The `git add .` command stages all modified and new files in the current directory for the next commit in Git.
git add .
Understanding Git Basics
What is Version Control?
Version control is a system that records changes to files over time, enabling multiple users to collaborate on projects efficiently. This system allows developers to track changes, revert to previous states, and manage different versions of their work seamlessly. The primary benefits of using a version control system (VCS) include:
- Collaboration: Multiple users can work on the same project concurrently without overwriting each other’s changes.
- Backup and Restore: You can restore previous file versions if mistakes are made, offering a safety net for your work.
- Branching and Merging: You can create separate branches for new features or bug fixes, and merge them back together once completed, maintaining the integrity of the main project.
Introduction to Git
Git is a distributed version control system that was created to enhance the handling of projects with a focus on speed, data integrity, and support for distributed, non-linear workflows. Its prominent features include:
- Speed: Git is optimized for performance, allowing operations such as commit, diff, and log to be executed quickly.
- Data Integrity: Every change is recorded in the form of a commit, and Git ensures the integrity of these changes through cryptographic hashes.
- Flexibility: Developers can work on multiple features independently using branches and merge them when ready.
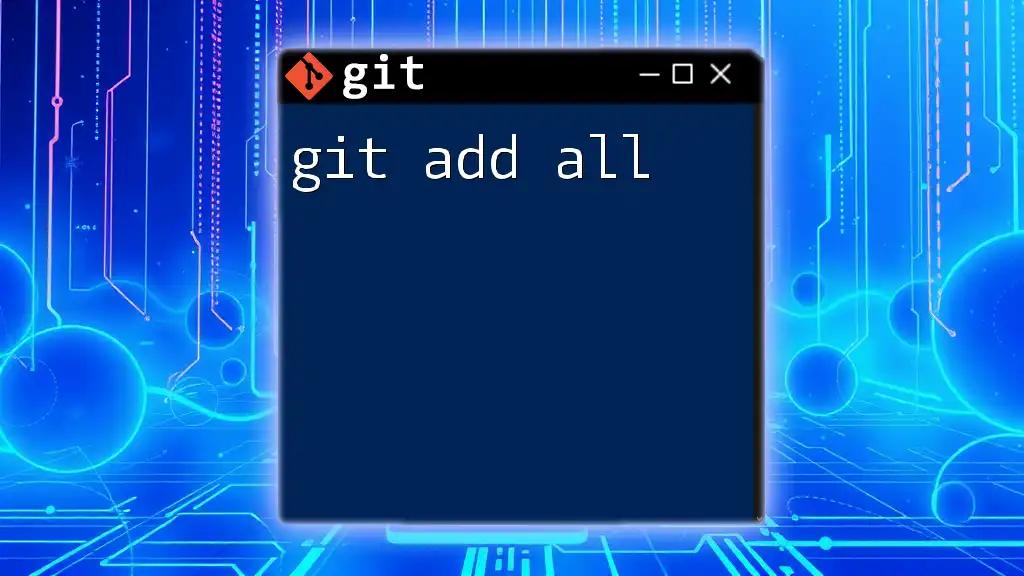
The Role of `git add`
What Does `git add` Do?
The command `git add` is essential for staging changes in your repository before committing them. It brings changes from your working directory into the staging area, informing Git about which changes will be included in the next commit. The staging area acts as a buffer between the working directory and the Git repository, allowing you to prepare and organize your changes precisely.
Why Use `git add .`?
The command `git add .` specifically stages all modified, new, and deleted files within the current directory and its subdirectories. This is particularly useful for a quick commit since it allows you to add multiple changes at once without having to specify each file individually. Understanding how `git add .` differs from other `git add` commands, such as `git add <file_name>`, is crucial for efficient version control.
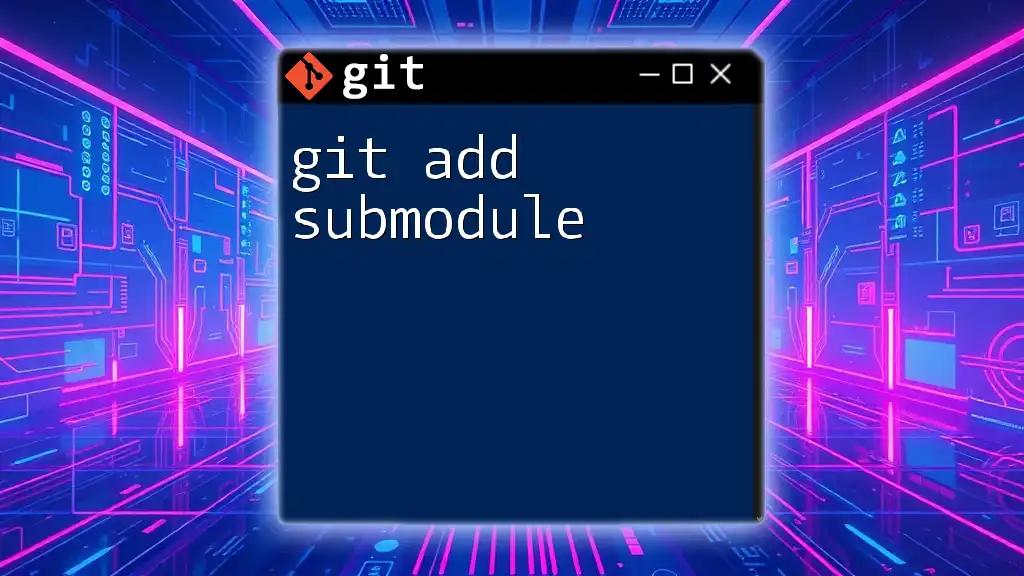
Syntax and Usage
Basic Syntax of `git add`
The general syntax structure of the `git add` command is as follows:
git add [options] [pathspec...]
This flexible syntax allows you to stage specific files, directories, or wildcard selections as needed.
Using `git add .`
When you execute the command:
git add .
all changes (including additions, modifications, and deletions) are staged for the next commit within the current directory and all child directories. This command is especially useful when you have made numerous changes and want to stage everything at once.
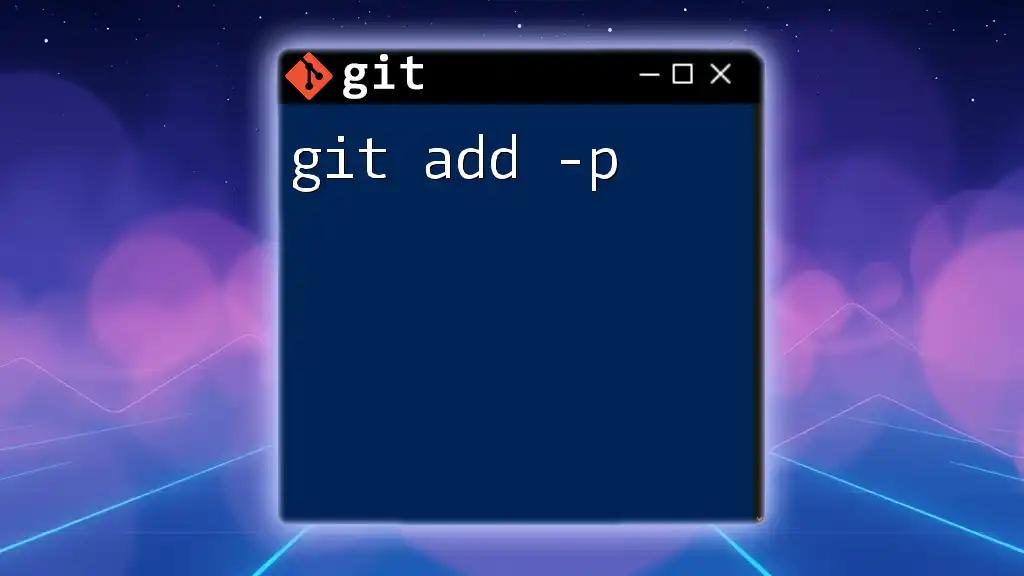
Practical Examples
Example 1: Adding New Files
Consider you have just created a new file in your project. For instance:
mkdir new_folder
cd new_folder
touch new_file.txt
git add .
In this scenario, the command `git add .` stages `new_file.txt` for your next commit. By adding everything at once, you streamline your workflow and reduce the chances of forgetting to stage essential files.
Example 2: Modifying Existing Files
If you modify an existing file in your repository and wish to reflect the changes:
echo "Some changes" >> existing_file.txt
git add .
Executing this command stages the changes made to `existing_file.txt`, ensuring that your next commit will include these updates.
Example 3: Removing Files
If you need to remove unwanted files and want Git to recognize these deletions, you can simply run:
rm unwanted_file.txt
git add .
In this case, `git add .` stages the removal of `unwanted_file.txt`, making sure that the deletion will be tracked during your next commit.
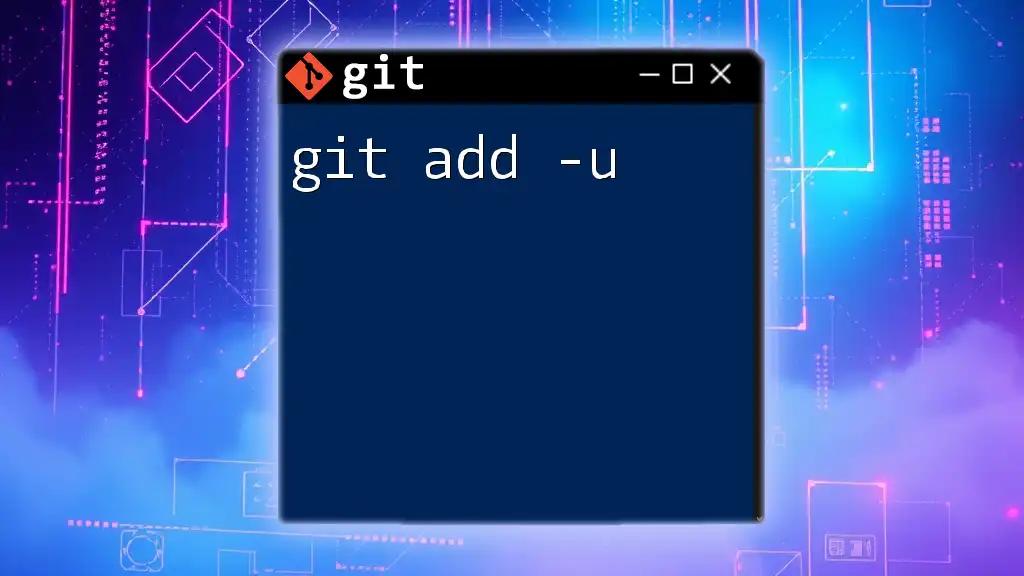
Common Mistakes to Avoid
Staging Unwanted Files
Using `git add .` can sometimes lead to unintentionally staging files that you didn’t mean to include. This happens when you add files that are under development or temporary files. To avoid this, be vigilant about the state of your working directory before running the command.
Difference Between `git add .` and `git add -A`
It's important to understand the subtle differences between `git add .` and `git add -A`. While both commands stage changes, `git add -A` stages changes across the entire repository, including deletions, modifications, and additions, regardless of your current directory. Using `git add .` confines its action to the current directory and its subdirectories. Therefore, use `git add -A` when you need to ensure all changes are staged across your project.
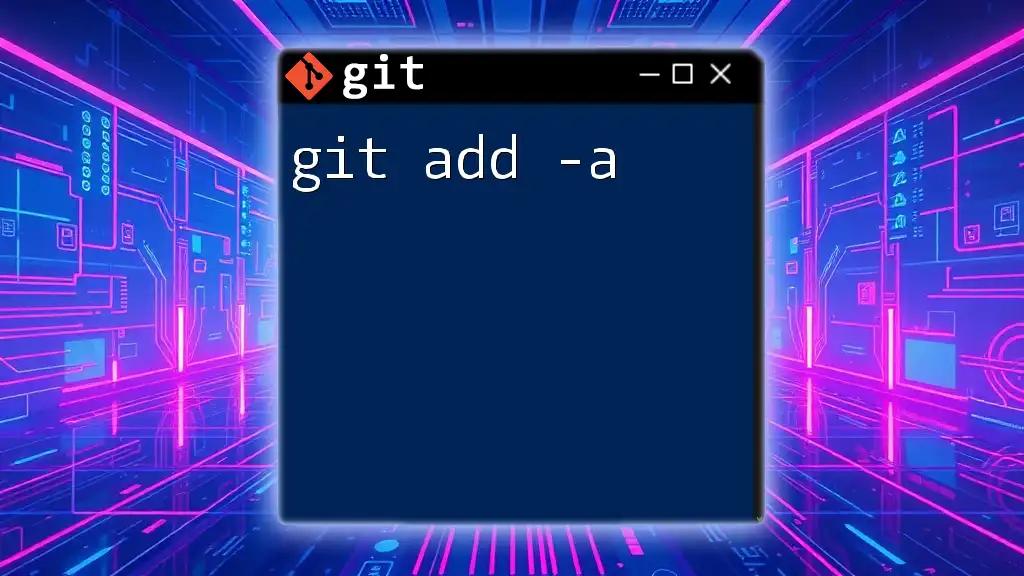
Tips for Efficient Usage
Use of .gitignore
To avoid accidentally staging files that should not be tracked, you can create a `.gitignore` file in your repository. This file allows you to specify patterns for files and directories that Git should ignore. For example:
# Example .gitignore content
*.log
node_modules/
This way, even if you run `git add .`, the specified files (like logs or `node_modules`) won’t be included in the staging area.
Regular Commit Practices
After using `git add .`, it’s crucial to commit your changes regularly. Adopt the habit of writing meaningful commit messages that describe the changes made. This practice will help you and your collaborators understand the project's history better.
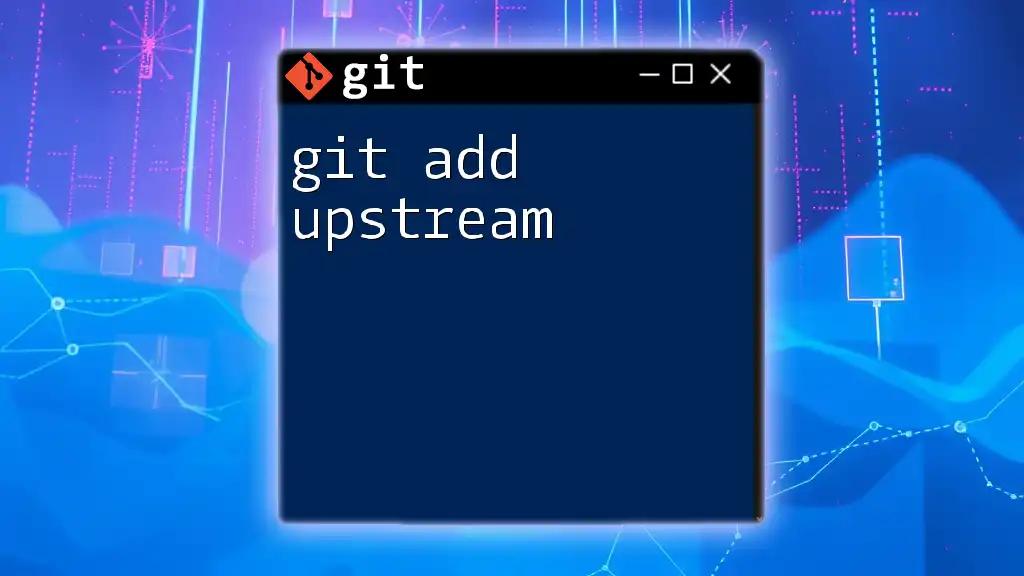
Summary
In this guide, we explored the command `git add .`, highlighting its significance in staging changes before committing. We discussed how the command functions, provided practical examples, and offered tips for avoiding common mistakes. Understanding `git add .` and its role will greatly enhance your development workflow.
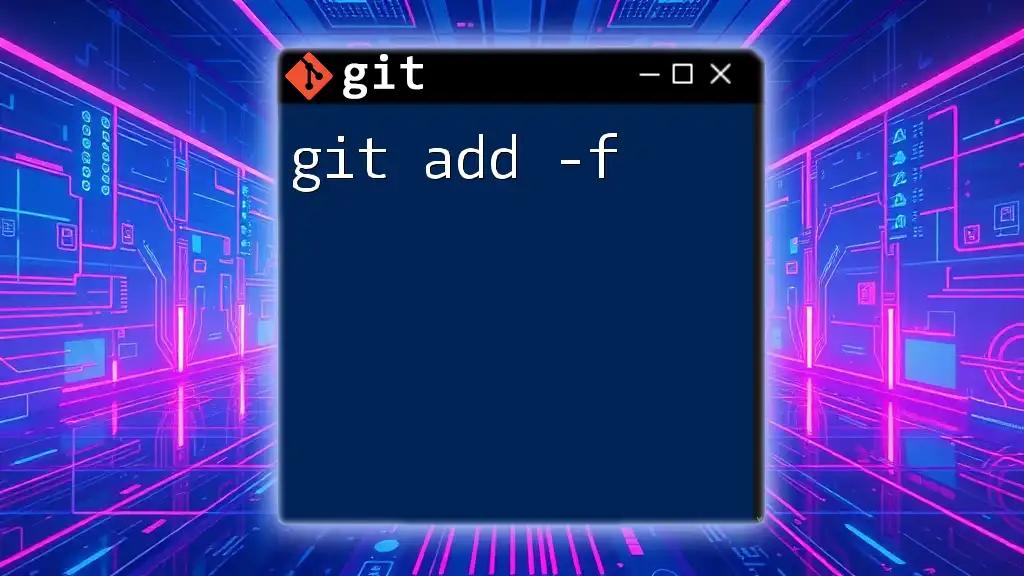
Additional Resources
To further your understanding of Git, you may refer to the official [Git documentation](https://git-scm.com/doc) and consider going through online tutorials to solidify your knowledge.
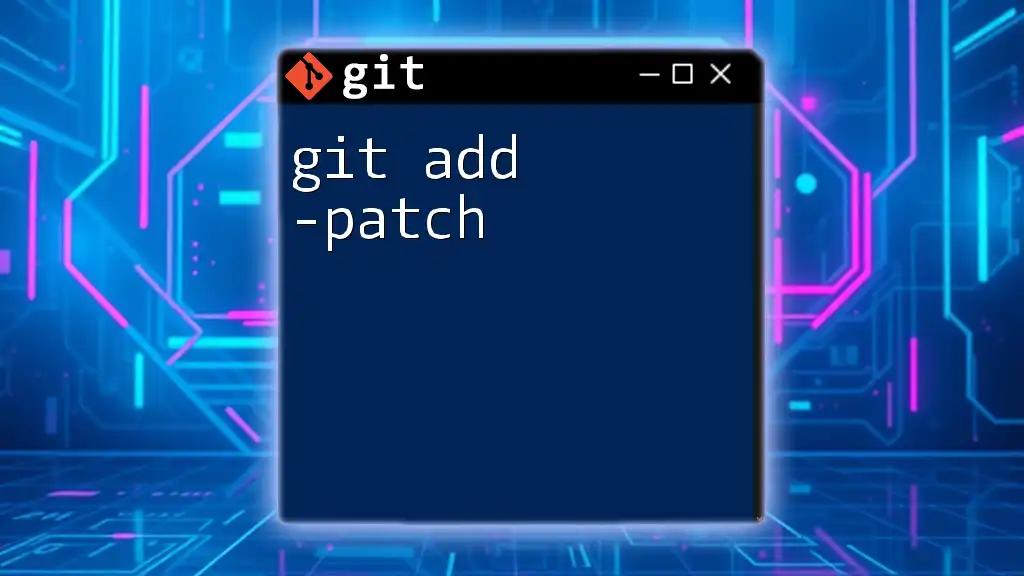
Conclusion
Mastering the command `git add .` is a fundamental step towards effective version control management. Embrace this powerful command in your daily development practices to streamline your workflow and collaborate more efficiently!