Git flow is a branching model that helps manage feature development, releases, and hotfixes in a structured way, allowing teams to work on different stages of a project simultaneously.
Here's a code snippet to illustrate starting a new feature branch using git flow:
git flow feature start <feature-name>
What is Git Flow?
Git Flow is a systematic branching model for using Git in a controlled and effective manner, primarily designed for software development projects. It provides a clear and structured way to manage changes by defining a set of roles for branches and rules for how they interact. By adopting the Git Flow versioning strategy, teams can facilitate better collaboration, minimize errors during merges, and streamline their release process.
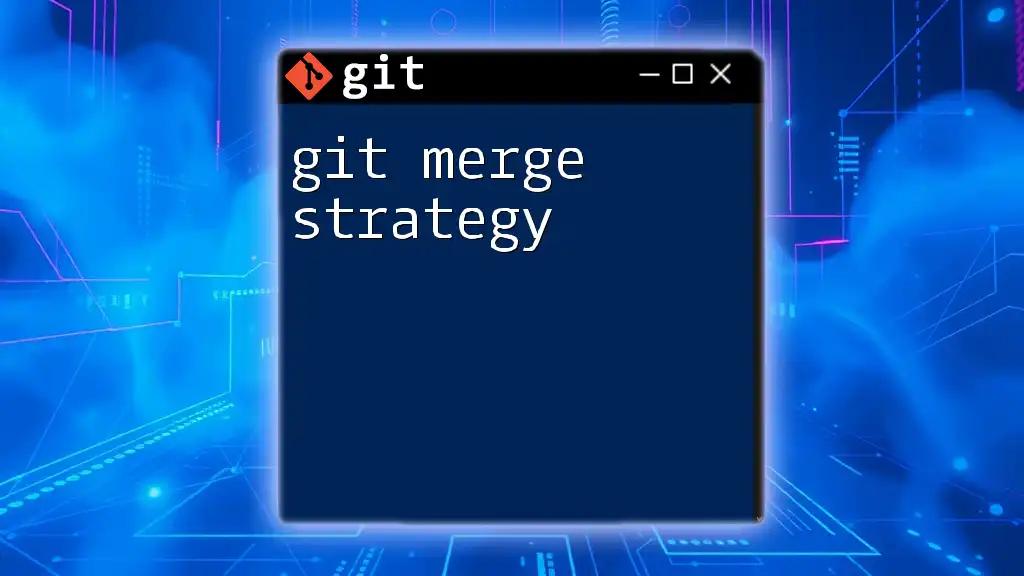
Benefits of Using Git Flow
Using Git Flow offers multiple advantages:
- Enhanced Collaboration: It provides clear guidelines on how to branch and merge, making it easier for team members to work concurrently on different features without stepping on each other’s toes.
- Clearer Project Structure: With well-defined branches for features, releases, and hotfixes, project status is easier to track, making it simpler to manage ongoing work.
- Streamlined Release Process: The structured approach allows for more predictable and organized releases, enhancing overall productivity.
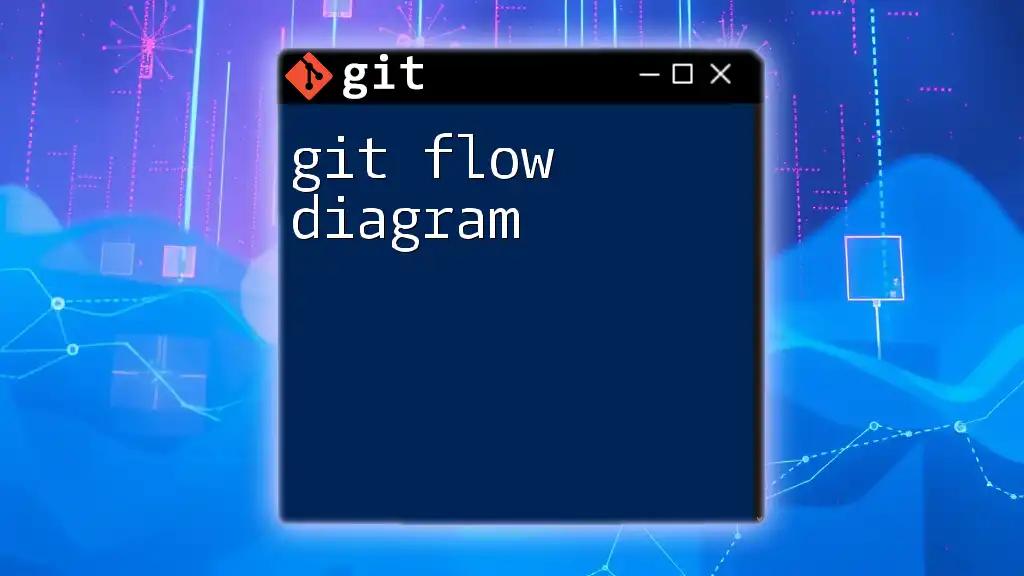
Core Concepts of Git Flow Versioning
Understanding Branches in Git Flow
Main Branches
In the Git Flow model, two main branches are utilized:
-
`master` or `main`: This branch contains the stable codebase and represents the product's current release. Every commit on this branch reflects a version that can be deployed to production.
-
`develop`: This is where the code for the next release lives. It serves as an integration branch for features and is where all active development takes place.
Supporting Branches
Feature Branches
Feature branches are created to develop new functionalities. They allow developers to work in isolation without disrupting the main codebase.
Naming conventions for feature branches often follow this structure:
feature/{feature-name}
For instance, if you’re adding user authentication, you might name your branch `feature/user-authentication`.
Release Branches
Release branches are used to prepare for a new version of the software. They allow for last-minute tweaks, testing, and documentation preparation without stopping feature development.
Common naming convention is:
release/{version-number}
For example, `release/1.0.0`.
Hotfix Branches
Hotfix branches are essential for addressing critical issues in a production environment. They can be initiated from the `master` branch, allowing for quick resolution of bugs.
Naming convention typically includes:
hotfix/{version-number}
As an example, a hotfix for version 1.0.1 would be `hotfix/1.0.1`.
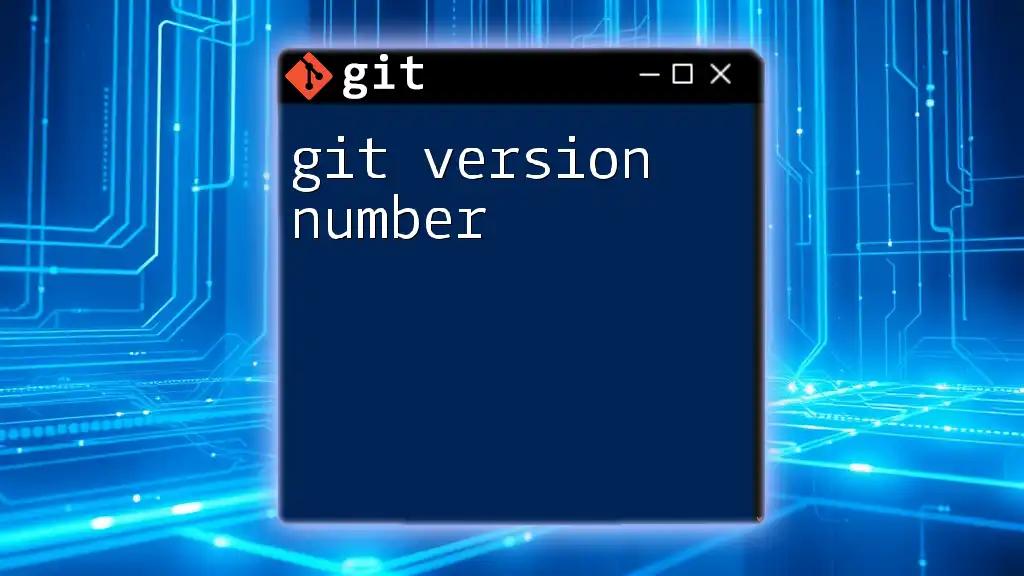
Implementing Git Flow
Prerequisites for Git Flow
Before diving into Git Flow, ensure you have set up a Git repository and installed Git Flow extensions. These extensions can be found for both command line and GUI interfaces.
Initializing Git Flow in Your Repository
To begin using Git Flow, you'll need to initialize it in your repository. Use the following command:
git flow init
This command prompts you to identify the primary branches and naming conventions that fit your workflow, setting up an organized structure right from the start.
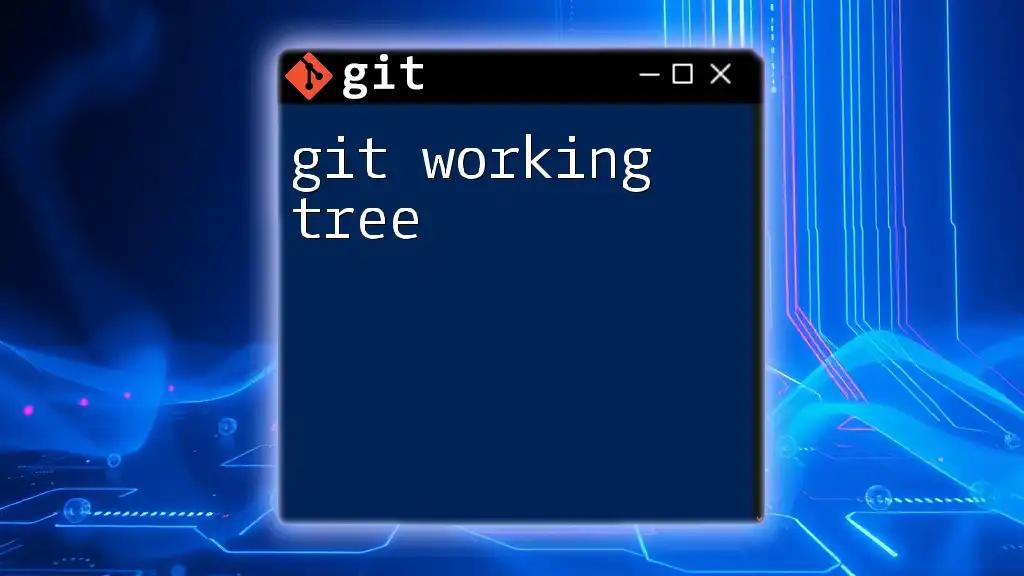
Using Git Flow Commands
Creating a Feature Branch
When ready to work on a new feature, create a feature branch using the following command:
git flow feature start <feature-name>
This command creates a branch and switches your working directory to this new branch. Once the feature is complete, you can finish it with:
git flow feature finish <feature-name>
This will merge the feature back into the `develop` branch, ready for integration and testing.
Creating a Release Branch
Once development on a new version is complete, it’s time to create a release branch:
git flow release start <version>
This will allow for any last-minute fixes or documentation updates. When your release is ready, finalize it with:
git flow release finish <version>
This command merges the release into both `master` (or `main`) and `develop` branches, tagging it in the process.
Handling Hotfixes
In cases where an urgent bug must be corrected immediately, initiate a hotfix branch:
git flow hotfix start <version>
Once the fix is implemented, close it out using:
git flow hotfix finish <version>
This merges the hotfix into both `master` and `develop`, ensuring the fix is included in the next release.
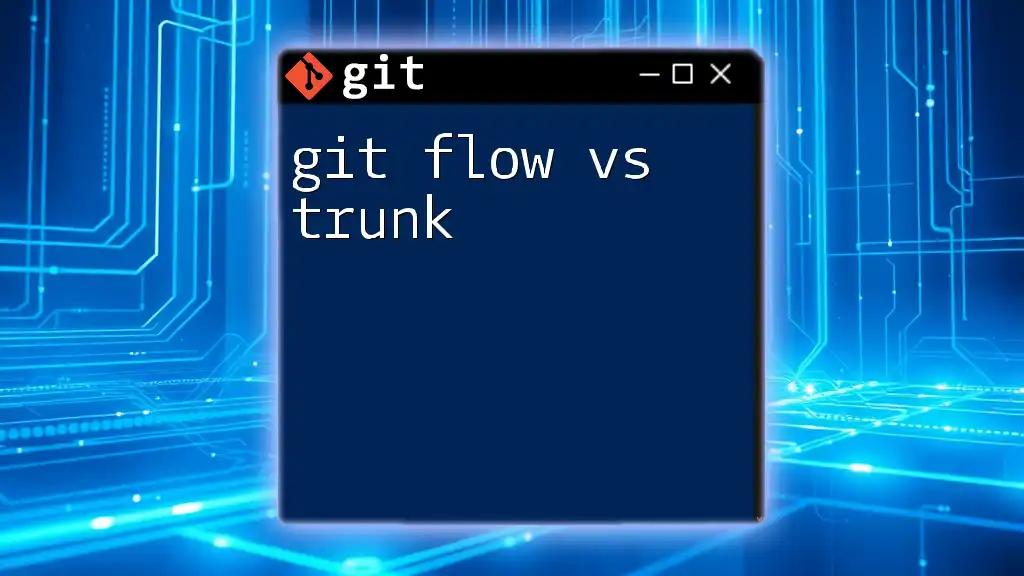
Versioning Best Practices with Git Flow
Semantic Versioning Explained
Semantic Versioning (often referred to as SemVer) is a widely adopted convention for versioning software. Following a format like `MAJOR.MINOR.PATCH`, it communicates the significance of changes. Git Flow aligns excellently with this strategy by using separate branches for active development versus releases.
Tagging Versions in Git
Tagging is a simple yet effective means to mark specific points in history as significant, commonly utilized for version releases. To tag a commit, use:
git tag -a v1.0.0 -m "Release version 1.0.0"
Tags are critical: they allow any team member to easily reference a particular version of your codebase.
Maintaining a Consistent Versioning Scheme
Having a consistent approach to versioning reduces complexity and promotes smoother collaboration, encouraging all team members to adhere to established protocols. Regular updates and reminders about your versioning strategy can ensure all team members remain aligned.
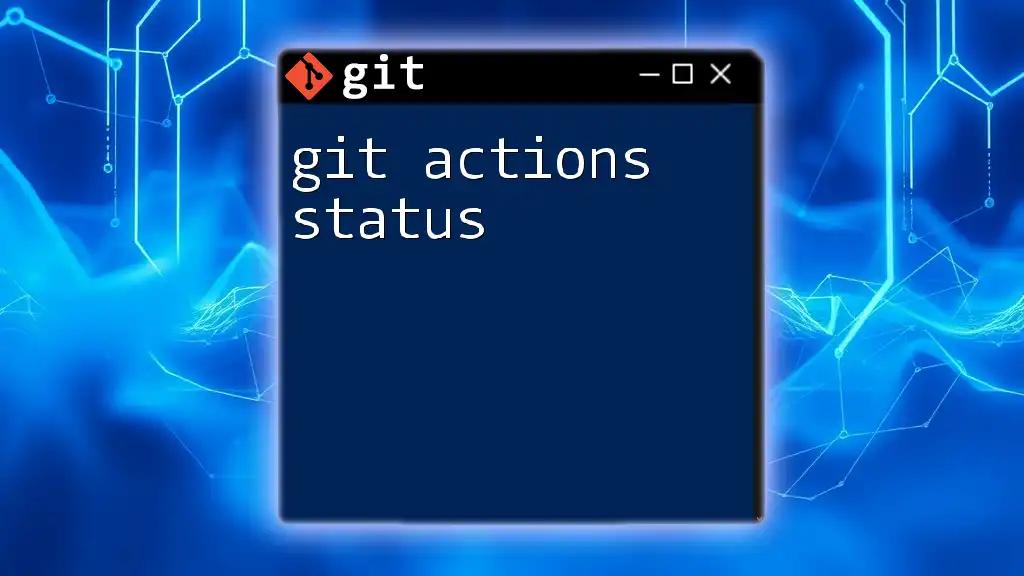
Real-World Example of Git Flow in Action
Consider a scenario where your team is building a new application feature for user profiles.
-
You start by initializing Git Flow and then create a feature branch:
git flow feature start user-profiles
-
After completing development, you would finish the feature:
git flow feature finish user-profiles
-
Next, as the project nears completion, you create a release branch:
git flow release start 1.0.0
-
After addressing final tweaks, you would finish the release:
git flow release finish 1.0.0
This structured process illustrates how Git Flow simplifies and organizes development, with clear paths for integration and release.
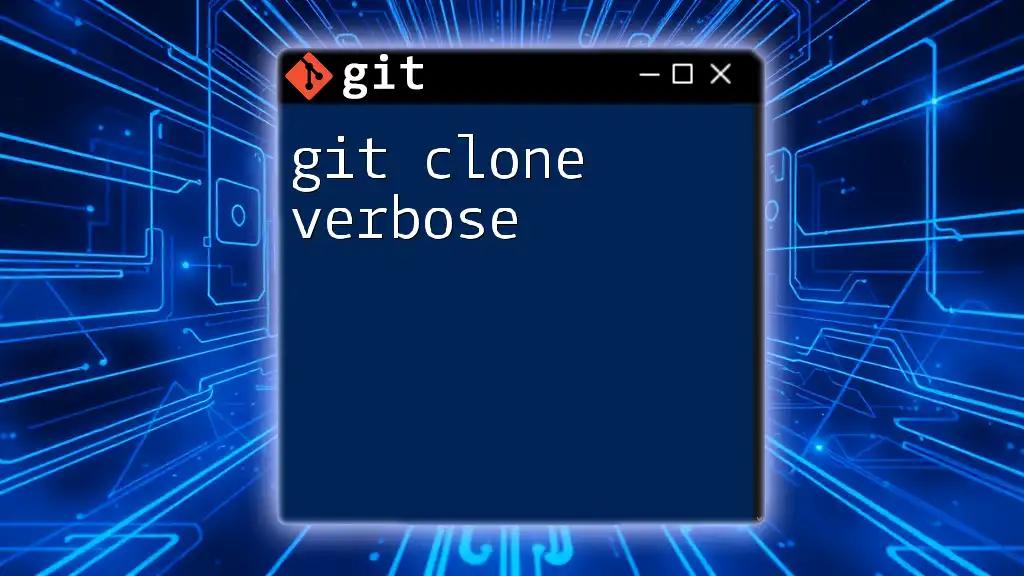
Common Challenges and Solutions
Potential Pitfalls of Git Flow
While Git Flow provides structure, it can introduce complexity, particularly in large teams or when the workflow isn’t consistently followed. Overcomplicating the process can overwhelm developers, leading to confusion.
Tips for Overcoming Common Hurdles
Regular education and training on Git Flow best practices can significantly enhance team performance. Implementing visual tools to display current branches and their statuses can also aid understanding and foster better collaboration.
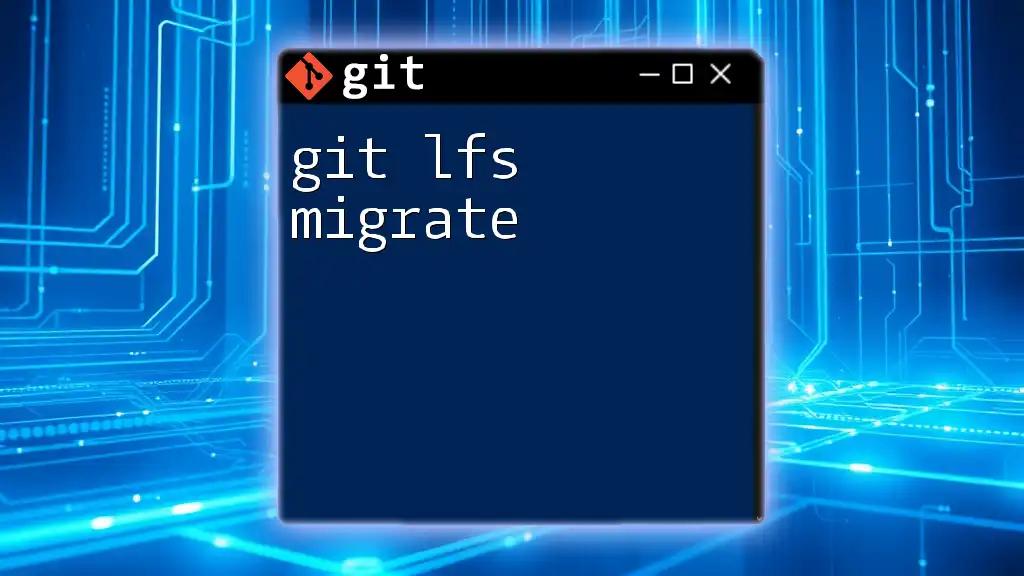
Conclusion
In summary, the Git Flow versioning strategy offers a robust and structured approach to managing software development. The clarity it brings to branching and merging ensures teams can work efficiently, reducing conflicts and paving the way for successful releases.
Additional Resources for Learning
For further exploration, consider referencing the official Git Flow documentation and checking out recommended books and online courses. Getting hands-on experience is also invaluable for mastering this powerful versioning strategy.
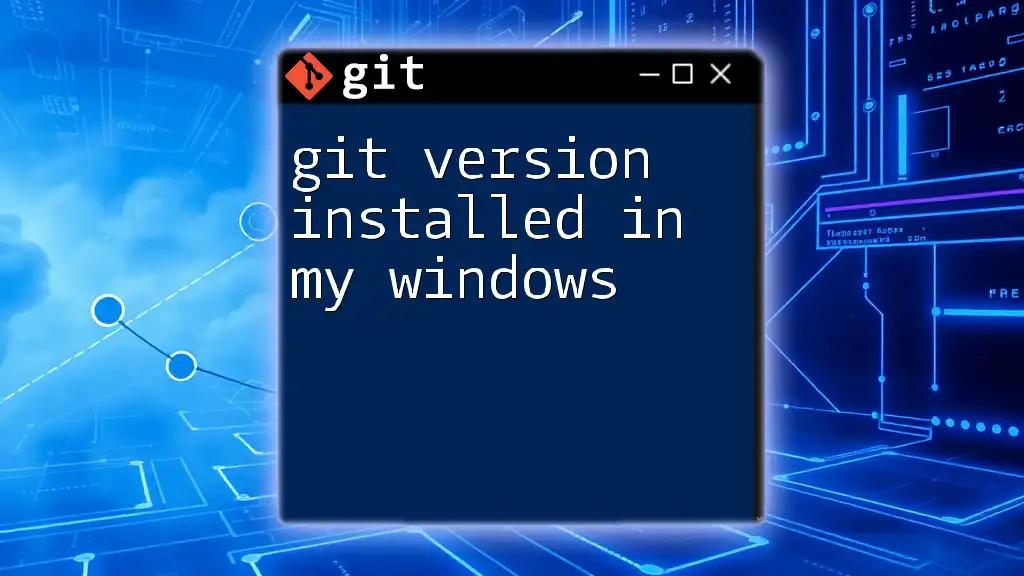
Frequently Asked Questions
What Makes Git Flow the Best Option for Versioning?
Git Flow stands out for its clear structure, promoting better collaboration while maintaining code integrity throughout the development process.
Can Git Flow Be Integrated with CI/CD Pipelines?
Yes, Git Flow can be seamlessly integrated with CI/CD workflows, permitting automated testing and deployment processes to enhance productivity.
How to Transition from a Different Versioning Strategy to Git Flow?
Transitioning involves educating your team on Git Flow principles, establishing consistent practices, and migrating existing branches to align with the new model. Regular communication can aid in smoothing the transition.