To update an older Python library in your project using Git, navigate to the library's directory and execute the `git pull` command to fetch the latest changes from the remote repository.
cd path/to/your/library
git pull origin main # Replace 'main' with the appropriate branch if necessary
Understanding Git Basics
What is Git?
Git is a distributed version control system that allows developers to track changes in their code over time. It's an essential tool in modern software development, enabling collaboration among teams and maintaining a history of modifications. By understanding Git, you can significantly simplify the process of managing updates for your Python libraries.
Key Git Commands for Library Management
Several Git commands are crucial for efficiently managing and updating your Python libraries:
-
`git clone`: This command is used to create a local copy of a remote repository, which is your starting point to modify the library.
-
`git fetch`: This retrieves updates from a remote repository but does not automatically merge them into your working directory. It allows you to see what's changed before you actually integrate those changes.
-
`git pull`: This performs a `fetch` followed by a `merge`, updating your local branch with changes from the remote repository. It's a convenient way to ensure your library is up to date with the latest code.
-
`git checkout`: This command allows you to switch between different branches or restore specific files to a previous state, giving you the ability to navigate the history of your project easily.
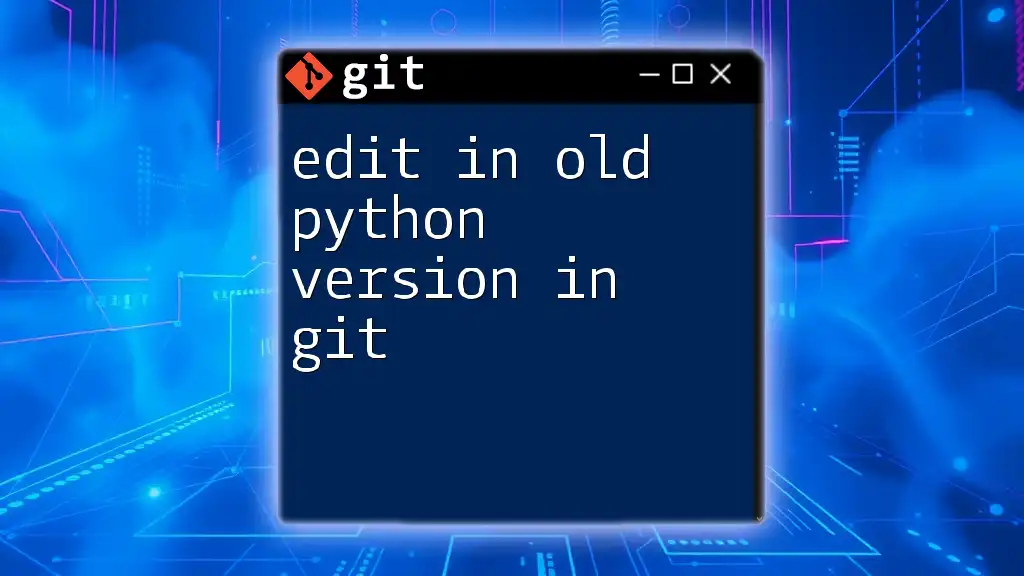
Locating the Python Library
Finding the Repository
To update an older Python library, you first need to locate its Git repository. This can often be found on platforms like GitHub, Bitbucket, or GitLab. You can search the library’s name or related keywords in these repositories. Make sure to look for the official repository associated with the library to ensure you're pulling from a trusted source.
Cloning the Repository
Once you have identified the repository, you can clone it to your local machine. This creates a complete copy of the repository, including all its history.
Example Command:
git clone <repository-url>
Replace `<repository-url>` with the actual URL of the library repository. This command sets up your local environment, allowing you to modify and update the library.
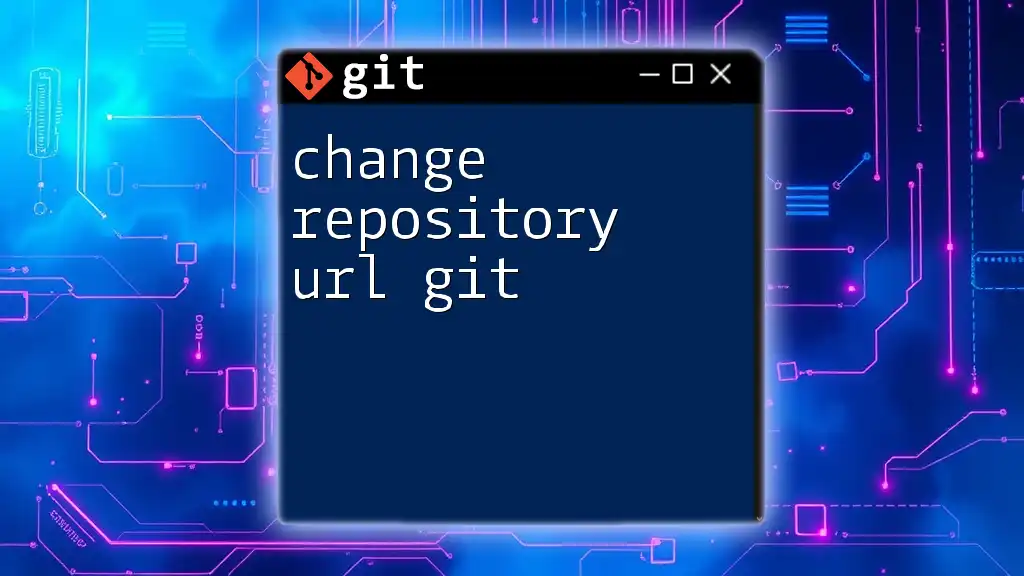
Updating the Library
Fetching Updates
After cloning the repository, it’s crucial to check for updates. The `git fetch` command is a safe way to download changes without altering your working directory.
Using `git fetch`:
git fetch origin
This command retrieves any new information from the remote repository without merging it immediately. It allows you to review updates and decide how to proceed.
Merging Changes
Using `git pull`
To combine the fetched changes into your local work branch, you can use `git pull`. This command automatically fetches and merges changes from your chosen branch.
Example Command:
git pull origin main
This command merges the latest changes from the `main` branch of the remote repository into your current branch. It’s essential to resolve any merge conflicts promptly, ensuring that your library integrates seamlessly with the latest updates.
Checking Out Specific Versions
Using Tags for Releases
Many libraries use tags to mark stable releases. You can view these tags and switch to a specific release as needed.
Example Command:
git tag
git checkout tags/<tag-name>
Replace `<tag-name>` with the relevant tag. This allows you to access a specific version of the library, ensuring compatibility with your projects.
Creating a Stable Branch
Maintaining a stable branch is also a best practice when updating libraries. This allows you to safeguard your work while testing new updates.
Example Command:
git checkout -b stable-branch
This creates a new branch named `stable-branch`, letting you experiment with the latest features and fixes without affecting your stable code.
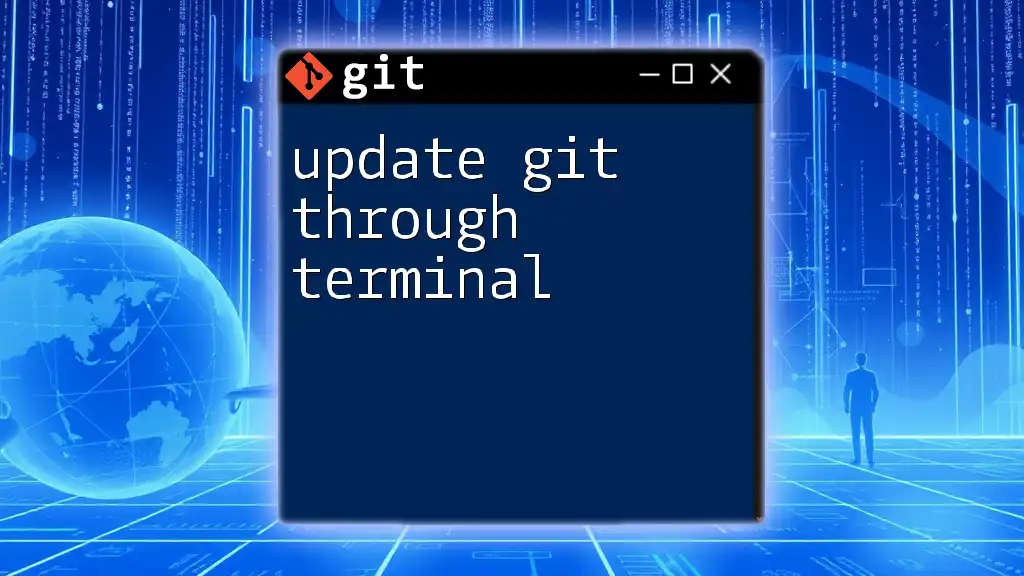
Testing the Updated Library
Running Tests Locally
Once you have updated the library, it’s crucial to test its functionality. This ensures that the new updates do not break existing features. Many Python projects utilize testing frameworks like Pytest or Unittest to facilitate this process.
Example of Running Tests
To run your tests, use a command like:
pytest tests/
This executes all tests located in the `tests` directory, helping you verify that everything works correctly after the update. Interpreting the test results promptly will guide you in making any necessary adjustments.
![How to Delete Origin Branch in Git [Step-by-Step Guide]](/images/posts/d/delete-origin-branch-git.webp)
Resolving Potential Issues
Common Problems During Updates
When updating an older Python library, you might encounter several common issues, including merge conflicts or broken dependencies. Merge conflicts arise when two branches have competing changes. Git will alert you to this, and you will need to resolve the conflicts manually.
Dependency issues may also occur after you pull updates, especially if the library has updated its dependencies. Carefully review the documentation or README file for any breaking changes.
Best Practices for Maintaining Libraries
To maintain a healthy project, regularly check for updates and patches. This practice ensures you are utilizing the latest features and improvements while ensuring security. Additionally, documenting changes in your code can provide clarity and simplify future updates.
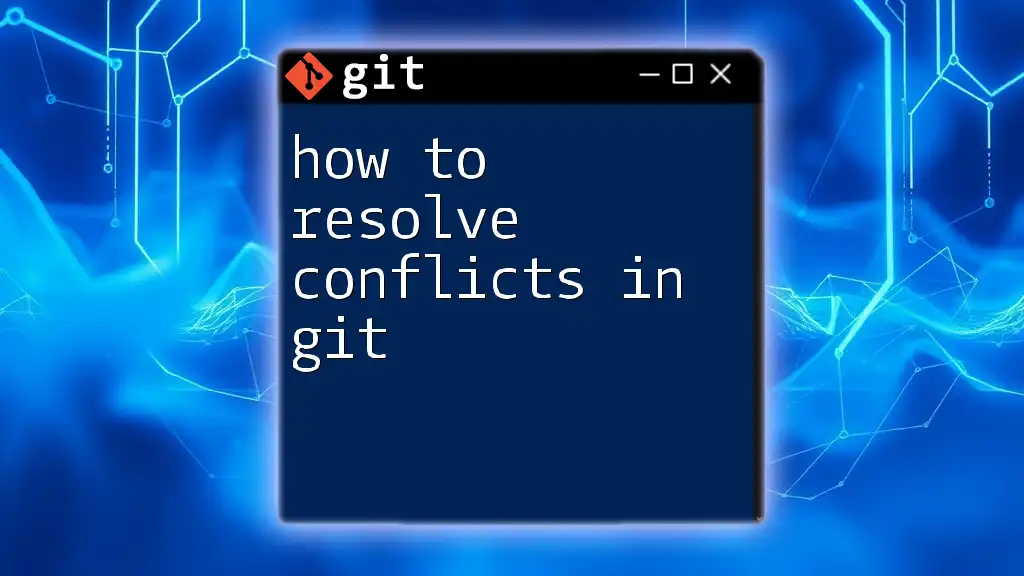
Conclusion
Updating an older Python library using Git doesn't have to be daunting. By following these steps—cloning the repository, fetching and merging changes, running tests, and maintaining a stable branch—you can efficiently manage updates. Start practicing with a sample library today, and as you grow comfortable, you will find updating libraries becomes second nature.
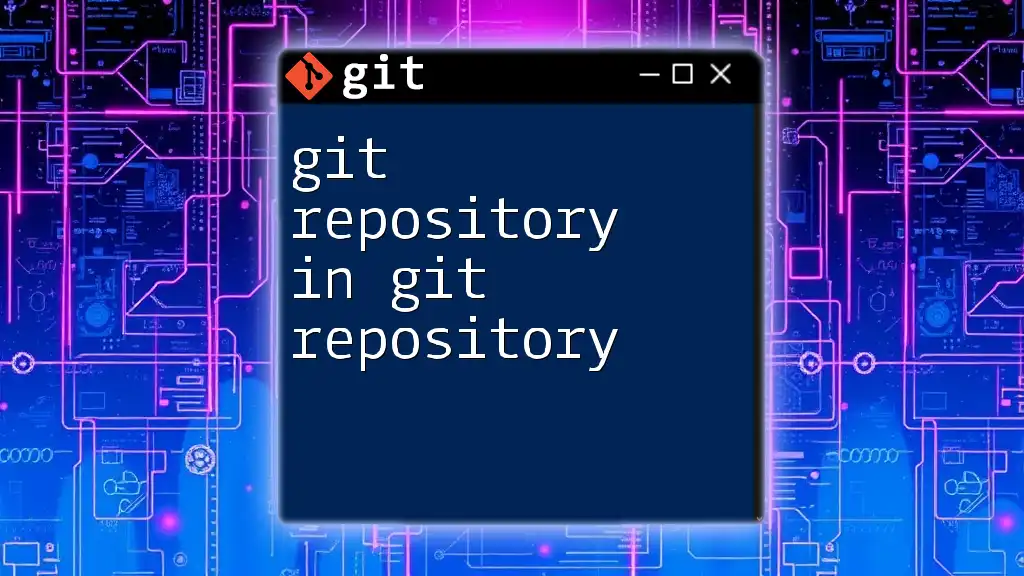
Additional Resources
For further reading, look into additional resources about Git commands or consult the official documentation of the Python libraries you are updating. Expanding your knowledge in both Git and Python libraries will enhance your programming skill set significantly.