The `$ git push` command uploads your local repository changes to a remote repository, allowing others to access your updates.
git push origin main
Understanding Git Push
What is `$ git push`?
`$ git push` is a crucial command in Git, a distributed version control system that allows multiple developers to collaborate efficiently. When you make changes to your local repository, such as adding new files or modifying existing ones, you need to share those changes with other team members. This is where `$ git push` comes into play. It uploads your local commits to the corresponding remote repository, effectively updating it with your contributions.
How `$ git push` Works
When you execute `$ git push`, you are essentially telling Git to take the local changes in a particular branch and send those changes to the remote repository associated with that branch. It ensures that your contributions are reflected in the shared, centralized codebase, which others can access. For example, if you have worked on the `main` branch locally and run:
git push origin main
This command communicates with the remote server (usually a platform like GitHub or GitLab) and pushes your committed changes from your local `main` branch to the `origin` remote's `main` branch.
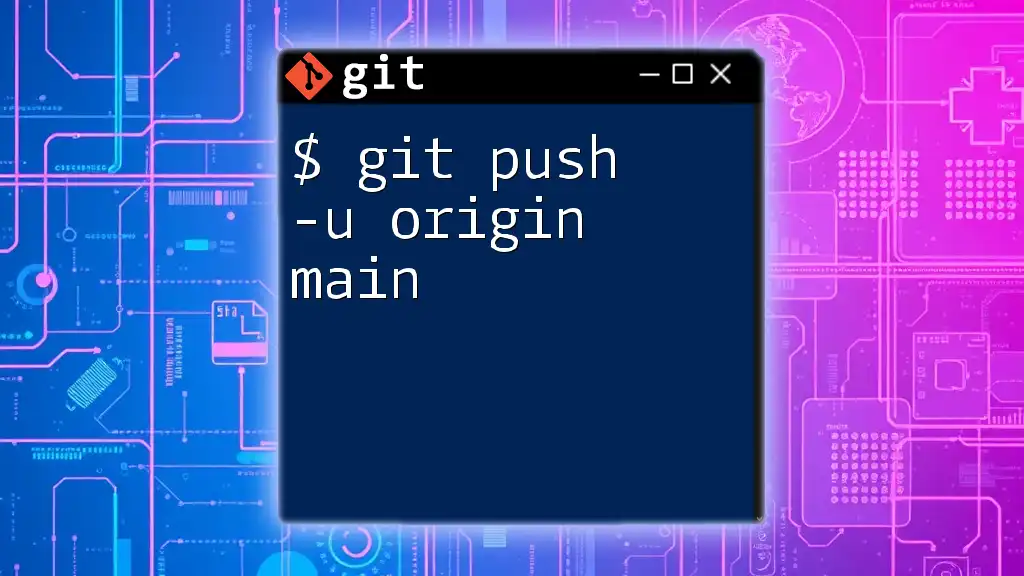
Prerequisites
Setting Up a Local Repository
Before using `$ git push`, you need a local repository. This can be accomplished by running:
git init <repository-name>
This command initializes a new Git repository in the specified directory. You can then start adding files and making changes.
Creating a Remote Repository
The next step is to create a remote repository where you can push your local changes. For instance, if you're using GitHub, sign in to your account and navigate to the "+" icon, then select "New repository." Follow the prompts to set it up. You'll usually need to use either SSH or HTTPS protocols for authentication.
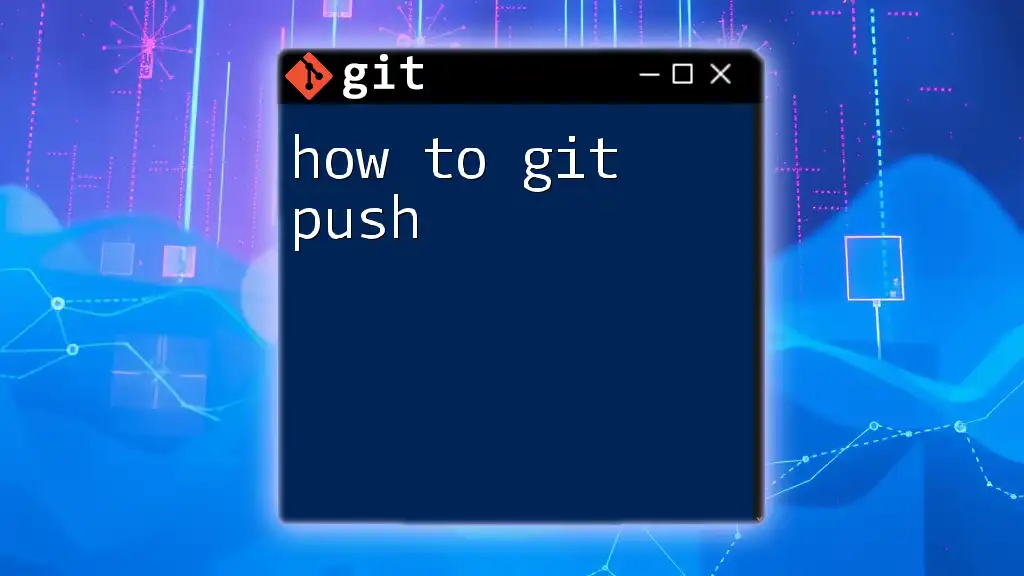
Basic Usage of `$ git push`
Syntax and Options
To utilize `$ git push` effectively, understanding its syntax and available options is essential.
Basic Syntax:
git push <remote> <branch>
- `<remote>`: This is typically `origin`, the default name given to the remote repository.
- `<branch>`: The name of the branch you want to push.
Pushing Changes to a Remote Repository
Once you have made your changes, you first need to stage them:
git add .
Next, you commit the changes:
git commit -m "Your commit message"
Finally, you can push these changes to your remote repository:
git push origin main
In this example, `origin` represents the remote repository, and `main` is the local branch whose changes you want to upload.
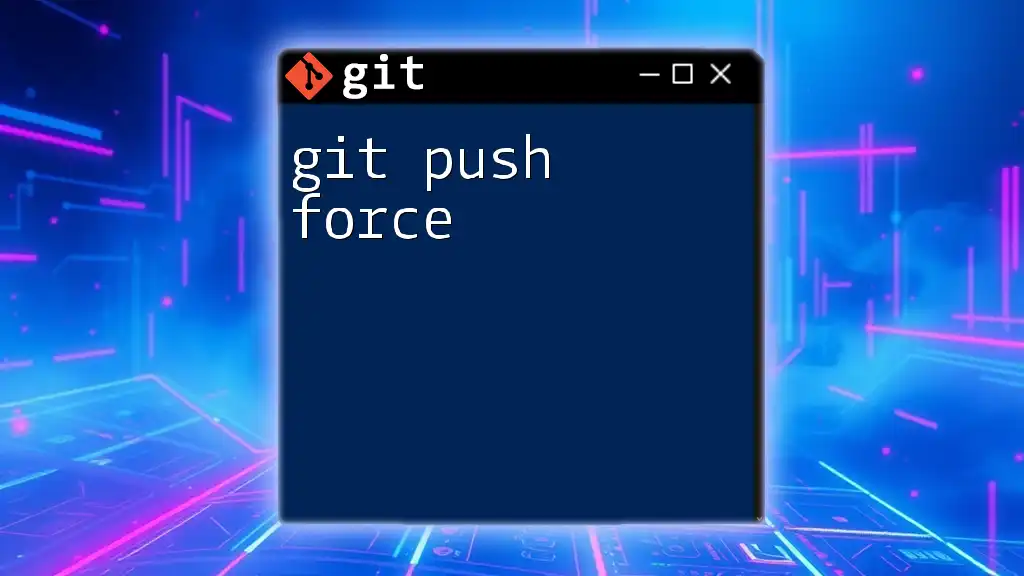
Advanced Pushing Techniques
Pushing to Different Branches
Git allows you to work on multiple branches simultaneously. If you are on a feature branch and you want to push your changes, you can specify the branch:
git push origin feature-branch
This command pushes your local `feature-branch` changes to the remote repository's `feature-branch`.
Force Pushing
Sometimes, you might find that your local branch is out of sync with the remote branch, typically due to rebases or other modifications. In such cases, you may consider using a force push.
However, be cautious: force pushing can overwrite changes in the remote repository, potentially erasing contributions made by others. Use it wisely:
git push --force origin main
Pushing Tags
Tags are vital for marking specific points in your repository, such as release versions. To create and push a tag, follow these steps:
First, create a tag:
git tag v1.0
Then, push the tag to the remote repository:
git push origin v1.0
This process allows your collaborators to easily reference the tagged version.
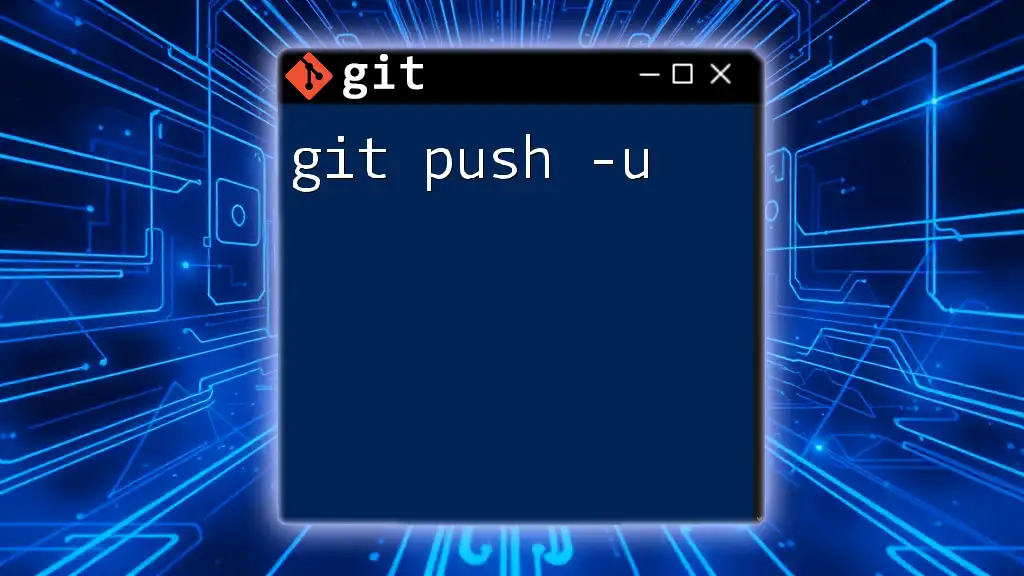
Handling Common Issues
Pushing Changes Yet Getting an Error
Errors can often occur during the push process, particularly if the remote branch has diverged from your local branch. An example of this is the non-fast-forward update failure. To resolve this, you can fetch the latest changes and merge them into your local branch:
git pull origin main
After merging, you can try to push again.
Resolving Merge Conflicts
During a push, if you encounter merge conflicts, it indicates that the changes on your local branch are incompatible with the remote branch. To resolve these, follow these steps:
-
Check which files are in conflict:
git status
-
Resolve the conflicts in your code editor, then stage the files:
git add <filename>
-
Finally, commit the resolved conflicts:
git commit -m "Resolved merge conflict"
Now, you can successfully push your changes.
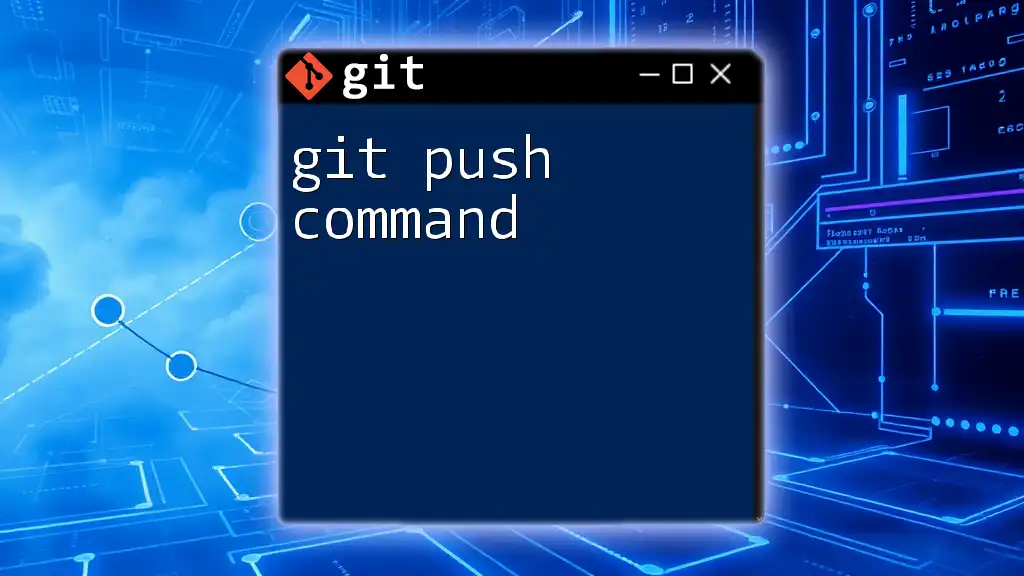
Good Practices
When to Push Changes
Regularly pushing your changes can help ensure that your work is backed up and accessible to your collaborators. It's a good practice to push after completing significant features or once your changes are tested and ready for review.
Using Branching Strategies
Adopting a structured branching strategy, such as Git Flow or feature branching, can streamline collaboration. Different strategies often require varied approaches to pushing. Being consistent in your workflow enhances team collaboration and code management.
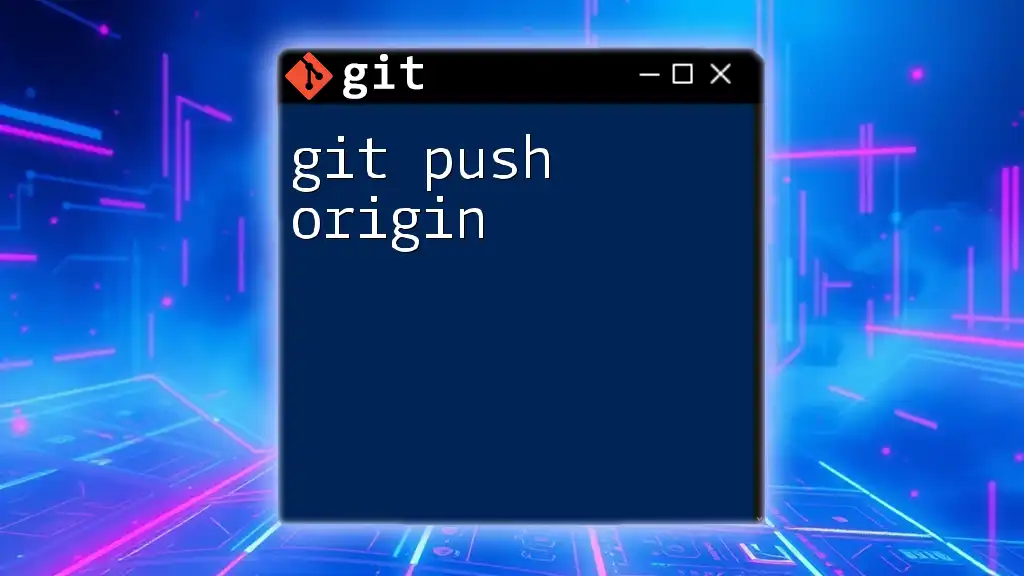
Conclusion
To conclude, `$ git push` is an indispensable command in Git workflows, allowing for seamless collaboration among developers. By understanding its functionality, best practices, and common pitfalls, you can improve your version control skills and contribute effectively to team projects.
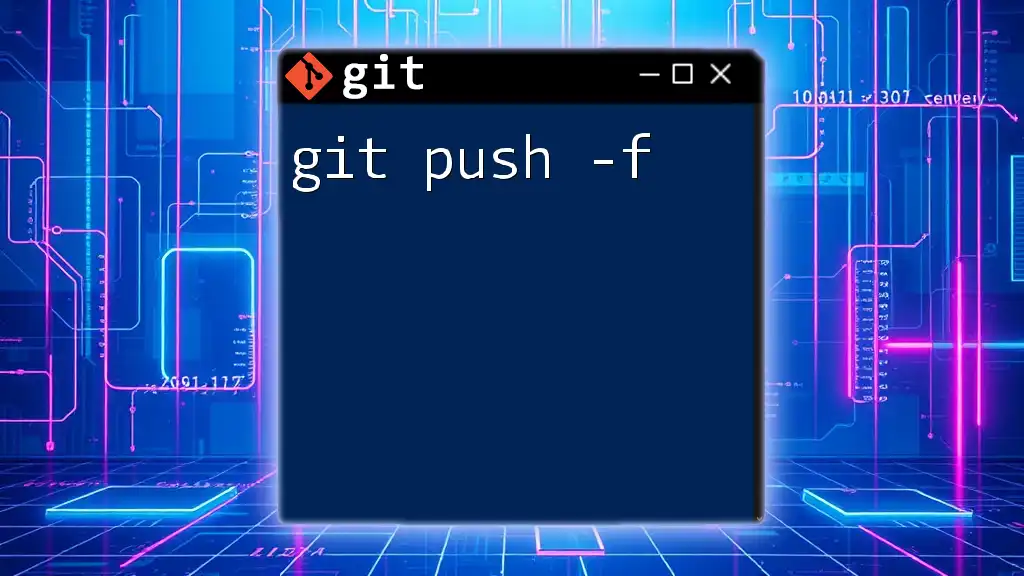
Further Resources
For more information and in-depth tutorials, consider checking out the official Git documentation or enrolling in courses that focus on Git basics and advanced techniques.
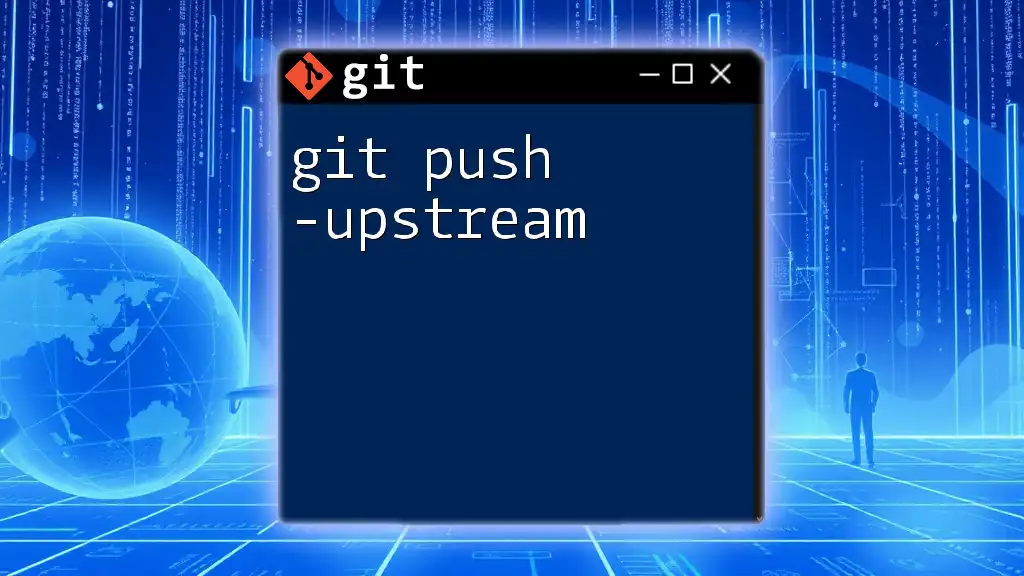
Call to Action
Feel free to share your experiences with `$ git push`, as well as any challenges you've faced while working with it. Your feedback and questions are invaluable for enhancing our learning community!