To find out which branch a specific commit belongs to, you can use the following command, which shows all branches that contain the commit:
git branch --contains <commit-hash>
Understanding Git Branches
What is a Git Branch?
A Git branch represents an isolated line of development in your project. It enables multiple developers to work on different features or bug fixes simultaneously without interfering with one another. The main branch, often called `main` or `master`, serves as the primary development line, while feature branches could be created for each new task.
The Basic Structure of Commits
In Git, every change you make is stored in a commit, which serves as a snapshot of the project at a specific point in time. Each commit has a unique hash identifier and is connected to its parent commit(s). Understanding the relationship between commits and branches is crucial for correctly navigating your repository’s history.
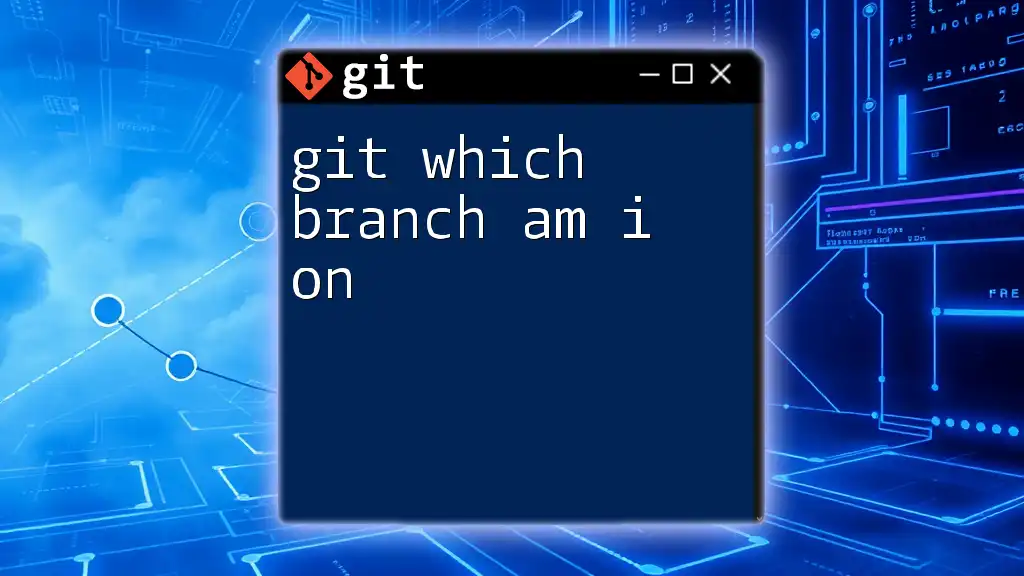
Why You Might Need to Find Out Which Branch a Commit Belongs To
Common Scenarios
There are several scenarios where you might need to know which branch a commit belongs to:
- Merging code: When preparing to merge changes, it's important to ensure that the commit is part of the branch being merged.
- Debugging issues: If a bug arises, identifying the branch related to the problematic commit can facilitate quicker fixes.
- Collaborating with team members: Understanding the origin of a commit can provide context when discussing code with team members, especially in collaborative environments.
Benefits of Knowing the Branch
Knowing which branch a commit belongs to streamlines your workflow and enhances collaboration, allowing developers to:
- Track changes and features more effectively.
- Avoid merge conflicts by recognizing where changes came from.
- Maximize productivity while minimizing confusion in projects with multiple branches.
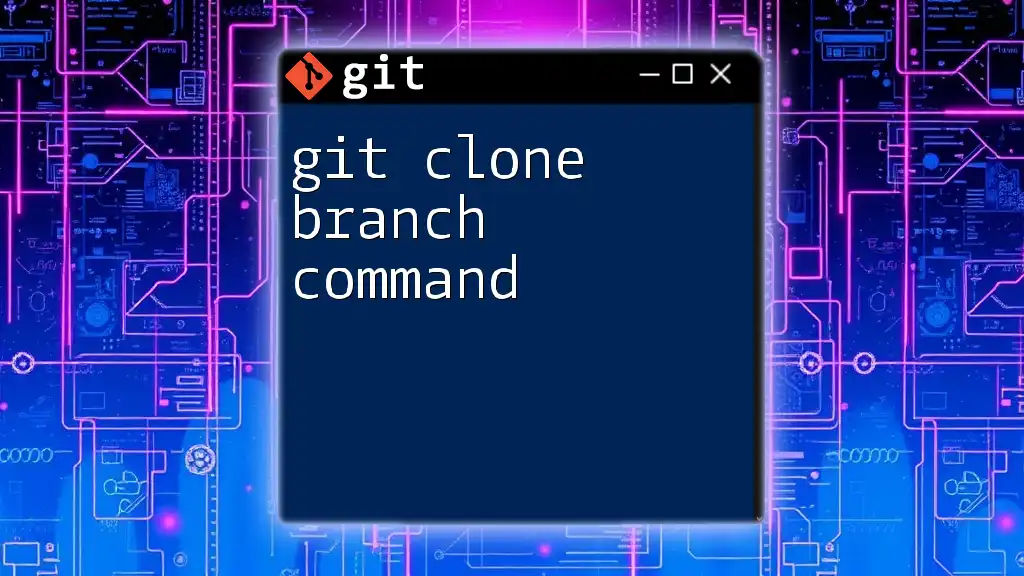
Methods to Find Which Branch a Commit Belongs To
Using Git CLI (Command Line Interface)
Listing All Branches
To get started, you can list all branches in your repository—this gives you an overview of the branches available. Use the following command:
git branch --all
This command displays all local and remote branches, letting you know where to look for your commit.
Finding a Specific Commit
To discover which branches contain a specific commit, leverage the following command:
git branch --contains <commit_hash>
Replace `<commit_hash>` with the actual SHA-1 hash of the commit you're investigating. This command will return all branches that include the specified commit. Understanding what `<commit_hash>` is can be crucial—this hash can be found by using `git log` or in commit messages. The output will show a list of branches, helping you pinpoint where the commit exists.
Using `git show`
Displaying Commit Details
You can also learn about the commit, including its parent and child commits, by using `git show`:
git show <commit_hash>
This command provides detailed information about the commit, including its message, authorship, and which branches might be affected. Analyzing this information can offer insights into other possible related branches.
Utilizing `git reflog`
Accessing Reflog for Commit Lookup
Reflog is an invaluable feature for tracking local repository activity, including lost commits and inactive branches. Access your reflog with:
git reflog
This command lists all actions performed on your Git repository, serving as a valuable tool for checking where your commits originated and identifying which branches they belong to.
Using Visual Git Tools
GUI Options for Easy Visualization
Sometimes, working with GUIs such as GitKraken, SourceTree, or GitHub Desktop can simplify the process of navigating branches and commits. These applications typically provide a visual representation of your repository, making it easier to track down commits and their respective branches.
Specific Features to Look For
In these tools, look for:
- Visual commit graphs that display commit history, often with color coding for different branches.
- Tools for comparing branches and identifying shared commits.
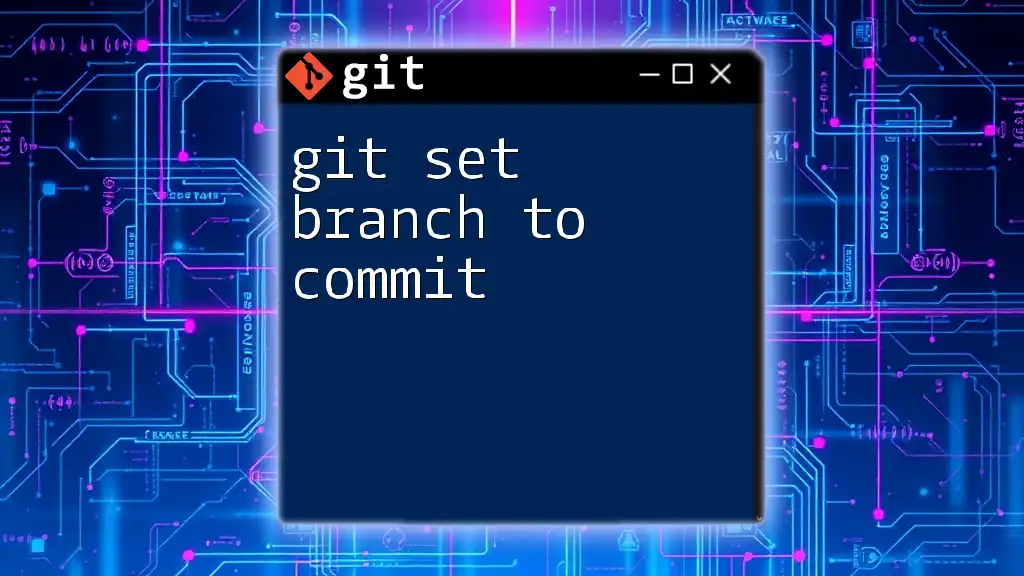
Practical Examples
Example Scenario #1: Finding a Commit in a Feature Branch
Imagine you have a commit with hash `abc1234` and need to find out which feature branch it belongs to. You’d type:
git branch --contains abc1234
The output might show `feature/login` if the commit is located there, confirming where the commit is integrated.
Example Scenario #2: Resolving a Merge Conflict
Suppose you encounter a merge conflict when merging a feature branch into the `main` branch. You can use:
git log --oneline --graph
This will visually depict the commit history and help you backtrack to identify which branch caused the conflict by finding out where the last common commit exists.
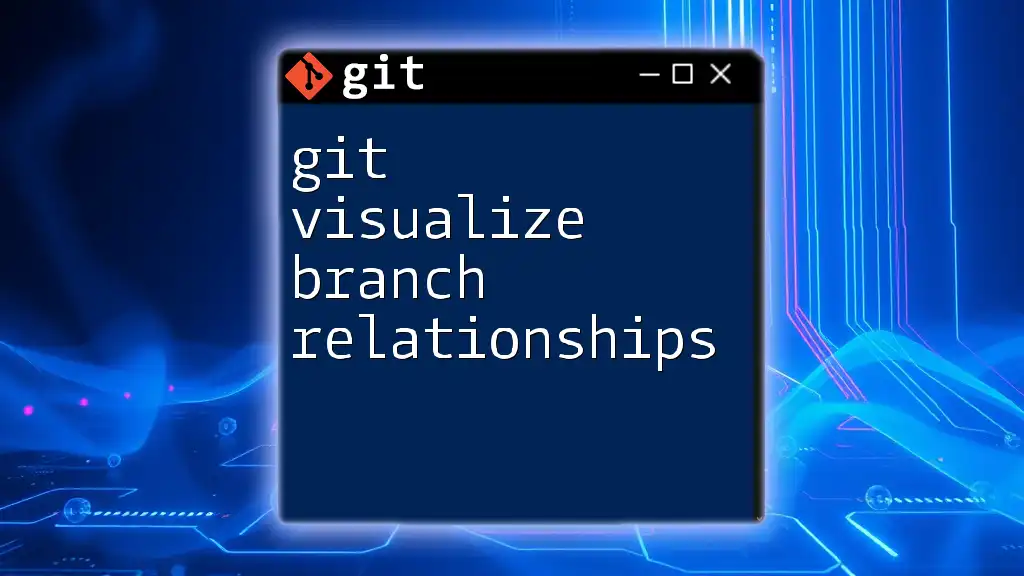
Best Practices for Managing Branches and Commits
Regularly Update Branches
Keeping your branches updated with the latest changes from `main` minimizes the chances of conflicts. Regular merges or rebases help streamline your development process.
Use Clear Commit Messages
Descriptive and informative commit messages can greatly enhance your ability to understand the context of each commit later on. Using standardized formats (like including issue numbers) is a recommended best practice.
Monitor Branch Activity
Consider adopting tools or scripts that help monitor branches for activity, such as automated alerts for when new commits are pushed to specific branches, thereby keeping your collaborative efforts well coordinated.
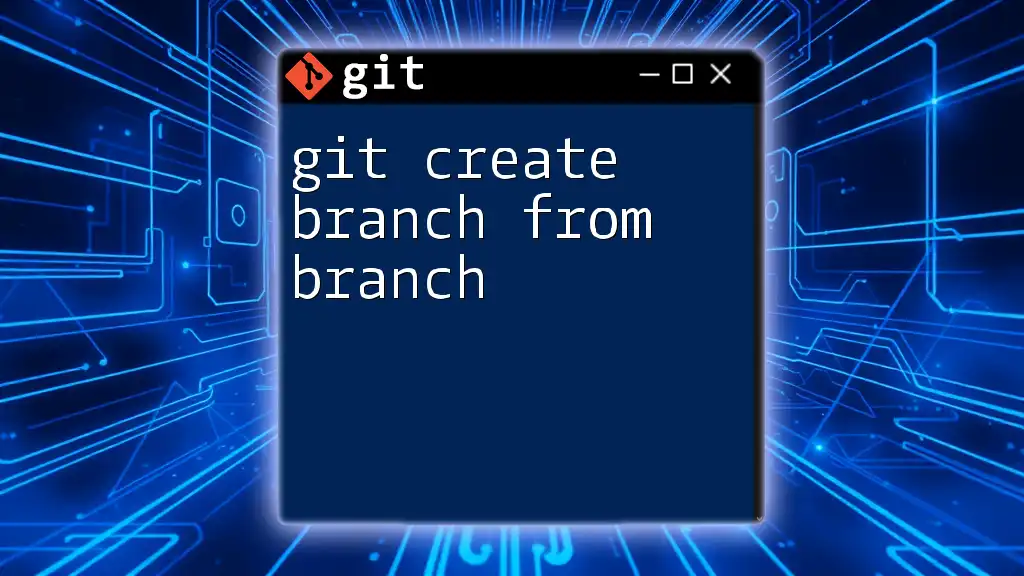
Conclusion
Recognizing which branch a commit belongs to is an essential skill in Git, greatly enhancing your workflow efficiency and collaborative efforts. By utilizing the methods outlined above, such as command-line tools, GUI applications, and effective workflow practices, you can navigate your Git repository with ease and confidence.
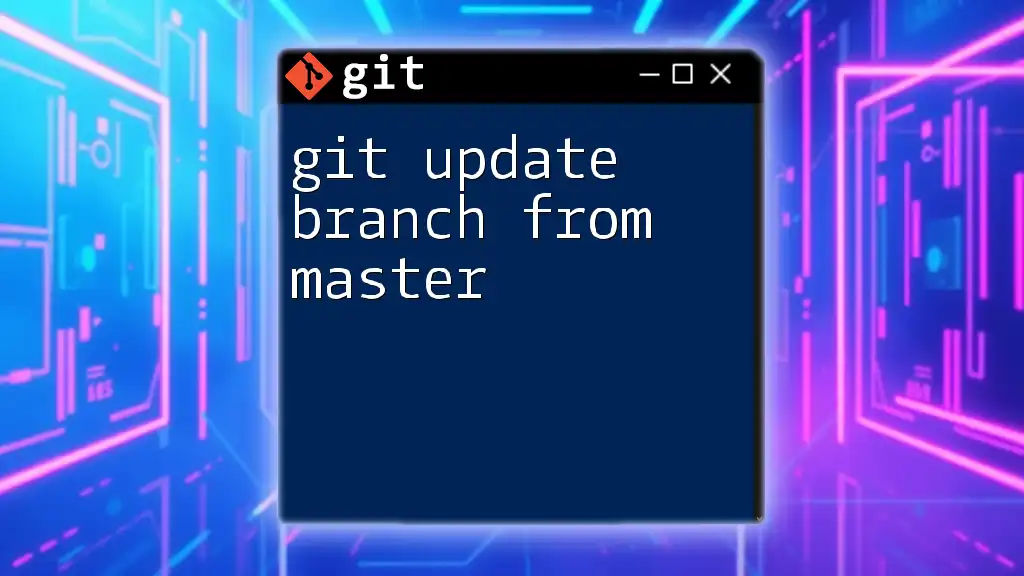
Additional Resources
Links to Official Documentation
For more detailed guidelines and specifications, consider checking the [official Git documentation](https://git-scm.com/doc), which provides extensive information about various Git commands and features.
Recommended Tutorials & Readings
You may also find value in online tutorials or instructional videos focused on Git branching and commit management for deeper insights into these concepts.
Community Forums and Support
Don’t hesitate to reach out to Git communities (like Stack Overflow or GitHub Discussions) where you can ask questions or seek help related to branch and commit issues you might encounter.
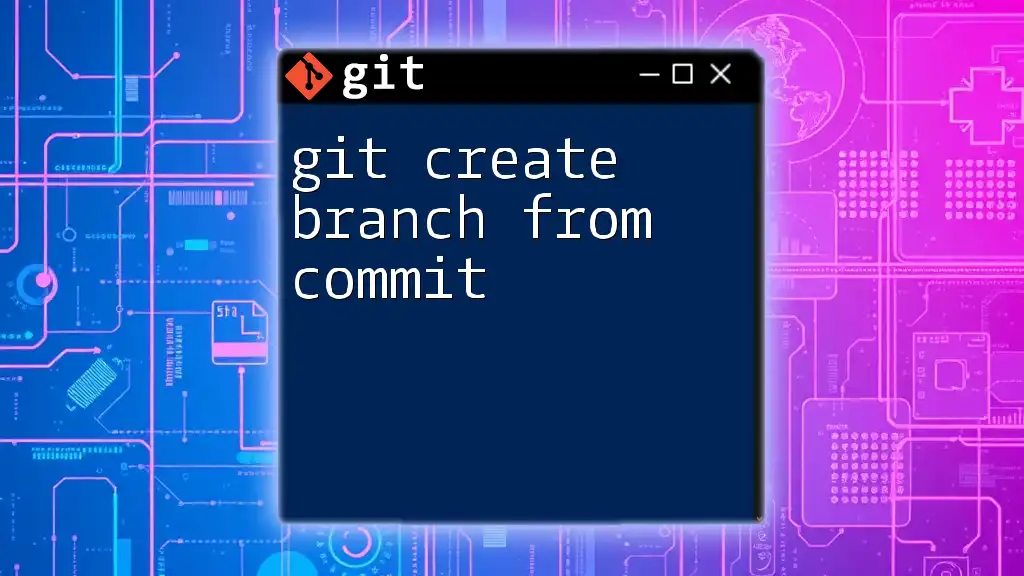
Call to Action
Try out these methods for identifying branches related to specific commits in your own projects. Share your experiences and insights in the comments, and subscribe to our blog for more concise Git tips and tricks.
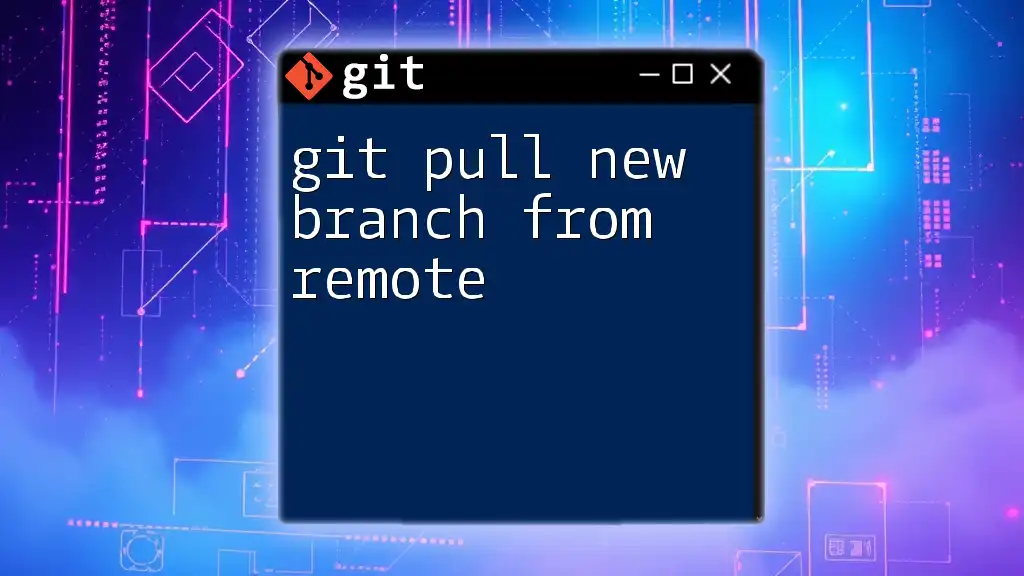
FAQs
Common Questions Related to Git & Branching
-
What should I do if I can't find a commit in any branch? Utilize `git reflog` to check if the commit was lost or detached—this can help you restore its state.
-
Are there limits to how many branches I can create? There is no hard limit in Git, but managing an excessive number of branches may complicate tracking and collaboration. Keeping branches organized is key!