The `git rm -r --cached` command removes tracked files from the staging area without deleting them from your working directory, allowing you to untrack files while keeping them locally.
git rm -r --cached <file_or_directory>
What is `git rm -r --cached`?
Understanding the Command
The command `git rm` is fundamental in Git for removing files from your project's tracking system. By default, when you use this command, it will remove files from both your working directory and the Git index (staging area). However, the addition of the flags `-r` and `--cached` modifies this behavior to suit various scenarios.
-
The `-r` flag stands for "recursive." This means that if you’re removing a directory, it will remove all files and subdirectories within that directory, not just the top-level files. This is crucial for effectively managing directories in your Git repository.
-
The `--cached flag` is particularly important as it tells Git to remove the files from the staging area while leaving them intact in your working directory. This is useful when you've accidentally added files to your repository that you wished would not be tracked, but you still want to keep them locally.
Why Use `git rm -r --cached`?
This command serves multiple purposes. Its primary use cases include:
-
Untracking Files: Situations arise where you may not want certain files or directories to be included in your version control. Whether they are temporary files, large assets, or configuration files, using `git rm -r --cached` allows you to stop tracking them while still retaining them in your local directory.
-
Reorganizing Your Project Structure: When changing project structures, there may be instances where you need to remove entire folders from version control without deleting the actual files. This command effectively allows you to do just that.
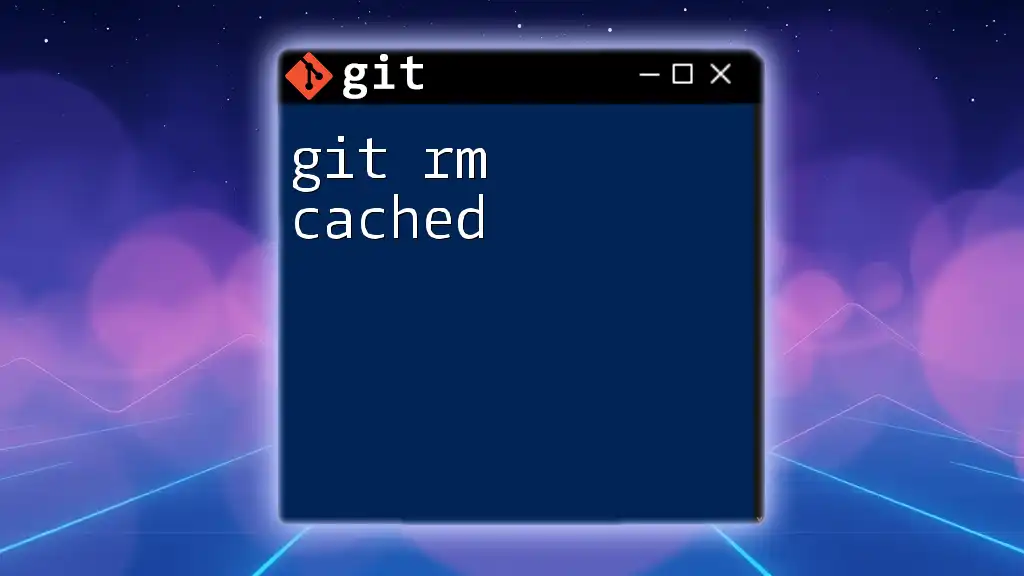
How to Use `git rm -r --cached`
Basic Syntax
To utilize this command, you can follow this general format:
git rm -r --cached <file/folder>
Step-by-Step Example
Let’s consider a concrete scenario where you're working on a project that contains a folder named `assets`, which houses large image files that you no longer wish to track in your Git repository.
-
Navigate to Your Project Directory: First, change into your project directory if you haven’t already:
cd /path/to/your/project
-
Check the Status of the Repository: It's a good habit to look at the current status of your Git repository:
git status
-
Remove the Folder from Cache: Now, run the command to remove the `assets` folder from the index:
git rm -r --cached assets/
-
Check the Status Again: After executing the command, check the status once more to confirm that the folder is staged for removal:
git status
-
Commit the Changes: Finally, commit your changes to ensure the repository reflects this removal:
git commit -m "Removed assets folder from tracking"
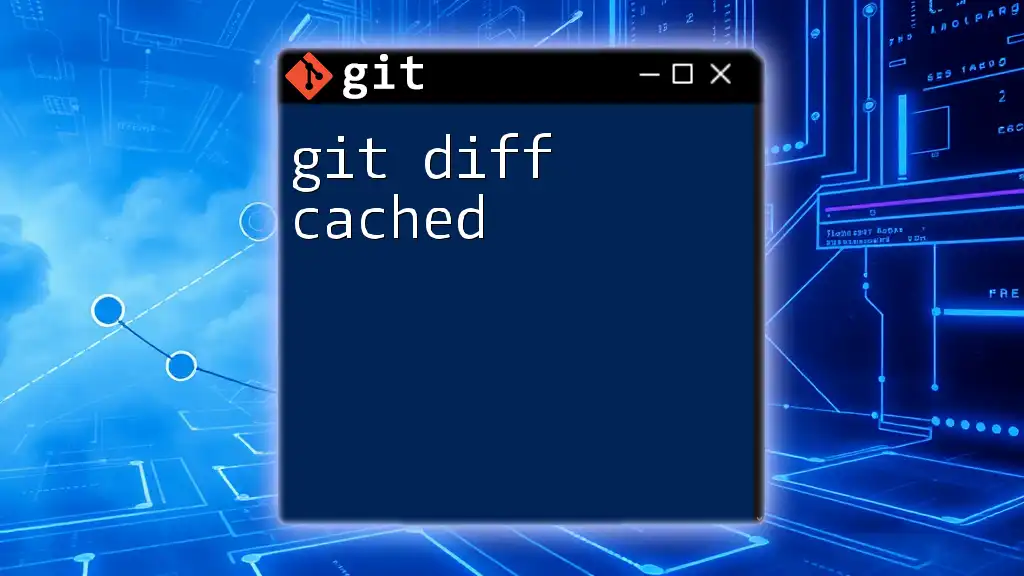
What Happens After Running the Command?
Effects on Your Working Directory
When you run the command `git rm -r --cached`, the files and folders specified are removed from the staging area (i.e., they are untracked), but remain in your working directory. You will still see these files on your local machine; they will just no longer be included in any future commits unless you add them back to the tracking.
Updating the Index
An internal aspect of Git you should understand is the index. After executing this command, the index is updated to reflect the removal of the specified files. This means that the next time you run `git status`, these files will no longer show up as tracked, and this clears space for new changes while allowing you to keep your local files as they are.
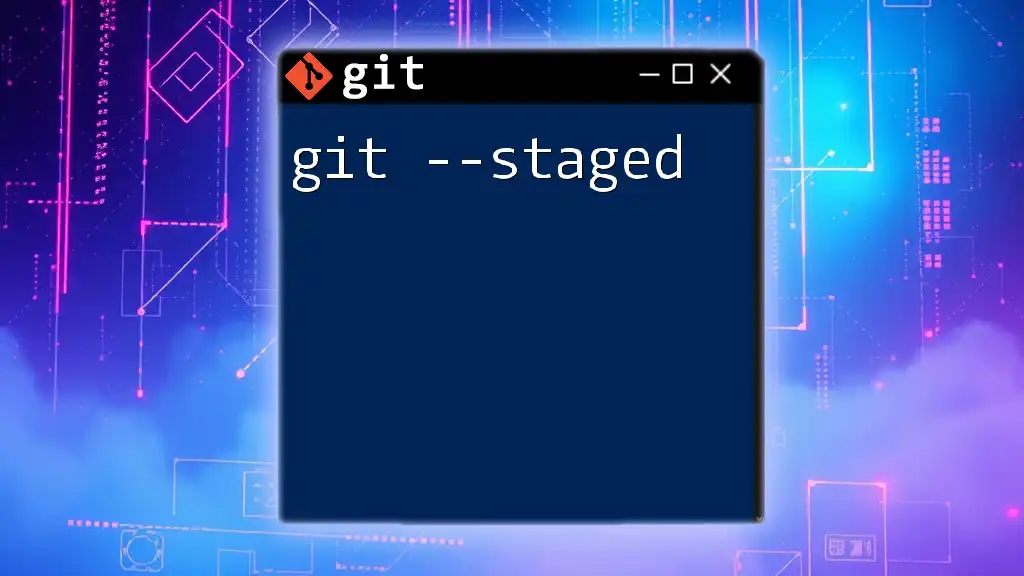
Common Mistakes & Troubleshooting
Running without Care
One common pitfall is executing this command without fully understanding its implications, especially in a shared repository. Always be cautious when removing files from the index, and consider creating a backup or branch before making significant changes. Removing files from the staging area can lead to confusion if collaborators expect those files to remain version-controlled.
Reverting Unintentional Changes
If you accidentally run `git rm -r --cached` and realize you still need the files tracked, don’t worry! You can easily recover them if you haven't yet committed the changes. Use the following command:
git checkout -- <file>
This command restores the file to its last committed state.
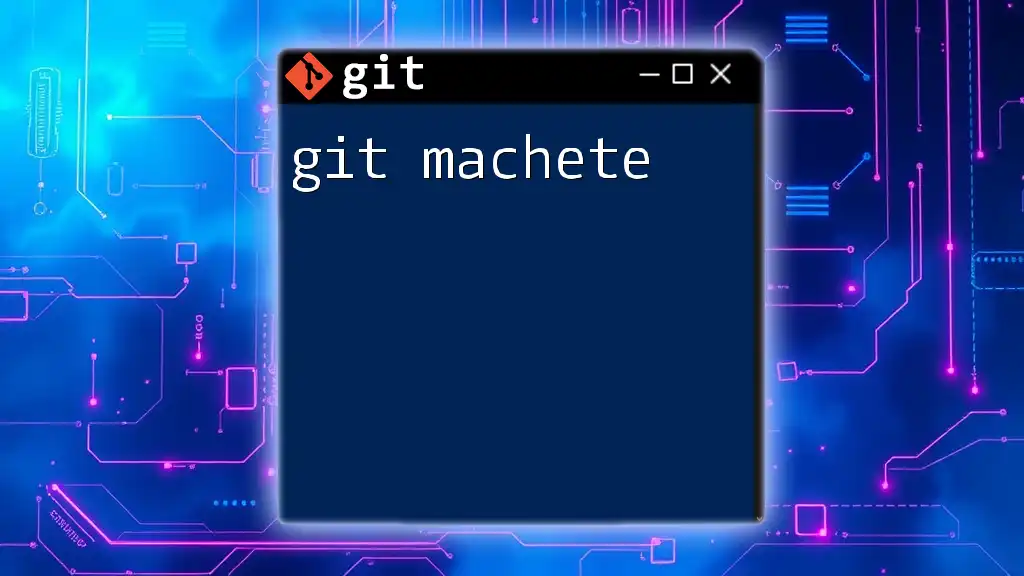
Best Practices for Using `git rm -r --cached`
When to Use This Command
This command is particularly effective when managing temporary files, large binaries, or sensitive configuration files that shouldn't be under version control. Use it when you determine that certain files clutter your staging area and should not be part of your project’s history.
Combining with `.gitignore`
To ensure that certain files or directories are never added to the version control again after using `git rm -r --cached`, consider using a `.gitignore` file.
Here’s an example of what you might add to your `.gitignore` file to prevent the `assets` folder from being tracked:
# .gitignore
/assets/*
This file will tell Git to ignore all files in the `assets` directory moving forward, helping you maintain a clean project.
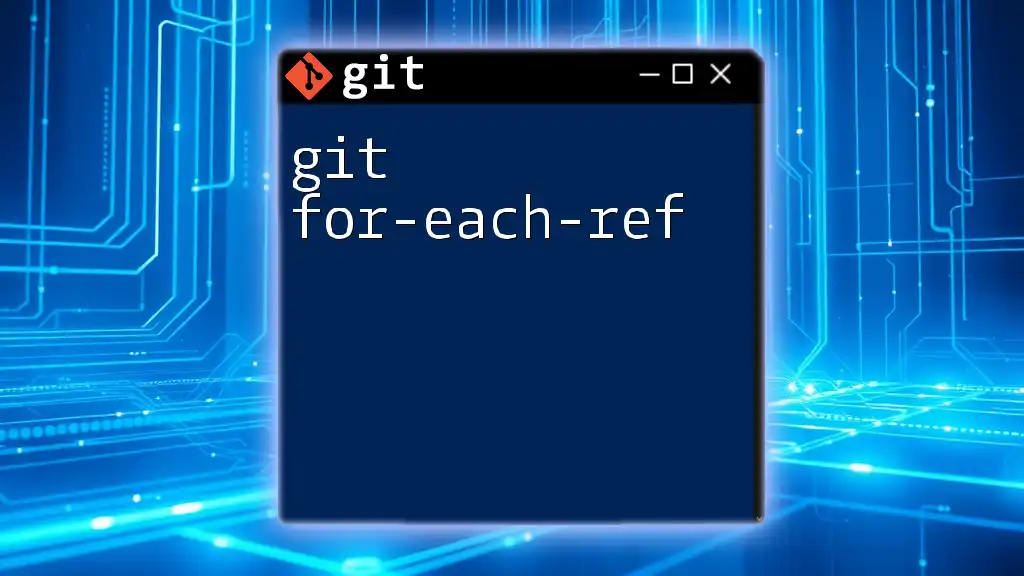
Conclusion
In summary, the command `git rm -r --cached` is a powerful tool for managing the files and directories in your Git projects. Understanding its implications allows for more strategic control over what is tracked in your repositories. Practicing these commands in a safe environment can enhance your version control skills and improve your project management efficiency.
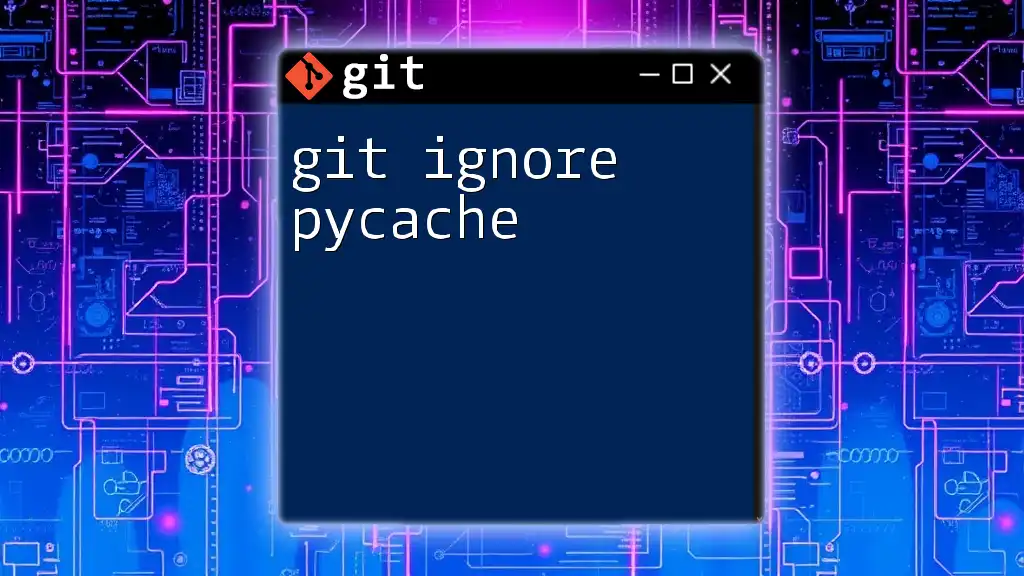
Additional Resources
For further learning, refer to the official Git documentation regarding `git rm` and other essential commands. Engaging with community forums or structured Git tutorials can also help solidify your understanding. Practicing in a sandbox environment like GitHub or GitLab will give you a space to experiment freely without the risk of affecting production code.
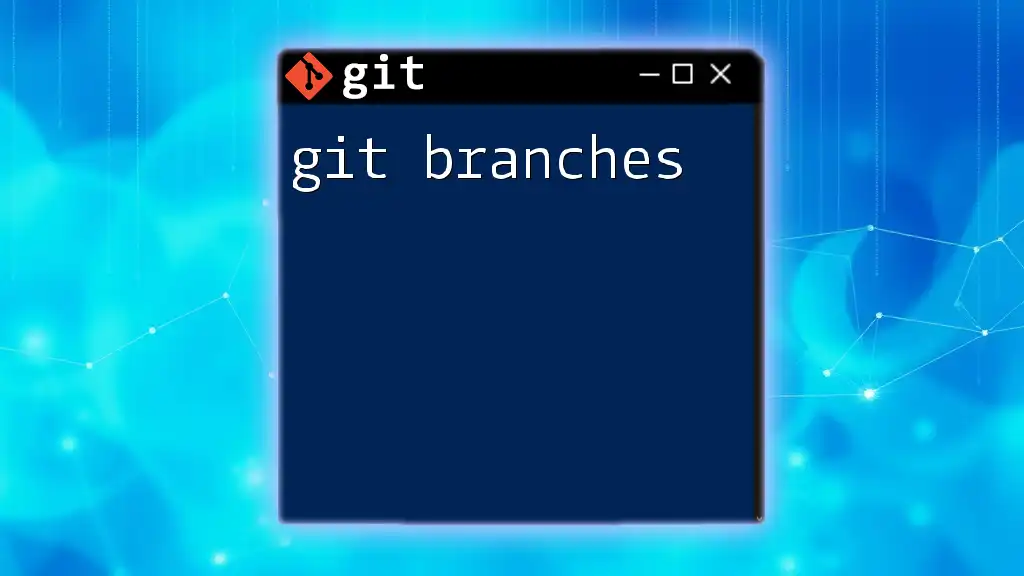
Call to Action
If you found this article helpful, be sure to subscribe for more tutorials on Git commands and best practices. We invite you to share your experiences or questions in the comments below!