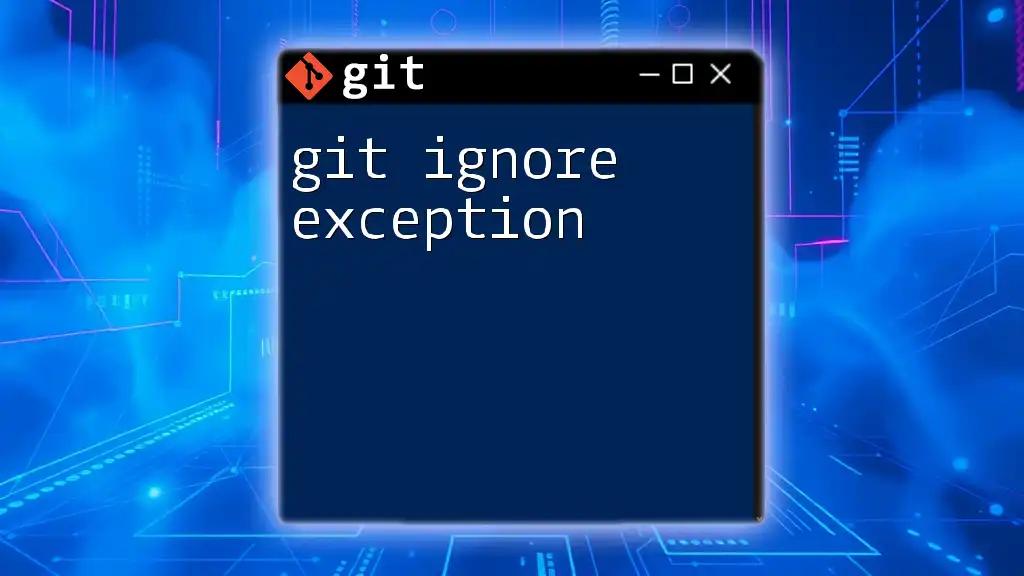
Additional Resources
For further reading and to refine your Git expertise, check out:
- The official [Git documentation](https://git-scm.com/doc).
- Recommended tutorials for practical applications and advanced features in Git.
Call to Action
Put these techniques into practice in your projects. Feel free to share your questions, challenges, or experiences related to staging files in Git in the comments section below!