The `git add -a` command stages all changes (including modifications, deletions, and new files) in the working directory for the next commit.
git add -a
What is `git add`?
`git add` is a fundamental command in Git that plays a crucial role in the version control workflow. It is used to stage changes in your working directory, preparing them to be committed to the repository's history. When you modify, add, or delete files in your project, you need to inform Git which changes you want to be included in the next commit. This is where `git add` comes into play.
Unlike other commands in Git that manipulate commits or branches, `git add` is responsible for updating the staging area with the changes you want to track. You can use it in several ways, including specifying individual files, directories, or even using wildcards to match file patterns.
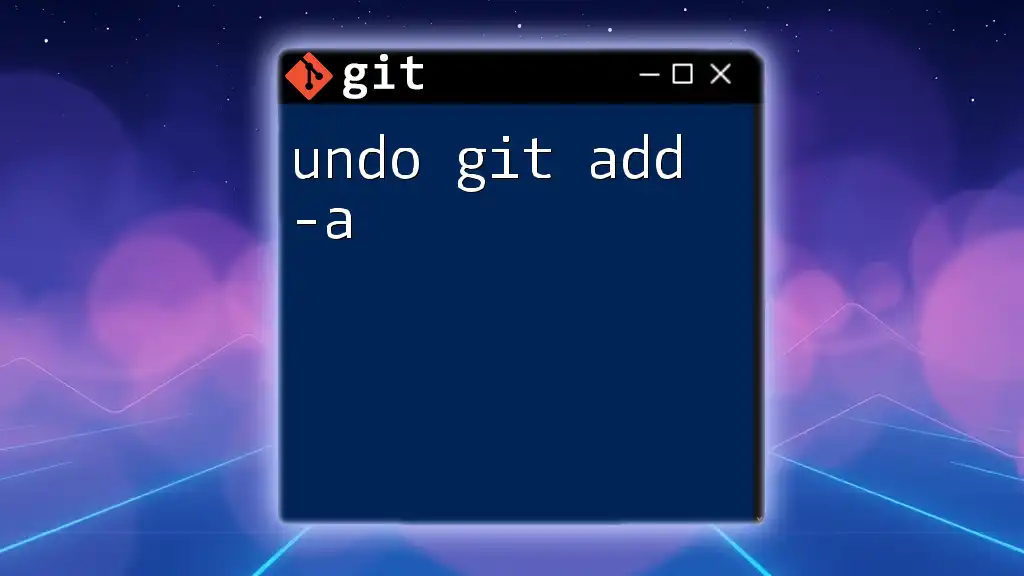
Understanding the `-a` Option
The `-a` flag stands for "all." When you use `git add -a`, you are instructing Git to stage all tracked changes in your working directory. This includes modifications to previously added files, deletions of files, and even new files that have been created. The `-a` option is particularly useful when you want to ensure you haven’t overlooked any changes.
What Does `git add -a` Include?
Using `git add -a` stages:
- Modified Files: Any changes made to files that were previously tracked.
- Deleted Files: Any files that have been removed from the working directory will also be staged for deletion.
- Newly Created Files: All new files that are not ignored will be staged.
This convenience allows developers to quickly stage multiple changes without the need to specify each one explicitly.
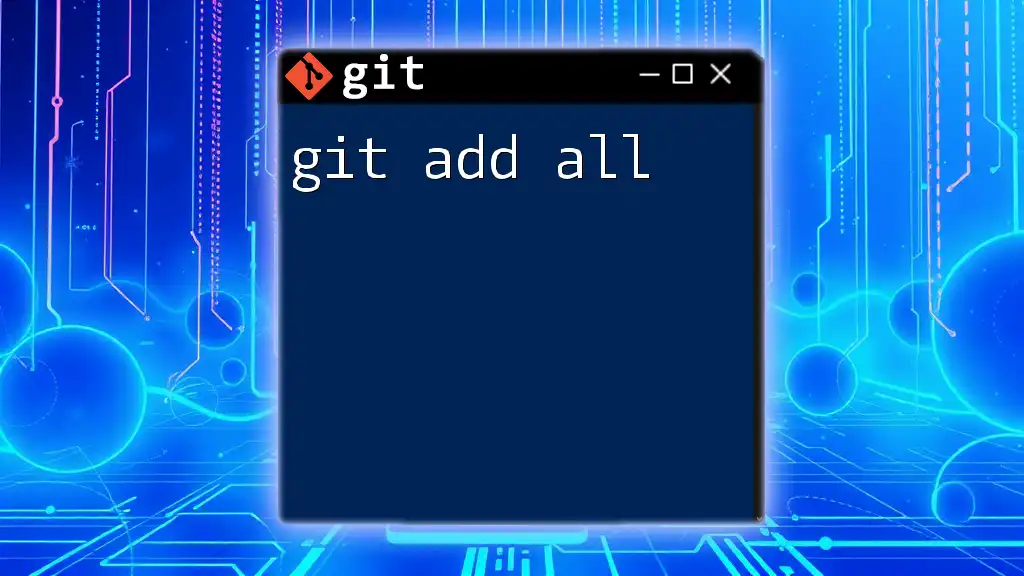
How to Use `git add -a`
The syntax for the command is straightforward:
git add -a
Use Cases for `git add -a`
-
Cleaning Up the Staging Area: If you've made several changes across multiple files and want to quickly stage all of them, `git add -a` ensures you're ready to commit without investigating each file individually.
-
Preparing for Commits: When you're ready to commit a comprehensive set of changes in your project, using this command will streamline your process, allowing you to focus more on crafting your commit message.
Step-by-Step Guide
To effectively use `git add -a`, follow these steps:
Preparing Your Working Directory
First, create a new Git repository and make some changes. For instance:
mkdir my_git_project
cd my_git_project
git init
touch file1.txt file2.txt
echo "Initial content" > file1.txt
echo "Initial content" > file2.txt
After modifying file1.txt, e.g., by adding more content, and deleting file2.txt, you're ready to prepare for staging.
Executing `git add -a`
Now run:
git add -a
You can verify which files are staged by checking the status:
git status
This command provides feedback on what changes have been staged for the next commit, allowing you to proceed with confidence.
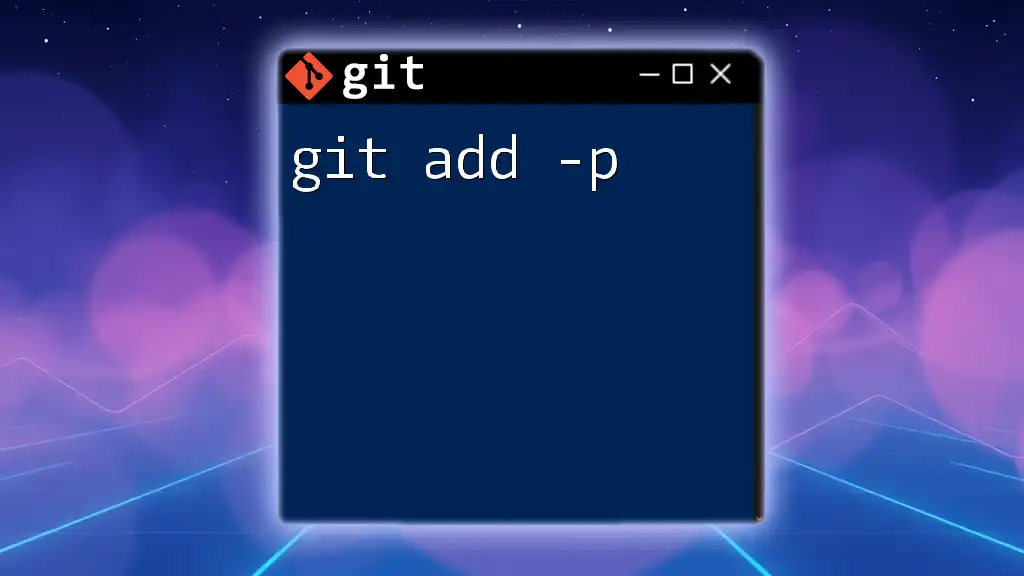
Practical Examples of Using `git add -a`
Example 1: Staging Various Types of Changes
Imagine a scenario where you've created a new file, modified an existing one, and deleted another. Here's how you can stage all these changes efficiently.
touch file1.txt
echo "Hello World" > file1.txt
rm file2.txt
git add -a
git status
By executing these commands, file1.txt will be staged with its new content, while file2.txt will be marked for deletion. Using `git status` will show you all the staged changes, confirming your successful operation.
Example 2: Handling Untracked Files
When you create a new file that hasn't been tracked by Git yet, `git add -a` can include it in the staging area as well:
touch file3.txt
git add -a
git status
Here, file3.txt will be staged even though it was untracked prior to the command.
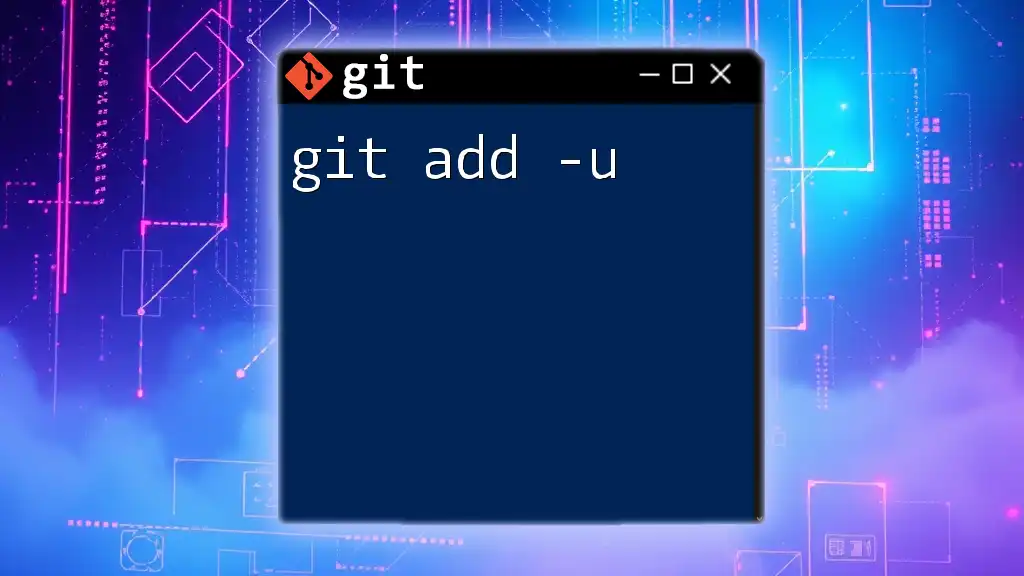
Common Mistakes to Avoid
Using `git add -a` can lead to some common pitfalls:
-
Adding Files That Shouldn’t Be Included: Always double-check your changes through `git status` before committing to ensure you're only staging intended modifications.
-
Confusing `-a` with Other Flags: It's easy to mix up options like `-A` and `-u`. Remember that `-A` stages all files, including new and deleted ones, while `-u` only stages changes to tracked files.
-
Forgetting to Check `git status`: Avoiding this check can lead to surprises in your commit history. It’s a best practice to always verify the staged files.
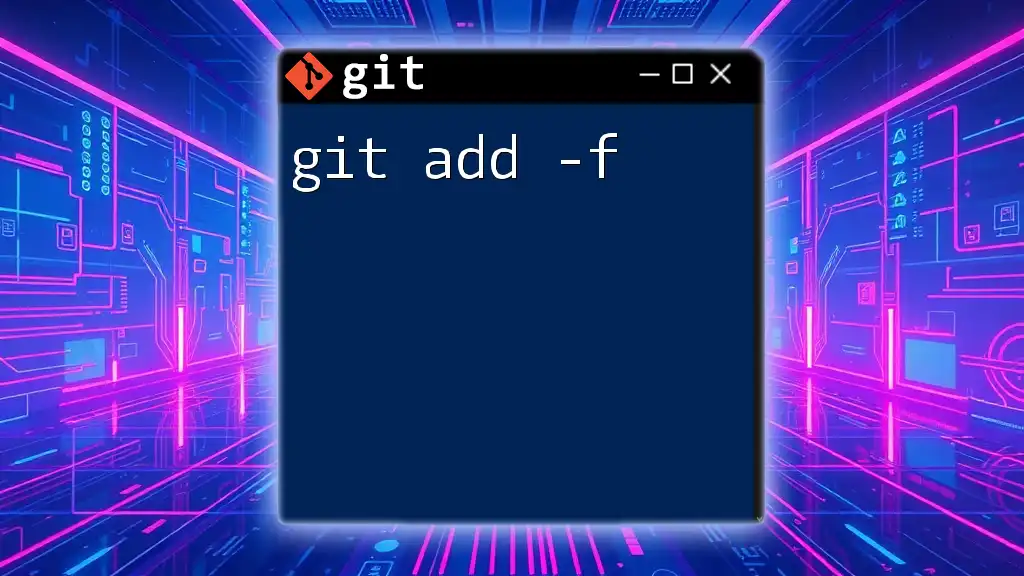
Best Practices for Using `git add -a`
To streamline your Git workflow, consider the following best practices:
-
Regularly Stage Changes: By consistently using `git add -a`, you maintain a clean commit history and ensure all relevant changes are included in your commits.
-
Utilize `.gitignore`: To prevent sensitive or unnecessary files from being staged, maintain a proper `.gitignore` file, allowing `git add -a` to function more effectively.
-
Commit Frequently: Regular commits with clear messages lead to better project management and easier collaboration.
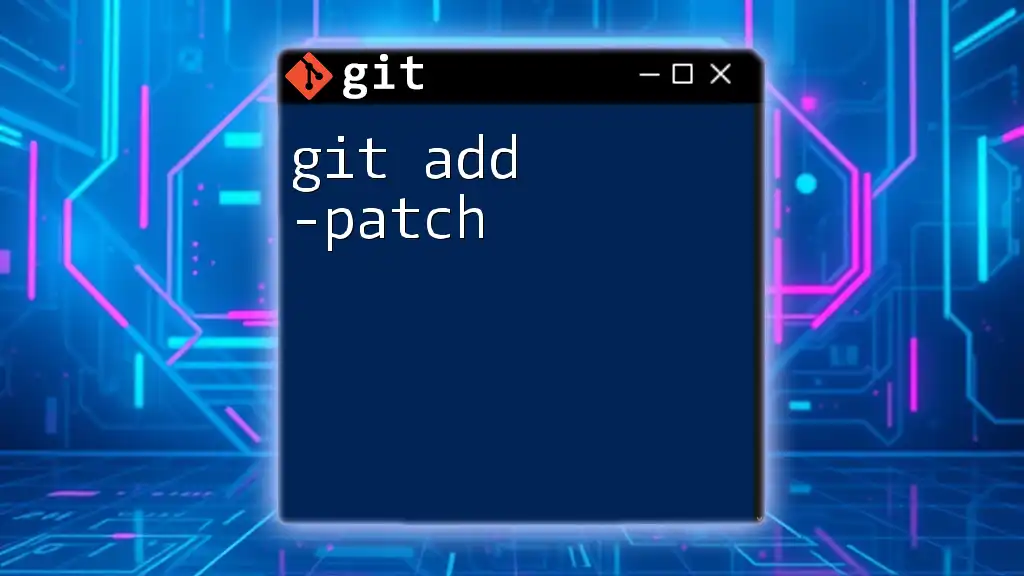
Conclusion
Understanding how to use `git add -a` effectively is a cornerstone skill for any developer working with Git. By using this command, you ensure a seamless transition between your working directory and the repository, capturing all your changes in one go. Regularly practicing with the command will enhance your confidence in using Git, allowing you to focus on more complex version control tasks.
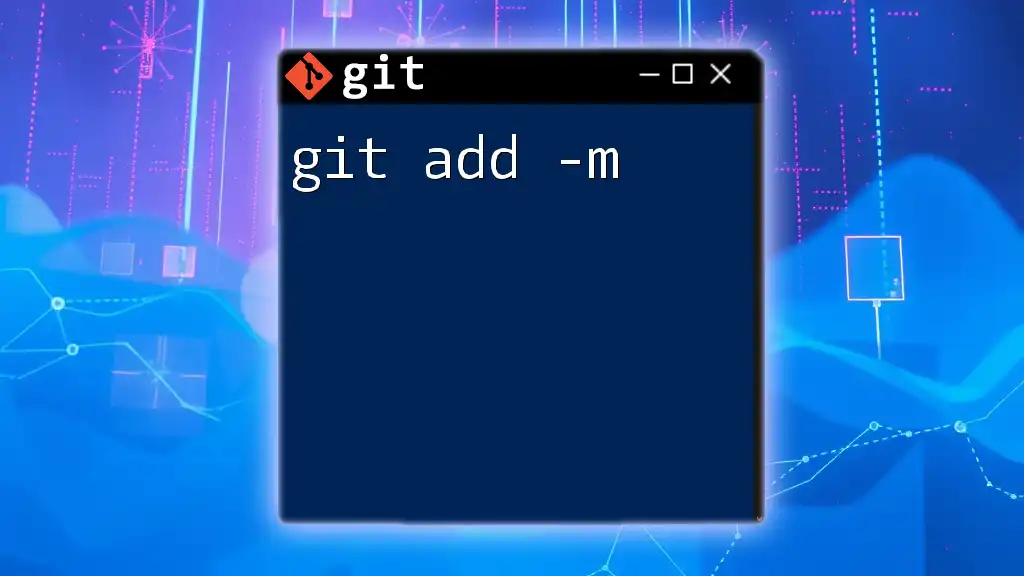
Additional Resources
For further learning, refer to the official Git documentation and other reputable resources dedicated to mastering Git commands and the best practices surrounding them.
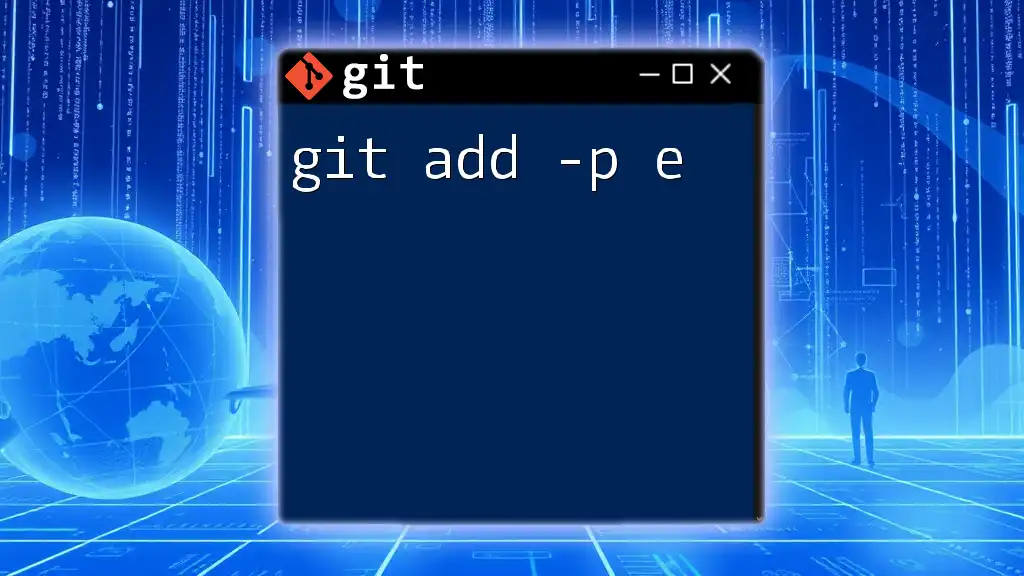
Frequently Asked Questions (FAQs)
What is the difference between `git add .` and `git add -a`?
While both commands can stage changes, `git add .` stages all changes in the current directory and its subdirectories, whereas `git add -a` stages all changes to tracked files, including removals, across the entire working directory.
Can `git add -a` stage ignored files?
No, `git add -a` respects your `.gitignore` settings and will not include files that are listed there.
Why is my file not being staged with `git add -a`?
This could occur if the file is in the `.gitignore` list, if your changes are not yet saved, or if the file is untracked and not modified or created during the current session. Always confirm with `git status`.
By mastering `git add -a`, you empower yourself with one of the essential tools in the Git arsenal, enabling a more efficient and organized coding experience.