"Build your own git" refers to creating a simplified or customized version of Git commands to enhance user understanding and efficiency in version control.
Here’s a basic command to initialize a new Git repository:
git init
Understanding Git Basics
What is Version Control?
Version control systems are essential tools for tracking changes in files, particularly in software development. They allow multiple individuals to collaborate effectively on projects without overwriting each other's work. By maintaining a complete history of changes, version control systems help developers manage revisions, revert to previous states, and facilitate collaboration.
Overview of Git
Git, created by Linus Torvalds in 2005, is one of the most widely used version control systems today. Its key features include:
- Distributed architecture: Each developer has a full copy of the repository, enabling offline work.
- Branching and merging: Git allows users to create branches for feature development and later merge their changes back into the mainline code seamlessly.
- Speed: With its efficient storage and handling of version history, Git can perform operations quickly, even on large projects.
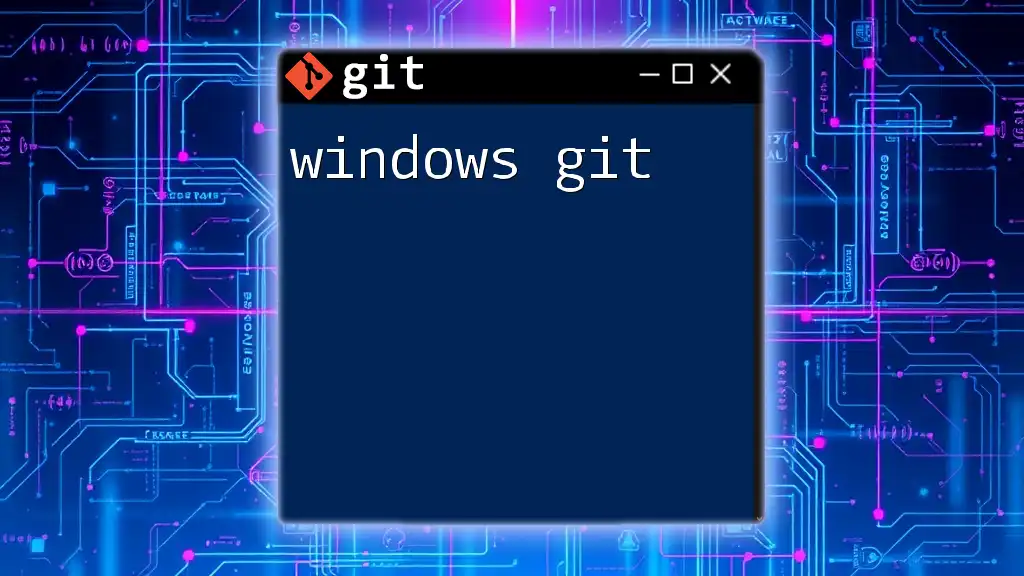
Setting Up Your Environment
Requirements
Before building your own version control system, it’s crucial to ensure you have the necessary tools and technologies in place. You will need:
- A code editor such as VSCode, Atom, or Sublime Text to write your code.
- Terminal access to execute commands in your development environment.
- A programming language that's easy to work with — Python or Node.js are excellent choices for this project.
Installation Steps
To start building your own Git-like system, follow these steps:
- Install Git (for reference) by downloading it from the official website and following the installation instructions for your operating system.
- Set up a local development environment by creating a new directory for your project:
mkdir my_vcs cd my_vcs
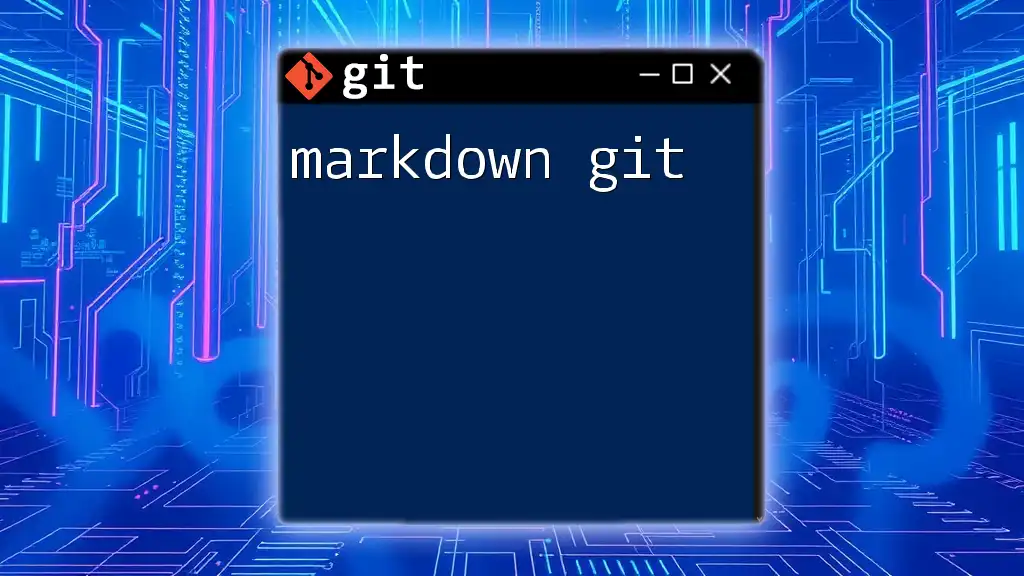
Building Your Version Control System
Choosing the Right Language
Selecting the right programming language is critical for building your version control system. Python is an excellent choice due to its readability and extensive libraries. Node.js is also a viable option, especially for developers familiar with JavaScript. Choose a language based on your current skills and the community support available.
Core Features to Implement
Initializing a Repository
The first step in building a version control system is implementing the ability to initialize a repository. This command sets up the structure needed to start tracking changes.
Code Snippet:
def init_repository():
# Create necessary directories and files
os.makedirs('.my_vcs', exist_ok=True)
with open('.my_vcs/README.md', 'w') as f:
f.write("# My VCS\n")
This function initializes a new repository by creating a hidden directory where all version control data will be stored.
Tracking Changes
To track changes in files, you'll need to implement an `add` command that stages the files you want to track.
Code Snippet:
def add(file_name):
# Read the file content and prepare it for tracking
with open(file_name, 'r') as f:
content = f.read()
# Store the content in the repository
with open('.my_vcs/staged/' + file_name, 'w') as f:
f.write(content)
By executing this function, the content of a specified file is staged for future commits.
Committing Changes
Committing changes allows users to save a snapshot of their current state. This is where you need to implement a `commit` function to record changes along with a message describing the commit.
Code Snippet:
def commit_changes(message):
with open('.my_vcs/commits.txt', 'a') as commit_file:
commit_file.write(f'{datetime.now()}: {message}\n')
This will append a commit message along with the timestamp to a log, providing a history of the commits made in the repository.
Viewing Commit History
To help users track changes over time, you need a function that displays the commit history.
Code Snippet:
def view_commit_history():
with open('.my_vcs/commits.txt', 'r') as commit_file:
for line in commit_file:
print(line.strip())
This simple function reads the commit log and prints its contents to the console, providing an easy way to view the progress and modifications made to the project.
Implementing Branching and Merging
What are Branches?
Branches are essential in a version control system, allowing you to create parallel lines of development. When you branch off, you can experiment or develop features without affecting the main project.
Creating Branches
You can create a new branch using the following method:
Code Snippet:
def create_branch(branch_name):
os.makedirs(f'.my_vcs/branches/{branch_name}', exist_ok=True)
This structure allows developers to manage their features independently from the main line of development.
Merging Branches
Merging is a crucial feature of any version control system, as it combines changes from different branches into a single branch.
Code Snippet:
def merge_branches(target_branch, source_branch):
# Logic to merge branches and handle conflicts
pass
You'll need to implement logic to handle conflicts, ensuring that changes from both branches can coexist.
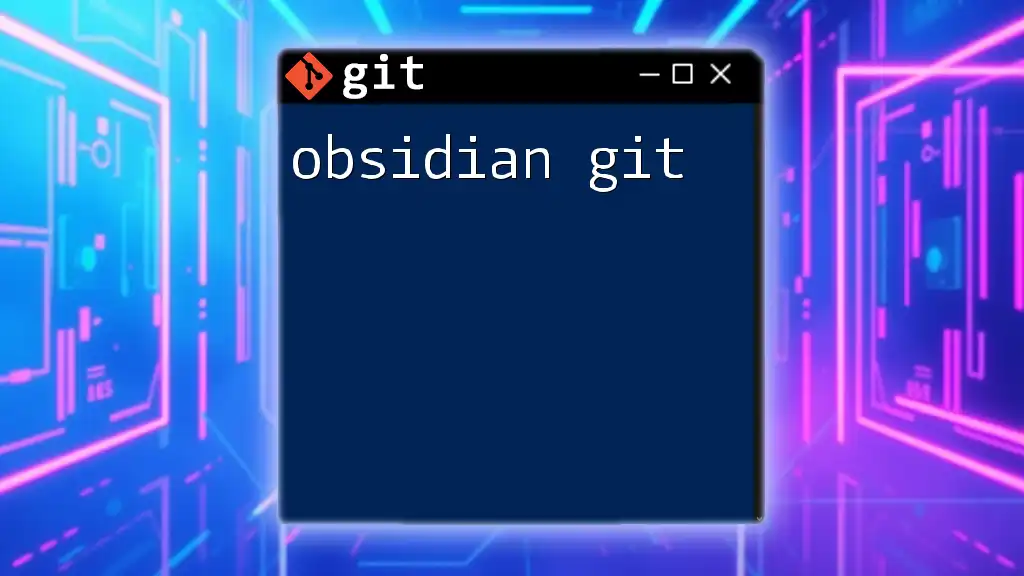
User Interface
Command Line vs GUI
When building your own Git-like system, you can choose between a command line interface (CLI) and a graphical user interface (GUI). CLIs are faster and more precise but may have a steeper learning curve for beginners. GUIs can be more user-friendly and visually appealing, enhancing user experience.
Creating a Simple CLI
Creating a command-line interface requires parsing user input and executing the corresponding functions you've developed.
Code Snippet:
import argparse
def main():
parser = argparse.ArgumentParser(description='My VCS Command Line')
parser.add_argument('command', choices=['init', 'add', 'commit', 'view', 'branch', 'merge'])
args = parser.parse_args()
if args.command == 'init':
init_repository()
# Similarly, handle other commands based on user input
if __name__ == "__main__":
main()
This script provides a basic command-line framework where users can execute commands related to the version control system.
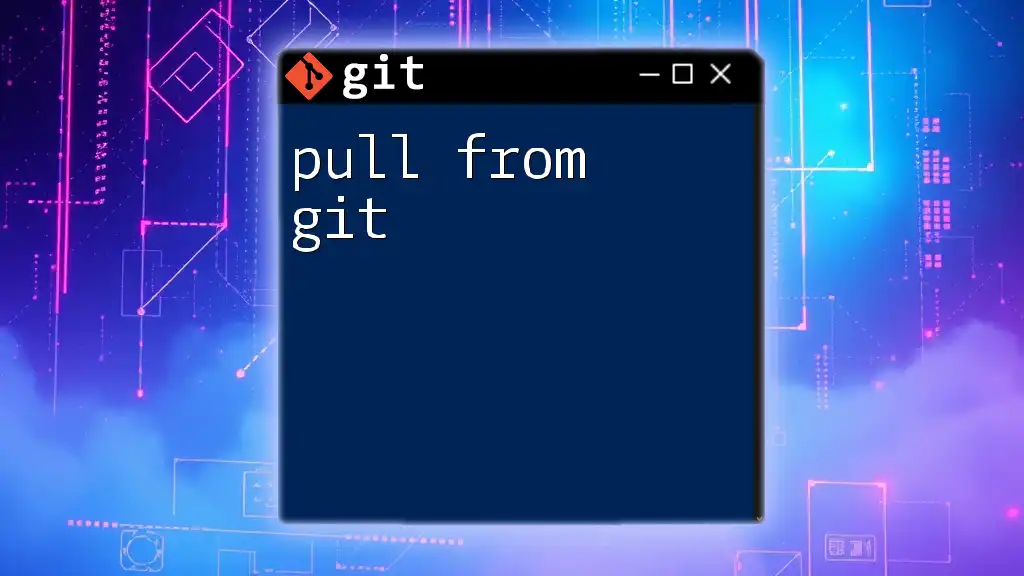
Testing Your VCS
Writing Test Cases
Testing is vital to ensure the reliability of your version control system. Write unit tests for each core feature to validate their functionality and catch any bugs before deployment.
Example:
def test_commit_changes():
commit_changes("Initial commit")
# Check if the commit was recorded correctly in commits.txt
Running Tests
Use testing frameworks like `unittest` or `pytest` to run and manage your tests effectively. They help automate the testing process and provide comprehensive feedback on the systems you build.
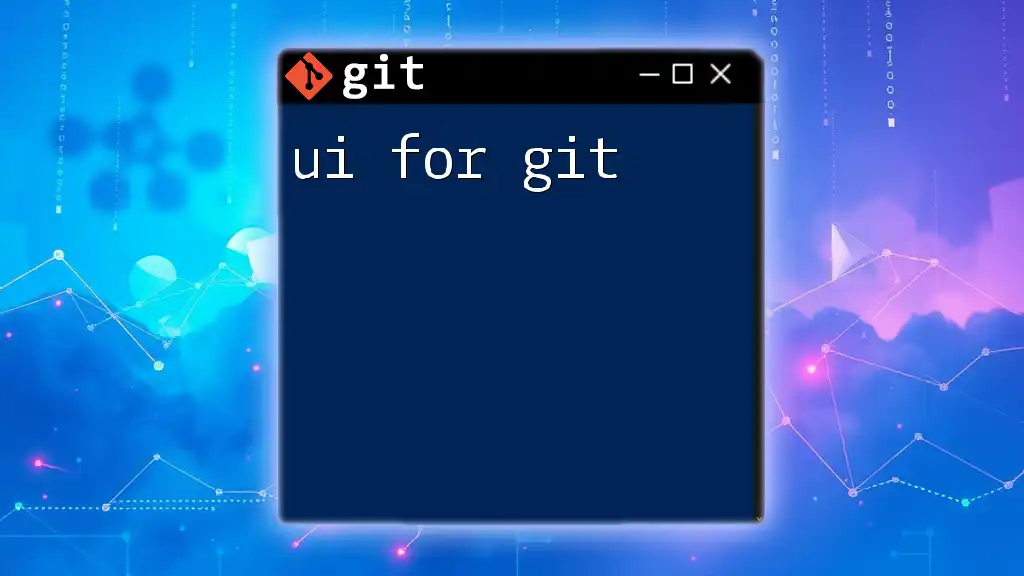
Deployment Considerations
Hosting Your VCS
After building your version control system, consider exploring options for deployment. You can host it locally on your machine or deploy it to the cloud for easier accessibility.
Continuous Integration
Integrating continuous integration (CI) practices allows you to automate testing and deployment for your version control system, improving its reliability and efficiency.
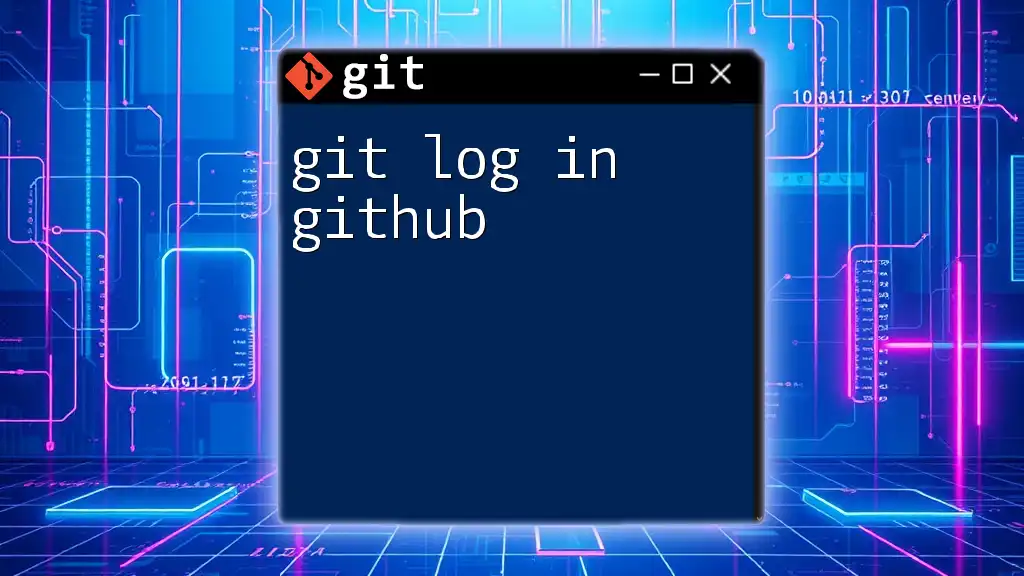
Conclusion
In this guide, we explored how to build your own Git-like version control system from the ground up. From understanding core features to implementing functionalities for branching, committing, and merging, this project empowers you to grasp the inner workings of version control tools. Don’t hesitate to experiment, innovate, and refine your design. Each enhancement brings you closer to achieving a powerful tool that may just rival established VCS systems.
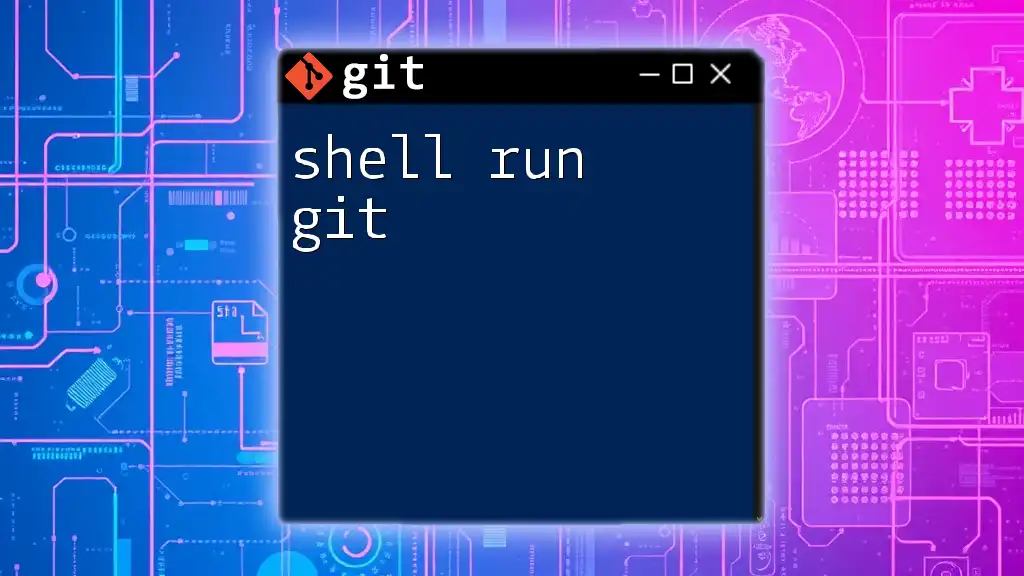
Further Resources
For additional learning materials, documentation, and communities, consider exploring:
- The official Git documentation for advanced features.
- Community forums like Stack Overflow and GitHub to connect with other developers.
- Open-source projects to see how other developers implement version control systems.
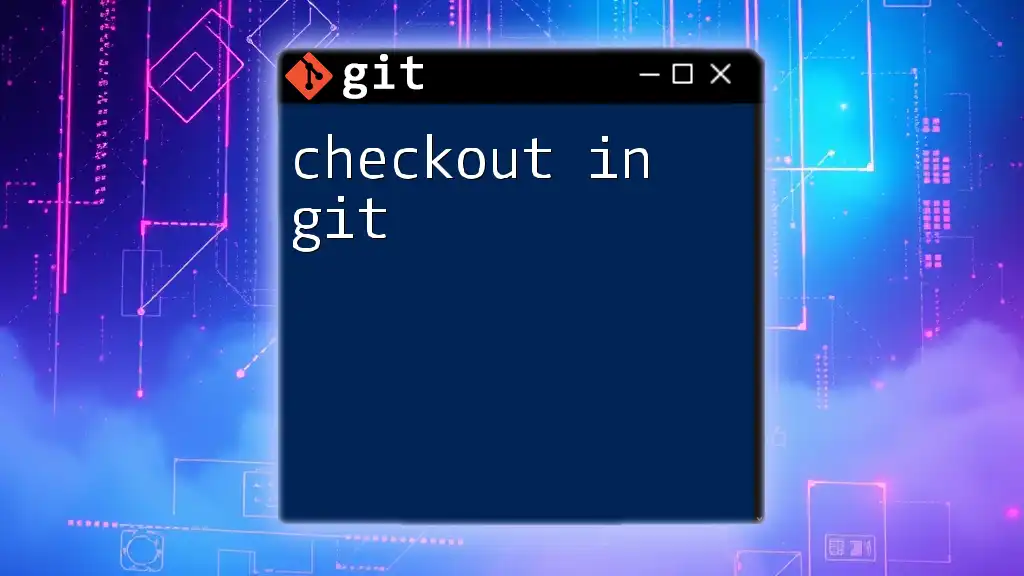
Call to Action
We invite you to share your experience as you embark on building your version control system. What challenges did you face? What enhancements would you like to make? Your insights can help others on their journey to mastering version control!