To revert a commit in the master branch in Git, use the following command to create a new commit that undoes the changes from the specified commit ID:
git revert <commit-id>
Understanding Commits in Git
What is a Commit?
A commit in Git is a snapshot of your project at a particular point in time. Each commit represents a set of changes that have been made, including new files added, modifications made, or files deleted. Commits are part of a project's history, allowing you to track changes, revert to previous states, and collaborate with others. A commit not only saves your changes but also includes a message that describes what those changes are, providing context to future developers (or yourself) reviewing the history of the project.
Why You Might Need to Revert a Commit
In software development, mistakes are inevitable. Sometimes, a commit introduces a bug, causes unexpected issues, or adds unwanted features. When such situations arise, knowing how to revert a commit is crucial. This ability allows you to maintain a clean and functional history in your project, ensuring that any problematic changes can be undone effectively, without losing the entire context of your development.
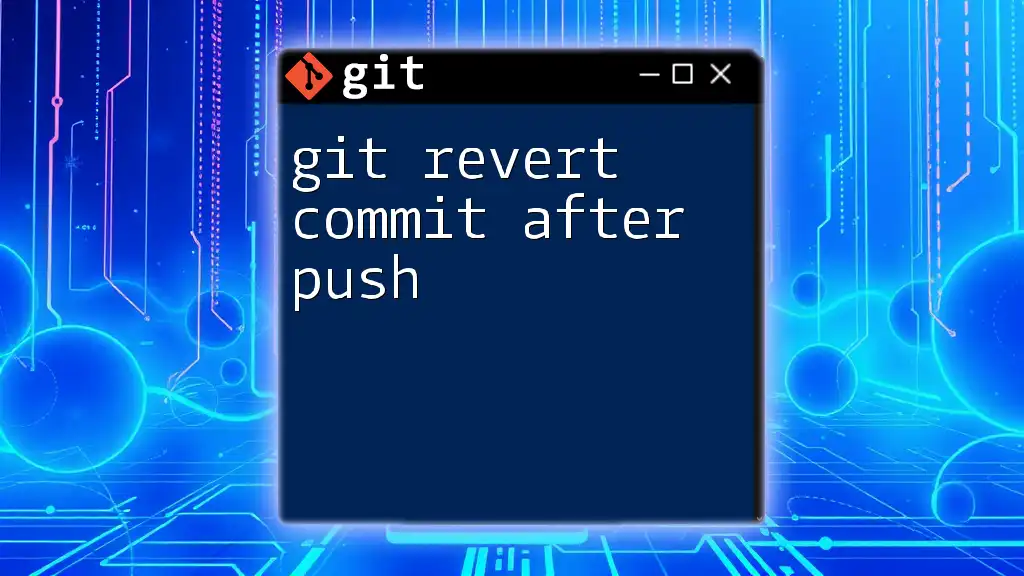
Preparing to Revert a Commit
Verify Your Current Branch
Before reverting a commit, it's essential to ensure that you are on the correct branch. The `master` (or `main`) branch is typically the primary development branch. Use the following command to switch to it:
git checkout master
This command tells Git to change your working directory to the `master` branch, ensuring you are making changes in the right place.
Identify the Commit to Revert
To revert a commit, you first need to identify which commit you want to undo. You can do this using the `git log` command, which displays the commit history in reverse chronological order. Each entry in the log will include a unique commit ID (hash), the author of the commit, date, and commit message.
Run the following command:
git log
You will see an output that looks something like this:
commit abc123def456...
Author: Your Name <you@example.com>
Date: Mon Oct 03 12:30:23 2023 -0700
Your commit message
From this information, you can easily locate the commit you wish to revert by noting its commit ID.
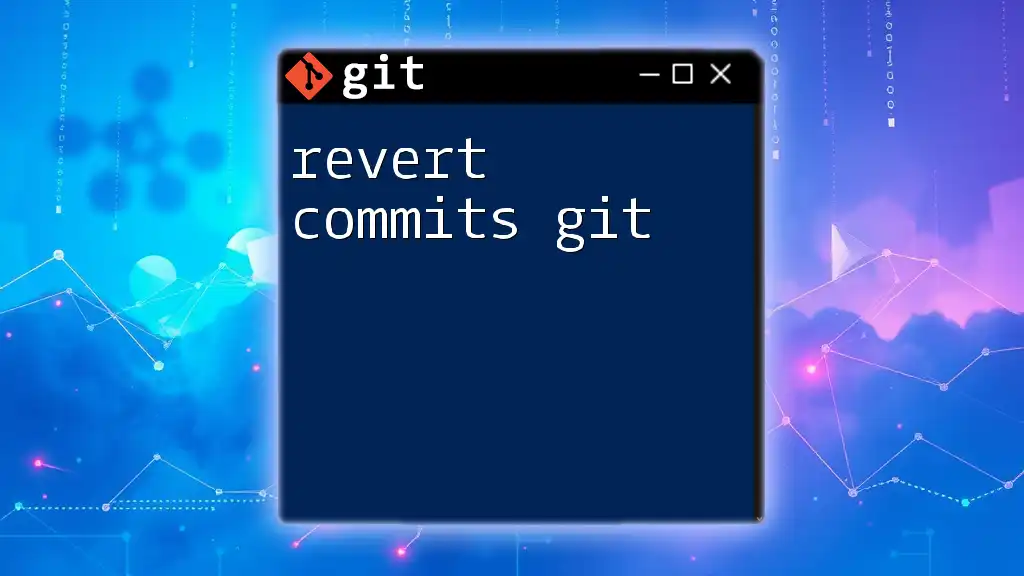
How to Revert a Commit in Git
Revert Command Overview
The `git revert` command creates a new commit that undoes the changes made by a previous commit. Unlike `git reset`, which rewinds the project state to a specific commit, `git revert` keeps the project's history intact while negating the effects of the specified commit. This is crucial in collaborative environments where you do not want to change the shared history.
Reverting a Single Commit
To revert a single commit, use the `git revert` command followed by the commit ID of the commit you want to undo. For example:
git revert abc123def456
After executing this command, Git will create a new commit that reverses the changes made in the specified commit. You might be prompted to enter a commit message for the revert. By default, Git provides a message stating that it is reverting the previous commit, but you can customize this to add more context if desired.
Reverting a Range of Commits
If you need to revert multiple commits in one go, you can adjust the `git revert` command to specify a range of commits. The syntax is as follows:
git revert <commit-id-1>..<commit-id-2>
This command will revert all commits from `<commit-id-1>` to `<commit-id-2>` (exclusive). Remember that the order of commits matters—start from the most recent commit when specifying the range to avoid potential issues.
Conflict Resolution When Reverting
Understanding Merge Conflicts
Sometimes, reverting a commit may lead to conflicts, especially if changes from the reverting commit overlap with subsequent changes. Conflicts occur when Git cannot automatically resolve the differences between the changes made and those you are trying to revert.
Resolving Conflicts
If you encounter a merge conflict during a revert, Git will notify you and pause the revert process. You can resolve conflicts by examining the conflicting files using:
git status
This command will show you which files need attention. Open the files to locate conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`) indicating the changes that need resolution. After resolving the conflicts by editing the file to your satisfaction, stage the changes with:
git add <file-name>
Then, complete the revert by committing the resolved changes:
git commit
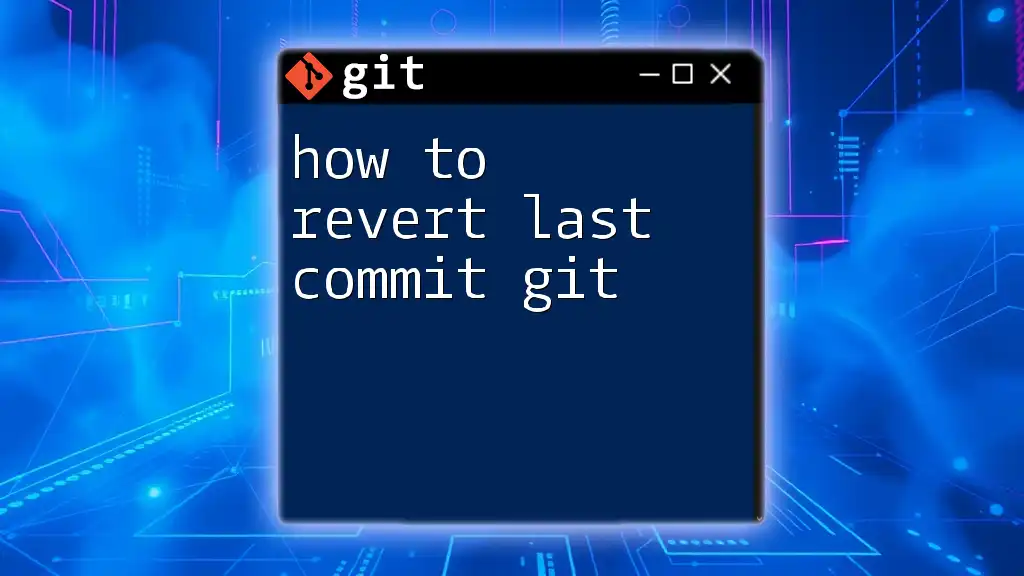
Testing Your Changes
Verifying the Revert
After the revert has been successfully committed, it's important to review the changes to ensure that the code or functionality is as expected. Use the `git log` command again to verify that the new commit has been created:
git log
You should see your revert commit listed, indicating that the previous changes have been successfully undone.
Best Practices Post-Revert
Once you've reverted a commit, ensure to push your changes to the remote repository if you are collaborating with others:
git push origin master
This step is crucial to maintain an up-to-date shared project, allowing all contributors to work on the same base.
It's also a good idea to conduct code reviews, especially in collaborative environments. This practice not only helps catch potential issues but also encourages knowledge sharing among team members, which can lead to a more robust codebase.
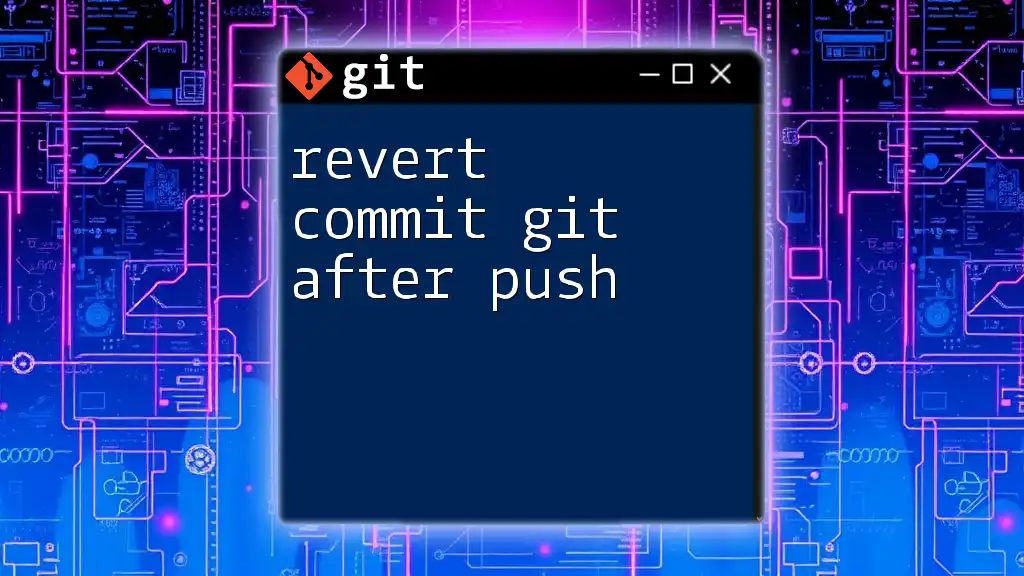
Conclusion
Reverting a commit in Git is a valuable skill for maintaining the integrity of your project. By understanding how to efficiently use the `git revert` command, you can undo problematic changes while preserving the history of your work. Regular practice with these commands will make you more adept at managing your Git repository effectively.
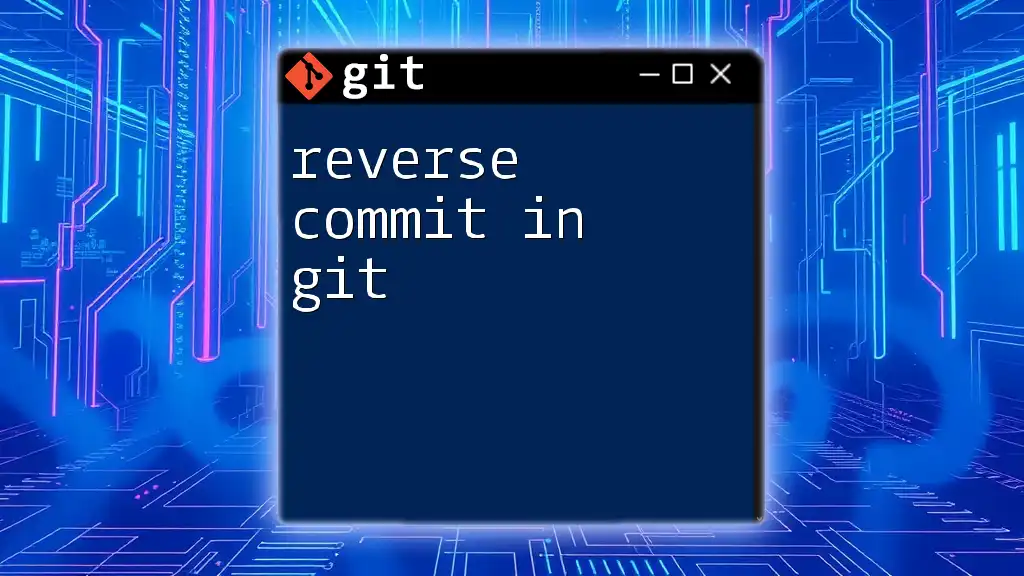
Frequently Asked Questions (FAQs)
Can You Revert a Commit After Pushing?
Yes, you can revert a commit that has already been pushed to a remote repository. The `git revert` command adds a new commit to the history, which negates the changes introduced by the previous commit. This preserves the history of your project, making it the preferred method for making corrections in shared environments.
What Happens to My Branch History After a Revert?
When you revert a commit, Git creates a new commit that undoes the changes made by the specified commit. This process does not erase the original commit but simply creates a new entry in the history that signifies the changes have been reversed.
Is There a Difference Between Reverting and Resetting?
Yes, reverting is a safe way to undo changes by creating a new commit that negates a previous commit, while resetting alters the project history by rewinding to a particular state, which can affect all collaborators working on the same branch. Resetting is more suitable for local changes where others are not involved, whereas reverting should be used in shared and collaborative projects to maintain consistency in version control history.
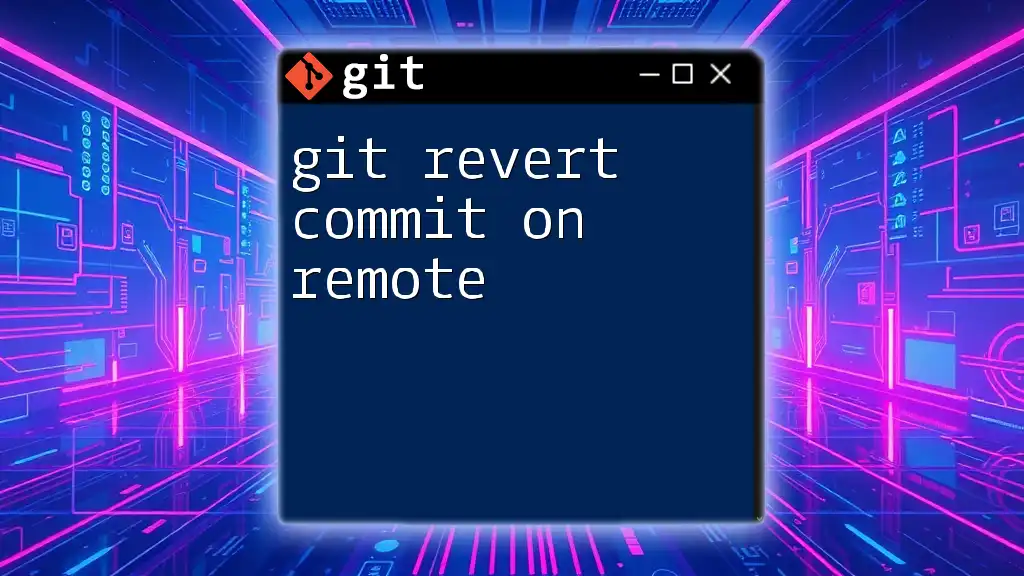
Additional Resources
- [Git Documentation](https://git-scm.com/doc)
- [Git Revert Command](https://git-scm.com/docs/git-revert)
- [Comprehensive Git Tutorial](https://www.atlassian.com/git/tutorials)
This comprehensive guide will empower you to effectively revert commits in Git, ensuring a smooth development process. Happy coding!