Visualizing git commits to a branch can be done using the `git log` command with a specific branch reference, which helps to track changes and understand the project history.
git log --oneline --graph --decorate <branch_name>
Understanding Git Branches
What is a Git Branch?
A git branch is essentially a pointer to a specific commit. In Git, branches allow you to work on different features or tasks in isolation from the main codebase, usually referred to as the main or master branch. This feature enables collaborative development and helps manage changes without affecting the stable version of your project.
Branches facilitate parallel development by letting multiple team members work on different features simultaneously. Each branch preserves its own history, allowing for easy switching, merging, or even deletion when a feature is completed.
Importance of Visuals in Git
Visualizing Git commits is crucial for understanding project history and the relationships between different branches. When you can see how commits are structured and how they connect, it becomes simpler to comprehend:
- The progression of a feature.
- How fixes and updates have rolled out.
- Collaborative efforts and contributions from multiple developers.
Visualizations not only make these relationships clear but also help in troubleshooting conflicts and understanding the overall flow of changes.
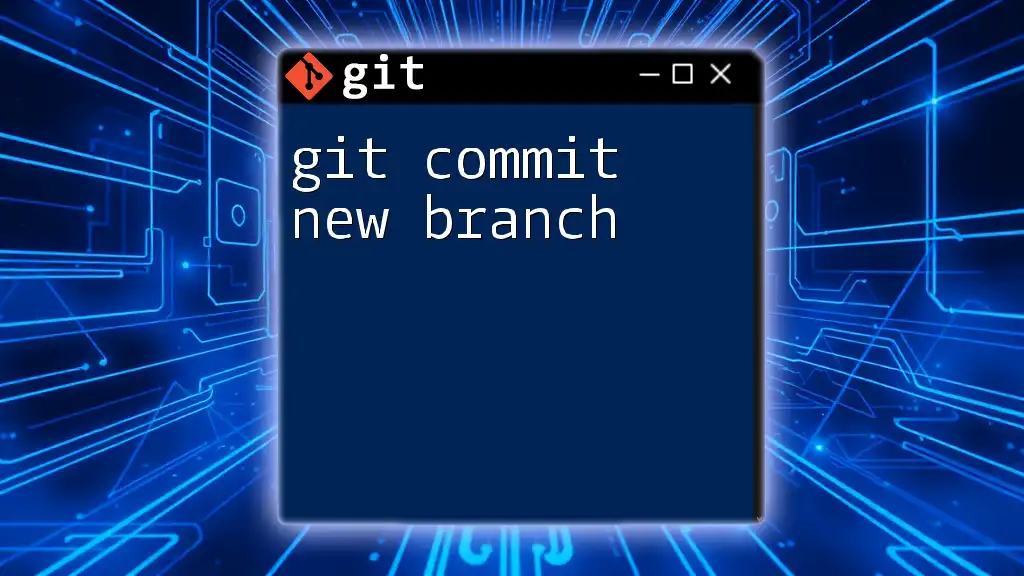
Tools for Visualizing Git Commits
Git Log
The `git log` command is one of the essential tools in Git for visualizing history. By default, it presents a chronological list of commits, detailing the commit messages, authors, and timestamps. However, it comes with a variety of options to enhance the output.
For example:
git log --oneline
This command condenses each commit to a single line, showing only the commit hash and the message, which simplifies the output for a quick overview.
To add a visual element, you can use:
git log --graph --decorate --oneline
This command generates a visual representation of commit history in a graph format. It will show branches, merges, and more, making it much easier to track how branches interact over time.
Git Show
To visualize a specific commit, the `git show` command comes in handy. This command provides a detailed view of a commit, including what changes were made, along with the commit message and metadata.
Here’s how you can use it:
git show <commit-hash>
Replace `<commit-hash>` with the actual commit ID. This command is highly useful when you need to analyze a particular commit's changes or understand context regarding a specific point in development.
Gitk
For those who prefer a graphical user interface, `gitk` is an excellent option. It provides a visual representation of the commit history, branching, and merges.
To launch `gitk` for a specific branch, use:
gitk <branch-name>
Upon execution, it opens a window displaying a tree-like structure showing the commits. You can click through different nodes to explore commit messages and details, thereby gaining insight into project evolution.
External Visualization Tools
While Git has powerful built-in tools, many developers benefit from third-party visualization tools. Here are some popular options:
- SourceGraph: An advanced code exploration tool that allows you to visualize how code segments interact.
- GitKraken: A user-friendly graphical interface for Git that enhances commit visualization with intuitive drag-and-drop features.
- GitHub Desktop: Ideal for beginners, providing an easy overview of repositories and branch changes in a clean interface.
While built-in tools are powerful, third-party options may offer unique functionalities, so it's worth exploring what best suits your workflow.
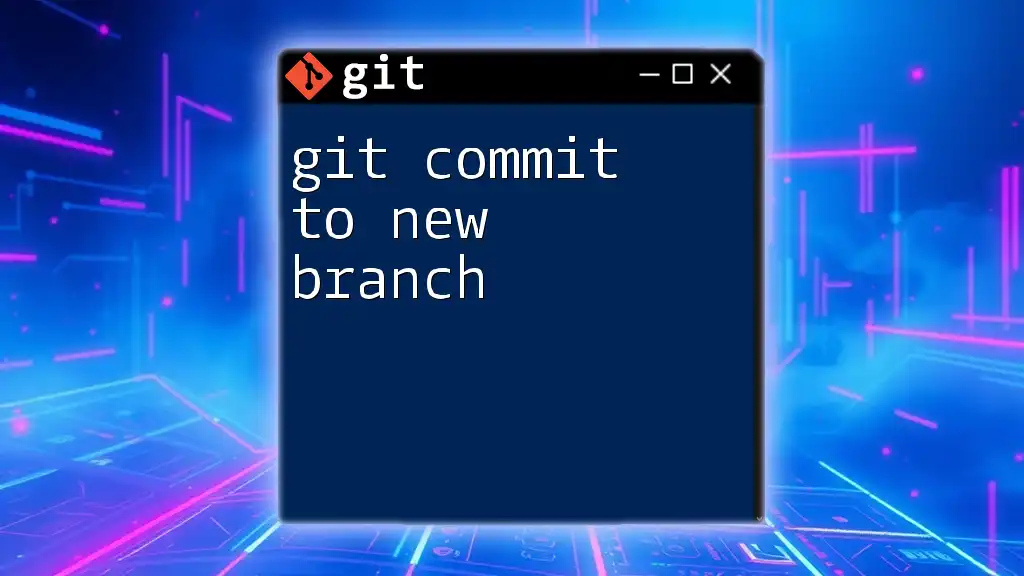
Working with Git Graph
Installing Git Graph in Visual Studio Code
One of the most popular extensions for visualizing Git commits is Git Graph, which integrates seamlessly with Visual Studio Code. To install:
- Open Visual Studio Code.
- Navigate to the Extensions view by clicking on the Extensions icon or pressing `Ctrl + Shift + X`.
- Search for "Git Graph" and click on the install button.
Using Git Graph
Once installed, you can open Git Graph through the command palette by pressing `Ctrl + Shift + P` and typing "Git Graph: View Git Graph."
The visualization displayed allows users to see branch history, commit information, and even perform actions like creating new branches directly from the interface. This tool is essential for visual and collaborative environments since it simplifies understanding complex commit histories.
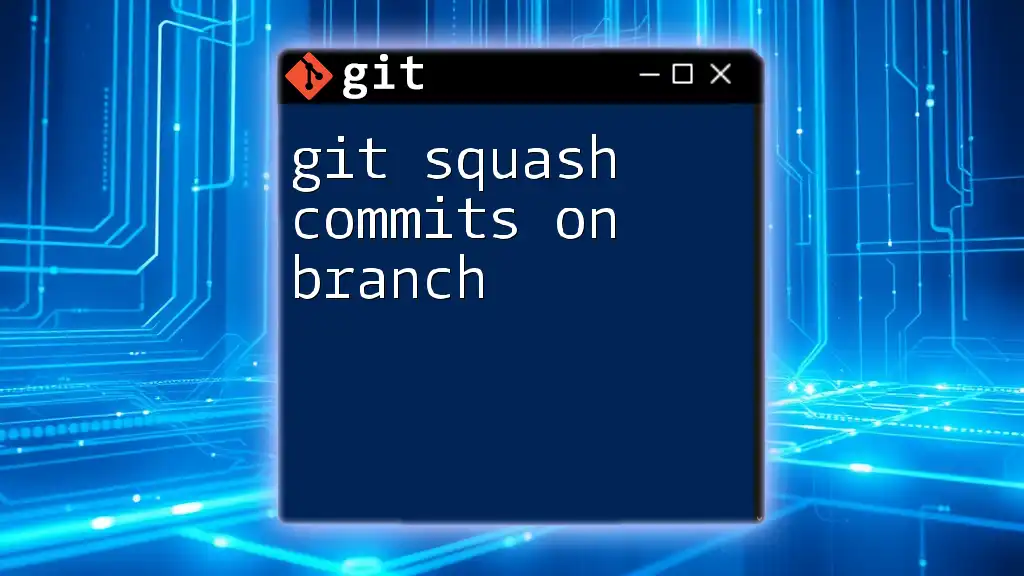
Visualizing Commits in Different Scenarios
Tracking Feature Development
When working on feature branches, visualizing commits to these branches is particularly important. It helps you monitor progress and identify when key features are ready for merging back into the main branch. For instance, viewing commit histories in a branching diagram helps determine if your feature is diverging too much from the main project, allowing timely intervention if necessary.
Monitoring Release Progress
During release cycles, visualizing commits provides clarity on what has been included in a release. It helps project managers and developers to ensure that all intended features and bug fixes are included, reducing the risk of last-minute surprises. You can view the commit logs leading up to a release to understand the trajectory of changes and prepare for the deployment accordingly.
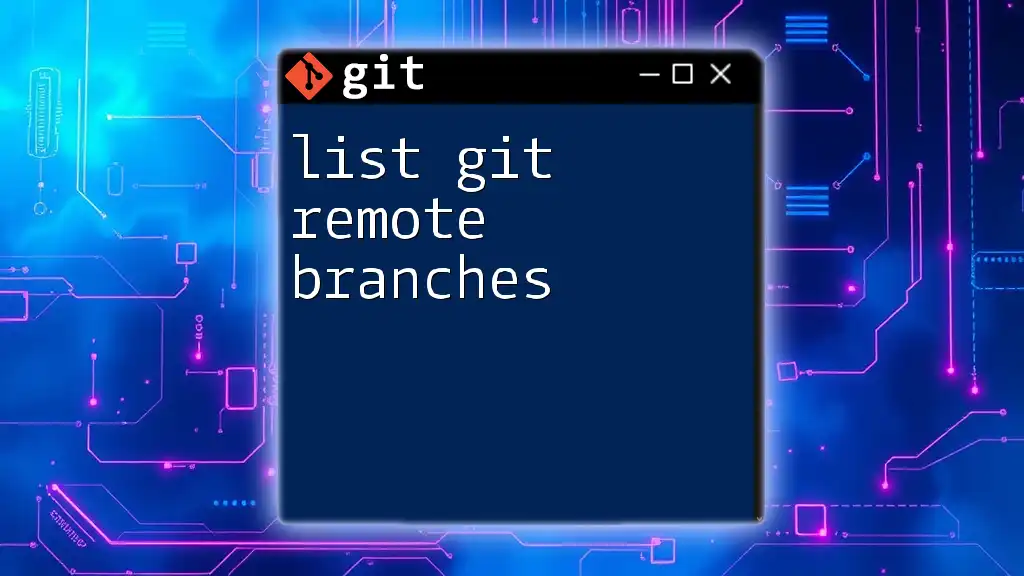
Best Practices for Viewing Git Commits
Consistency in Commits
Commit messages should be clear and consistent. Good messages help others understand the purpose behind changes without having to dive into the code. For example, an ideal commit message follows this template:
- What the change is: "Fix typo in README."
- Why the change was made: "Improved clarity for installation instructions."
This practice ensures that your history is informative and easier to navigate.
Regularly Check Branches
Visualizing commits should not be a one-off task. Regularly checking the commit history can prevent confusion and help keep branches organized. Make it a habit to visualize the commit history, especially when merging, to observe potential conflicts early.
Collaborating with Teams
When working in teams, shared visualizations become crucial. Tools like GitHub or GitLab provide common platforms where team members can review commits together. Encourage regular meetings to discuss these visualizations; it fosters collaboration and ensures everyone is on the same page regarding project progress.
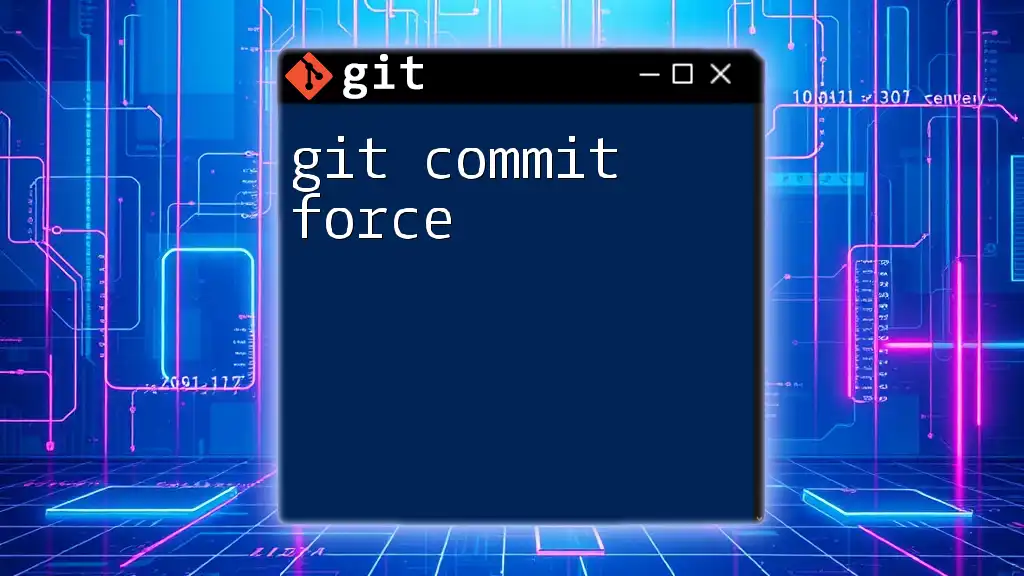
Conclusion
Visualizing Git commits to a branch is an invaluable skill for developers, facilitating better understanding and management of project histories. By employing tools like `git log`, `git show`, and Git Graph, you can gain insights into your project's direction, make informed decisions, and collaborate more effectively.
Incorporate these visualization techniques into your Git practices, and you'll find that not only does your productivity increase, but your ability to manage complex projects becomes more manageable and less stressful.
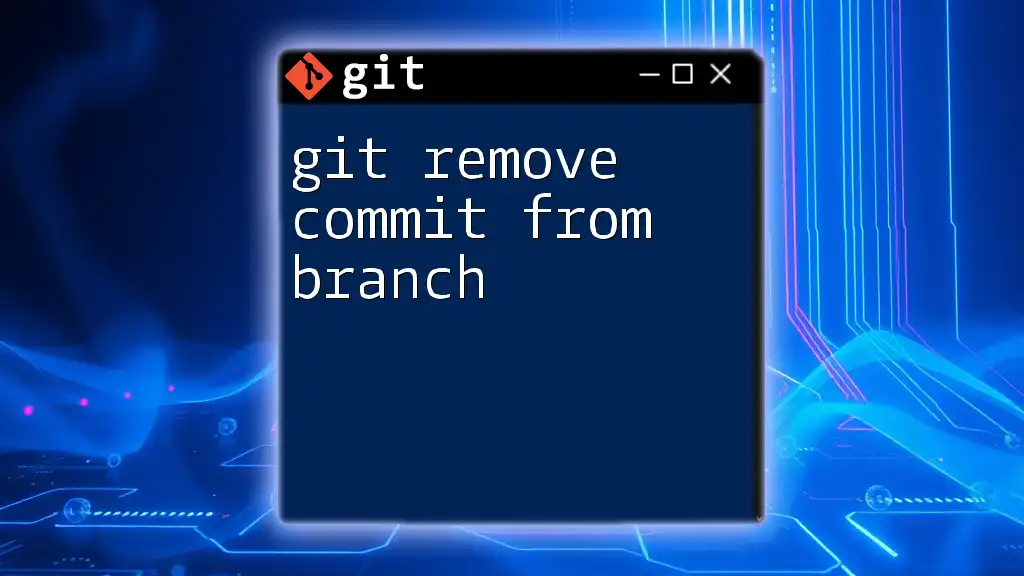
Additional Resources
Explore the official Git documentation for an in-depth understanding, alongside recommended courses available online for mastering Git commands and visualization strategies.