To view the log of the first commit on a specific branch associated with a tag in Git, you can use the following command:
git log --reverse --oneline <tag_name>..HEAD
Replace `<tag_name>` with the actual name of your tag to get the first commit reference for that branch.
Understanding Git Tags
What are Git Tags?
Git tags are specific points in your repository's history that are marked for reference. They serve as a valuable tool for indicating significant milestones in your project, such as release versions. There are two types of tags in Git:
- Lightweight tags: Essentially bookmarks to a specific commit, they’re quick to create and do not store any additional information.
- Annotated tags: These are more comprehensive and are stored as full objects in the Git database. They contain metadata, such as the tagger's name, email, and date, along with an optional message.
Why Use Tags?
Tags are vital in software development for several reasons:
- Version control: They allow teams to label and track releases, making it easier to reference specific versions when necessary.
- Milestones: Tags can signal the completion of significant features or achievements within your project.
- Rollbacks: If issues arise after a release, tags provide a convenient way to revert to a previous stable point in the codebase.
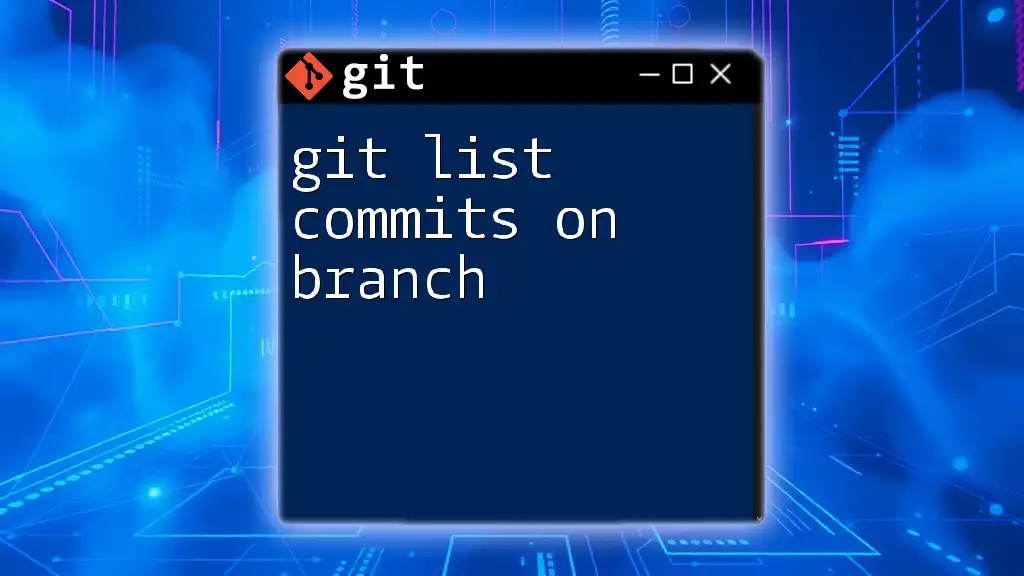
The Secret Behind Git Branches
What is a Git Branch?
Branches in Git act like independent lines of development within a project. They enable multiple team members to work concurrently on different features or fixes without interfering with each other’s progress.
How Branches Enhance Workflow
Using branches enhances the workflow in several significant ways:
- Isolation: Each branch can represent a unique feature or bug fix, keeping changes isolated until they are ready to be incorporated into the main codebase.
- Experimentation: Developers can explore new ideas without jeopardizing the stability of the main branch, typically named `main` or `master`.
- Easy merging: Once the work on a branch is finished, it can be merged back into the main line of development, facilitating a straightforward integration process.
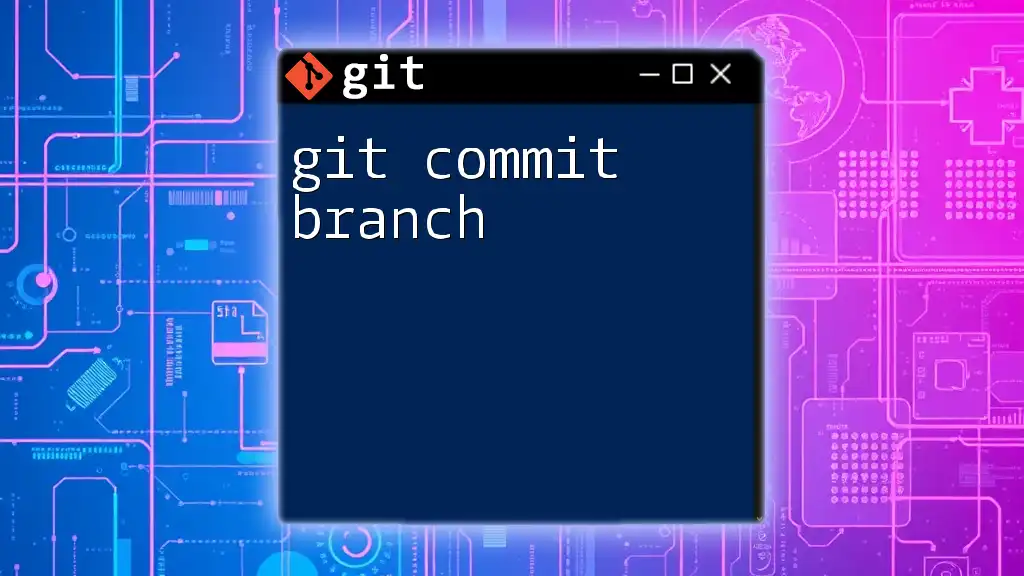
Committing to a Branch
Making Your First Commit
To start working on a new feature, you never want to commit directly to the main branch. Instead, follow these simple steps to create a branch and make your first commit:
-
Create a new branch:
git checkout -b new-feature
-
Add files and changes:
echo "Initial commit" > readme.txt git add readme.txt
-
Commit changes:
git commit -m "First commit on new-feature branch"
This command creates a new branch called `new-feature`, adds a file called `readme.txt`, and commits it with a descriptive message.
Understanding Commit History
The commit history in Git is a chronicle of changes made to the project. Visualizing and tracking this history is essential to understand where your project stands and how it has evolved over time. The `git log` command allows you to see the history of commits, providing insights into both your work and the contributions of your team.
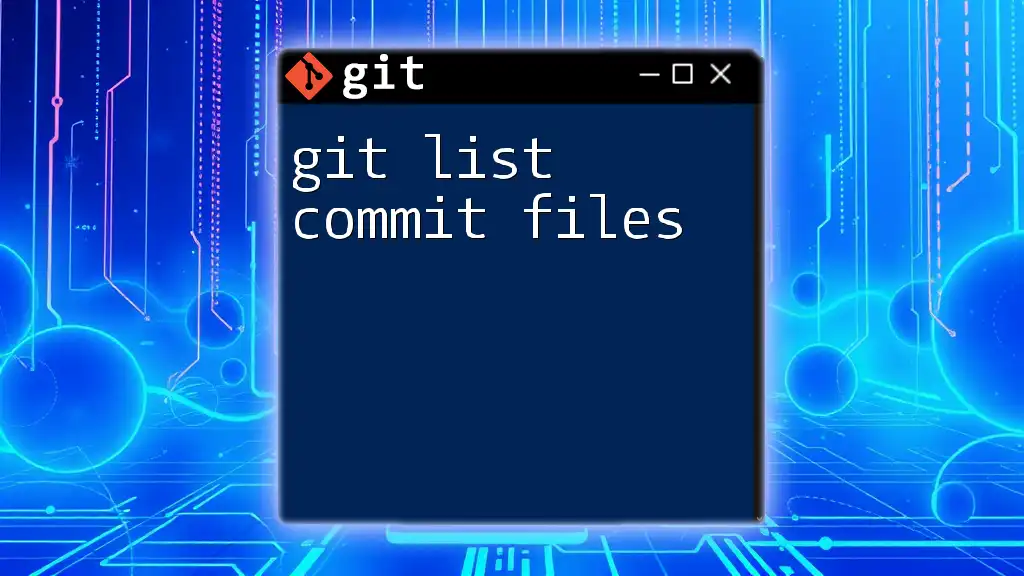
Retrieving the First Commit in a Branch
The Git Log Command
The `git log` command is an essential tool for exploring the history of commits in your repository. Here are some of its useful options:
- `--oneline`: Displays a simplified view of each commit in a single line.
- `--graph`: Illustrates the branching and merging history visually.
- `--decorate`: Shows references like branch names and tags alongside commit messages.
To see a concise commit history, you could simply run:
git log --oneline
Finding the First Commit on a Branch
To find the first commit on a specific branch, you can use the following command:
git log --reverse --pretty=format:'%h - %an, %ar : %s' new-feature
This command reverses the order of commits and formats the output to provide a clear and readable list of commits.
Example Explained
In the example above:
- `%h`: Abbreviated commit hash.
- `%an`: Author name.
- `%ar`: Time since the commit was made.
- `%s`: Commit message.
This output will give you a detailed view of all commits, starting from the first one on the `new-feature` branch, allowing you to track its history easily.
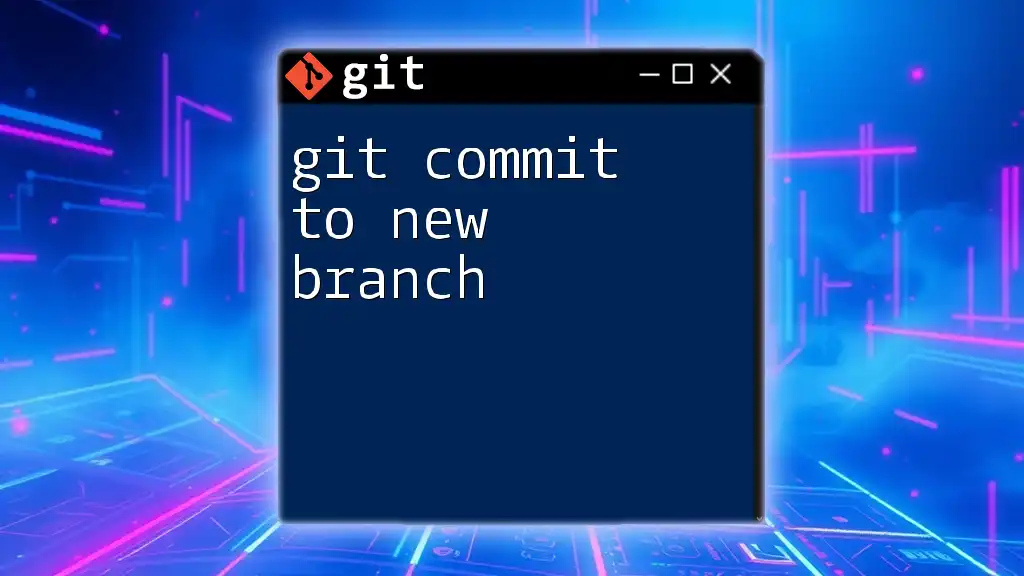
Tagging the First Commit
How to Create a Tag
Once you retrieve the first commit of your branch and wish to mark it, creating a tag is a straightforward process. Use the following command to create an annotated tag:
git tag -a v1.0 -m "First release of new feature"
In this command:
- `-a v1.0`: Makes an annotated tag named `v1.0`.
- `-m "First release of new feature"`: Adds a message to describe the tag.
Verifying Your Tag
After creating the tag, it's crucial to verify that it has been successfully added. Use the following command to display a list of tags in your repository:
git tag
This will show all existing tags, including the newly created `v1.0`, confirming the successful tagging of your first commit.
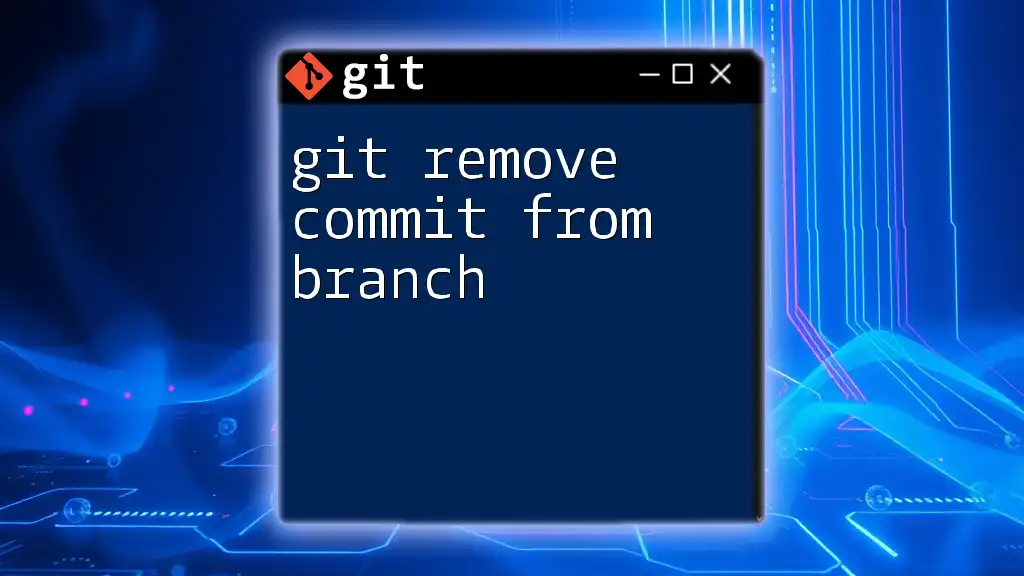
Conclusion
In conclusion, being able to retrieve the first commit on a branch and tag it is fundamental for effective version control using Git. Tags act as markers for significant points in your project's lifecycle and can greatly enhance the clarity of your versioning strategy. By employing branches effectively and utilizing the `git log` command, you can maintain a well-organized codebase that reflects the hard work put into development.
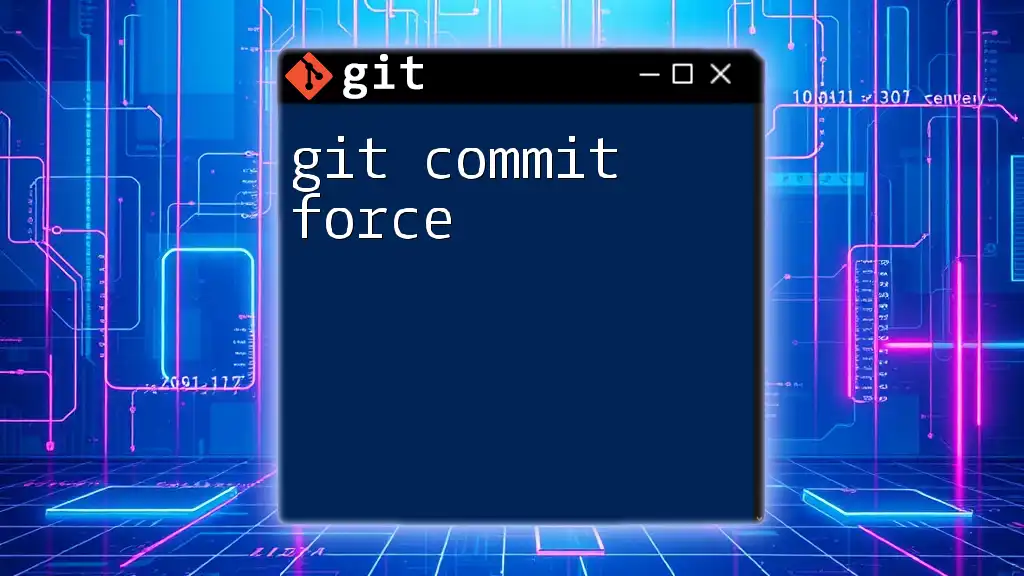
Additional Resources
Helpful Resources for Further Learning
- Check out [Git Documentation](https://git-scm.com/doc).
- Explore [Pro Git Book](https://git-scm.com/book/en/v2) for in-depth Git concepts.
- Consider online courses from platforms like Udemy or Coursera that elaborate on Git commands and practices.
Engaging with the Community
Engaging with community forums and discussion boards can provide insights and solutions for any Git-related challenges you face. Sharing experiences can vastly enrich your understanding of Git practices and encourage collaboration with other developers.