The term "git build" is often a misunderstanding, as Git itself doesn't have a specific build command; instead, developers typically use Git in conjunction with build tools to compile or package their code.
Here's a code snippet to illustrate how to check the status of your Git repository before you build your project:
git status
What is Git Build?
Git build refers to the process of creating an executable format of your application from your source code using Git as part of your version control management. As developers collaborate on different projects, they often need to compile the latest version of a codebase to ensure all new changes are integrated properly. Understanding the intricacies of Git builds is crucial for maintaining a seamless development workflow.
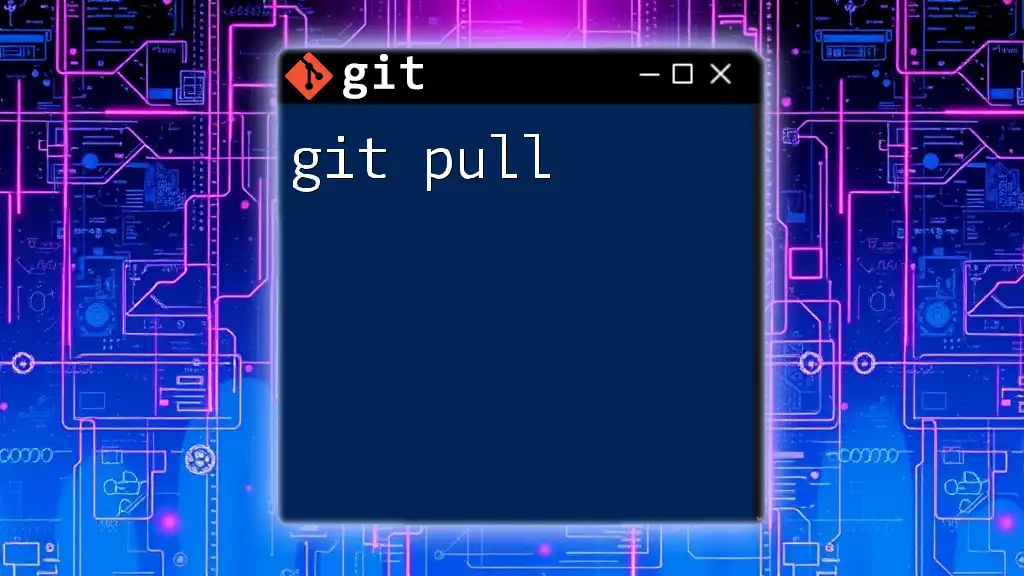
Understanding the Basics of Git
What is Git?
Git is a distributed version control system that enables multiple developers to work on the same project simultaneously without overwriting each other’s changes. By maintaining a full copy of the repository on each user’s machine, Git facilitates efficient collaboration. Key concepts within Git include:
- Repositories: Where all the versions of the project are stored.
- Commits: Snapshots of the project at a certain point in time.
- Branches: Independent lines of development within a repository.
- Clones: Full copies of repositories made by users.
Core Git Commands Related to Building
Key commands used in the context of preparing for a build include:
- `git clone`: This command creates a local copy of a remote repository on your machine, making it the first step in any build process.
- `git pull`: Updates the local repository with the latest changes from its remote counterpart.
- `git checkout` vs. `git switch`: While both commands are designed for changing branches, `git switch` is the more modern and user-friendly alternative.
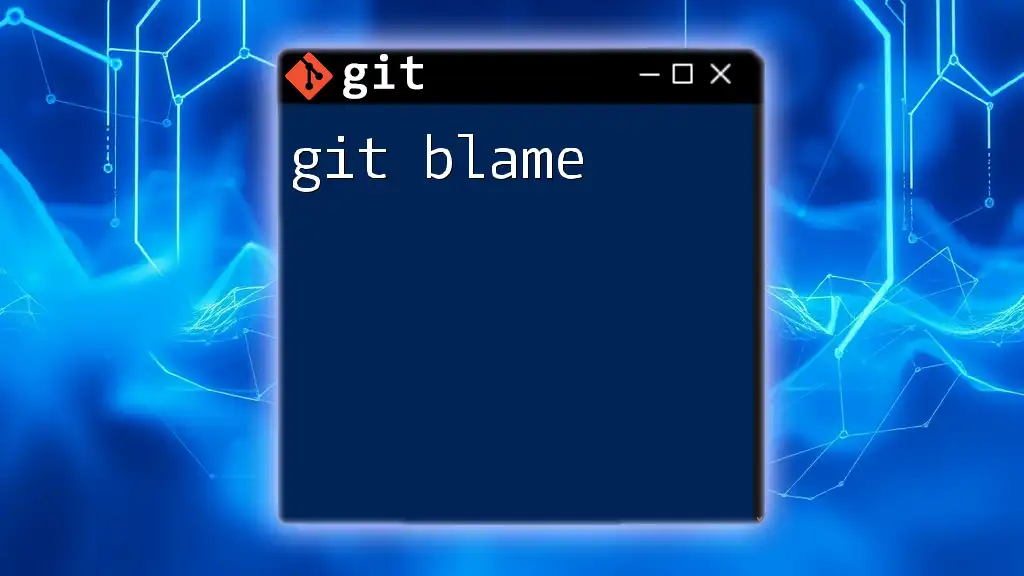
What Does “Build” Mean in the Context of Git?
Defining the Build Process
In software development, the build process is a sequence of steps that generates a compiled version of your application from source code. This includes compiling language files, linking libraries, and packaging files. Understanding this process is essential for ensuring your code integrates effectively with the rest of your team’s contributions.
Common Build Tools Used with Git
Several build tools work seamlessly with Git:
-
Make: A widely used tool for managing builds in a Unix environment. It provides a way to define builds through a Makefile, which includes target definitions and dependencies.
-
Maven: Popular in Java ecosystems, Maven automates the process of building, packaging, and managing dependencies for Java applications.
-
Gradle: A flexible build automation tool that supports multiple programming languages and is especially favored for Android applications due to its speed.
Each of these tools has its strengths and weaknesses, and the choice often depends on the specific requirements of your development environment.
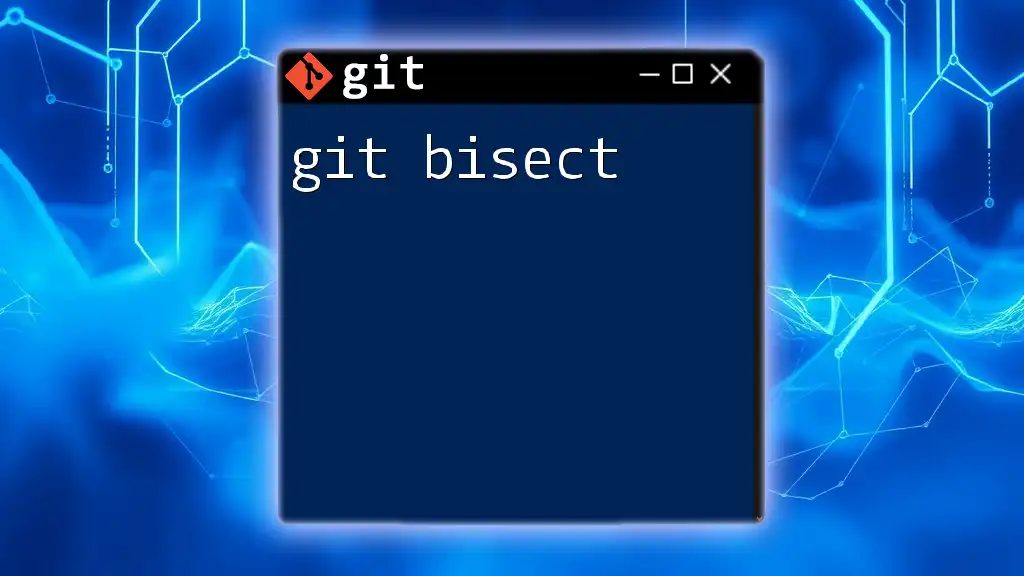
Setting Up Your Git Environment for Building
Installing Git
To use Git effectively, you first need to install it. You can find instructions tailored to your operating system at [Git's official website](https://git-scm.com).
Configuring Git
After installation, it’s vital to configure Git for your development environment. Here are some essential configurations to set:
-
User Information: Command to set up your username and email, which Git uses to identify authorship of commits.
git config --global user.name "Your Name" git config --global user.email "youremail@example.com"
This simple step can save you time and confusion down the line when you're reviewing commit histories.
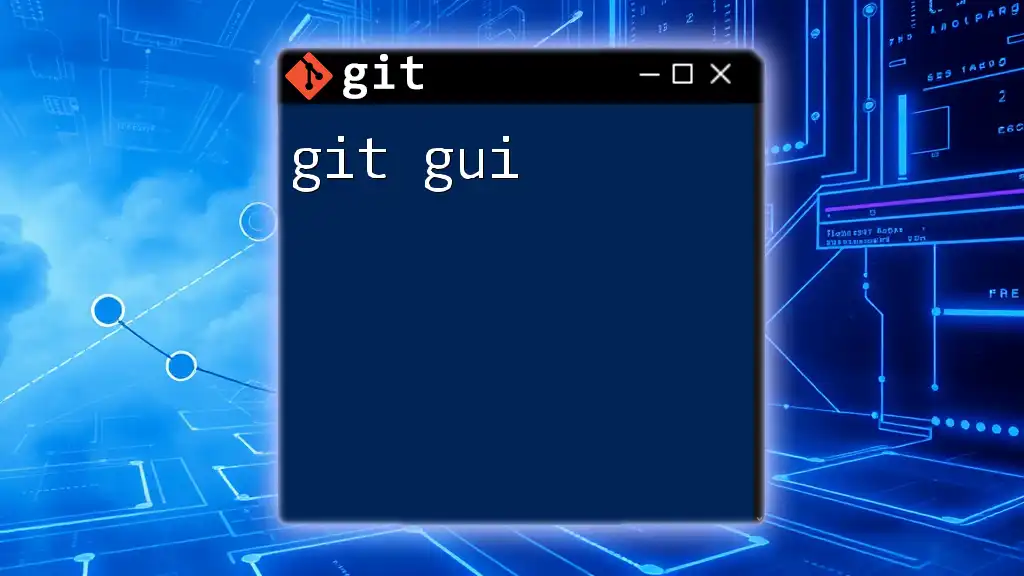
Basic Git Commands to Prepare for a Build
Cloning a Repository
To start working with a codebase, your first step is to clone the repository:
git clone https://github.com/username/repo.git
This command creates a complete local copy of the repository, allowing you to start making changes immediately.
Checking Out Branches
When collaborating on a project, you may need to switch between different branches. Use `git switch` for a smoother experience:
git switch feature-branch
This command allows you to seamlessly switch to a branch where you’ll perform your builds.
Pulling Updates and Handling Merge Conflicts
Regularly pulling updates ensures that you have the latest changes from your collaborators. If Git detects conflicts during a pull, you’ll need to resolve them manually before proceeding with your build. Here’s a typical flow for resolving merge conflicts:
-
Use `git pull` to update your local branch.
-
Identify the conflict in files and edit them accordingly.
-
After resolving the conflicts, run:
git add resolved-file.txt git commit -m "Resolved merge conflict"
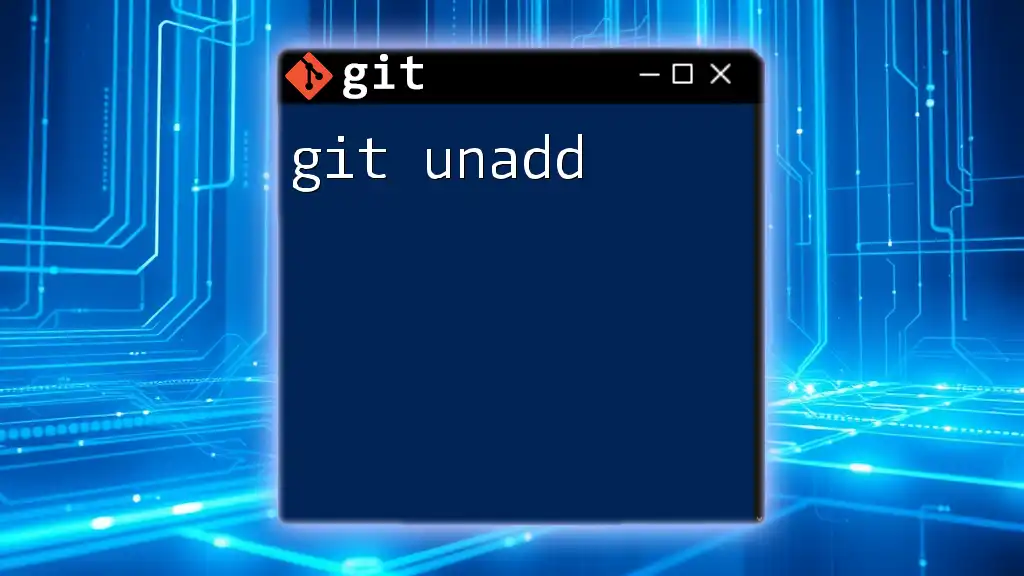
The Build Process: Step-by-step
Creating Build Scripts
Build automation relies heavily on scripts that define how to compile and package your application. Here’s a basic Bash script example:
#!/bin/bash
echo "Building the project..."
make
echo "Build completed!"
This script will execute a build process via Make, outputting each step of the process for clear visibility.
Executing the Build
To execute your build script, run it in your terminal. If your script is named `build.sh`, you would first give it execute permission and then run it:
chmod +x build.sh
./build.sh
For large projects, incorporating hooks to trigger builds on specific events (like commits or merges) is invaluable.
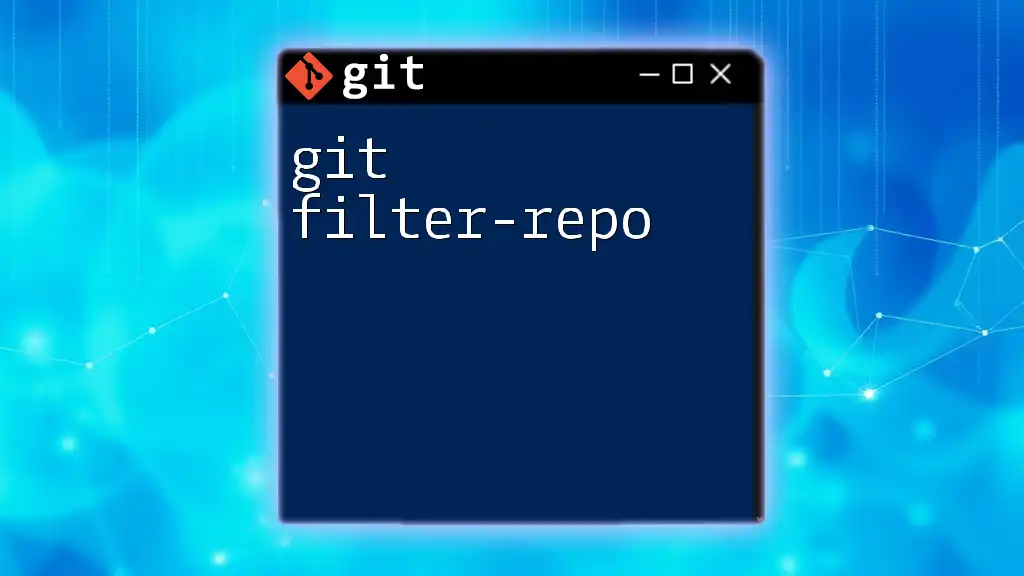
Continuous Integration and Git Build
Introduction to Continuous Integration (CI)
Continuous Integration (CI) is a modern software development practice that encourages developers to integrate their changes frequently into a central repository. This practice allows for instant feedback on the latest changes, which is crucial for quality assurance.
Setting Up CI Tools
Popular CI tools that work well with Git include:
-
Jenkins: Known for its flexibility and customizability but requires more manual setup.
-
Travis CI: Easy to configure with GitHub, ideal for open-source projects.
-
GitHub Actions: A powerful built-in CI/CD feature of GitHub that allows you to create workflows directly in your repository.
For example, a simple GitHub Actions configuration for a Node.js project can look like this:
name: Node.js CI
on:
push:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js 14
uses: actions/setup-node@v2
with:
node-version: '14'
- run: npm install
- run: npm test
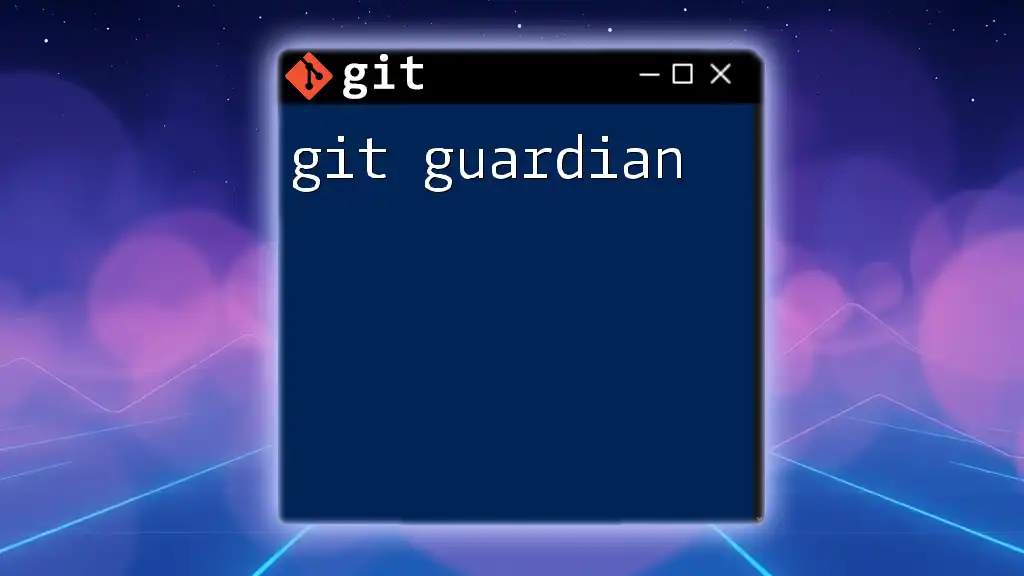
Best Practices for Managing Builds in Git
Maintaining Build Artifacts
Build artifacts are the files produced after a build process. Managing these effectively can reduce redundancy and improve performance. Consider using tools like Artifactory or Nexus to maintain a central repository of your artifacts.
Versioning Your Builds
Employing semantic versioning for your builds helps communicate the nature and significance of changes. Use the following command to tag your commits, providing a clear reference for future builds:
git tag v1.0
This will help you keep track of stable versions of your software as your project evolves.
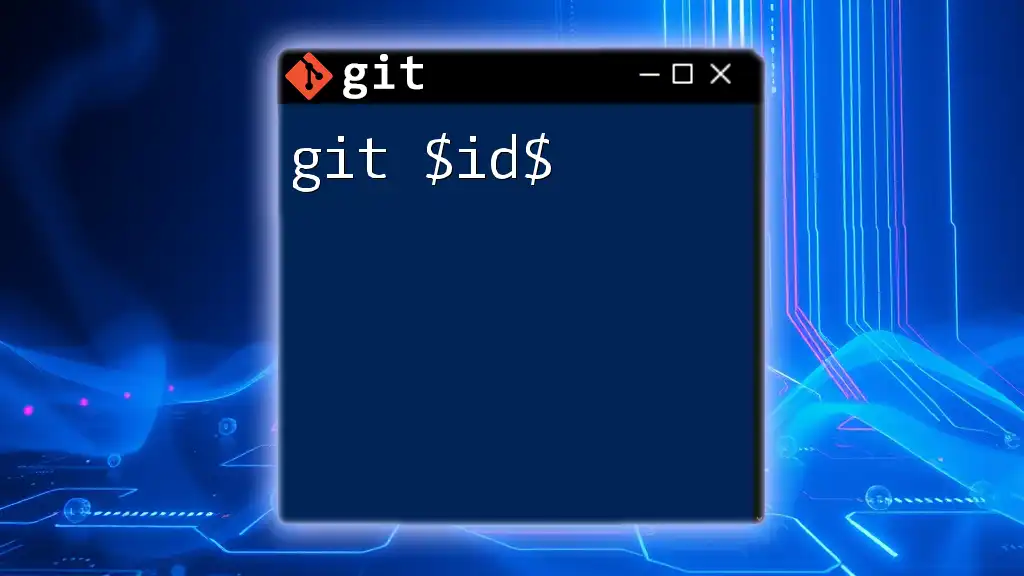
Troubleshooting Common Issues
Debugging Build Failures
When a build fails, first check the output message. Look for common indicators such as missing files or unresolved dependencies. Referring to documentation for the build tools you are using can often help in quickly identifying the issue.
Reverting to Previous Builds
If you run into problems after a build and need to revert to a stable version, Git gives you robust tools for this purpose. Using `git revert` for specific commits or `git reset` for entirety can restore your working state effectively. Execute:
git revert <commit-hash>
This will create a new commit that undoes the changes made by the specified commit but retains project history.
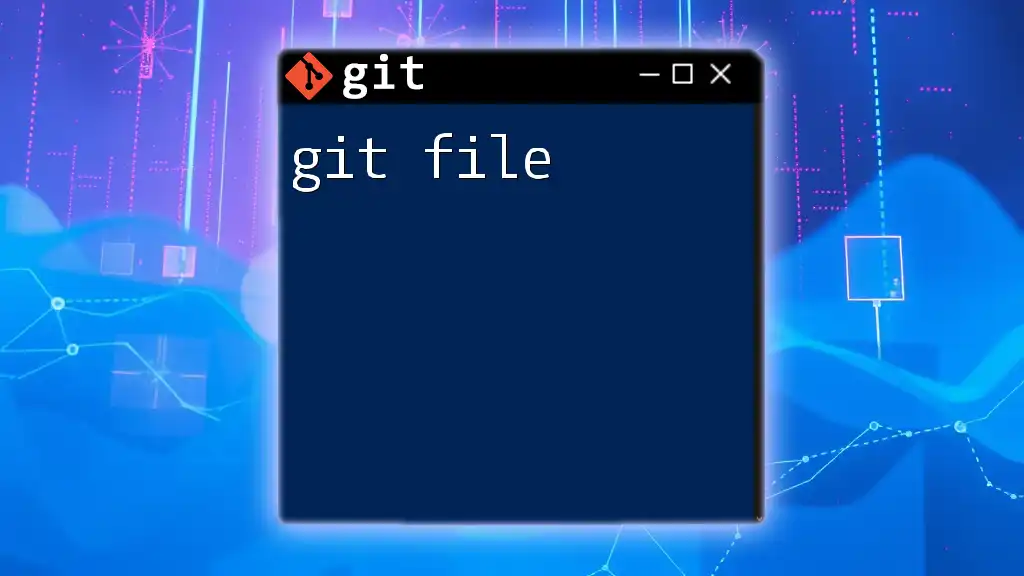
Conclusion
Mastering git build processes can dramatically enhance your software development lifecycle. Not only does it streamline collaboration among team members, but it also solidifies the integrity and reliability of your codebase. I encourage you to explore the details of your Git build processes, experiment with the UI of your chosen build tools, and strive to automate more of your workflows over time.
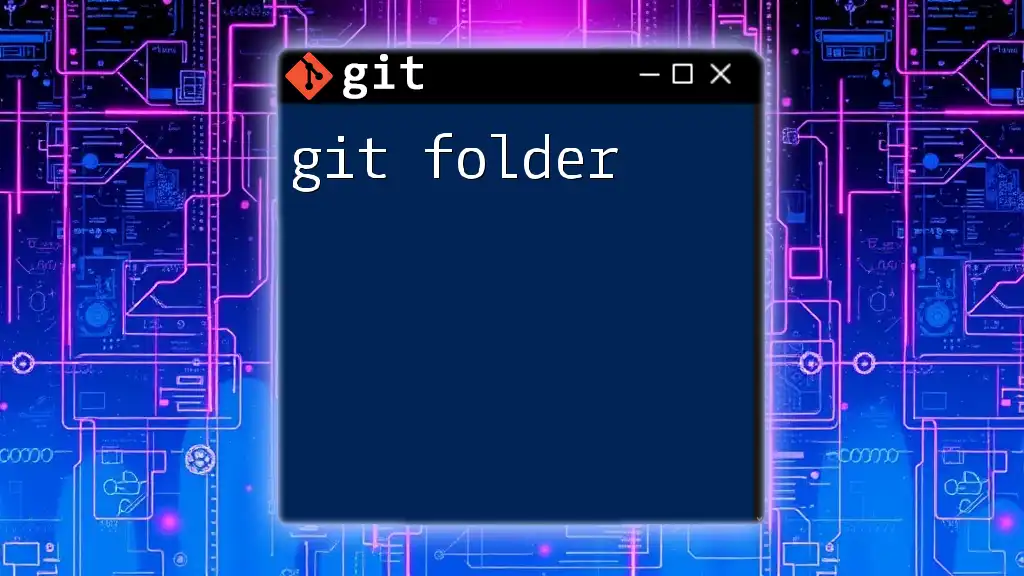
Call to Action
Now that you have a stronger understanding of Git builds, I invite you to experiment with setting up your own Git build process. Start small, explore the capabilities of your favorite tools, and don’t hesitate to share your experiences with your peers!