When you encounter the error "git did not commit, you have 3 file conflicts," it indicates that there are three files with merge conflicts that need to be resolved before you can successfully make a commit.
Here's a quick command to see your current conflicts:
git status
Understanding Git Conflicts
What is a Git Conflict?
A Git conflict occurs when Git is unable to reconcile changes made to the same line or content across different branches during operations like merging or rebasing. Conflicts typically arise when multiple developers are simultaneously making changes to shared files, and Git doesn't know which version to preserve.
Common Causes of Conflicts
-
Simultaneous edits: When two or more developers edit the same line of a file in different branches, a conflict will emerge when those branches are merged.
-
File restructuring: If one developer renames or moves a file while another developer is working on that file, it can lead to conflicts during merging.
-
Different branches: When attempting to merge branches that contain divergent changes, there is a higher likelihood of encountering conflicts.

Identifying the Conflicts
How to Recognize Conflict Messages
When a merge attempt encounters a conflict, Git displays specific error messages to indicate the issue. A typical message would look like this:
CONFLICT (content): Merge conflict in file.txt
Automatic merge failed; fix conflicts and then commit the result.
This message signifies that changes in `file.txt` from both branches cannot be automatically reconciled, and manual intervention is required to resolve the conflicts.
Viewing Affected Files
To see which files have conflicts, use the following command:
git status
This command provides a summary of the current repository status, highlighting files that are in a conflicted state. Look for entries marked as "both modified," which indicates the files that need to be addressed.

Resolving Conflict Files
Step 1: Open the Conflicted Files
To resolve the conflicts, start by opening each affected file in your preferred text editor. You'll encounter sections marked with conflict indicators:
<<<<<<< HEAD
// Your changes
=======
// Incoming changes
>>>>>>> feature-branch
These markers delineate the conflicting changes from the two branches. The section between `<<<<<<< HEAD` and `=======` represents your current branch, while the section between `=======` and `>>>>>>> feature-branch` shows changes from the incoming branch.
Step 2: Manual Conflict Resolution
Resolving conflicts requires careful consideration of the changes made. Follow these guidelines for effective resolution:
-
Examine the changes: Understand the nature of the code changes and evaluate how they impact functionality.
-
Decide what to keep: You have three main options:
- Keep changes from your branch.
- Accept the incoming changes.
- Combine both changes judiciously to create a new solution.
-
Edit the code: After deciding which parts to keep or modify, delete the conflict markers, leaving only the final desired code.
Always aim for clean, organized code that preserves the functionality intended. Take the time to ensure that merging different changes doesn’t introduce new errors.
Step 3: Marking Conflicts as Resolved
Once you've addressed conflicts in a file, you need to inform Git that the resolution is complete. This is done using:
git add file.txt
By adding the file, you're indicating to Git that you’ve resolved the conflicts.

Testing After Conflict Resolution
Importance of Testing Changes
After resolving conflicts, it’s crucial to verify that your merged code functions as intended. This can include:
-
Running unit tests: If your project has automated tests, run them to catch any potential issues introduced during conflict resolution.
-
Manual testing: Perform exploratory testing to ensure features are working as expected.
Using Version Control to Maintain Integrity
After marking the conflicts as resolved and running tests, check the state of your project with:
git status
This command will provide confirmation that your branch is ready for committing.

Committing the Resolved Changes
Finalizing the Commit
To finalize the resolution of the conflicts, you need to commit the changes:
git commit -m "Resolved conflicts in file.txt"
Crafting a meaningful commit message is essential. Clearly describe what conflicts were resolved and the rationale behind your choices, as this information can be invaluable for collaborators.
Best Practices for Commit Messages
A well-structured commit message enhances readability and context. Consider including:
- A brief overview of the issue.
- Specific files affected and what was changed.
- The outcome of the resolution process.
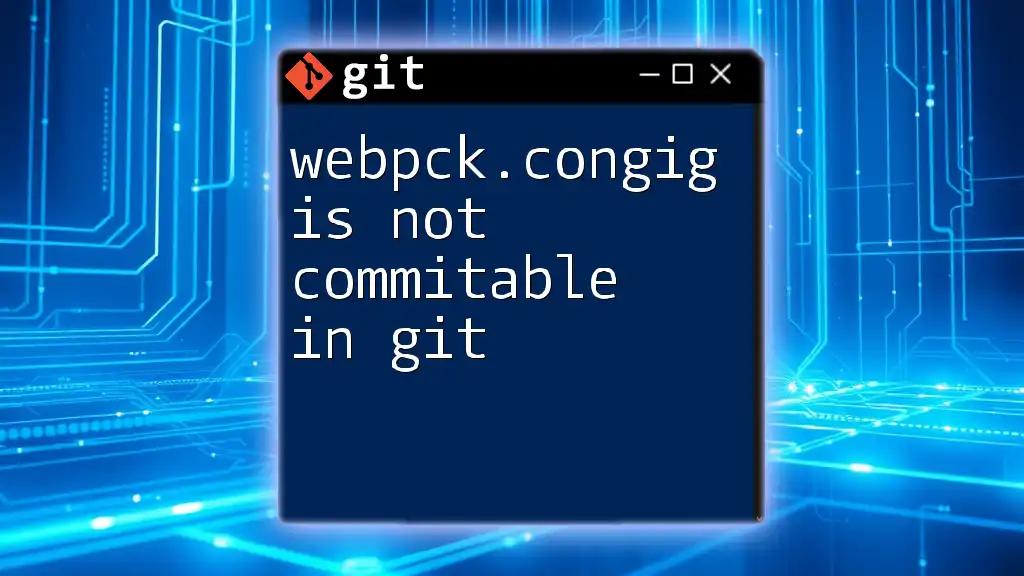
Preventing Future Conflicts
Best Practices for Collaboration
Preventing conflicts begins with effective collaboration strategies:
-
Frequent pulling: Regularly pull changes from the main branch to keep your feature branch up to date. This minimizes the chance of significant divergence.
-
Clear communication: Maintain open lines of communication within your team. Regular meetings and updates about ongoing changes can help, ensuring no two developers are working on the same parts simultaneously.
Using Tools for Conflict Resolution
Several tools and IDEs offer visual conflict resolution features, making the process easier. For instance, tools like Visual Studio Code and GitKraken provide user-friendly interfaces that highlight changes side-by-side. These tools can help streamline the conflict resolution process and reduce errors.

Conclusion
Understanding how to manage and resolve file conflicts in Git is crucial for effective version control, especially in collaborative environments. By following the steps outlined above, you can approach conflicts methodically, ensuring your codebase remains stable and functional. Practice regularly resolving conflicts to enhance your proficiency with Git, making it a seamless part of your development workflow.

Additional Resources
For more in-depth knowledge, refer to the official Git documentation on conflict resolution and explore several tutorials and tools designed to boost your Git skills.