The command `git add -m` does not exist in Git; however, `git commit -m` is used to stage changes for commit along with a message describing the changes made.
Here's how you would typically use the `git commit -m` command:
git commit -m "Your commit message here"
Understanding `git add`
Git serves as a critical tool in the world of version control systems (VCS). It enables teams and developers to track changes, collaborate efficiently, and maintain the integrity of code at various stages of development. Within this ecosystem, the `git add` command plays an essential role by staging changes and preparing them for the commit phase.
What is `git add`?
The `git add` command is used to add changes in your working directory to the staging area. Staging is an important concept in Git, as it allows you to compile and review changes before they are officially recorded in the repository. When you stage a file, you are essentially letting Git know that you want to include this file in your next commit.
The Purpose of Staging
Understanding the Git workflow is crucial for effective version control:
- Working Directory: This is where you make changes to your files.
- Staging Area: Think of this as a preview of your commit. It lets you gather all the modifications you want to include in your next commit.
- Repository: This is where the finalized version of your project lives, after you’ve committed your changes.
By staging your changes, you have a chance to review what will be included in your next commit, allowing for better organization and clarity.
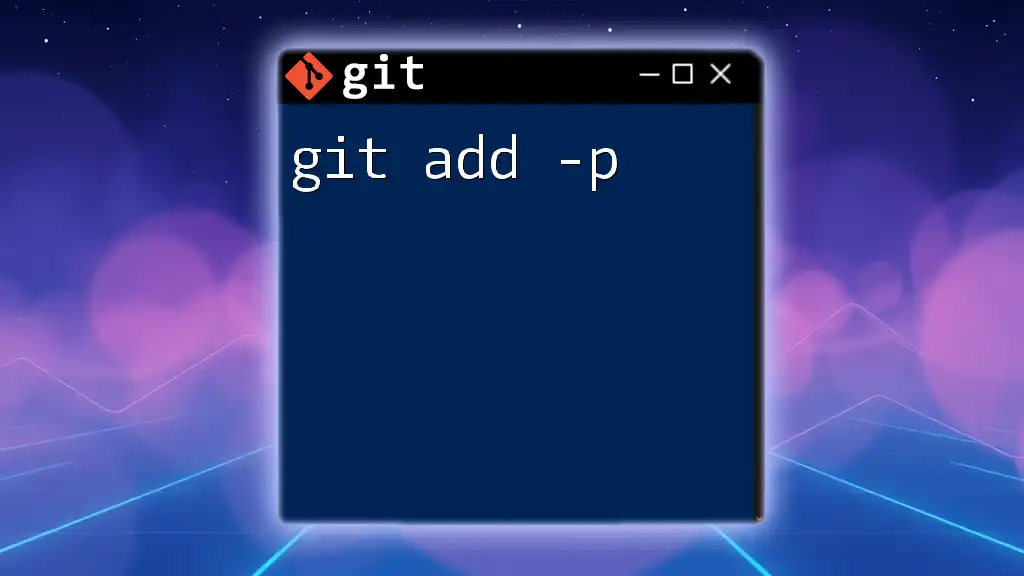
The `-m` Option
What Does `-m` Stand For?
The `-m` option stands for "message". This option is used with `git add` to provide a brief description of the changes you are staging. While you can certainly stage changes without a message, using `-m` helps maintain a clear history of your changes.
Why Use `-m`?
Utilizing the `-m` option can significantly enhance your development workflow. Here are some benefits:
- Clarity: By providing a clear, descriptive message, you can easily understand what changes were staged for a particular version.
- Documentation: A well-written commit message serves as a documentation tool, which can be useful during code reviews or when revisiting your code in the future.
Common Misconceptions About `-m`
Many newcomers to Git mistakenly believe that `-m` only relates to committing changes, but it can also play a role in defining the context of staged changes. However, it is crucial to recognize that the `git commit` command is the one most often associated with commit messages, and this should not be confused with `git add`.
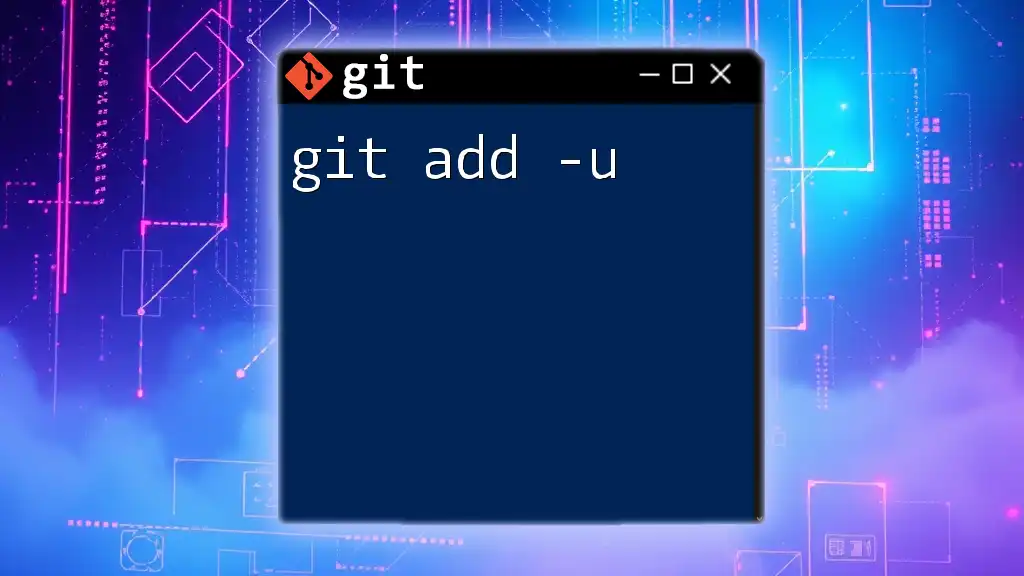
How to Use `git add -m`
Step-by-Step Guide
-
Set Up a Git Repository To start using Git, initiate a repository:
git init
-
Make Changes to Files Modify files as per your project requirements. For example, update a README file.
-
Using `git add -m` After making your changes, you can stage them with a message:
git add -m "Add feature X"
In this snippet, replace `"Add feature X"` with a concise description of the changes made.
Code Snippet Example
Using the `-m` option is straightforward. Here’s how to do it effectively:
git add -m "Update styling for the homepage"
This command stages your changes and adds a meaningful context, indicating what exactly was updated.
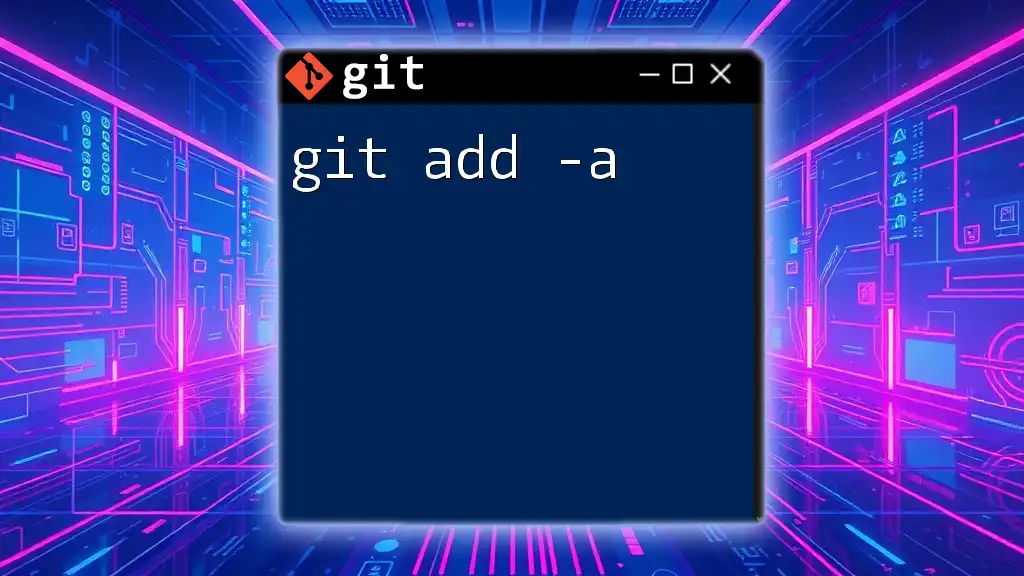
Best Practices for Using `git add -m`
Writing Effective Commit Messages
Writing clear and concise commit messages is vital for future reference. A good commit message should generally contain:
- A Brief Summary: The first line should summarize the change in less than 50 characters.
- A More Detailed Explanation (Optional): If needed, provide more context in a following paragraph.
Poor vs. Good Commit Messages
- Poor Message: "Fixed things" – This lacks detail and context.
- Good Message: "Fix spelling errors in documentation" – This is specific and informative.
When Not to Use `-m`
There are instances when using `-m` may not be appropriate:
- If you find your message is too long or detailed, consider using `git commit` separately for more extensive documentation and context.
- For larger changes that require multiple commits, you might want to stage files separately and provide distinct messages for each.
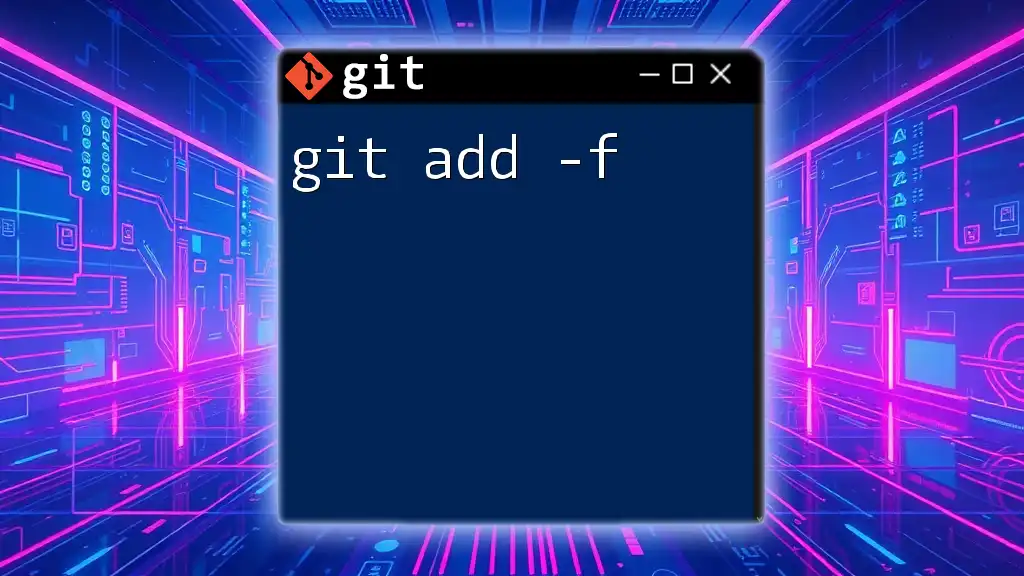
Troubleshooting Common Issues
Problems with Staging
Many beginners encounter issues when staging changes. Common problems can include forgetting to save files or mistakenly adding unwanted files. To troubleshoot:
- Always use `git status` to check what files are staged.
- Make sure all changes are saved in your editor before staging.
Conflicts when Using `-m`
Conflicts can occur if intervening changes happen. If you try to stage files while code conflicts exist, Git may prevent you from doing so. To resolve these:
- Make sure to resolve conflicts in your code editor before attempting to use `git add -m`.
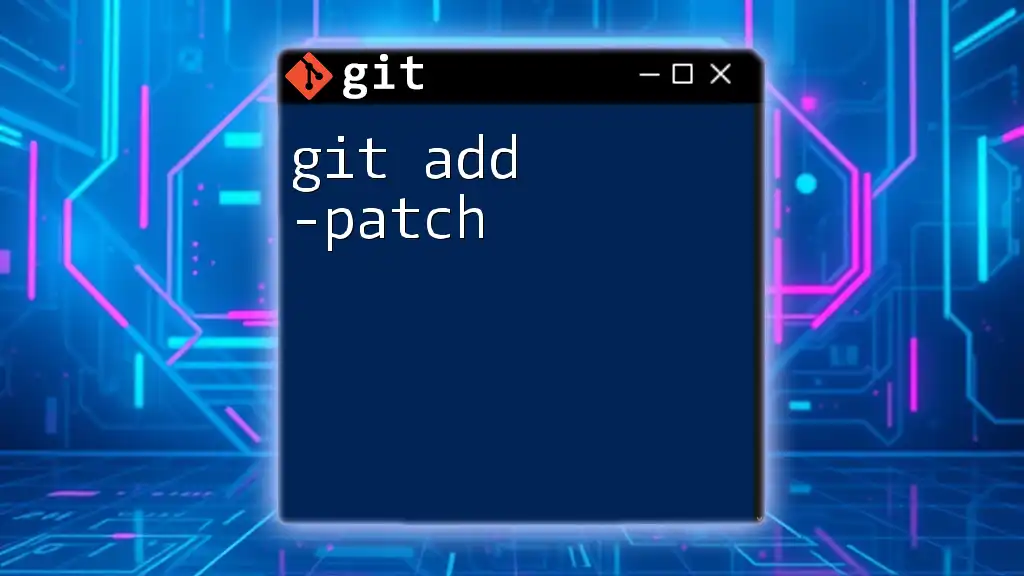
Conclusion
Understanding the `git add -m` command provides a way to efficiently stage your changes while maintaining clarity in your commit history. By following best practices for writing messages and recognizing when to use or omit the `-m` option, you can elevate your Git workflow. Practice regularly to master not just the command itself but also the foundational principles of version control.
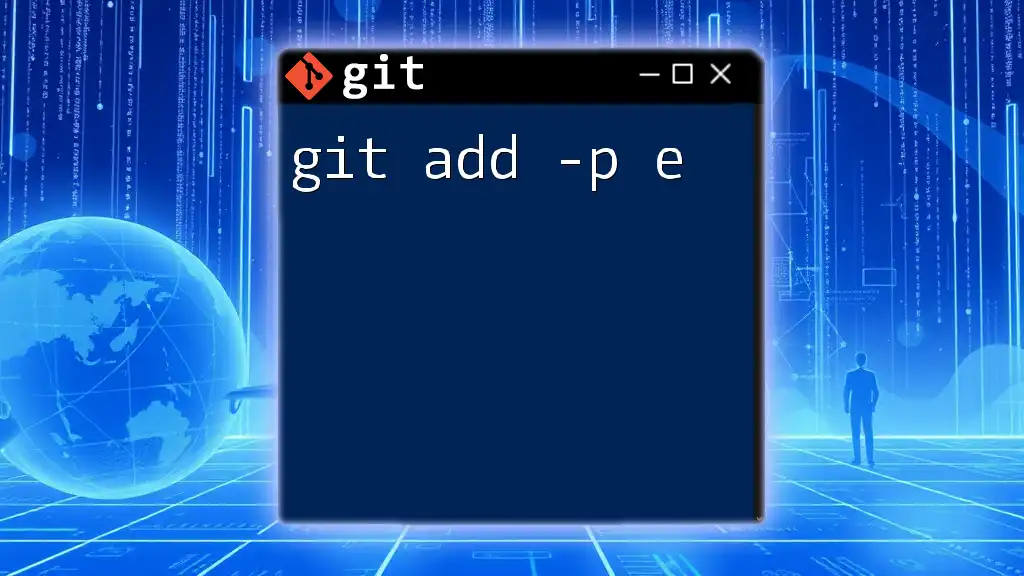
FAQs
Can I use `git add -m` with other flags?
Yes! The `-m` option can be combined with other flags for customized functionality.
Is `-m` only for commits, or can it be used in other commands?
The `-m` option is primarily associated with commit messages in Git commands. It doesn’t apply to commands outside of `git commit`.
How to view staged changes before committing?
Before committing, always check what has been staged with:
git status
This command helps you confirm whether the correct changes are staged.
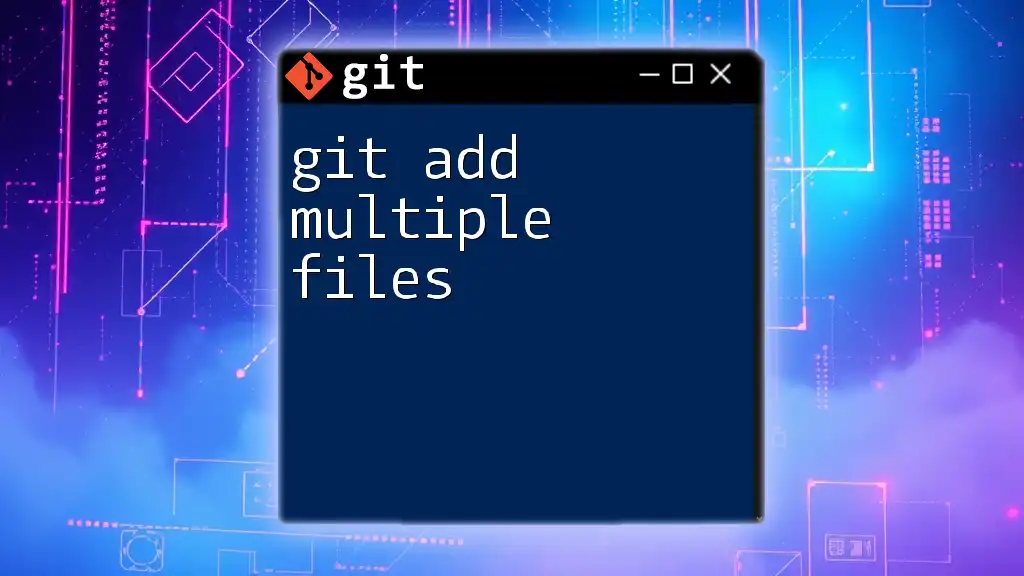
Additional Resources
For more advanced learning, consider referring to the official Git documentation or diving into community forums to engage with other Git users.