In this post, we will create a simple "Hello World" program using Git to demonstrate the basic commands required for initializing a repository, adding a file, and committing changes.
Here’s a quick code snippet to get you started:
# Initialize a new Git repository
git init
# Create a new file and add "Hello, World!" to it
echo "Hello, World!" > hello.txt
# Add the file to the staging area
git add hello.txt
# Commit the changes
git commit -m "Add hello world program"
What is Git?
Git is a powerful version control system that allows developers to efficiently track changes, collaborate on projects, and manage code throughout its lifecycle. Created by Linus Torvalds in 2005, it has since become an essential tool in software development. Understanding Git’s functionality is crucial for anyone looking to work in programming or software engineering.
Why Learn Git?
Learning Git brings several benefits to developers. It enhances collaboration by allowing multiple individuals to work on a single project without conflicts, thereby streamlining the development process. Additionally, Git provides flexibility in managing project versions, making rollbacks possible and minimizing the risk of losing work when errors occur. Mastering Git is also an invaluable asset for career growth, as most tech positions require familiarity with version control systems.
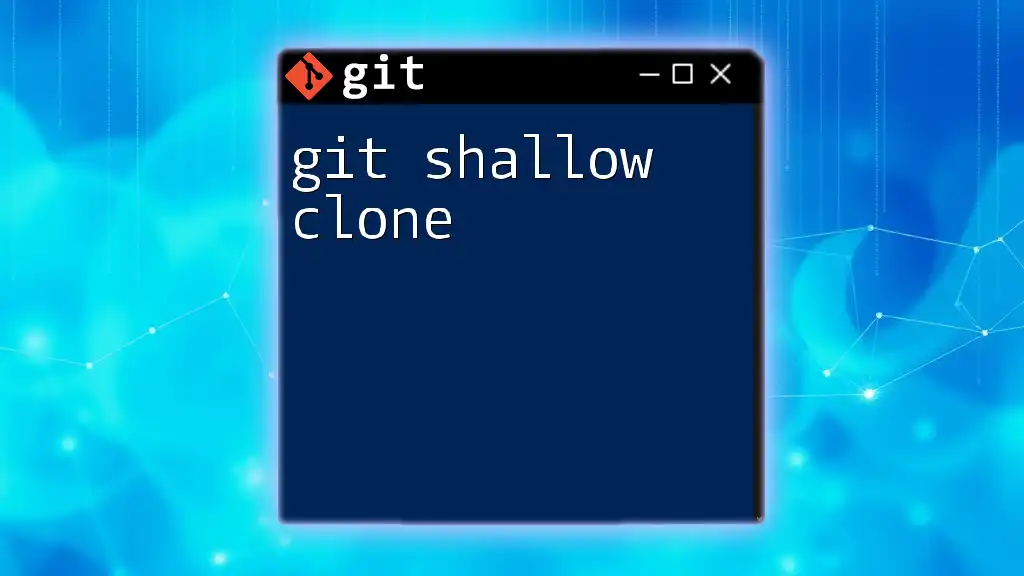
Setting Up Your Git Environment
Installing Git
To get started with using "git hello world," the first step is to have Git installed on your computer. The installation process differs across operating systems:
-
Windows: Download the Git installer from the [Git website](https://git-scm.com/download/win) and run it. Follow the prompts to complete the installation.
-
macOS: You can either download it from the Git website or use Homebrew by running:
brew install git
-
Linux: Use the package manager for your distribution. For Ubuntu, for instance, you can use:
sudo apt-get install git
Configuring Git for the First Time
After installation, configuring Git is the next important step. Setting your identity helps Git to track who makes changes. Use the following commands to set your name and email:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
The `--global` flag means these settings apply to all repositories on your machine. If you ever need specific settings for a single project, you can omit `--global`.
To verify your configuration, check the settings with:
git config --list
These commands ensure that all your commits will be attributed correctly.
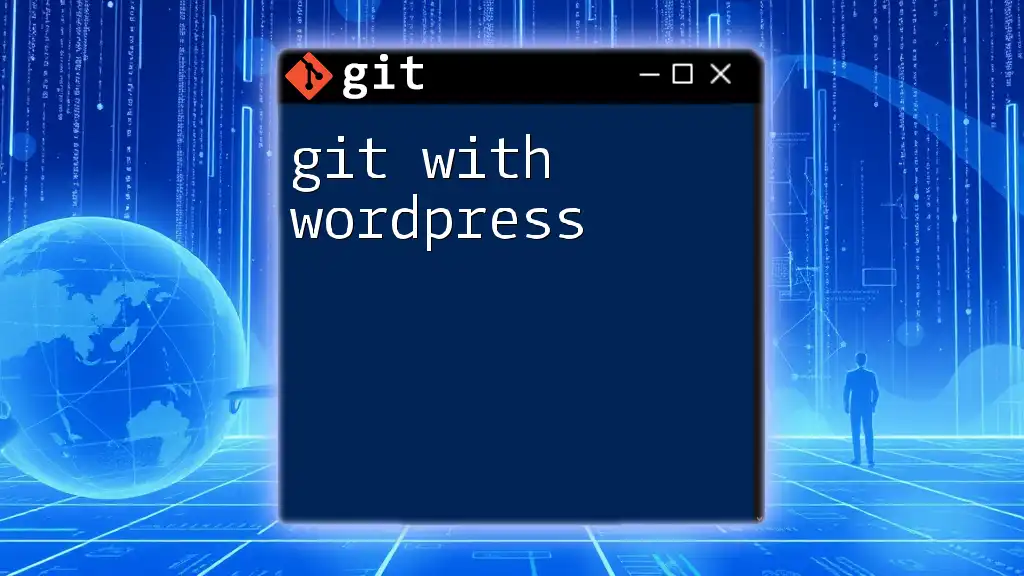
Creating Your First Git Repository
What is a Git Repository?
A Git repository (or "repo") is where your project’s files are stored along with the entire history of changes made to them. There are two types of repositories: local repositories (stored on your machine) and remote repositories (stored on servers).
Initializing a New Repository
To begin with "git hello world," create a new directory and initialize your Git repository. Here’s how you can do that:
git init hello-world
This command creates a new directory called `hello-world` and sets it up as a Git repository. Inside the `hello-world` directory, you'll find a hidden `.git` directory that contains all the version control information.
Adding Files to Your Repository
Next, let’s add our first file. Create a simple text file with the following command:
echo "Hello, World!" > hello.txt
Now, you need to stage the file for committing by using the `git add` command. This command tells Git to start tracking the changes to `hello.txt`:
git add hello.txt
At this point, `hello.txt` is in the staging area, waiting to be committed.
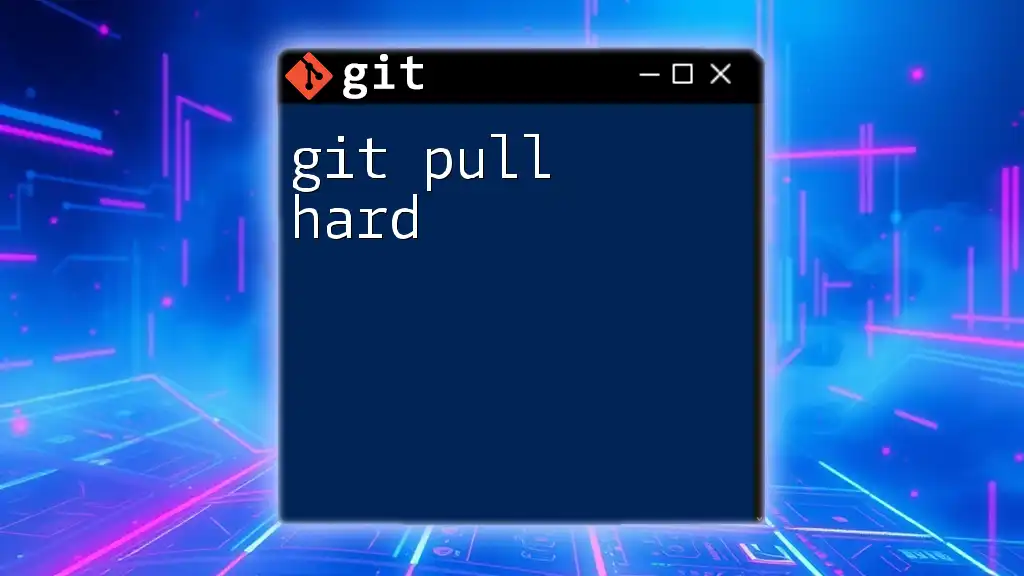
Committing Your Changes
Understanding Commits
Committing is like taking a snapshot of your files at a given moment. Commits in Git create a history of changes, making it easy to backtrack if necessary. Each commit should include a message that succinctly describes the changes made.
Making Your First Commit
Now it’s time to commit your staged changes:
git commit -m "Initial commit: Add hello world text file"
The `-m` flag allows you to write a message directly in the command line instead of launching a text editor. Crafting meaningful commit messages helps anyone—your future self included—understand project changes.
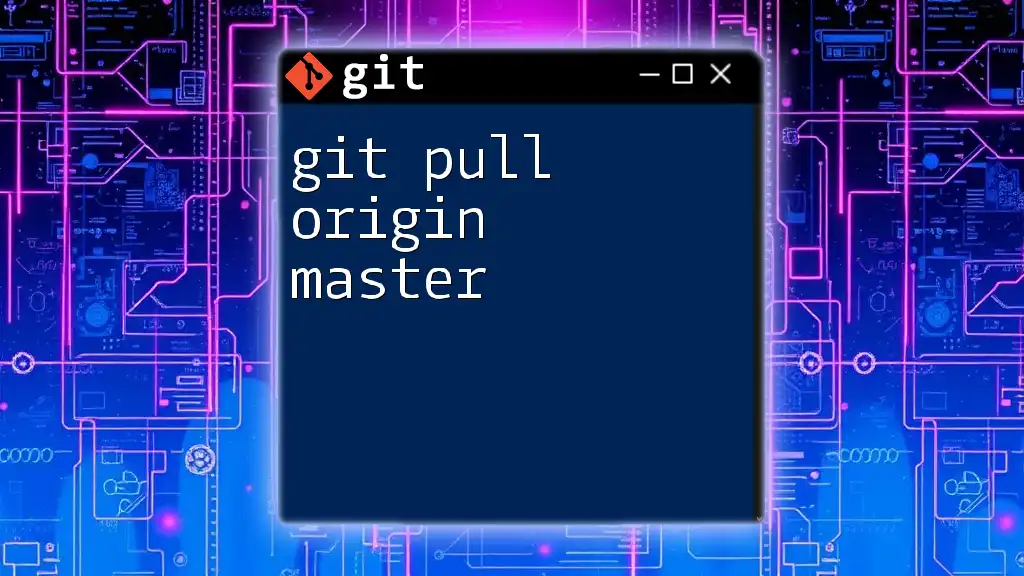
Viewing the Repository History
Checking the Commit Log
You can view the history of your commits using:
git log
This command lists all commits in reverse chronological order, showing the commit hash, author, date, and message.
Understanding the Commit Information
Each commit provides useful information. For instance, the commit hash is a unique identifier, while the author's name and email clarify who made the change. The date identifies when the changes were made, and the message describes the nature of the changes.
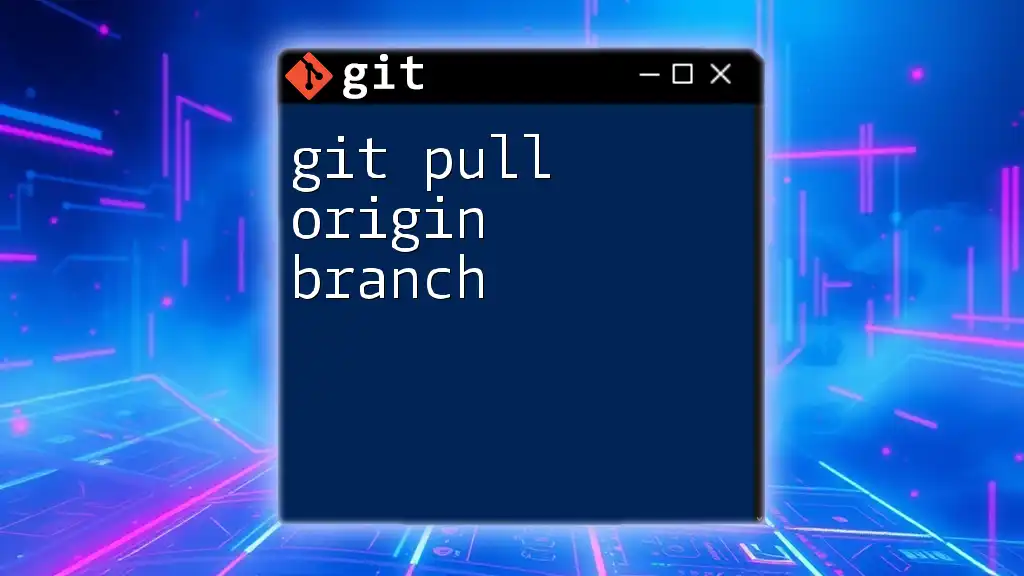
Making Changes and Updating Your Repository
Modifying Files
Let’s modify our `hello.txt` file. Change the contents to reflect a new message:
echo "Hello, Git!" > hello.txt
Once you modify the file, it’s time to stage and commit the changes.
git add hello.txt
git commit -m "Update hello world message"
Viewing Changes Before Committing
Before committing, you might want to see what changes you made. You can use the `git diff` command like this:
git diff hello.txt
This command shows you the differences between the current working version of the file and the last committed version, helping you confirm your changes before you finalize them.
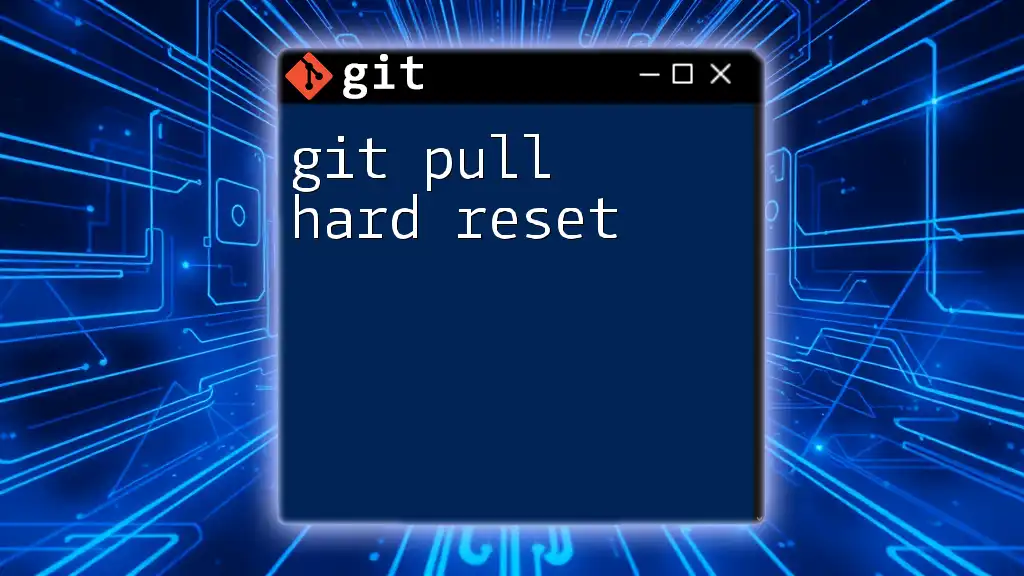
Branching and Merging (Optional for Beginners)
Introduction to Branching
Branches in Git allow you to isolate changes and work on different tasks independently. This is crucial for collaborative environments where you might not want to disrupt the main codebase.
Creating and Switching Branches
To create a new branch and switch to it, you can use:
git branch new-feature
git checkout new-feature
Alternatively, you can create and switch to a new branch in one command:
git checkout -b new-feature
Merging Branches
Once you’re done with your feature in the new branch, you may want to merge it back into the main branch. To do this, first check out the main branch:
git checkout main
Then, merge your branch into main:
git merge new-feature
This merges the changes you made in `new-feature` into your main codebase.
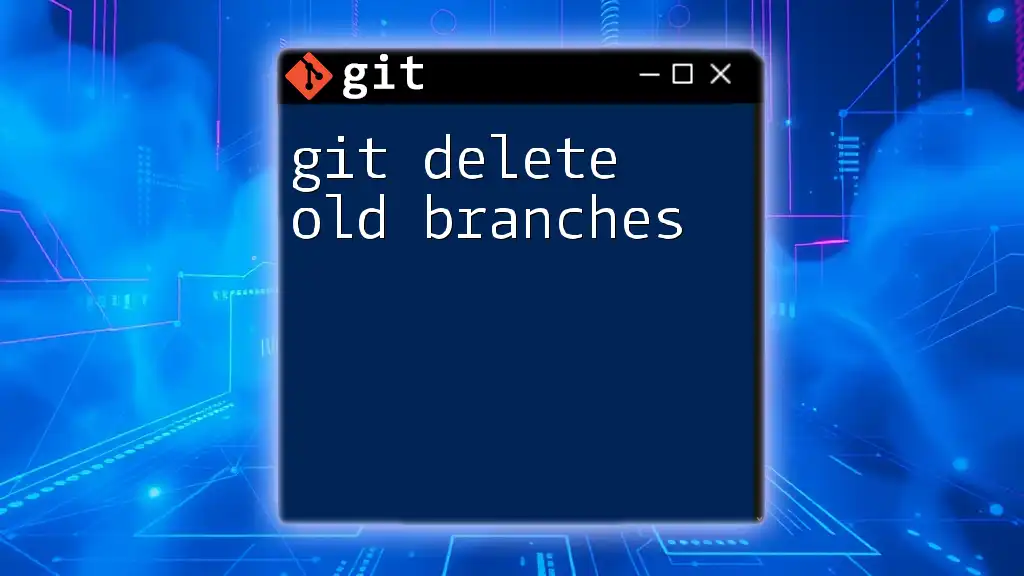
Conclusion
Recap of What You Learned
In this comprehensive guide to "git hello world," you learned about installing and configuring Git, creating your first repository, adding and committing files, viewing project history, and even handling basic branching and merging.
Next Steps
Now that you have a grasp of the basics, consider exploring more advanced Git topics, such as remote repositories on platforms like GitHub, collaborative workflows, and advanced branching strategies.
Final Thoughts
Mastering Git is essential for modern software development. It enhances collaboration, provides tracking tools, and creates a robust environment for managing code. Dive deeper into Git, practice regularly, and watch your skills grow—your future projects will thank you!