The `git rm -f` command is used to forcefully remove a file from the working directory and the staging area, even if it has been modified or is not tracked by Git.
git rm -f filename.txt
Understanding `git rm`
What is `git rm`?
`git rm` is a command used in Git to remove files from the working directory and the staging area. When executed, it effectively tells Git that you no longer want to track a file's changes. It’s important to note that the removal process occurs not only from your local directory but also prepares the change to be recorded in your next commit.
Why use `git rm`?
There are various scenarios where you might need to remove files from your Git repository. These can include:
- Removing obsolete files that are no longer needed.
- Fixing mistakes such as adding the wrong file to the repository.
- Organizational purposes, like cleaning up a project and maintaining efficient structure.
It's crucial to differentiate `git rm` from merely ignoring a file. While `git rm` deletes a file that is tracked by Git, you can prevent Git from tracking a file by using the `.gitignore` file, which specifies files to be excluded from the repository.
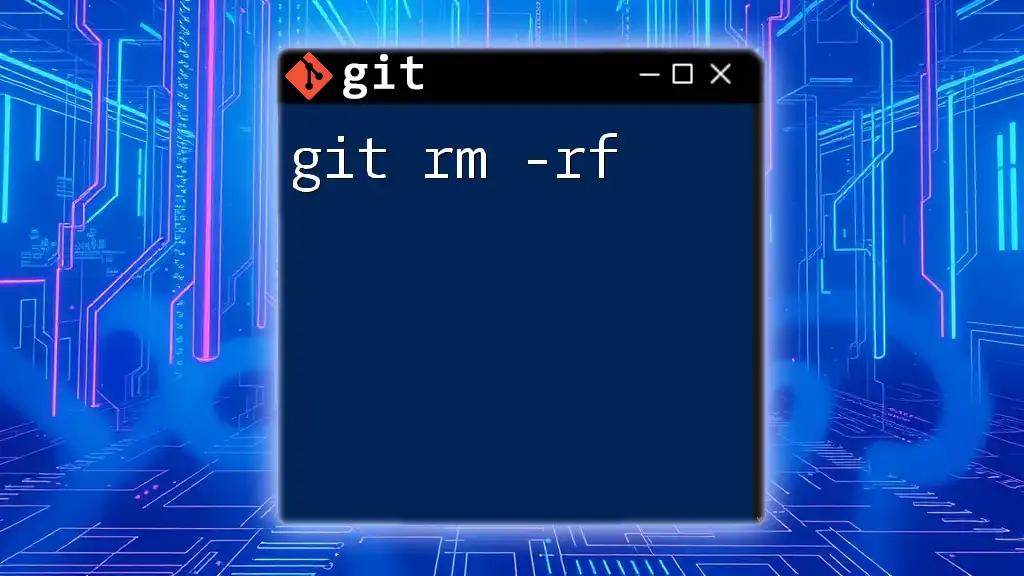
The `-f` Flag Explained
What does `-f` stand for?
The `-f` flag in the `git rm` command stands for force. This flag is particularly useful in situations where Git would otherwise prevent a file from being deleted. For example, if changes to a file have been made but not staged, Git will block removal to prevent unintended data loss.
When to use `git rm -f`?
Using `git rm -f` is appropriate when you are sure you want to delete a file, despite any uncommitted changes. It is a powerful option that, while convenient, opens the door to possible issues, such as accidental deletions. Always proceed with caution by double-checking the files you plan to remove.
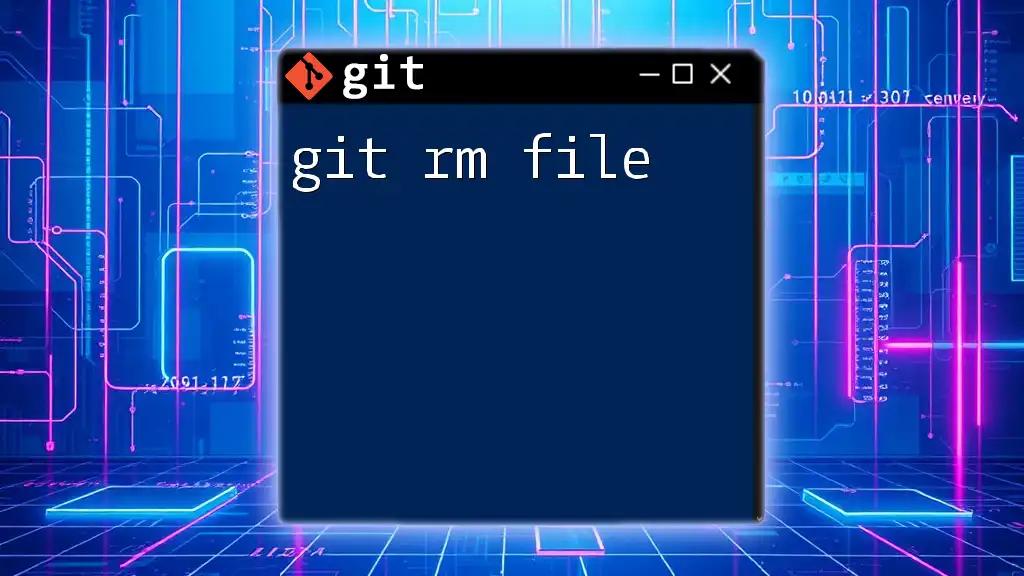
Syntax of `git rm -f`
Basic Syntax
The basic syntax for the `git rm -f` command is as follows:
git rm -f <file>
Here, `<file>` represents the name of the file you want to remove.
Usage with multiple files
You can also remove multiple files in one command. For instance:
git rm -f file1.txt file2.txt
This command will forcefully remove both `file1.txt` and `file2.txt` from your repository.
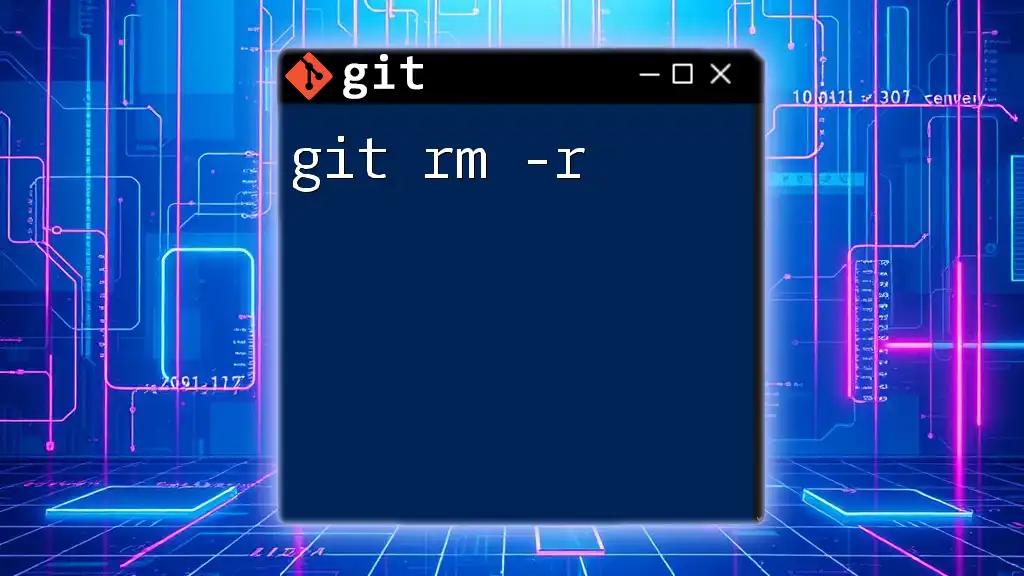
Practical Examples
Removing a tracked file
To remove a tracked file, you would typically follow these steps. Consider the case where you want to remove a file named `unwanted-file.txt`. You can execute:
git rm -f unwanted-file.txt
git commit -m "Remove unwanted file"
This example shows how to both remove the file from your project and register the change in your version history with a commit.
Removing files not in the staging area
Sometimes you may want to remove files that haven't been staged. First, it can be helpful to check the current status of your repository using:
git status
With the output indicating that `file-not-in-staging.txt` needs to be removed, you can use the forceful command like this:
git rm -f file-not-in-staging.txt
git commit -m "Force remove file from repo"
This ensures the file is deleted from your working directory as well as the tracking history in Git.
Using in combination with other commands
The `git rm -f` command can be combined with other Git commands. For example, if you want to remove an old version of a file and commit that change in one line, you can use:
git rm -f old-version.txt && git commit -m "Force removed older version"
This single line removes the file and commits the change, keeping your workflow efficient.
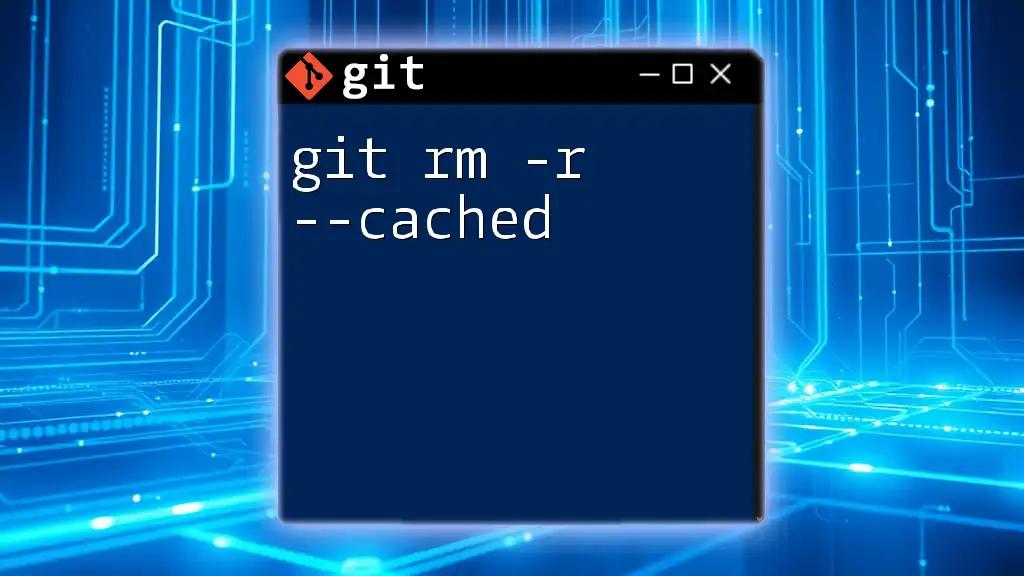
Handling Errors
Common Error Messages
Users may encounter several common errors when using `git rm -f`. For instance, if you attempt to remove a file that does not exist or has already been deleted, Git will return an error message.
How to troubleshoot these errors
To troubleshoot, the first step is to check the status of your files with:
git status
This will give you insight into what is currently tracked in your repository. If you accidentally remove a file, use Git's reflog or checkout commands to recover it.
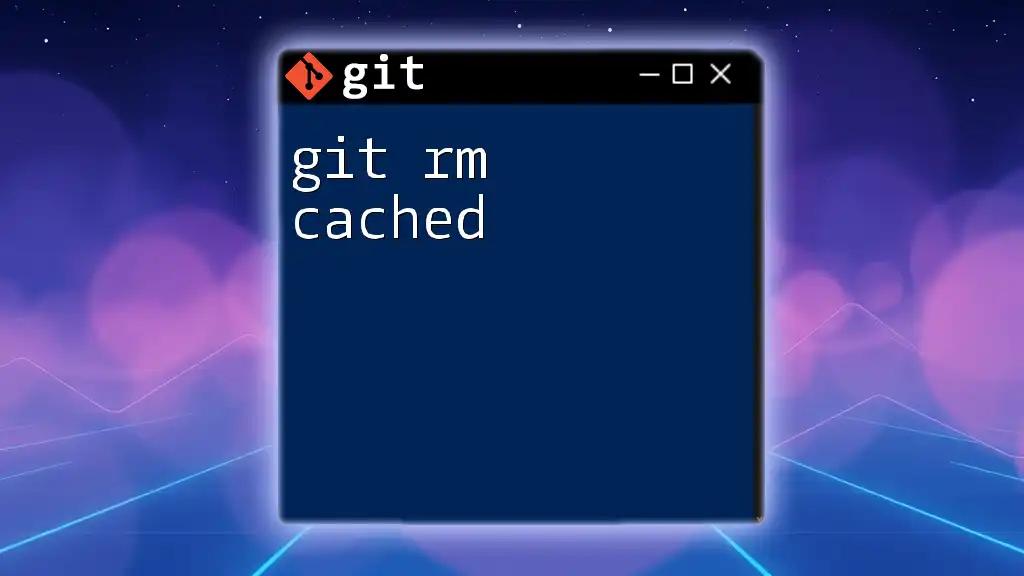
Best Practices
When to avoid using `-f`
Avoid using the `-f` flag if you are uncertain about the data. Situations where you might be hesitant, such as files with significant uncommitted changes or files that are critical to your project should warrant a pause before forcing their removal.
Confirming File Removal
After executing a `git rm -f` command, it is wise to confirm that the file has been effectively removed. You can check your current repository status by running:
git status
This will reflect the changes, indicating that the file is no longer being tracked.
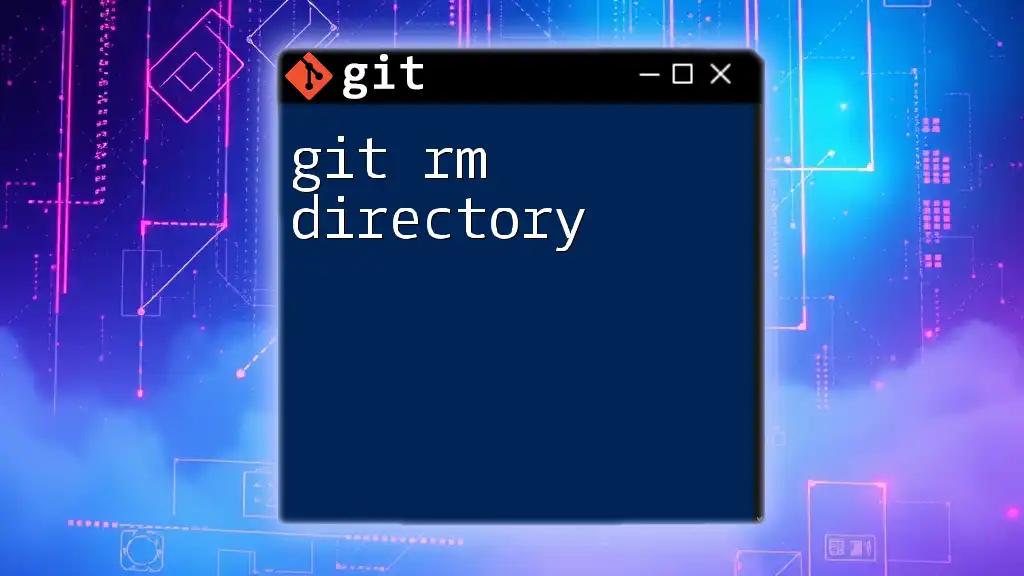
Conclusion
In summary, the `git rm -f` command is an essential tool in Git for removing files with confidence and precision. It’s widely useful for developers who need to manage their codebase effectively. As with all powerful commands, it should be used with care and consideration to avoid unintended data loss.
To enhance your proficiency, practice using `git rm -f` in a safe environment and explore other Git commands to become an adept version control user. Familiarizing yourself with Git’s functionalities can greatly improve your development workflow and code management strategies.
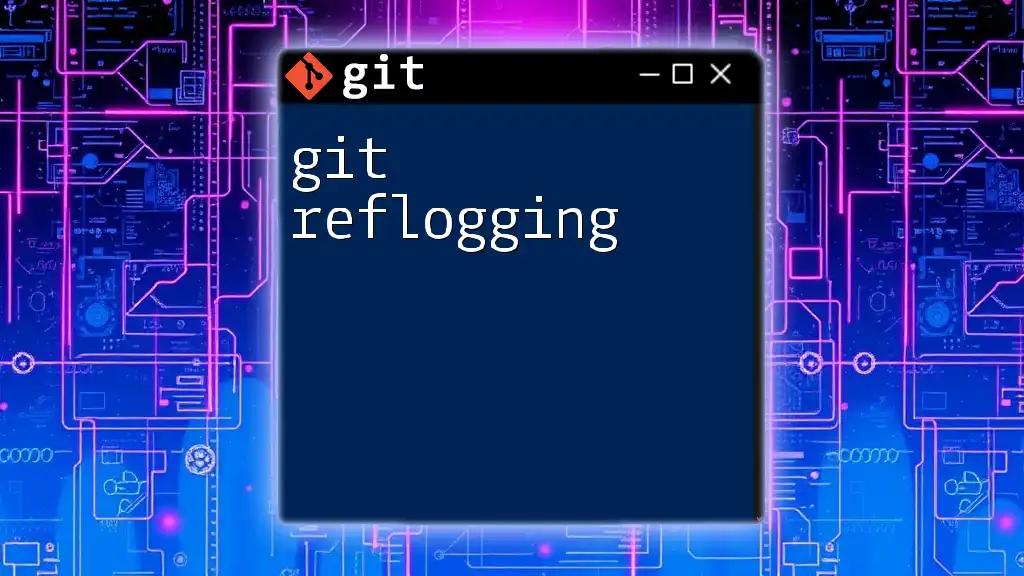
Additional Resources
For further learning, consider referring to the official Git documentation, utilizing Git command cheat sheets, or exploring platforms dedicated to Git training. Engaging with these resources can offer deeper insights into all aspects of Git, enhancing your skills and troubleshooting capabilities.