In Git, the `webpack.config.js` file is often excluded from commits due to its frequent changes in configuration, which can clutter the version history, and thus it's typically listed in the `.gitignore` file to prevent accidental commits.
echo 'webpack.config.js' >> .gitignore
Understanding `webpack.config.js`
What is `webpack.config.js`?
`webpack.config.js` is a crucial configuration file for Webpack, a powerful module bundler for JavaScript applications. It defines how Webpack processes and transforms application files, allowing developers to customize various aspects of their build process. A typical `webpack.config.js` might look like this:
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist')
}
};
This simple setup demonstrates how to specify the entry point of your application and define where the output file should be placed after bundling.
Common Customizations
Developers often customize `webpack.config.js` to tailor their builds according to project needs. This might include specifying loaders for different file types, setting up plugins for additional functionalities, or even defining multiple entry points.
For example, here’s how to add a Babel loader to handle JavaScript files:
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
}
]
}
};
This additional configuration allows Webpack to process JavaScript files with Babel, providing the ability to use the latest JavaScript features.
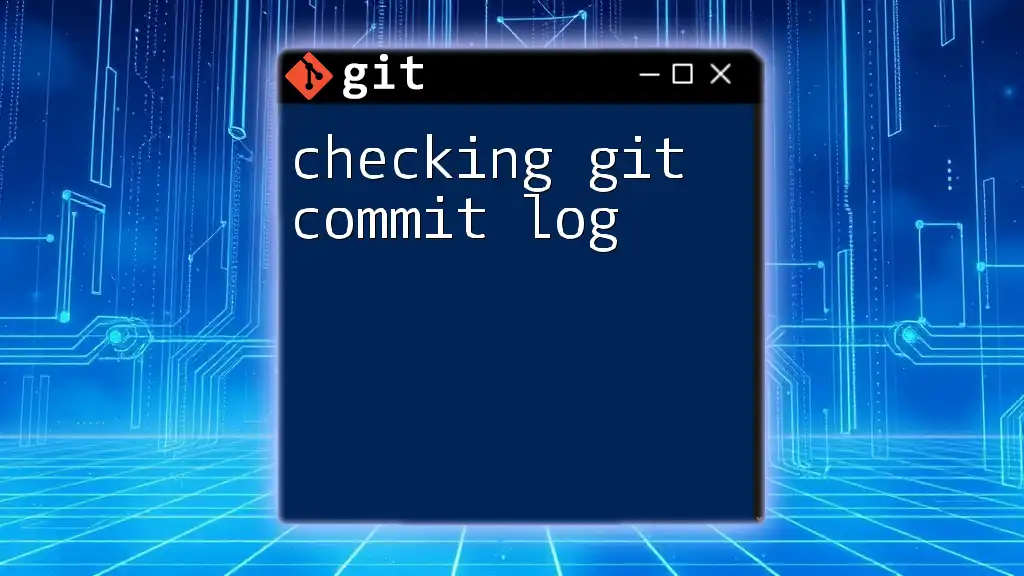
Why `webpack.config.js` is Often Ignored
Reasons Developers Exclude It from Git
Many developers choose not to commit `webpack.config.js` to their repositories for several reasons.
-
Environment-specific settings: Often, different environments (e.g., dev, staging, production) require unique configurations. Directly committing these settings can lead to misconfigurations and unexpected behaviors.
-
Sensitive information: Config files may inadvertently include sensitive information, such as API keys or database credentials. Committing these can expose critical data.
-
Local machine differences: Developers may have different local setups or global variations. This can lead to challenges if one person's configuration works while another's does not.
Git Ignore Patterns
To prevent `webpack.config.js` from being committed, developers often rely on a `.gitignore` file. This special file tells Git which files or directories to ignore.
To include `webpack.config.js` in your `.gitignore`, simply add the following line:
webpack.config.js
This ensures that any changes made to `webpack.config.js` are not tracked by Git.
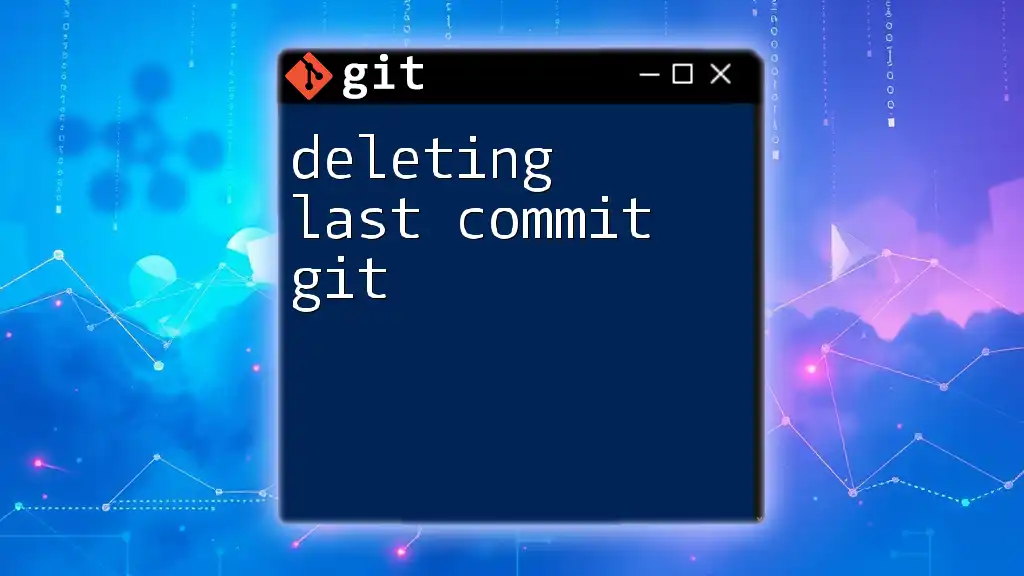
When to Commit `webpack.config.js`
Team Collaboration Scenarios
In collaborative development environments, having a consistent `webpack.config.js` is vital. It allows all team members to build and run the project with the same settings. A shared configuration ensures that everyone is aligned, reducing friction when onboarding new team members.
For example, in a team project, your `webpack.config.js` may include configuration for handling images, CSS, and other assets, which looks like this:
module.exports = {
module: {
rules: [
{
test: /\.(png|jpg|gif)$/,
use: [
{
loader: 'file-loader',
options: {
name: '[path][name].[ext]'
}
}
]
}
]
}
};
Best Practices
To effectively manage and share `webpack.config.js` among team members, adhere to certain best practices:
- Always document changes: Maintain clear comments explaining the purpose of various configurations.
- Version control your configuration: Consider committing the file only in certain scenarios where it's safe and required.
Handling Sensitive Information
Using Environment Variables
One effective way to manage sensitive information in `webpack.config.js` is by using environment variables. Instead of hardcoding API keys, you can access environment variables using the `process.env` object. Here's an example:
const apiKey = process.env.API_KEY;
module.exports = {
plugins: [
new SomePlugin({
API_KEY: apiKey
})
]
};
This approach not only helps in keeping sensitive data secure but also allows for different values in different environments.
Using `.env` Files
An alternative method to manage environment variables is by utilizing `.env` files. These files can house your sensitive information and be accessed in your configuration file using the `dotenv` package.
First, install the package:
npm install dotenv
Then, create a `.env` file in your project root:
API_KEY=your-secret-api-key
Then, in your `webpack.config.js`, load the environment variables:
require('dotenv').config();
module.exports = {
plugins: [
new SomePlugin({
API_KEY: process.env.API_KEY
})
]
};
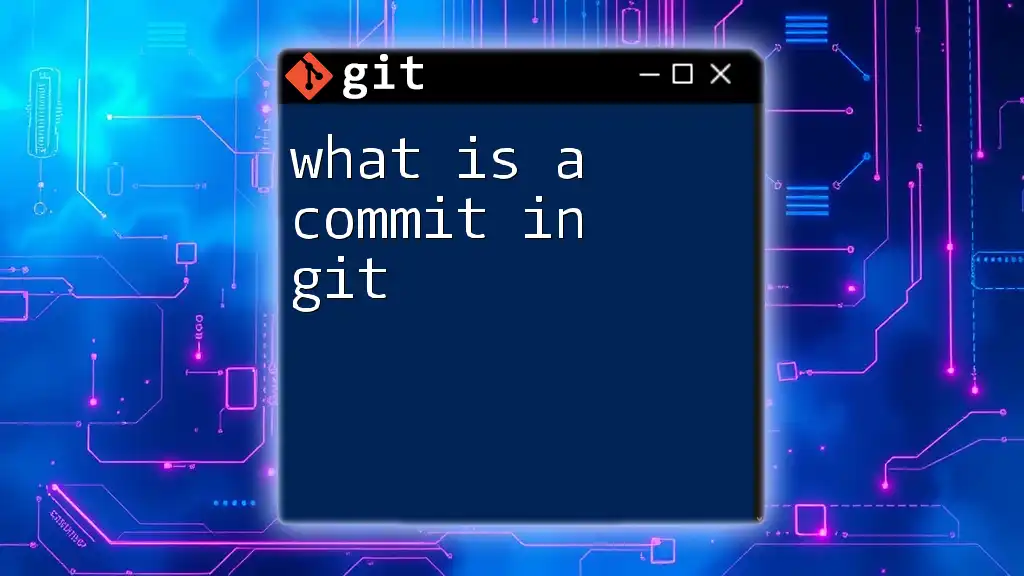
Structuring Your Repository
Organizing Configuration Files
As projects grow, modularizing configuration files becomes essential. Instead of using a single `webpack.config.js`, consider creating specific files for different environments.
This approach could look like this:
- `webpack.prod.config.js`
- `webpack.dev.config.js`
Each file can contain configurations suited for its respective environment, which enhances clarity and maintainability.
Versioning Configurations
Properly versioning your configurations is critical. When changes are made to `webpack.config.js`, ensure those changes are tracked. To help manage configurations for different environments, consider using Git branches. For instance, branch out different configurations and merge them back when stable.
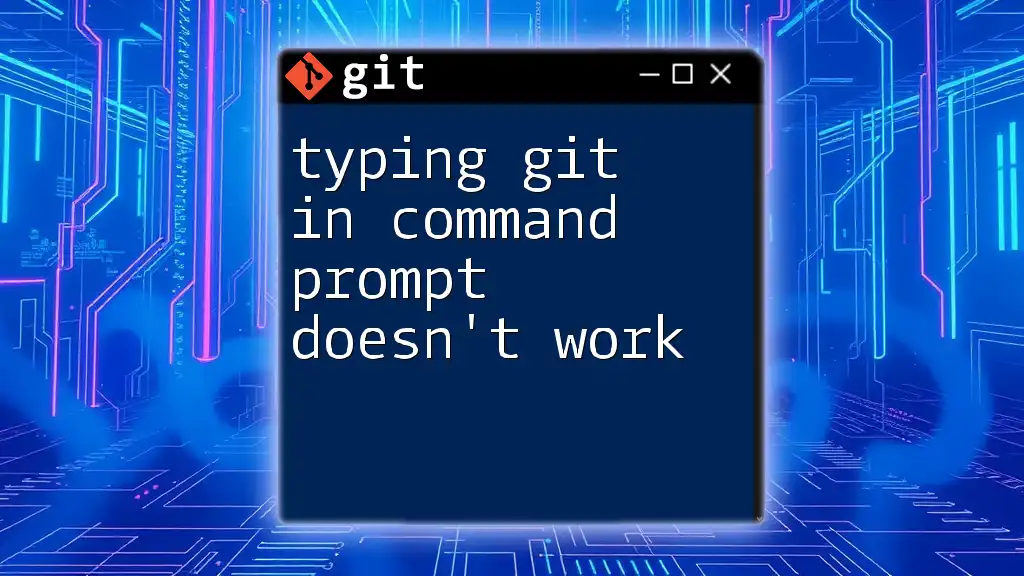
Conclusion
In summary, the statement "webpack.config is not committable in Git" holds true under certain circumstances yet can also be beneficial in collaborative settings when handled prudently. Understanding when to exclude or include `webpack.config.js` is vital for secure and efficient project development.
By employing best practices such as managing sensitive information through environment variables and maintaining organized configuration files, you can significantly improve code maintainability and security, leading to smoother team collaboration.
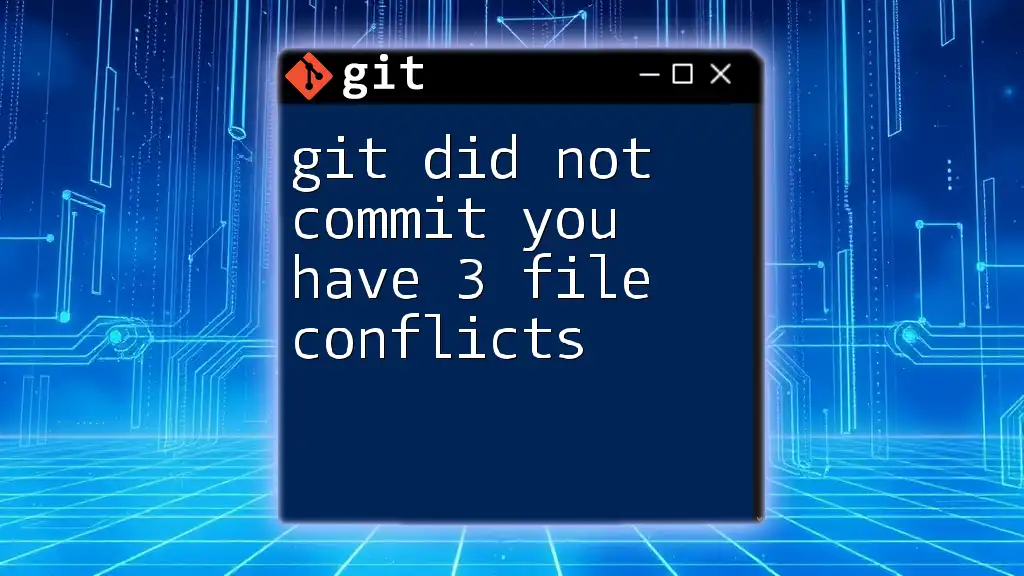
Additional Resources
For more detailed information on Git practices and Webpack configurations, explore the official documentation:
- [Webpack Documentation](https://webpack.js.org/concepts/)
- [Git Documentation](https://git-scm.com/doc)
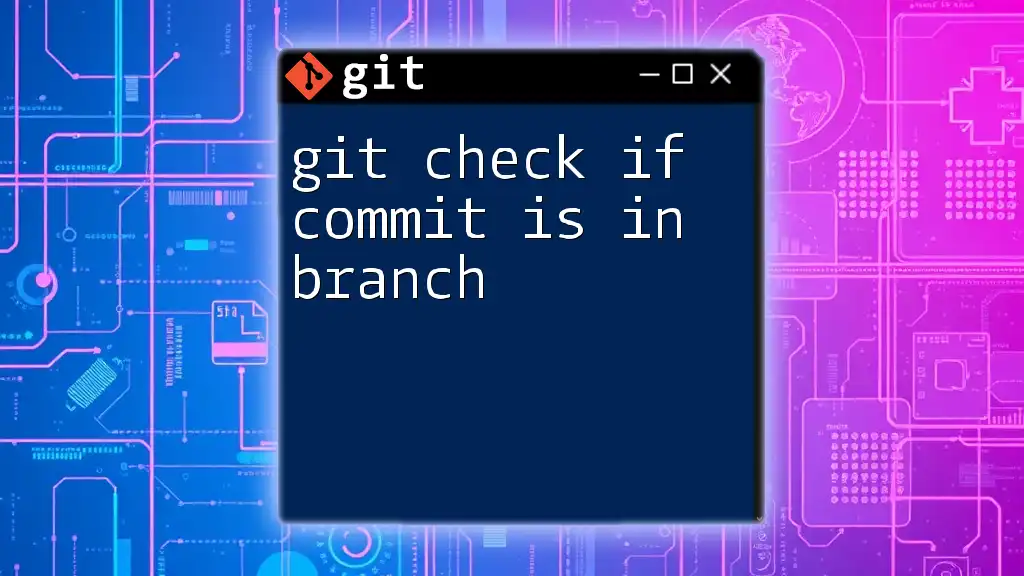
Call to Action
Ready to enhance your understanding of Git and improve your workflow? Join our platform for concise and practical guides on Git commands tailored for developers of all skill levels. Share your experiences and put your newfound knowledge to the test!