To revert to a specific commit in Git and push the changes to the remote repository, use the `git revert` command followed by the commit hash, and then push the changes with `git push`.
Here's how you can do it in the command line:
git revert <commit-hash>
git push origin <branch-name>
Understanding Git Revert
What is Git Revert?
Git revert is a command that allows you to undo changes made by a previous commit without altering the commit history. This is particularly useful when you wish to remove specific changes while preserving the integrity of your project's logs. Unlike commands such as `git reset`, which can permanently erase commits, `git revert` safely creates a new commit that counteracts the specified one.
When to Use Git Revert
You should consider using `git revert` in scenarios where:
- You want to undo changes introduced by a commit that is already part of the project's published history.
- You need to maintain a clear record of all changes, ensuring that your project history remains intact.
- You are collaborating on a project, and removing commits could lead to confusion or issues for others.
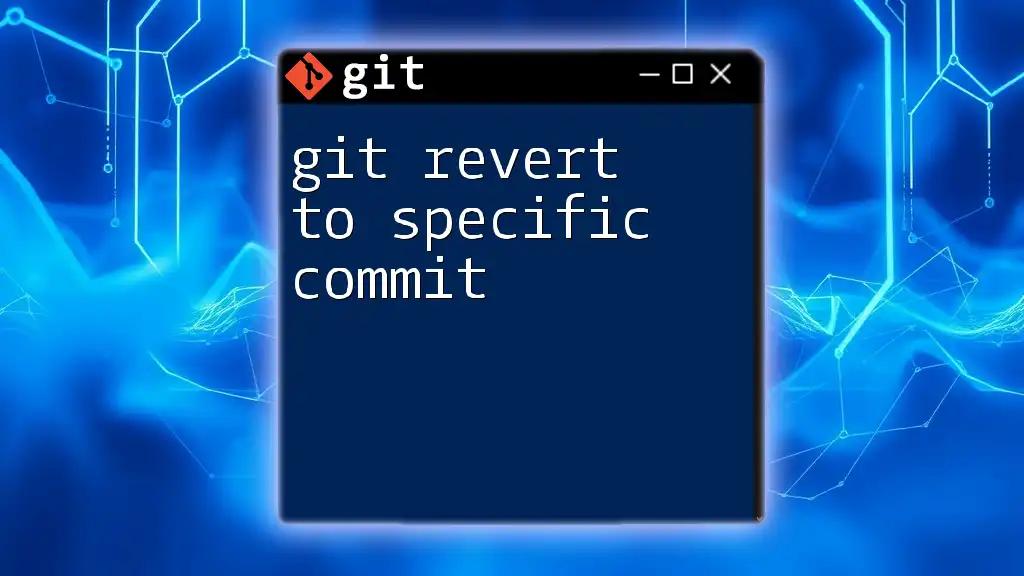
Finding the Commit to Revert
Using Git Log
To effectively revert to a specific commit, you first need to identify that commit. This is done using the `git log` command, which displays the commit history in a list format.
Run the command:
git log
The output will include the commit hashes, author names, dates, and commit messages. It’s crucial to carefully read through this list to find the exact commit you want to revert.
Identifying the Commit Hash
Once you have accessed the commit history, look for the desired commit's hash. A commit hash is a long string of characters that uniquely identifies each commit. Take your time reviewing the messages to ensure you choose the correct commit for your revert action.
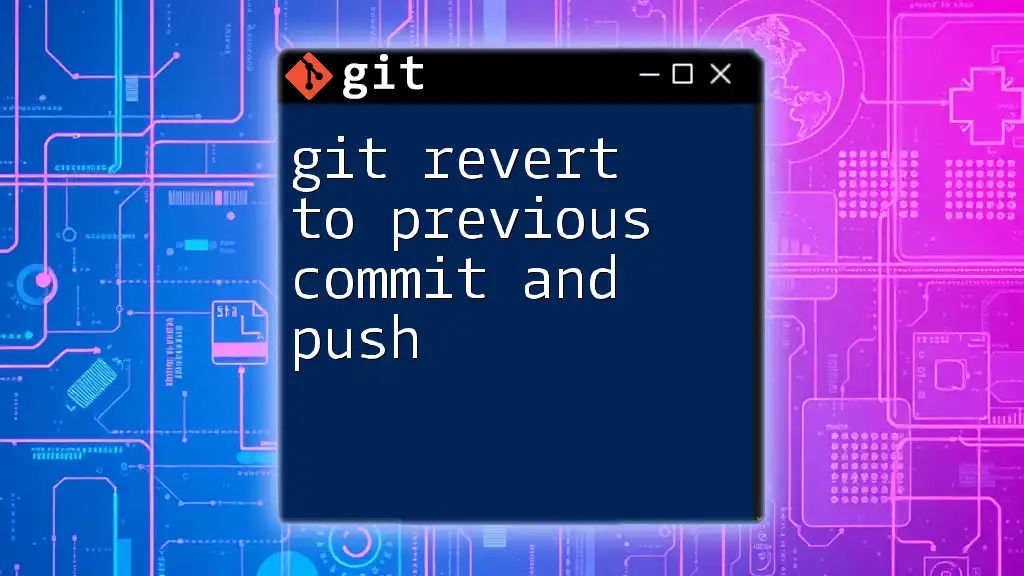
Performing Git Revert
The Basic Command
With the commit hash in hand, you can now execute the `git revert` command. The basic syntax is as follows:
git revert <commit-hash>
For example, if you want to revert a commit with the hash `abc1234`, you would use:
git revert abc1234
This command initiates the process of creating a new commit that undoes the changes made in `abc1234`.
Handling Conflicts
In some cases, you may run into conflicts if the changes from the commit you are reverting interact adversely with other changes in your code.
If you experience a conflict, Git will notify you, and you will need to resolve it manually. Review the conflicted files, make necessary adjustments, and then mark them as resolved by staging changes using:
git add <file>
Once you've resolved all conflicts, you can complete the revert process by committing your changes.
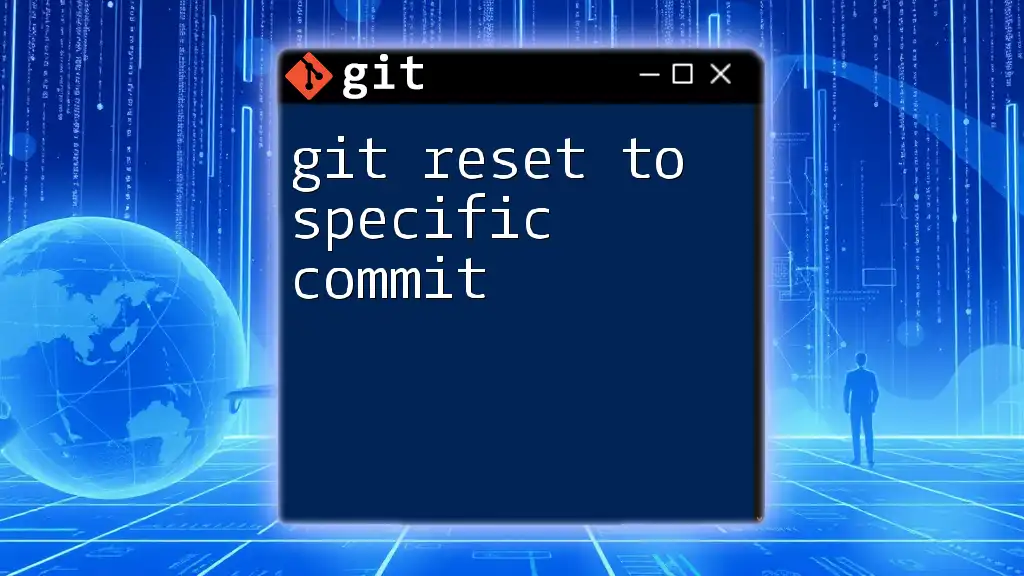
Reviewing Your Changes
Checking the Status
After performing the revert, it's crucial to check your repository's status to ensure everything is in order. Use:
git status
This will indicate whether you have any additional changes waiting to be committed or if the revert was successful.
Commit Message
When you execute `git revert`, Git automatically generates a default commit message that describes what it did (e.g., "Revert 'commit message here'"). However, you can customize this commit message if you want to provide more context regarding the revert action.
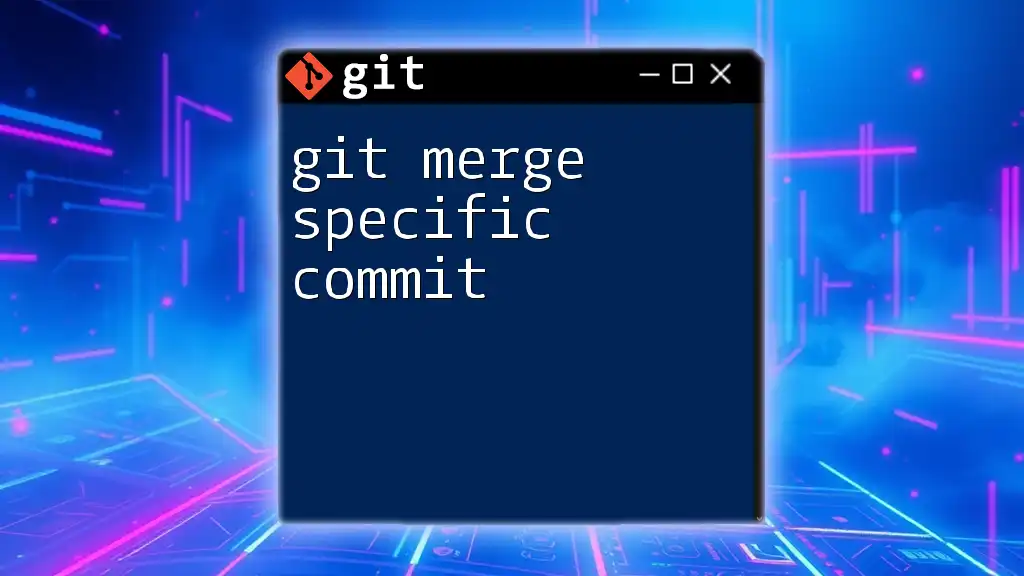
Pushing Changes to the Remote Repository
Using Git Push
After completing the revert and reviewing your changes, the next step is to push your modified commit to the remote repository. This ensures that all collaborators are updated with the latest changes.
The command for pushing to the origin is:
git push origin <branch-name>
Replace `<branch-name>` with the name of the branch you are working on, such as `main` or `develop`.
Example Command
Putting it all together, here’s a full example that encompasses the entire process:
git revert abc1234
git push origin main
This sequence of commands will revert the specified commit and push the updates to the main branch of your remote repository.
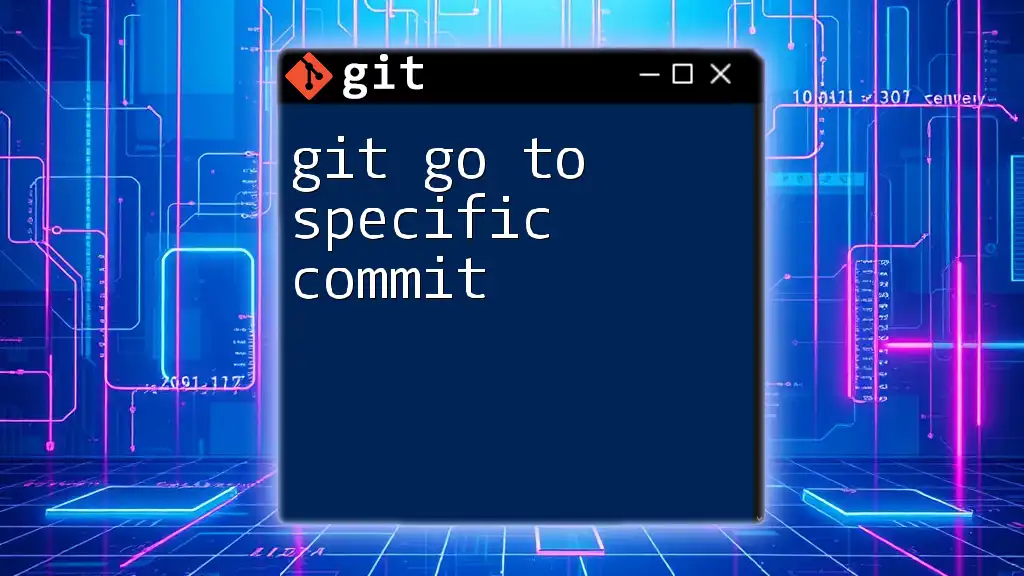
Best Practices for Using Git Revert
Commit Tactfully
Always remember to make meaningful and well-defined commits. Avoid cluttering your commit history with minor changes or experiments. This practice will make it easier for you and others to navigate your project’s history.
Understanding Your Repository
Before performing any operations like reverting commits, ensure you have a solid understanding of your repository's overall structure and history. Regularly auditing your repository helps maintain clarity and reduces the chance of reverting the wrong commits.
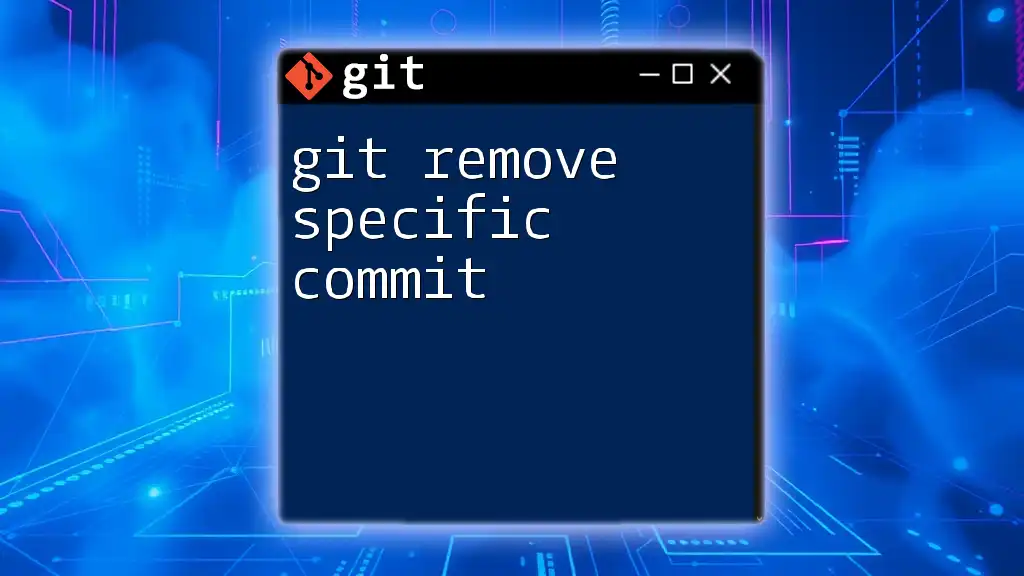
Conclusion
Reverting to a specific commit with Git is a powerful tool for managing your version control history effectively. By following the outlined steps, you can confidently navigate back in time within your project, ensuring that your codebase remains accurate and trustworthy.
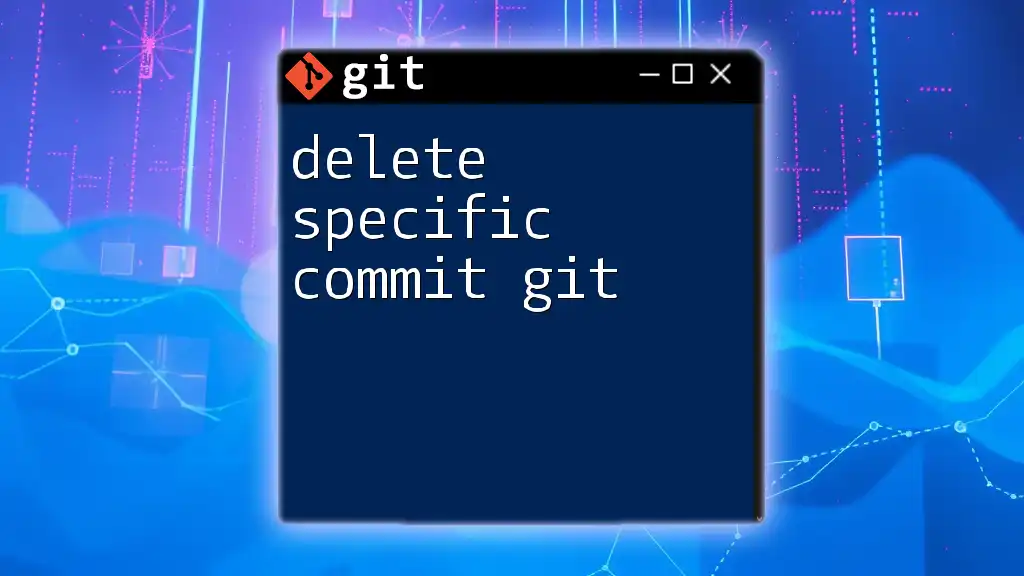
Additional Resources
To dig deeper into Git and learn more advanced techniques, consider referring to the official Git documentation and exploring various tools and software that can enhance your version control experience. Understanding and practicing commands like `git revert` can significantly improve your workflow and teamwork in software development.