To edit files in an older version of Python while using Git, you can check out a specific commit where the old Python version was used, and then make your desired changes. Here's how you can do that:
git checkout <commit_hash> -- <filename>
Replace `<commit_hash>` with the hash of the commit you want to revert to and `<filename>` with the name of the file you wish to edit.
Understanding Git and Python Versioning
What is Git?
Git is a powerful version control system that allows developers to track changes in their codebase over time. Its ability to manage multiple versions of code simultaneously makes it an essential tool for collaborative programming projects. With Git, you can easily roll back to previous versions of your project, merge changes made by different contributors, and keep a detailed history of all modifications.
Python Versioning Basics
Python follows a structured versioning system, which includes significant updates like Python 2.x and 3.x. Each version introduces unique features and capabilities, and compatibility between versions can become a critical issue. Understanding the differences between versions is crucial when you need to edit code in an older Python version. For instance, the print statement in Python 2 is simply `print "Hello, World!"`, whereas in Python 3, it has become a function, requiring parentheses like so: `print("Hello, World!")`.
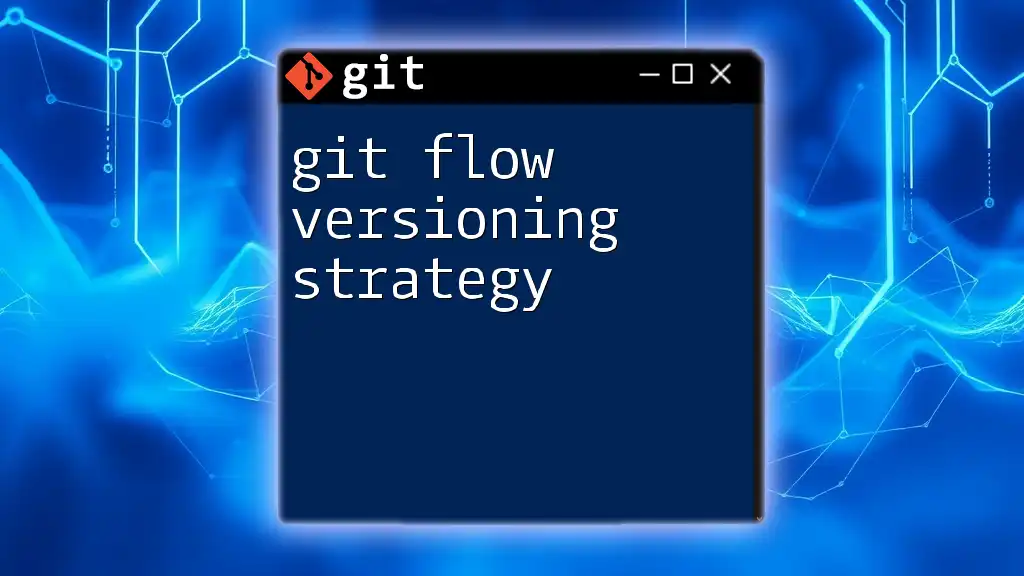
Setting Up Your Git Repository
Initializing a Git Repository
To begin working with Git, you first need to initialize a repository. This can be done using the command:
git init
This command creates a new Git repository in your current directory, enabling you to start tracking your changes.
Cloning a Repository with a Specific Python Version
If you wish to work on a project that already exists, you can clone it using:
git clone <repository-url>
After cloning, it's essential to set up a virtual environment that matches the version of Python you're targeting. You can create one using `virtualenv` or `venv` like so:
python2 -m virtualenv venv
Activate the virtual environment to ensure that the older Python version is used for your modifications.
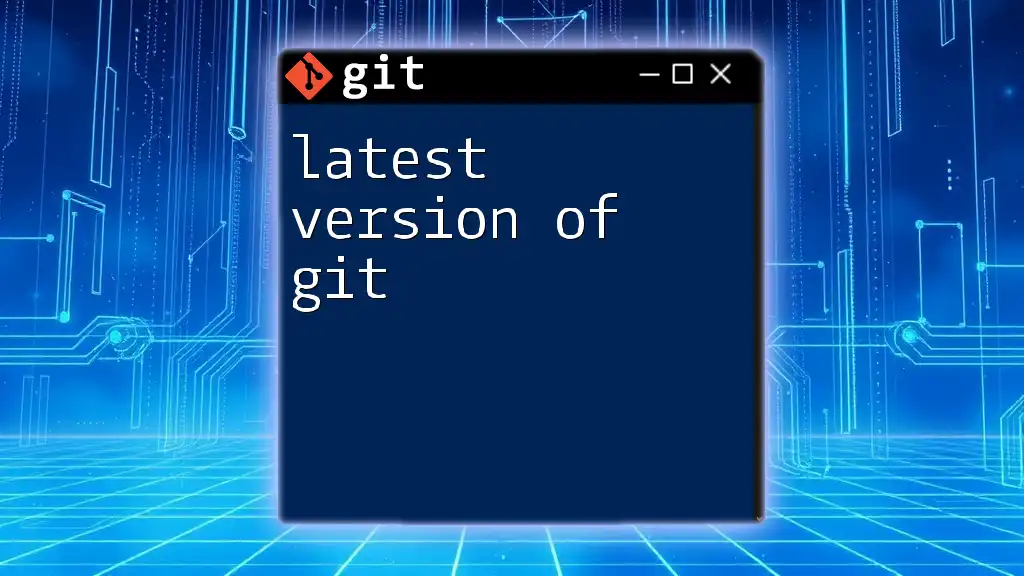
Managing Branches in Git
Creating and Switching Branches
When editing in an old Python version, it’s a good practice to create a separate branch to isolate your changes. This can be done using:
git checkout -b python-legacy
Creating branches allows you to experiment without affecting the main codebase, making it easier to manage different versions of your code.
Merging Branches Safely
To integrate your changes back into the main branch, you can merge your legacy branch:
git merge python-legacy
During this process, you may run into merge conflicts. Git will highlight the differences in code, and you can manually resolve them by choosing the appropriate changes for compatibility.
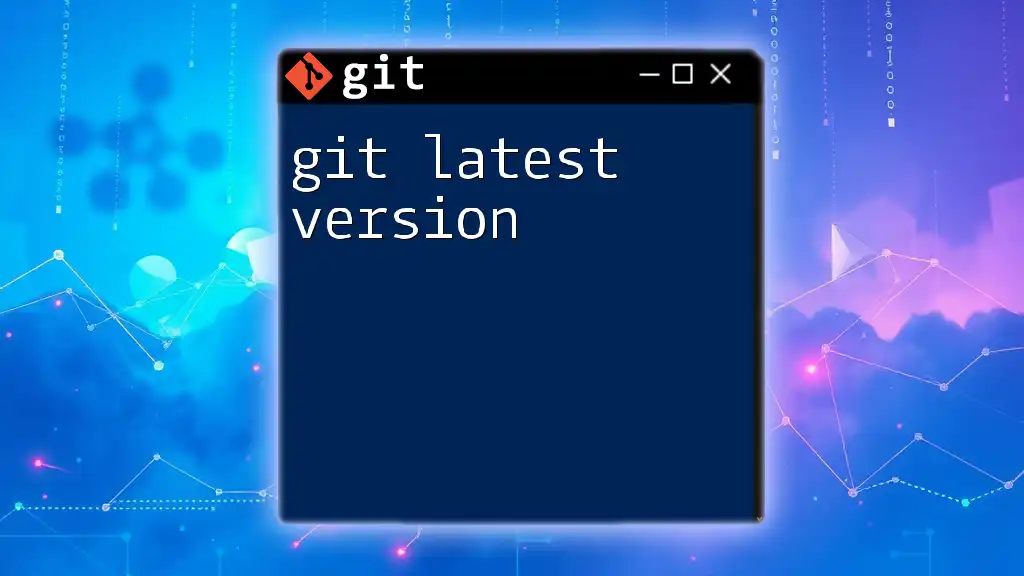
Editing Code for Compatibility
Best Practices for Writing Code in Older Python Versions
When editing code in an older Python version, there are several best practices to keep in mind:
- Use compatible syntax: As mentioned earlier, be mindful of the differences in syntax, such as print statements and integer division.
- Utilize backward-compatible features: Where applicable, always choose features that exist in both versions to enhance the code's resilience.
For instance, when doing a division:
# Python 2
result = 5 / 2 # yields 2
# Python 3
result = 5 // 2 # yields 2
Helpful Python Libraries for Legacy Code
Several libraries maintain compatibility across Python versions. Common libraries include:
- six: A library designed to help write code compatible with both Python 2 and 3.
- future: A tool that allows you to use Python 3 syntax and features while still supporting Python 2.x.
Using these libraries facilitates smoother transitions and compatibility.
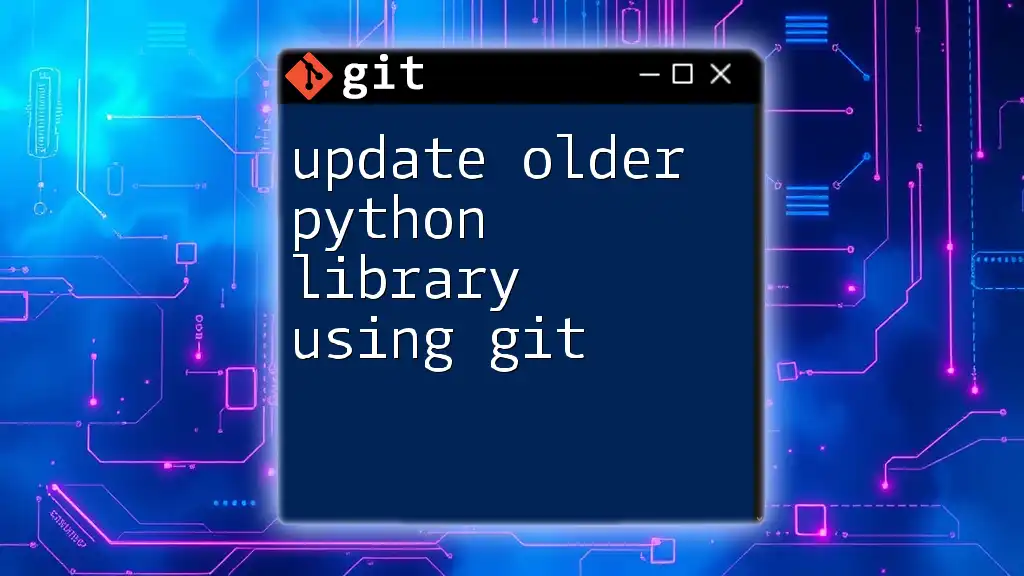
Testing Your Changes
Writing Test Cases for Older Versions
Testing is paramount when working with older Python versions. Utilizing frameworks like `unittest`, you can ensure your code behaves as expected:
import unittest
class TestLegacyCode(unittest.TestCase):
def test_addition(self):
self.assertEqual(1 + 1, 2)
if __name__ == '__main__':
unittest.main()
This allows you to create formal test cases that can be run to verify the integrity of your changes.
Running Tests and Ensuring Compatibility
To ensure your code runs as intended, execute your tests with the specific Python version you are targeting. Use the following command:
python -m unittest discover
This command will search for all test cases in the current directory and execute them, providing feedback on your code's functionality.
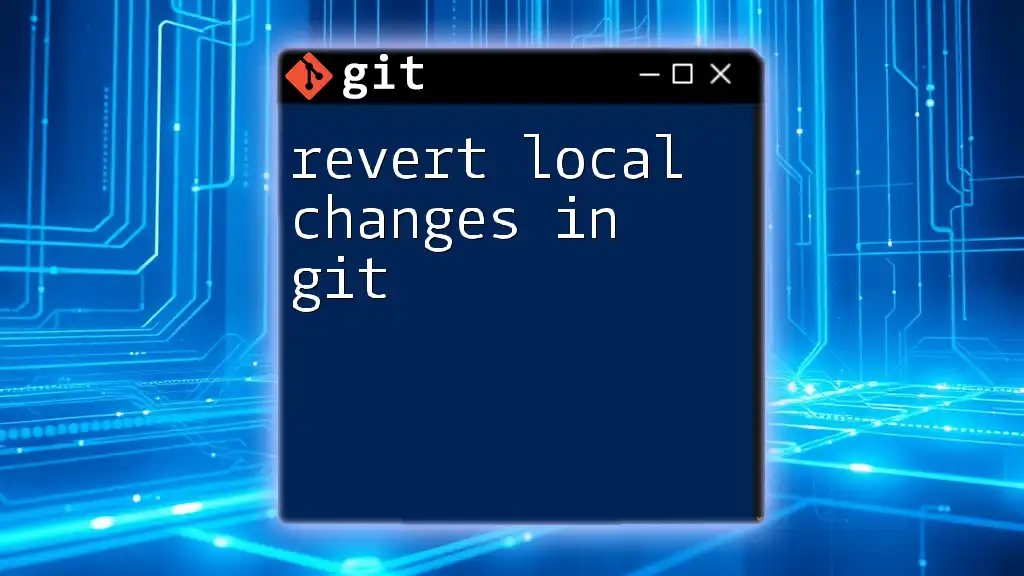
Committing Your Changes
Understanding the Commit Process
Once you have verified that your edits function correctly, it’s time to commit your changes. An effective commit message describes what you've changed and why. Execute:
git commit -m "Fix compatibility issues with Python 2.7"
Well-structured commit messages enhance the clarity of the project history, making it easier for other collaborators to understand the rationale behind changes.
Pushing Changes to Remote Repositories
After your modifications are complete and committed, the final step is to push your changes back to the remote repository. To do this, simply run:
git push origin python-legacy
This command uploads your changes to the specified branch in the remote repository, making them accessible to other team members.
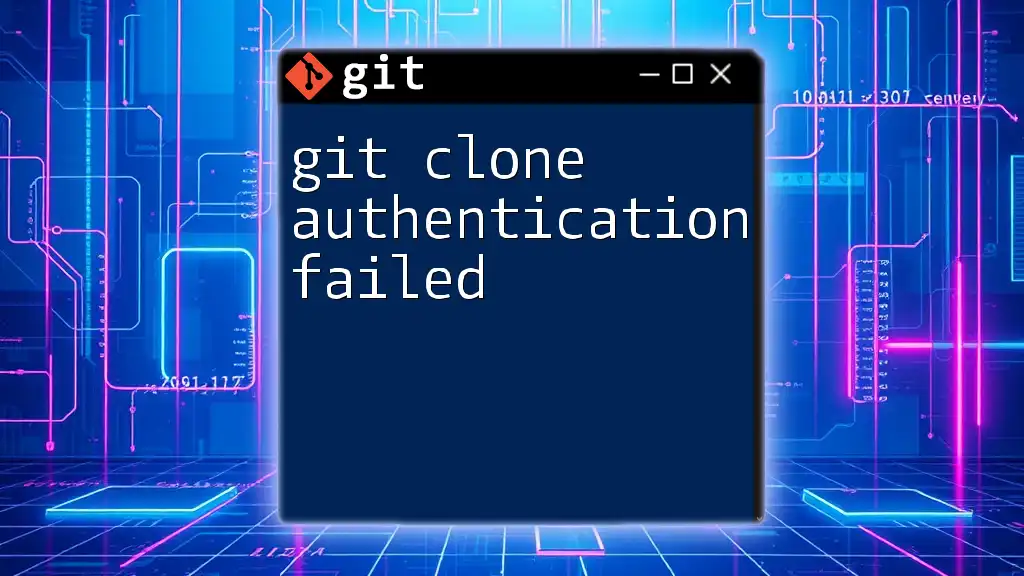
Dealing with Common Issues
Resolving Version Conflicts
When merging or working in different environments, you might encounter version conflicts. A common issue occurs when you use libraries that have not been updated to support newer versions of Python. In such cases, revert to an earlier version of the library or explore alternative libraries to restore compatibility.
Using Docker for Version Management
To manage different Python versions more effectively, consider using Docker. Docker allows you to create containers, each with its unique environment, simplifying compatibility issues. A basic Dockerfile for an older Python version might look like this:
FROM python:2.7
# Set the working directory
WORKDIR /app
# Copy code into the container
COPY . .
# Install dependencies
RUN pip install -r requirements.txt
# Command to run the application
CMD ["python", "your_script.py"]
With this configuration, you can ensure that the environment is consistent regardless of where the code is run, thus mitigating version conflicts.
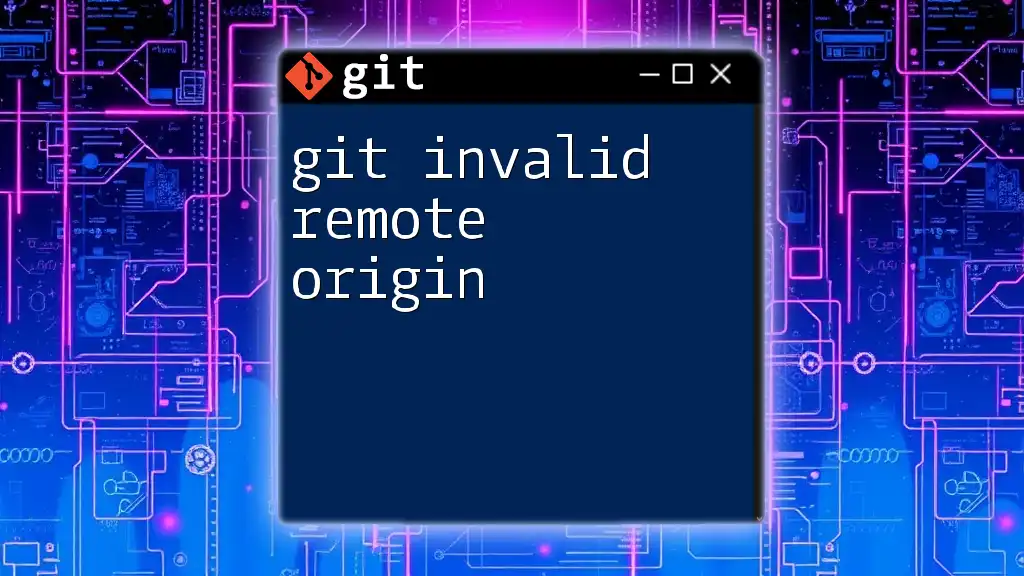
Conclusion
Editing in an old Python version in Git requires a clear understanding of both Git functionalities and the specific Python version differences. By creating separate branches, ensuring compatibility, and properly managing your environment, you can navigate legacy code with confidence. With practice, these skills will serve you well in maintaining and evolving your projects effectively. Embrace these practices, and you’ll find yourself equipped to handle any challenges that may arise in your journey through version control and legacy code maintenance.
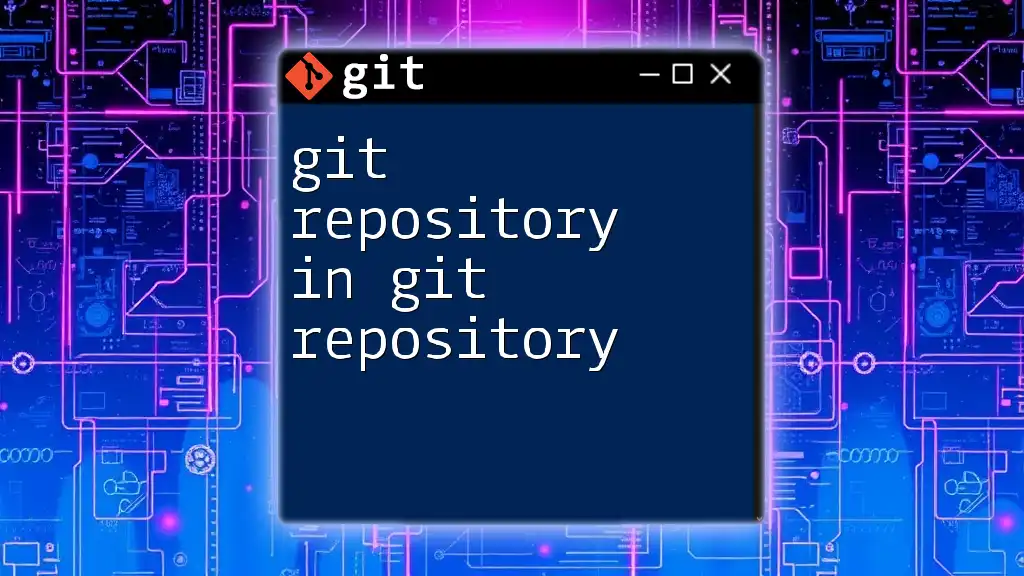
Additional Resources
To deepen your understanding of Git and legacy Python code, consider exploring additional reading materials, online tutorials, and community forums. Engaging with these resources will only augment your skills and enhance your coding prowess.