To enter a commit message in the Git terminal, use the `git commit -m "Your commit message"` command, which allows you to describe the changes you made in a concise way.
git commit -m "Fixed bug in the user login feature"
Understanding Git Commit Messages
What is a Commit Message?
A commit message is a brief text description that provides context about changes made to a codebase within a specific commit. It serves as a communication tool in version control, allowing developers to understand the nature and purpose of changes over time.
Importance of Good Commit Messages
Effective commit messages play a crucial role in collaboration and maintenance of a project. They enhance clarity and facilitate code reviews, making it easier for team members to grasp changes.
Consider the difference between the following examples:
- Poor Commit Message: "Update files"
- Effective Commit Message: "Fix bug in user login mechanism"
The first message lacks clarity and context, while the second one tells you exactly what was changed and why it matters.
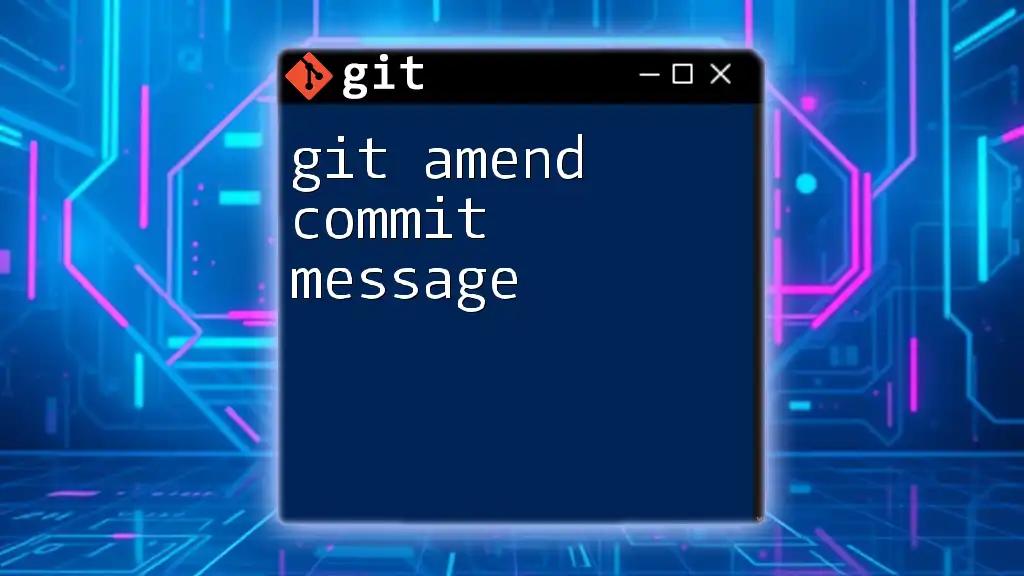
Basic Syntax for Committing in Git
The Git Commit Command
To record changes in Git, you use the `git commit` command. This command allows you to create a snapshot of your repository at a specific point in time. The `-m` flag is essential for adding a commit message directly in the command line, streamlining the process.
Basic Usage
To make a commit with a message, use the following syntax:
git commit -m "Your commit message here"
For example, you can enter:
git commit -m "Fix bug in user login mechanism"
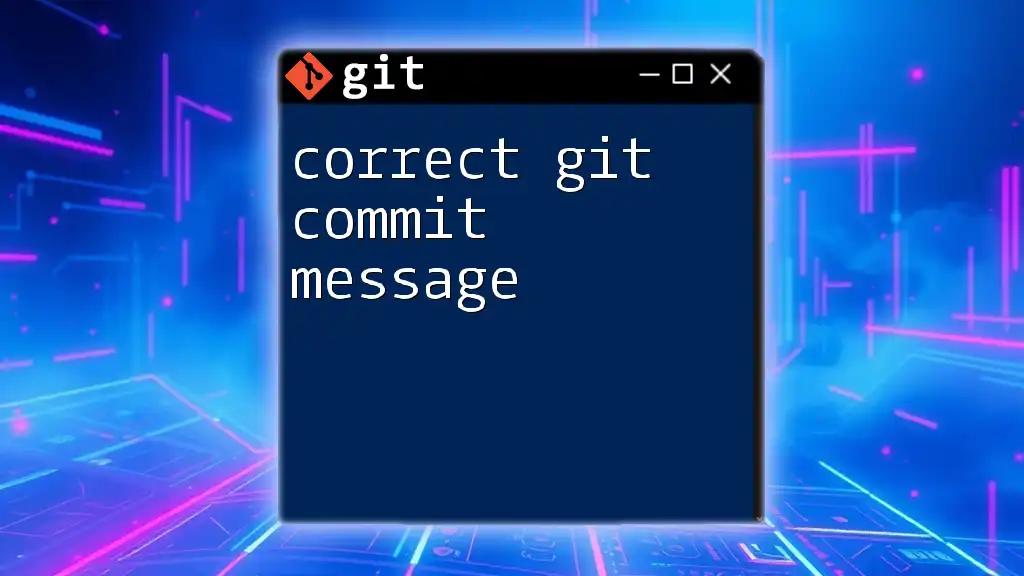
Crafting Effective Commit Messages
Structure of a Good Commit Message
A well-crafted commit message typically adheres to a specific structure. Follow this general guideline:
- Short Summary: Keep it within 50 characters. Focus on the essence of the change.
- Detailed Explanation: If necessary, provide additional context in a new paragraph, separated by a blank line.
Best Practices
When writing commit messages, consider the following best practices:
-
Use the Imperative Mood: Write in the style that commands what the commit does.
- Example: "Add user authentication feature" instead of "Added user authentication feature".
-
Clarity and Conciseness: Aim for clear, concise messages that convey the change effectively.
-
Reference Related Tasks or Issues: Whenever applicable, mention relevant tasks or issue numbers.
- Example: "Fix #123 – correct spelling in README file".
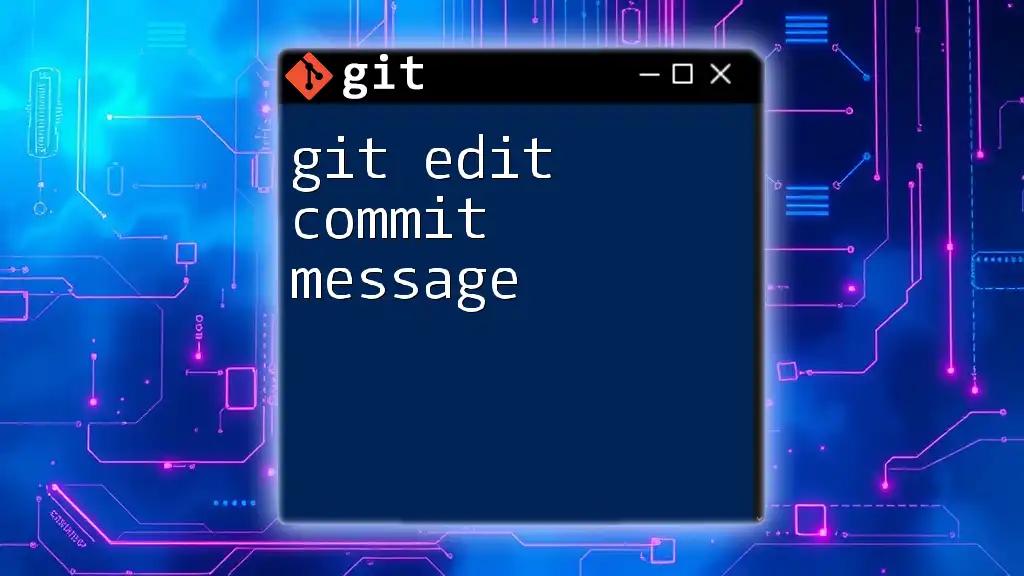
Using Multi-line Commit Messages
The Need for Multi-line Messages
Sometimes, a single line isn’t enough to describe the change you’ve made adequately. In these cases, using a multi-line commit message is more suitable.
How to Write Multi-line Commit Messages
To format a multi-line commit message in Git, use:
git commit -m "Short summary" -m "Detailed description..."
For instance:
git commit -m "Implement user profile feature" -m "This feature allows users to edit their profiles. Must address edge cases."
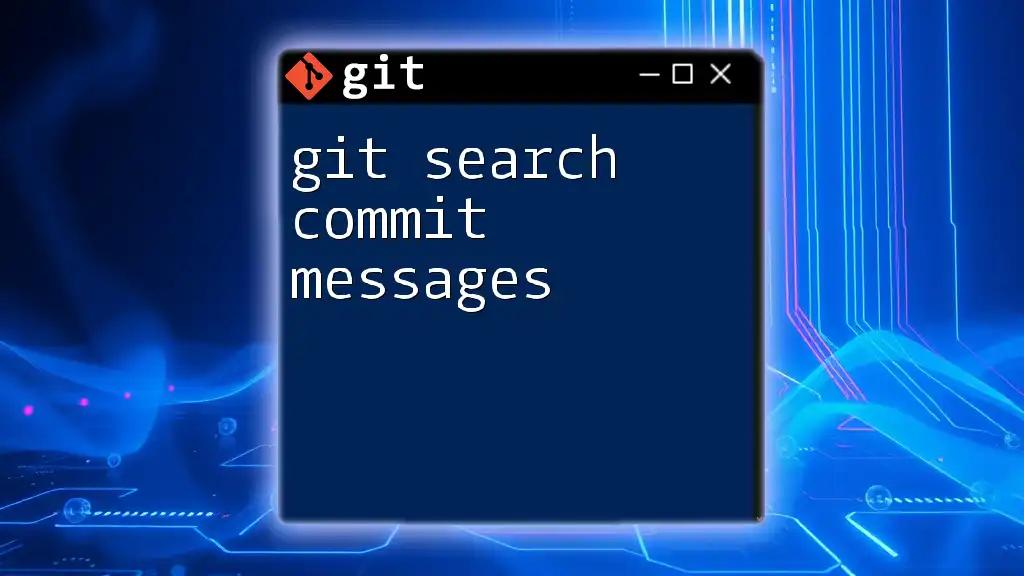
Advanced Commit Message Techniques
Using a Template for Commit Messages
Having a consistent structure for commit messages can improve their quality. Git allows users to set a commit message template.
To create and configure a commit message template, you can use this command:
git config --global commit.template ~/.gitcommit-template
You would then define your message format within the specified template file.
Editing Commit Messages After Committing
There may be instances when you need to edit a commit message after you’ve already committed your changes. Git provides a straightforward way to do this by using the `--amend` flag.
For example, to amend the last commit message, you could execute:
git commit --amend -m "Updated commit message"
This feature is particularly handy for fixing typos or clarifying the commit's purpose.
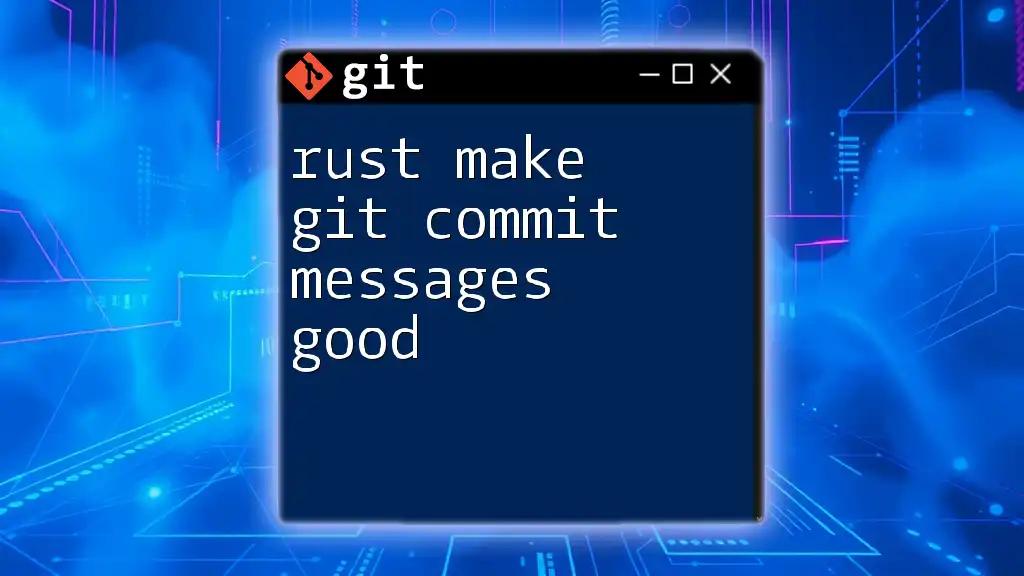
Common Pitfalls
Overly Generic Messages
One of the most common mistakes in writing commit messages is being overly vague. Generic messages like “Update files” do not convey any useful information about what was changed or why. Aim for specificity to improve clarity.
Forgetting Contextual Information
Commit messages should provide enough context to understand the change without needing to look at the code. For instance, stating "Fixed bug" provides little value compared to "Fixed bug #456 affecting user registration." Always strive to include relevant details.

Conclusion
In conclusion, knowing how to enter commit messages in Git terminal effectively is essential for maintaining clarity within your codebase. Good commit messages serve as a valuable reference for yourself and others, enhancing collaboration and project sustainability. Always strive for clarity and context in your Git workflow.
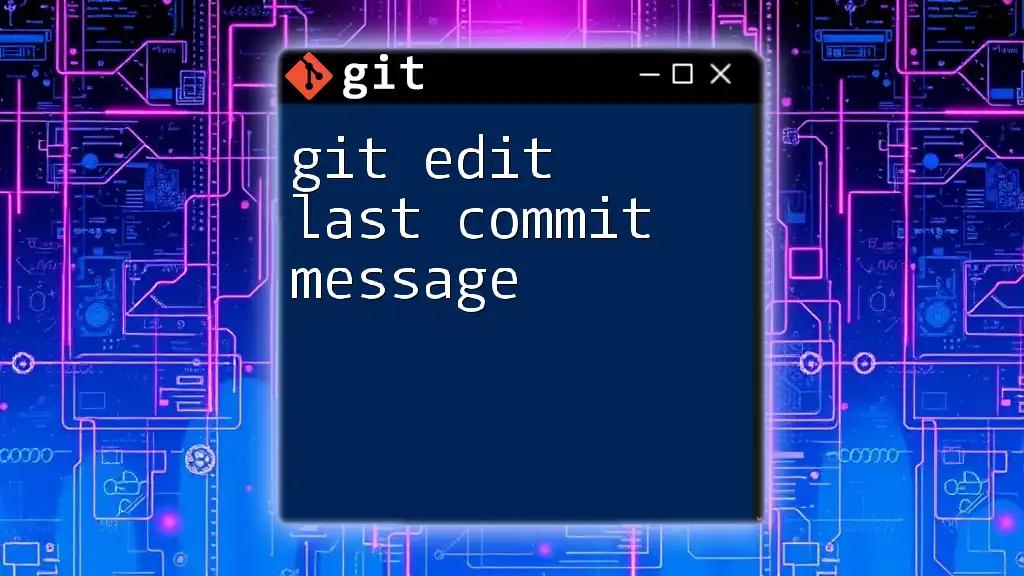
Additional Resources
Further Reading
Look for guides and documentation that delve deeper into Git and version control. Engaging with these resources can greatly enhance your understanding and proficiency.
Join the Conversation
We invite you to share your best practices and experiences with Git commit messages. What techniques have you found most effective? What challenges have you faced? Your input can help foster a richer understanding of Git for everyone!