A "git book" refers to a comprehensive guide or resource that explains Git commands and workflows, helping users quickly master version control.
Here's a simple code snippet demonstrating how to clone a repository:
git clone https://github.com/username/repository.git
Understanding Git Basics
What is a Git Repository?
A Git repository is essentially a storage space where your project files, along with their entire version history, reside. Understanding the difference between local and remote repositories is crucial for effective version control.
- Local repositories exist on your local machine. This is where you will be developing and making changes to your project.
- Remote repositories, on the other hand, are hosted on a server, which can be accessed by multiple collaborators. Platforms like GitHub, GitLab, and Bitbucket provide these remote repositories.
Git Installation
Before you can reap the benefits of a git book or any Git commands, you'll need to install Git on your machine. Here’s how to do it on various operating systems:
Prerequisites: Ensure your system meets the following requirements—an up-to-date operating system, permissions to install software, and a command line interface available.
Step-by-Step Installation Guide
- Installing on Windows:
choco install git
- Installing on macOS:
brew install git
- Installing on Linux:
sudo apt-get install git
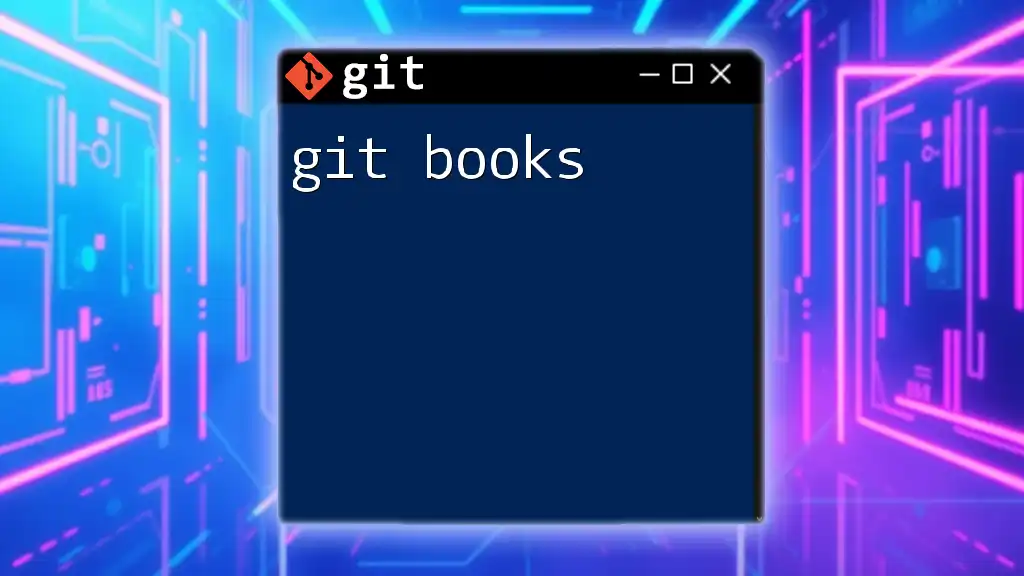
Fundamental Git Commands
Getting Started with Your First Repository
Creating your first Git repository marks the beginning of your journey. To initiate a new repository, you can use the following command:
git init my-repo
This command creates a new directory called my-repo where a hidden folder named `.git` will contain all the tracking information. This is the foundation for your project.
Tracking Changes
To leverage the power of Git effectively, you need to understand how to track changes.
Staging Changes
Before you can commit your changes, you'll need to stage them. The staging area allows you to add changes you want to track. To stage a file, use:
git add filename.txt
This command prepares the specified file for the next commit. Staging gives you full control over what gets recorded in the history.
Committing Changes
After staging your changes, you're ready to commit. A commit in Git acts like a snapshot of your project at that moment. Use the following command to commit your changes:
git commit -m "Initial commit"
Always write informative commit messages. This practice enhances collaboration and helps track changes over time.
Managing Branches
Branches in Git facilitate working on different features or fixes simultaneously without affecting the main codebase.
Understanding Branches
You can create a new branch to isolate changes from the main code. This is how you do it:
git branch new-feature
Creating branches allows you to experiment without the fear of breaking the main project.
Switching Branches
To switch between branches, use the following command:
git checkout new-feature
This command moves you to the `new-feature` branch, allowing you to work on it.
Merging Changes
Once you're satisfied with your feature branch, it’s time to merge these changes back into the main branch.
Merging Branches
To integrate changes from your feature branch into the main branch, use:
git merge new-feature
Merging combines the histories and content of both branches into the current branch you're on.
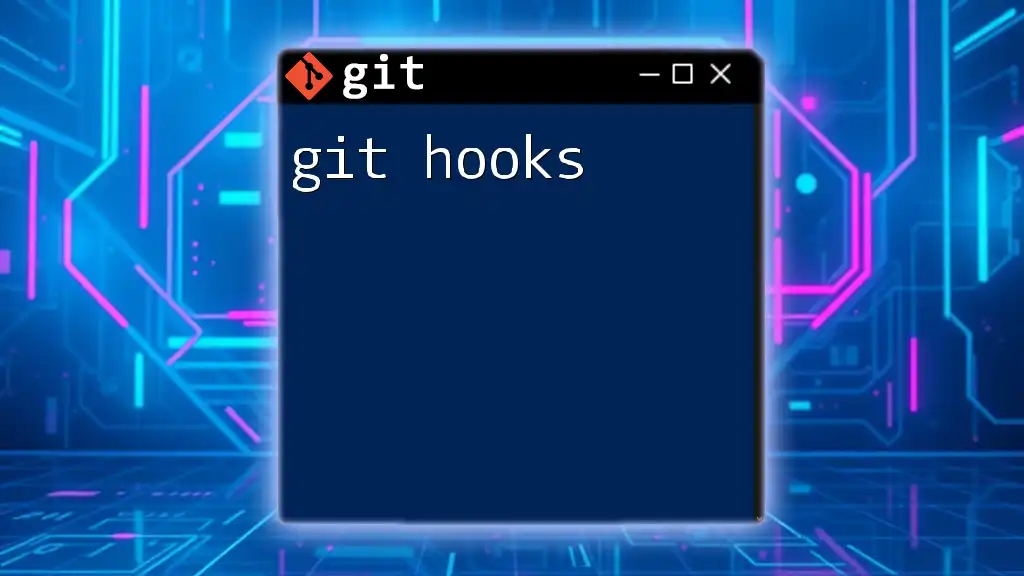
Advanced Git Concepts
Remote Repositories
Working with remote repositories is essential for collaboration and storage.
Configuration of Remote Repositories
To start pushing your local changes to a remote repository, you first need to connect it. Use this command:
git remote add origin https://github.com/user/repo.git
This command creates a shortcut pointing to your remote repository, allowing you to push and pull updates seamlessly.
Pushing Changes to Remote
When you’re ready to share your changes, push to the remote repository using:
git push origin main
This command uploads your changes to the remote `main` branch.
Pulling Updates
To stay synchronized with the remote repository, you will often need to pull updates from it.
Pulling Changes from Remote
You're able to keep your local branch up-to-date with:
git pull origin main
This command retrieves changes from the remote repository and merges them with your local copy.
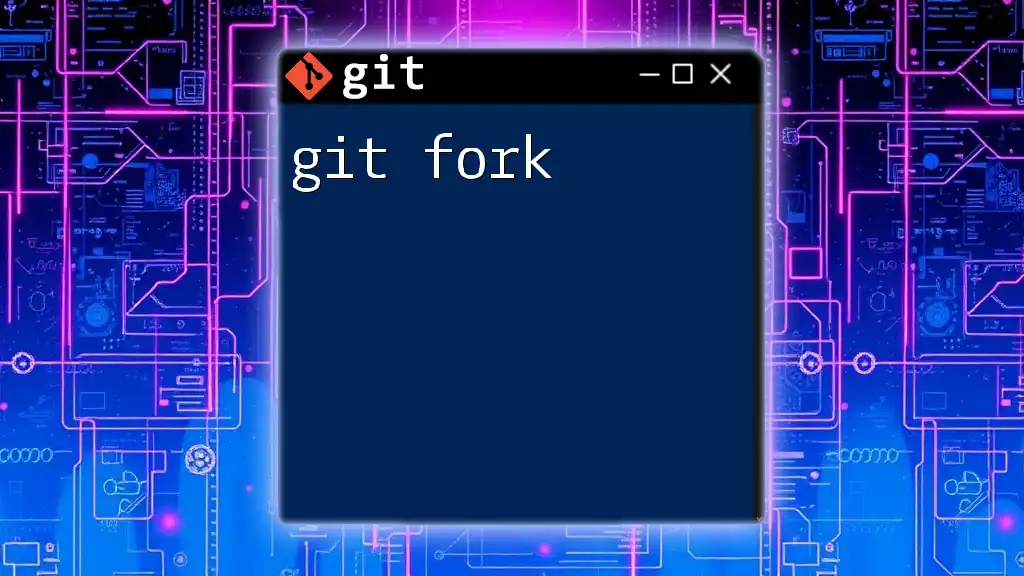
Pro Tips for Git Users
Common Git Workflows
Understanding effective Git workflows is crucial for a smooth development process.
Feature Branch Workflow
This workflow involves creating new branches for each feature or bug fix. This keeps the development clean and organized.
Git Flow
The Git Flow model introduces specific roles for branches, such as `feature`, `develop`, `release`, and `hotfix`, making management more structured.
Git Hooks
Git hooks are scripts that are triggered by specific events during the Git lifecycle.
What are Git Hooks?
Git hooks automate processes and checks, such as running tests before commits.
Examples of Useful Hooks
A practical example is using the pre-commit hook to run linters or tests, ensuring code quality before any changes are committed.
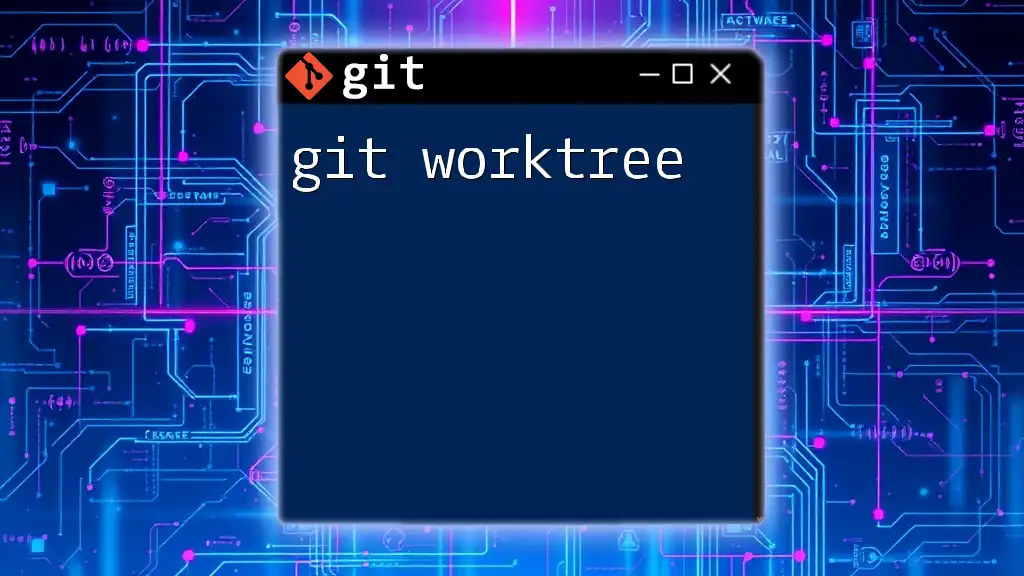
Troubleshooting Common Issues
Resolving Merge Conflicts
Merge conflicts happen when multiple branches make changes to the same line of file.
What is a Merge Conflict?
When you attempt to merge and both branches have conflicting changes, Git will halt and require resolution.
How to Resolve Merge Conflicts
Here's how to handle conflicts:
git status
git merge --abort
Use `git status` to identify which files need attention. After resolving them, continue with your merge.
Undoing Changes
Mistakes can happen! Knowing how to undo changes is vital.
Undoing Mistakes
To revert an accidental change, use:
git checkout -- filename.txt
This command returns the specified file to its last committed state without affecting other files in the repository.
git reset HEAD filename.txt
This command unstages the file but keeps changes in your working directory.
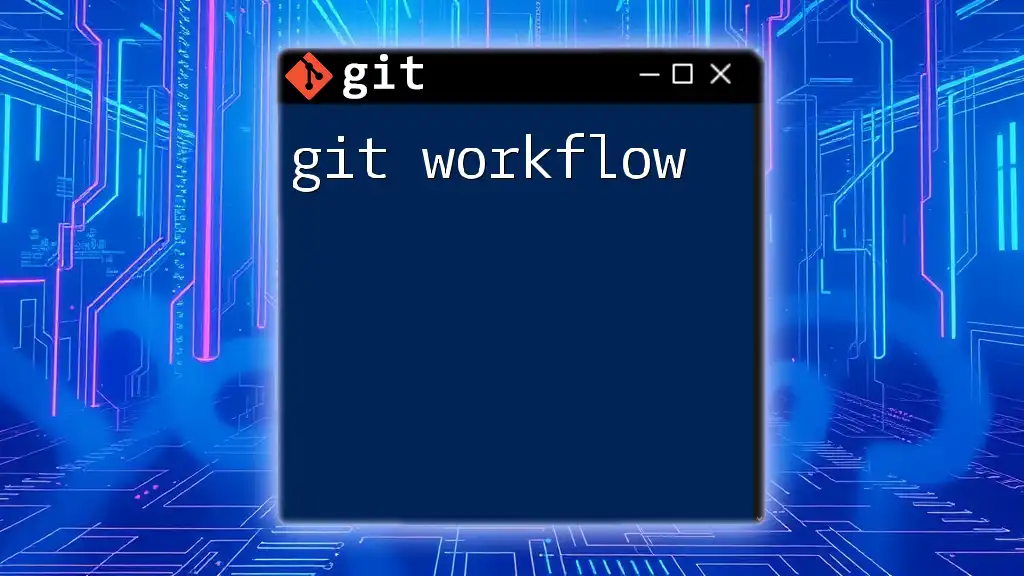
Conclusion
Mastering Git commands is indispensable for modern software development. This git book serves as a comprehensive guide to familiarize yourself with essential and advanced commands alike. By practicing these concepts and commands, you'll become proficient in utilizing Git effectively in your everyday development tasks.
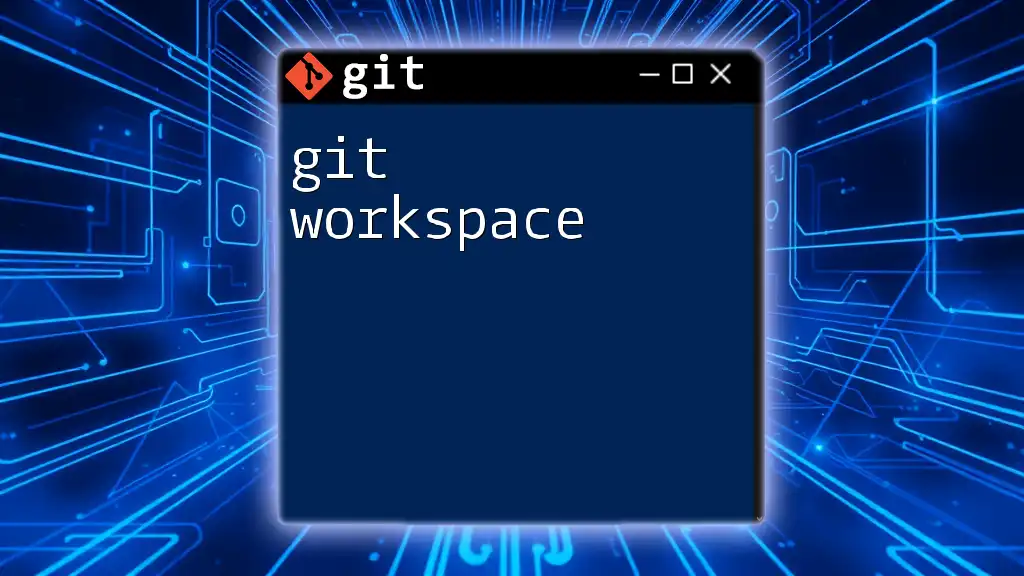
Call to Action
Consider enrolling in our Git course to deepen your understanding and improve your skills in using Git. We welcome your questions and feedback—reach out to us anytime!