Git hooks are scripts that Git executes before or after specific events in the Git lifecycle, allowing you to automate tasks such as enforcing commit messages or running tests.
Here's a basic example of a pre-commit hook script that prevents committing code with TODO comments:
#!/bin/sh
if git grep -q 'TODO'; then
echo "Commit failed: You have TODO comments in your code."
exit 1
fi
What are Git Hooks?
Git hooks are scripts that Git executes before or after events such as commits, merges, and pushes. They serve as powerful tools for automating tasks, enforcing rules, and enhancing workflows within version control systems. By leveraging Git hooks, developers can streamline their processes and maintain code quality.
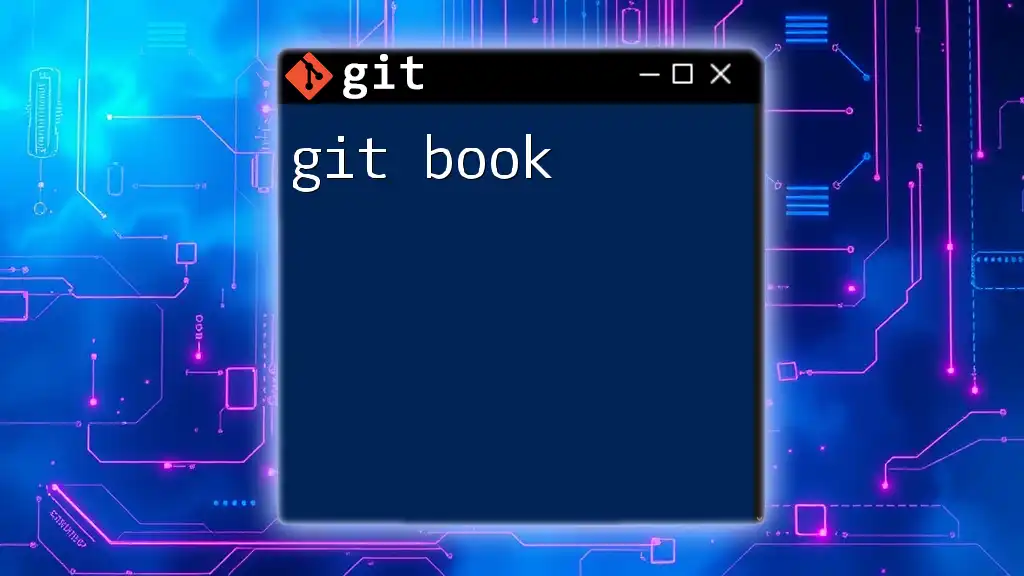
Why Use Git Hooks?
Using Git hooks brings several benefits to your Git workflow. They allow you to automate repetitive tasks, enforce coding standards, and create notifications for team members. This automation reduces human error and frees up time for developers, leading to a more efficient development process.
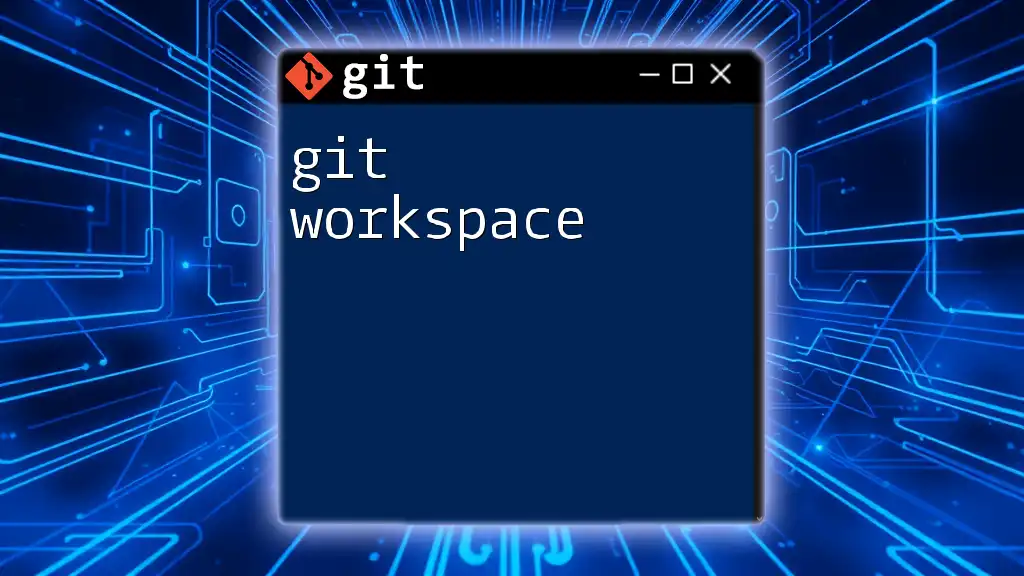
Types of Git Hooks
Git hooks can be classified into two main categories: client-side hooks and server-side hooks. Understanding the difference between these two types is crucial for leveraging their capabilities.
Client-Side Hooks
Client-side hooks are triggered by operations that occur on the local repository. They are primarily used to perform checks or automate actions before or after significant actions like commits.
Example: Pre-Commit Hook
The pre-commit hook executes before a commit is finalized. It is an ideal place to run tests, check code formatting, or prevent the inclusion of unwanted files.
Code Snippet:
#!/bin/sh
npm test
The above code snippet runs the `npm test` command to ensure that all tests pass before allowing a commit. This practice helps prevent broken code from being added to the repository.
Example: Post-Commit Hook
The post-commit hook runs after a commit has been made. It can be used for tasks like sending notifications or performing actions that do not affect the commit itself.
Code Snippet:
#!/bin/sh
echo "Commit successful! A notification has been sent."
In this example, a notification is sent whenever a commit is successfully made. Utilizing post-commit hooks is essential for staying informed during collaborative development.
Server-Side Hooks
Server-side hooks are executed on the repository server and are responsible for managing actions that occur in the remote repository. They provide greater control over incoming changes and collaboration among team members.
Example: Pre-Receive Hook
The pre-receive hook runs on the server before any changes are accepted. It can enforce rules that incoming changes must follow. This is particularly useful for maintaining coding standards and ensuring that commit messages follow a specific format.
Code Snippet:
#!/bin/sh
while read oldrev newrev refname; do
if ! git rev-list --grep='JIRA-[0-9]*' $newrev; then
echo "Aborting push: Commit message must reference a JIRA ticket."
exit 1
fi
done
This pre-receive hook checks if each commit message contains a reference to a JIRA ticket. If not, it aborts the push, thus helping maintain traceability in project management.
Example: Update Hook
The update hook allows the server to respond to references being updated. It provides immediate feedback to developers about operations that affect branches.
Code Snippet:
#!/bin/sh
echo "Updating references!"
Although the update hook in this example is simple, its implementation can lead to valuable insights into branch management.
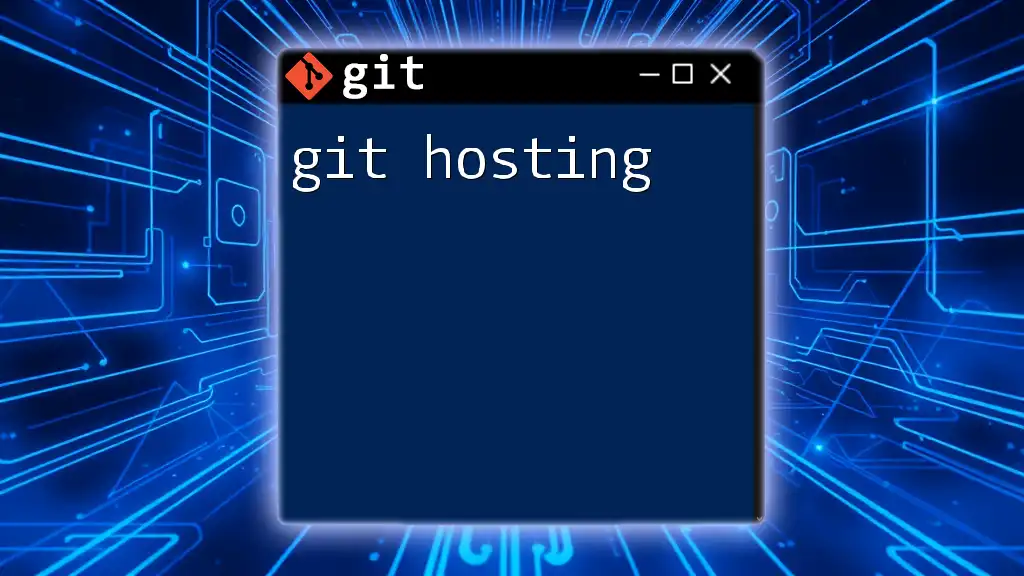
Setting Up Git Hooks
Where to Find and How to Create Hooks
Hooks are located in the `.git/hooks` directory of your repository. Each hook is a script that can be tailored to your needs. By default, Git creates sample scripts for various hooks in this directory. You can edit these or create your own.
Creating a Custom Hook
Creating a custom hook is straightforward. Simply create a new file in the `.git/hooks` directory and make it executable. Here’s a simple example.
Code Snippet:
#!/bin/sh
echo "This is my custom git hook!"
To make this script executable, run the following command in your terminal:
chmod +x .git/hooks/my-custom-hook
Now, your custom hook will run whenever the corresponding Git action is triggered.
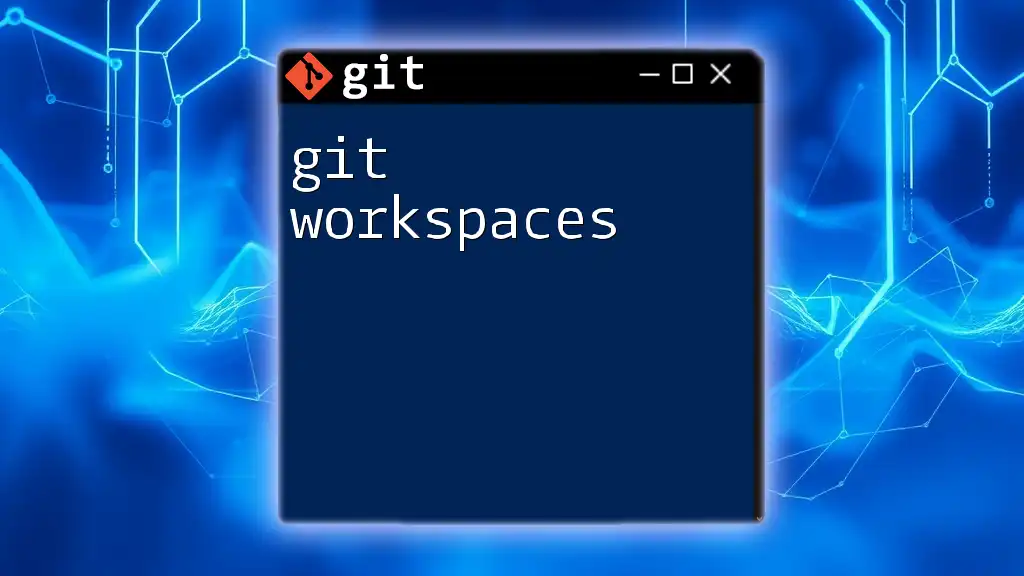
Managing Git Hooks
Distributing Git Hooks
Sharing hooks across your team can be vital to maintaining consistency. There are several ways to distribute Git hooks:
- Git Templates: Use a template repository that includes all hooks, allowing team members to clone it easily.
- Scripts: Write scripts to set up the hooks automatically for new team members.
- Manual Copying: Share the hook files directly via a shared drive or repository.
Testing Your Hooks
Testing your hooks before utilizing them in a production environment is essential. Test hooks in a separate branch or repository to ensure they function correctly without affecting others.
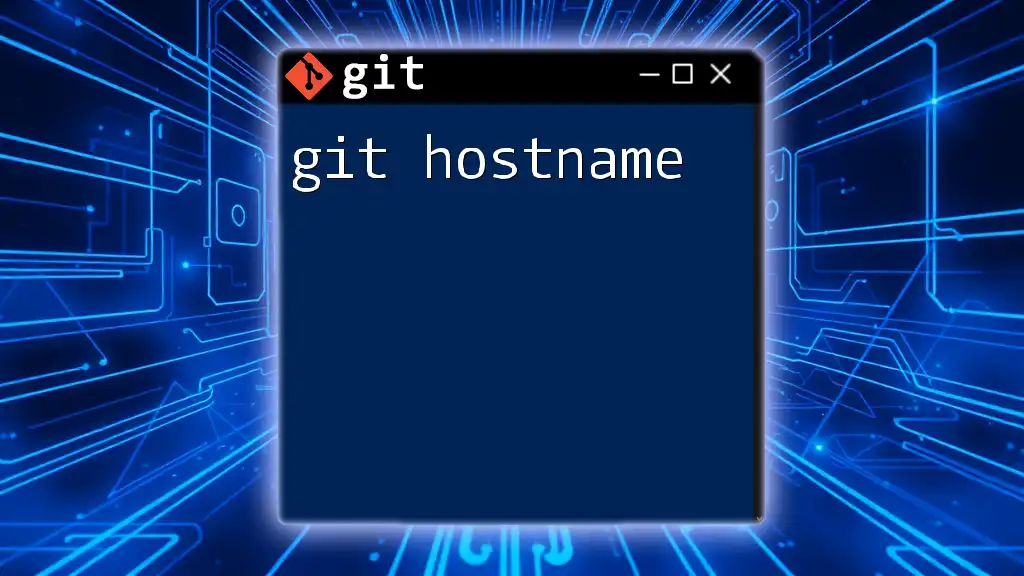
Common Use Cases for Git Hooks
Automating Tasks
Git hooks are perfect for automating tasks that occur frequently throughout the development process. Examples include:
- Code formatting: Using a pre-commit hook to run code beautifiers before a commit is finalized.
- Running linters: Ensuring code adheres to specific standards by running linters as part of the commit process.
Enforcing Code Quality
By implementing hooks, teams can enforce coding standards more effectively. For instance, using a pre-commit hook to run ESLint can help prevent code style issues before code is committed, ensuring adherence to guidelines throughout the project.
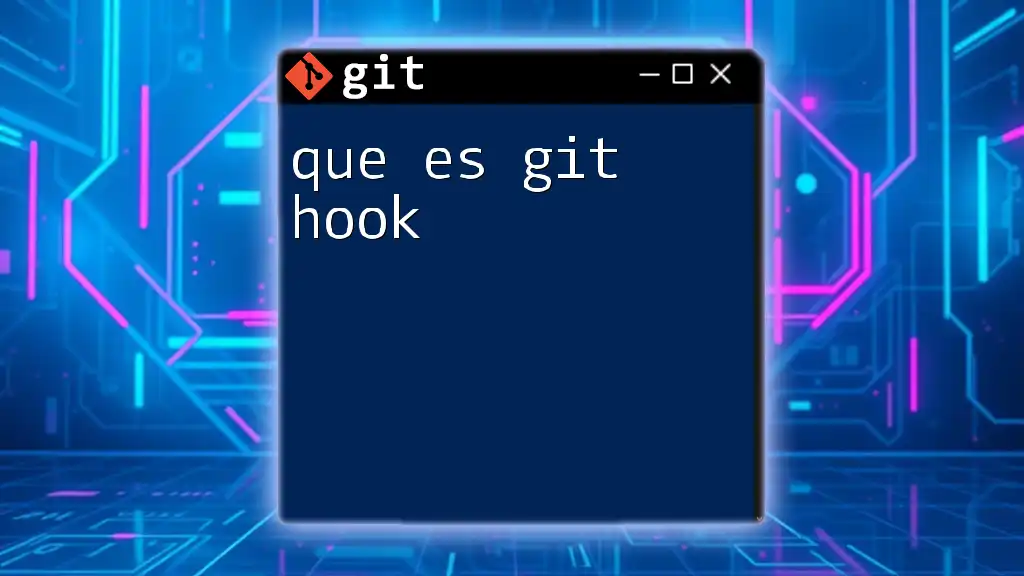
Best Practices for Using Git Hooks
Keep it Simple
Simplicity is fundamental when creating Git hooks. Complicated scripts can lead to confusion and may introduce errors. Aim for clarity and functionality, addressing one task per script.
Avoiding Pitfalls
Common issues with misconfigured hooks can disrupt workflows significantly. It's essential to check permissions and ensure that hooks do not enter infinite loops or execute extensively long processes.
When a hook fails, it typically stops the action it is associated with. Therefore, implementing debugging logs can help identify issues quickly. Try including basic `echo` statements to trace execution flow during hook execution.
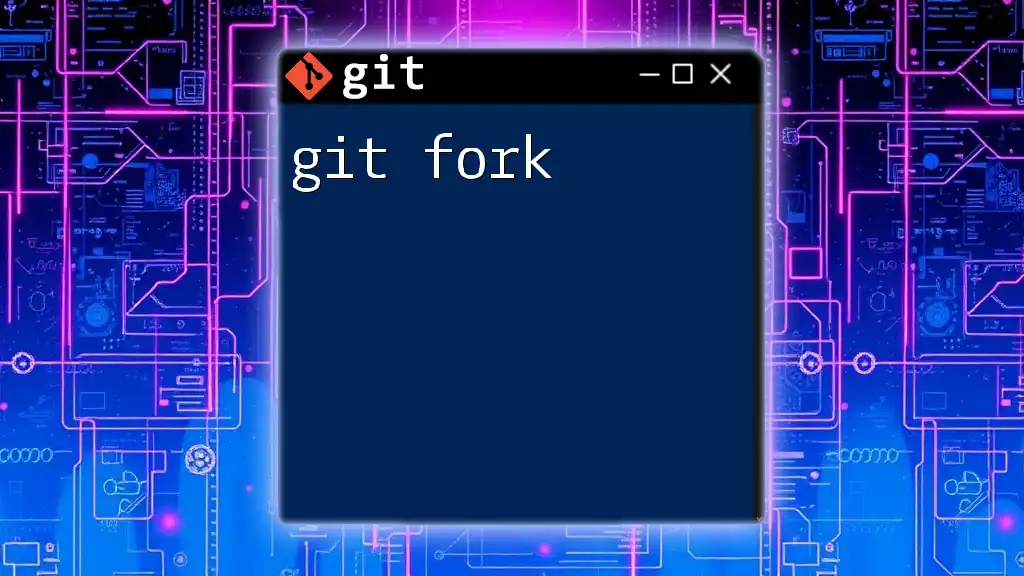
Conclusion
In summary, Git hooks provide a flexible and powerful way to automate tasks, enforce coding standards, and enhance the overall development workflow. By understanding the types of hooks available and how to implement them effectively, you can improve your team's productivity and code quality.
Join Our Community
If you're eager to dive deeper into Git commands and practices, consider joining our teaching platform. We offer comprehensive resources and an engaging community to help you master Git and stay updated with best practices!