The `git rm -rf` command is used to forcibly remove files or directories from both the working directory and the staging area in a Git repository.
git rm -rf path/to/directory_or_file
What is `git rm`?
Understanding the Command
The `git rm` command is a powerful tool in the Git version control system that allows you to remove files from both your working directory and the staging area. Unlike simply deleting a file from your filesystem, which doesn’t inform Git of the change, `git rm` not only deletes the specified files but also stages this deletion for the next commit, ensuring that your repository stays in sync with your intended changes.
The Role of `-r` and `-f` Flags
Recursive Deletion (`-r`)
The `-r` flag stands for recursive, which tells Git to remove directories and their contents. This is crucial when you want to delete an entire folder rather than just individual files. For instance, if you wanted to remove a directory named `old_project`, you would use:
git rm -r old_project
Force Deletion (`-f`)
The `-f` flag signifies force deletion. This is particularly useful when you are trying to remove files that are staged for commit. In Git, if a file is marked as changes to be committed, you may run into errors when attempting to remove it without this flag. For example, if you have modified a file named `example.txt` and you want to remove it forcefully, you can do so with:
git rm -f example.txt
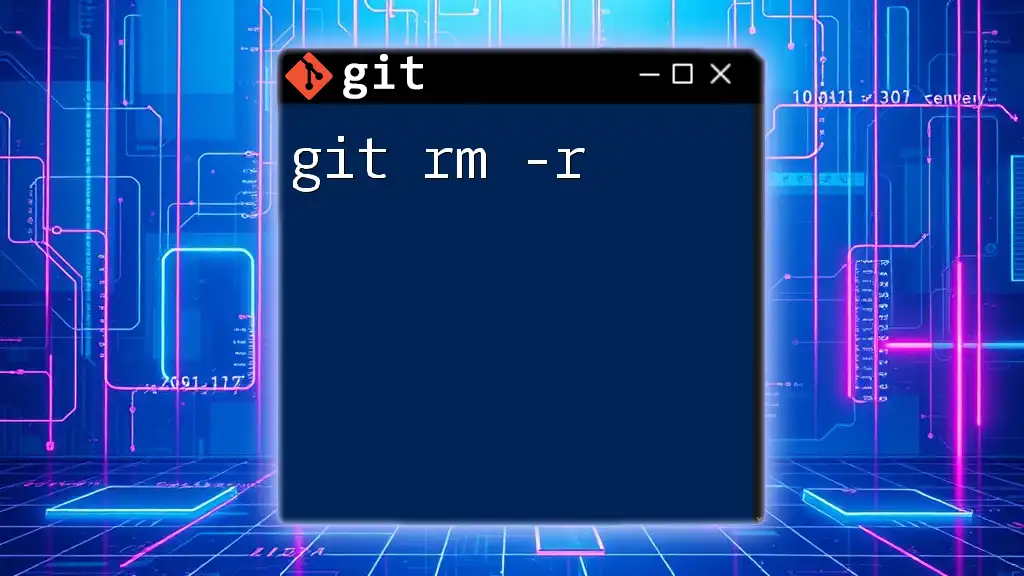
Detailed Breakdown of `git rm -rf`
Basic Syntax and Usage
The complete syntax for the `git rm -rf` command is:
git rm -rf <path>
Here, `<path>` refers to the file(s) or directory you wish to remove. It is vital to be precise about the path to avoid deleting unintended files.
Real-World Scenarios
Scenario 1: Removing Multiple Files
Suppose you have a series of `.log` files created during development, which you want to delete. You can invoke:
git rm -rf *.log
This command effectively removes all files ending with `.log` from both your working directory and staging area.
Scenario 2: Removing Directories
If your repository contains a directory named `temp` that you no longer need, you can delete it and its contents using:
git rm -rf temp
This removes everything inside `temp`, ensuring a clean working directory.
Practical Tips
Using `git rm -rf` can be quite handy, but it's essential to be aware of when to use it. This command is best suited for cleaning up files that are no longer needed or before starting fresh with a new version of your project. Just remember that when you execute this command, the changes must be committed to permanently affect your repository.
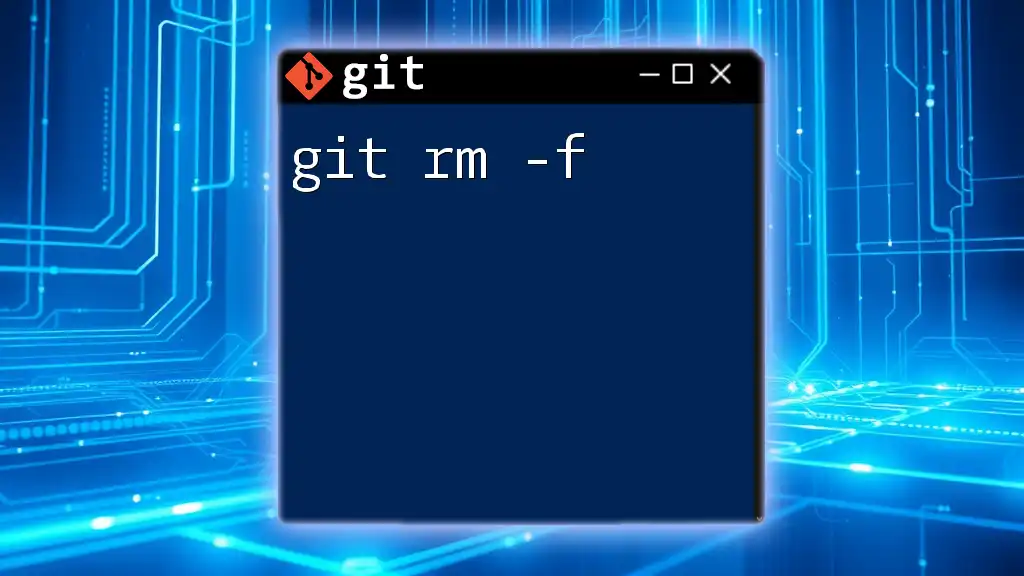
Safeguarding Your Work
Best Practices Before Running `git rm -rf`
Backup Important Files: Always take precautions by creating backups of vital files before running destructive commands. Using `git stash` to temporarily store changes or creating a backup branch can protect your work from accidental deletion.
Double-Checking the Path: Before executing the command, ensure that you verify the path you plan to remove. Using the `ls` command to list the contents of the directory can help confirm what's about to be deleted:
ls path/to/directory
Recovery Options
Sometimes mistakes happen, and files may be deleted unintentionally. Fortunately, if you realize shortly after executing `git rm -rf` that you've made a mistake, you can restore files by using the following command:
git checkout HEAD -- <path>
This command checks out the version of the file from the most recent commit, effectively restoring it.
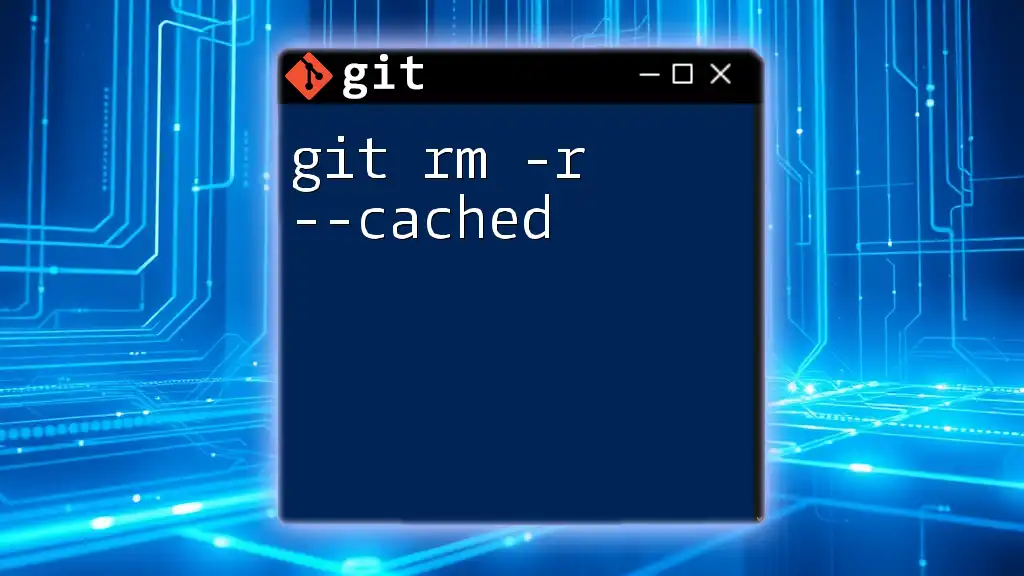
Common Pitfalls
Mistakes to Avoid
Accidental Deletions of Important Files: Due to the power of `git rm -rf`, it's easy to delete files you didn't mean to. A hasty command can lead to significant disruption in your project. Always ensure you review your intended changes before confirming deletions.
Forgetting to Stage and Commit Changes: After using `git rm -rf`, remember that your changes are still pending in the staging area. Forgetting to commit these changes means that the deletions will not be recorded in your repository's history. Always follow up with:
git commit -m "Removed unnecessary files"
Error Messages Explained
Users may encounter various errors when executing `git rm -rf`, such as "pathspec '...' did not match any files." This message generally indicates that the specified file or directory either doesn’t exist or the path was incorrectly typed. Always double-check your syntax and paths.
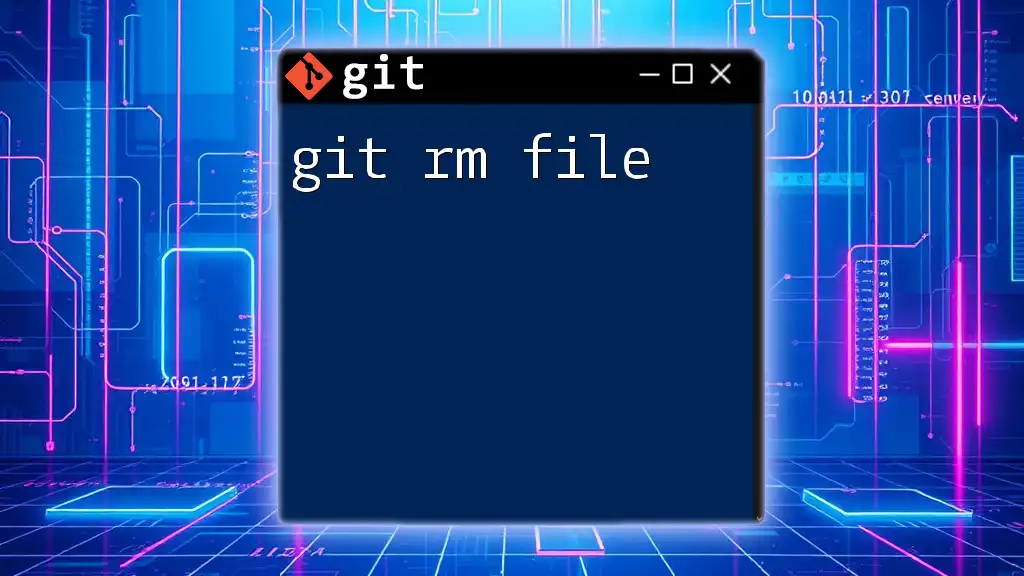
Conclusion
The `git rm -rf` command is an invaluable asset in maintaining a clean and efficient project structure. Understanding how to use it correctly, while being aware of the potential risks, allows you to manage your files effectively within Git. As with any potent tool, practice is essential; consider trying out this command in a safe testing environment to build your confidence. With these insights, you are now better equipped to harness the full potential of Git for your projects.
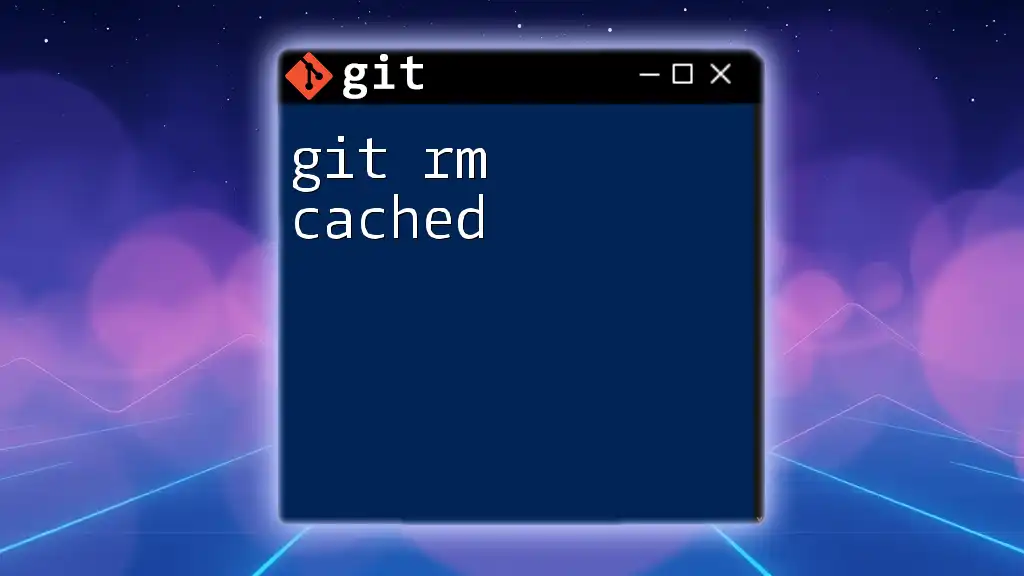
Additional Resources
For further reading, check out the official Git documentation and various online Git courses or tutorials to enhance your skills and understanding of version control. Keeping up-to-date with Git tools and best practices will ensure a smooth development process as you continue to build your repertoire as a Git user.