The command `git clean -n` is used to preview which untracked files and directories will be removed from your working directory without actually deleting them.
git clean -n
What is `git clean`?
`git clean` is a command designed to remove untracked files or directories from your working directory. Untracked files are those files that are not being tracked by Git, meaning they haven’t been staged or committed. This command helps maintain a tidy workspace by allowing you to clean up files you no longer need or that were generated during the development process.
Differences between Tracked and Untracked Files
- Tracked files are files that have been added to the staging area (with `git add`) and are part of the version history.
- Untracked files are new files that exist in your working directory but have not been staged or committed.
Understanding the difference is crucial, as `git clean` operates specifically on untracked files, leaving your tracked files untouched.
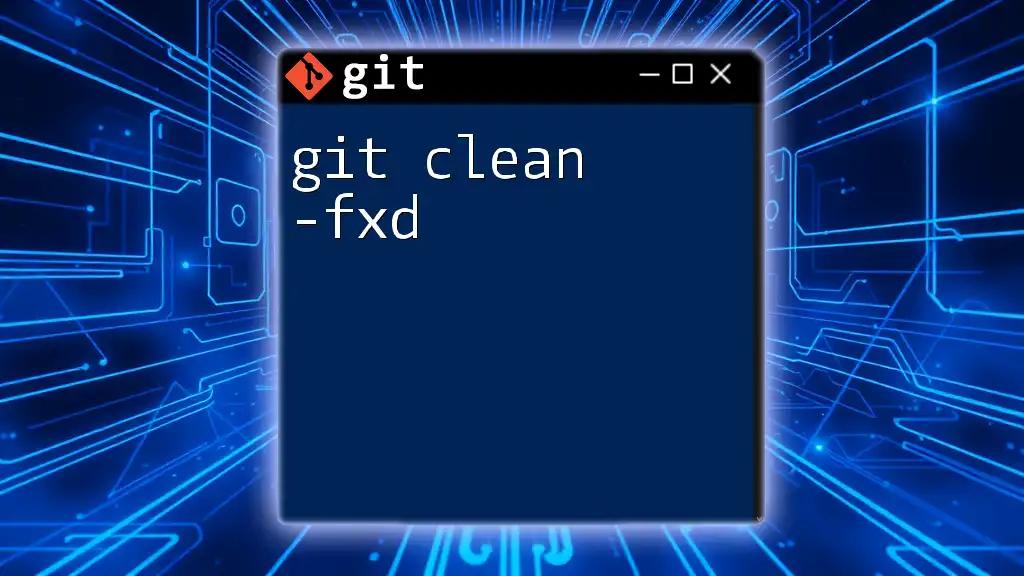
Understanding the `-n` Option
What does the `-n` flag do?
The `-n` flag stands for “dry run.” When you use this option, Git simulates the removal of untracked files without actually deleting anything. It will display what files would be removed if you executed the command without this flag.
Importance of Using the `-n` Option
Using `git clean -n` is essential for preventing accidental data loss. By running the command in simulation mode, you can review which files are marked for deletion. This step is particularly important in large projects, as it helps you ensure that no important files are lost.
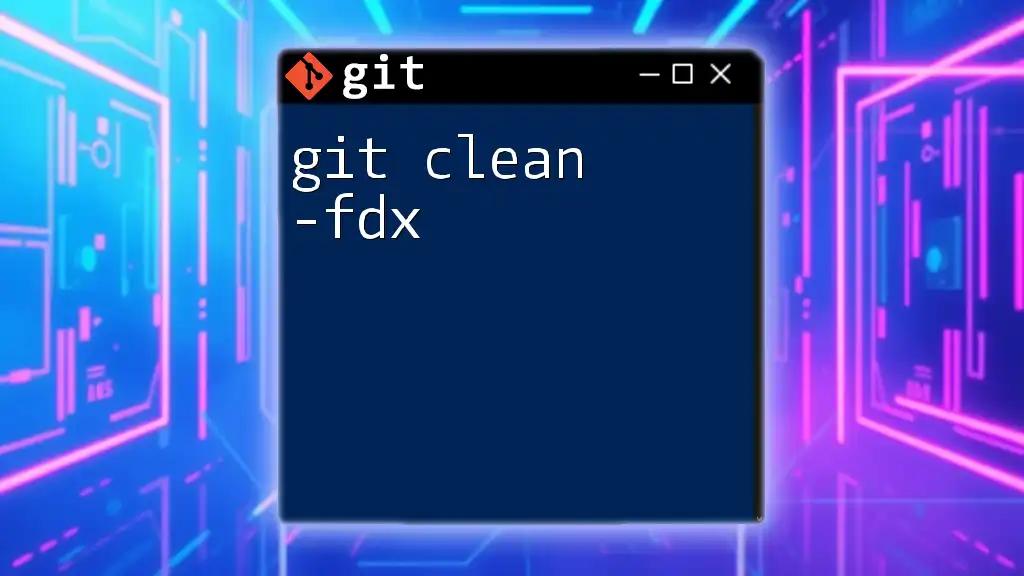
Use Cases for `git clean -n`
Cleaning Up Your Workspace
Over time, your working directory may accumulate untracked files, such as temporary files, build outputs, or ignored dependencies. Running `git clean -n` allows you to quickly identify and manage these files, keeping your workspace organized.
Preparing for a Clean Build
When switching branches or preparing to deploy, it's often beneficial to ensure no old or unwanted files interfere with your workflow. Cleaning untracked files can help achieve a stable and predictable build environment.
Case Study
Imagine you're working on a feature branch and have created some temporary files for testing. After finishing your work, you decide to return to the main branch. Running `git clean -n` enables you to see which temporary files are untracked, thereby providing insight before you execute a cleaner command.
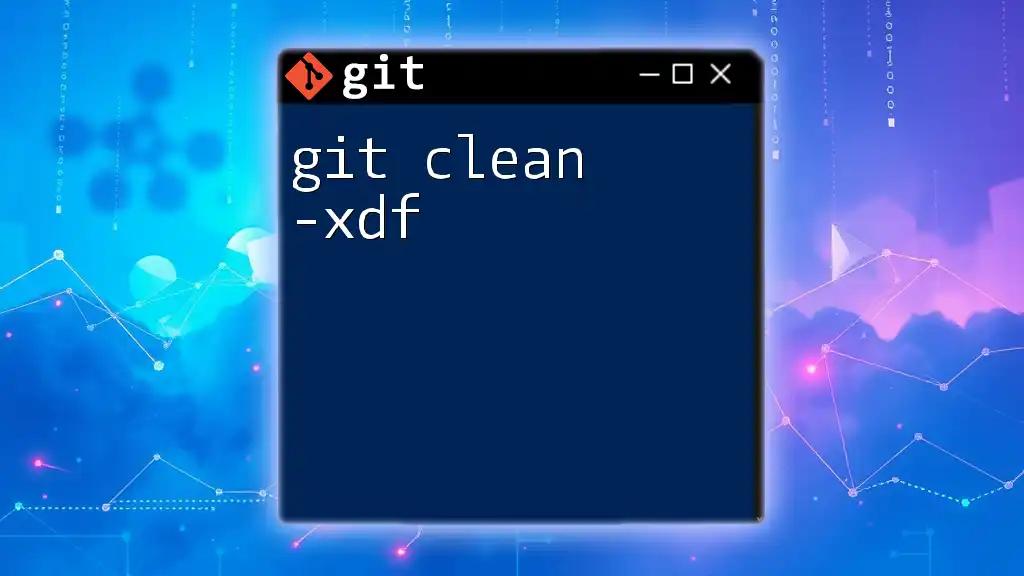
How to Use `git clean -n`
Basic Syntax
To begin using this command, the basic syntax is as follows:
git clean -n
Common Flags with `git clean`
- `-d`: This flag removes untracked directories as well. By default, `git clean` only removes untracked files, so using `-d` is necessary if you want to clean up also directories.
- `-x`: This option will cause Git to ignore your `.gitignore` rules, meaning it will also delete files that are usually ignored.
- `-f`: Use this flag to forcefully remove files. Note that `-f` is necessary to actually delete files, as Git protects against accidental data loss.
Example Commands
-
Basic example to display what will be cleaned:
git clean -n
-
To include untracked directories in the output:
git clean -nd
-
To see all files, including ignored ones:
git clean -n -x
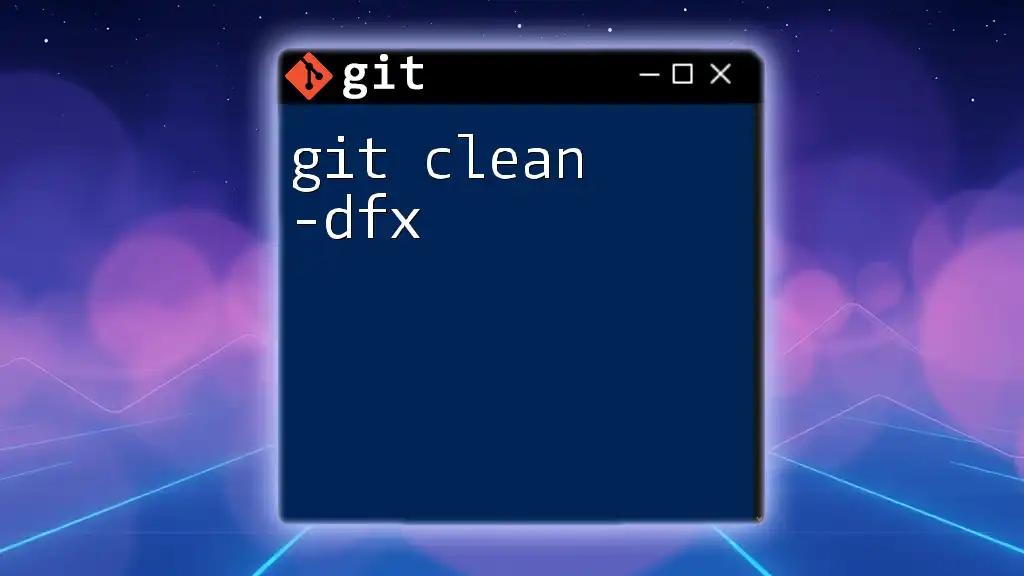
What Happens When You Execute `git clean -n`?
When you run `git clean -n`, Git provides a list of files and optionally directories that it would remove. This output is critical for allowing you to make an informed decision about what to clean.
Output Format
The command will typically output the paths of untracked files that are potential candidates for deletion. Files will be listed line-by-line, allowing for easy review.
Interpreting Results
After running the command, review the output carefully. Take note of any files that are important and should not be deleted. This assessment can prevent accidental deletions and help maintain your workflow integrity.
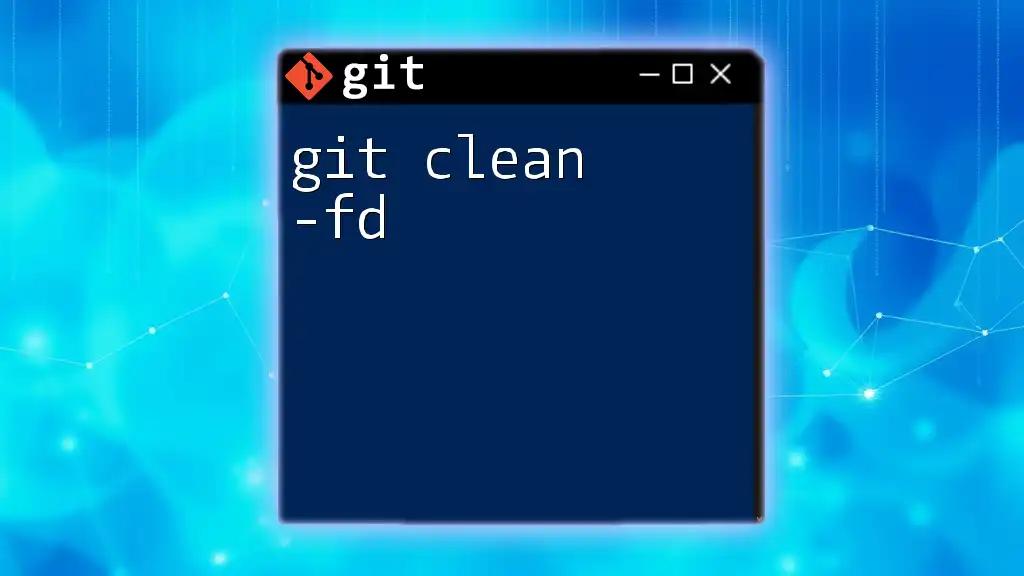
Best Practices When Using `git clean`
Always Run in Simulation Mode First
Before executing any destructive command, it’s best practice to use the `-n` option. This habit ensures you always know what files will be affected before making any changes.
Review Changes Before You Clean
Don’t rush into executing `git clean`. Make it a habit to check the output of `git clean -n` every time. Knowing what will be removed allows you to back up important files if needed.
Backup Important Work
If you have untracked files that you think might be necessary later, consider moving them to a temporary directory or using Git stashing to preserve their state before cleaning.
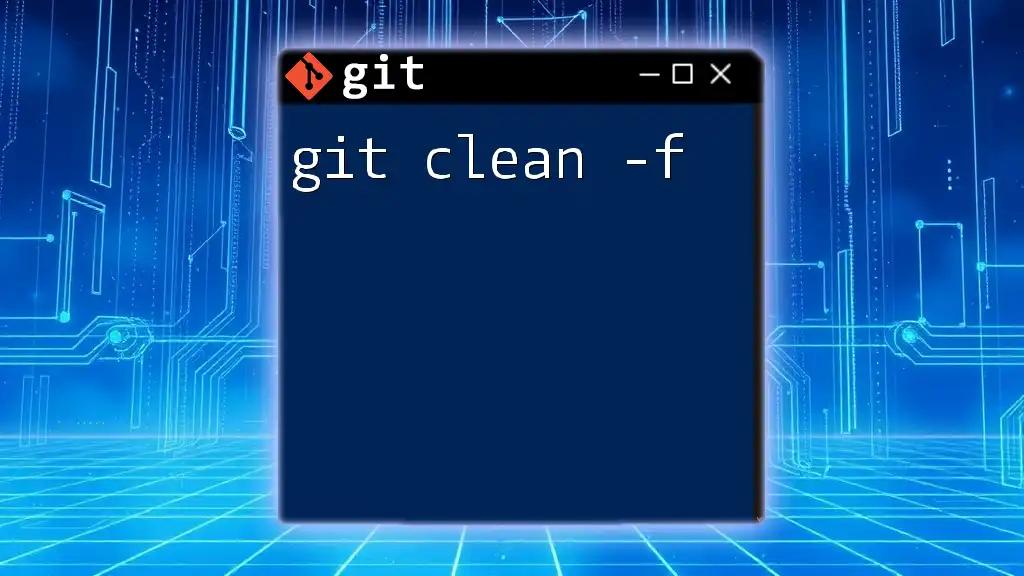
Common Mistakes to Avoid
Forgetting to Add the `-n` Flag
Executing `git clean` without the `-n` flag can lead to data loss. Always remember that this command will permanently delete files unless you double-check through simulation first.
Improper Understanding of Untracked vs. Ignored Files
It’s important to understand that `git clean` targets only untracked files. Be cautious if you believe you have important files hidden in your `.gitignore`.
Assuming `git clean` vs. `git rm`
`git clean` is for untracked files, while `git rm` is for tracked files that you wish to remove from the staging area and delete. Mixing these commands can lead to confusion about which files will be affected.
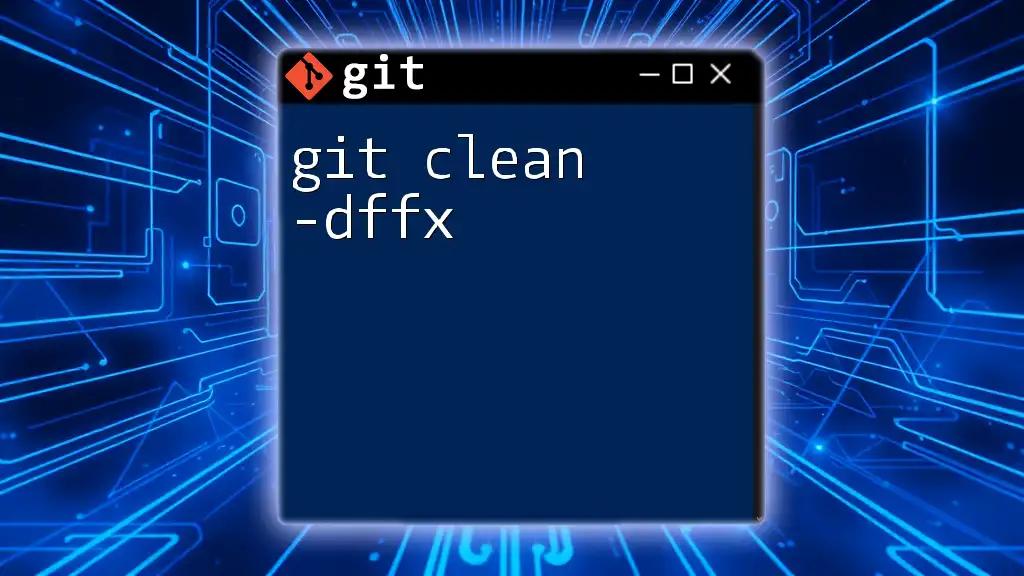
Additional Resources
Official Git Documentation
For more detailed information, refer to the [official Git documentation](https://git-scm.com/docs/git-clean). This resource offers an extensive explanation of all options and use cases for the command.
Tutorial Videos
If you are a visual learner, consider exploring various Git tutorial videos available on platforms like YouTube that delve deeper into usage examples.
Community Forums
Engaging with community forums such as Stack Overflow can provide answers and insights from other developers who have faced similar challenges with `git clean`.
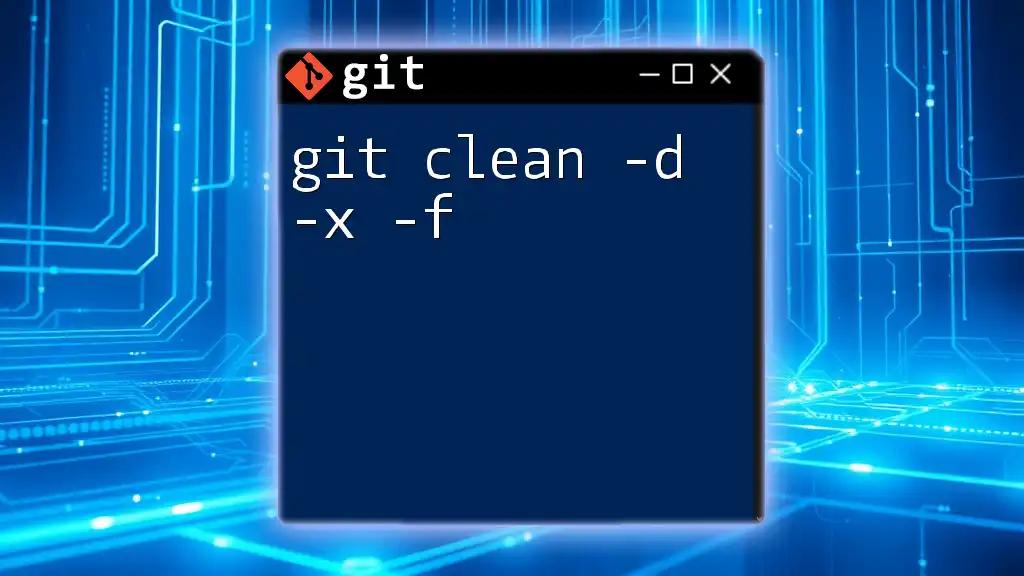
Conclusion
In summary, using `git clean -n` is a vital practice for developers looking to maintain a clean working environment. By simulating deletions, you can protect your important files while enjoying the benefits of an organized workspace. Regularly practicing this command not only enhances your Git skills but also ensures a smoother development process.
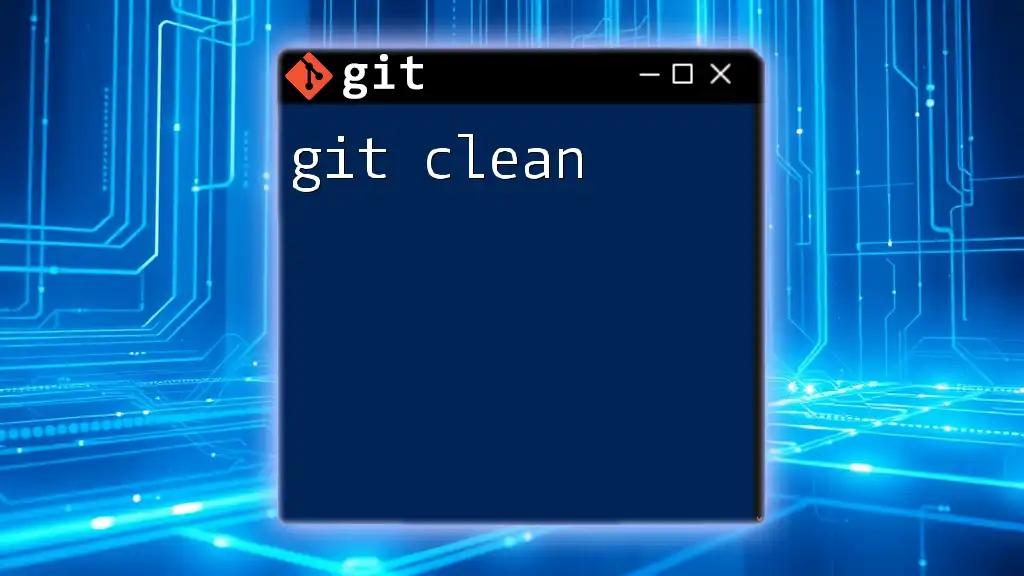
FAQs about `git clean`
What does `git clean -n` do?
`git clean -n` shows which untracked files would be deleted without actually removing them, allowing for a safe review before executing cleanup.
Can I recover files deleted by `git clean -f`?
Recovering deleted files is tricky. Properly understanding and using the `-n` flag first can help prevent unwanted deletions and loss.
Is there a way to view untracked files without using `git clean`?
Yes, you can use `git status` which will list all untracked files under the "Untracked files" section. This can provide insight into your workspace without cleaning any files.