In Git, a "ref" is a pointer to a commit object, typically used to reference branches, tags, and other identifiers within the repository.
Here's an example of how to list all refs in a Git repository:
git show-ref
Understanding Git Refs
What are References (Refs)?
In Git, a ref is a pointer to a specific commit in your project’s history. It plays a crucial role in helping developers track and manage different points of development in a project. Refs can direct to branches, tags, and even to specific commits, enabling seamless navigation between the various states of your codebase.
Different Types of Refs
- Branches: These are the primary method for working on different features or fixes simultaneously. Each branch represents a distinct line of development.
- Tags: Tags are used to mark specific points in your repository’s history, typically used for releases. They serve as a snapshot of your code at certain milestones.
- HEAD: This is a special reference that points to the current commit in your working directory, indicating which branch you are currently working on.
Anatomy of a Git Ref
A Git ref consists of a reference name that points to a specific commit by its SHA-1 hash. The structure looks like this:
refs/heads/branch-name
In this structure, `refs/heads/` signifies that it refers to a branch. By having a ref, Git quickly retrieves the commit pointed to by that branch. When you make changes and commit them, Git updates the ref to point to the newly created commit.
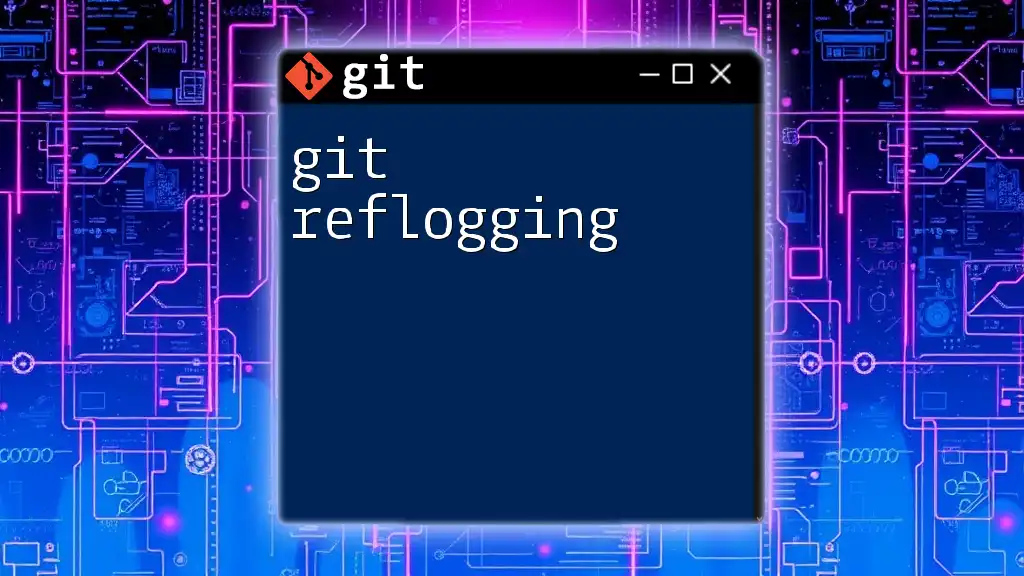
Types of Git Refs
Branches
Branches enable you to work on features and fixes without disrupting the main line of development.
Creating a Branch: To create a new branch, you can use the following command:
git branch <branch-name>
Switching Branches: Once the branch is created, you can switch to it using:
git checkout <branch-name>
Best Practices for Using Branches:
- Keep your branch names meaningful to give context to other developers.
- Regularly merge back changes to avoid large merge conflicts later.
- Delete merged branches to maintain a clean repository.
Tags
Tags are primarily used for marking releases. Unlike branches, tags do not change as new commits are made.
Creating a Lightweight Tag: You can create a simple tag with:
git tag <tag-name>
Creating an Annotated Tag: To add metadata, such as the tagger's name and date, you can create an annotated tag with:
git tag -a <tag-name> -m "Tag message"
Viewing Tags: To see all available tags, run:
git tag
Deleting Tags: If you need to remove a tag, you can do so with:
git tag -d <tag-name>
HEAD
HEAD is a reference that points to your current working location in the repository. It typically points to the latest commit on your currently checked-out branch.
Viewing the Current HEAD: To determine which commit your HEAD is pointing to, use:
git rev-parse HEAD
Understanding Detached HEAD State: If you check out a specific commit rather than a branch, you enter a "detached HEAD" state. In this mode, any new commits will not be associated with any branch unless you create a new branch from that state.
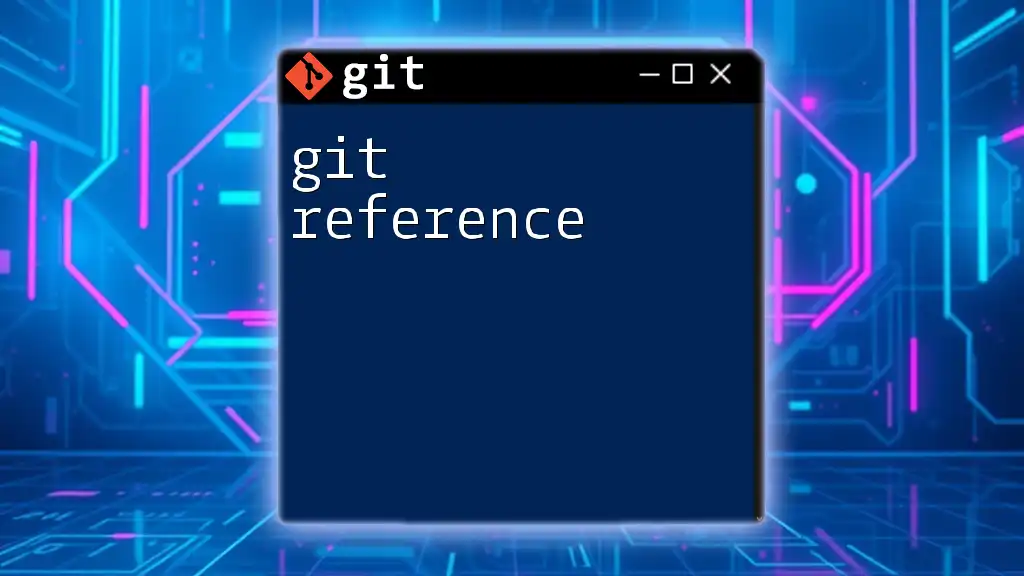
Using Git Refs Effectively
Listing Refs
To see all the refs in your repository, you can use:
git show-ref
This command lists the refs along with their corresponding commit SHA-1 hashes. Understanding this list is essential for tracking which commits your branches or tags point to.
Ref Patterns and Navigation
Ref Patterns: You can utilize ref patterns to make navigation simpler. For example, instead of using the full name `refs/heads/master`, you can simply use `master`.
Relative References: Understanding relative references is vital for various Git operations. For instance, `HEAD^` refers to the previous commit before your current HEAD, while `HEAD~1` is equivalent. These shortcuts enable easier navigation through your commit history.
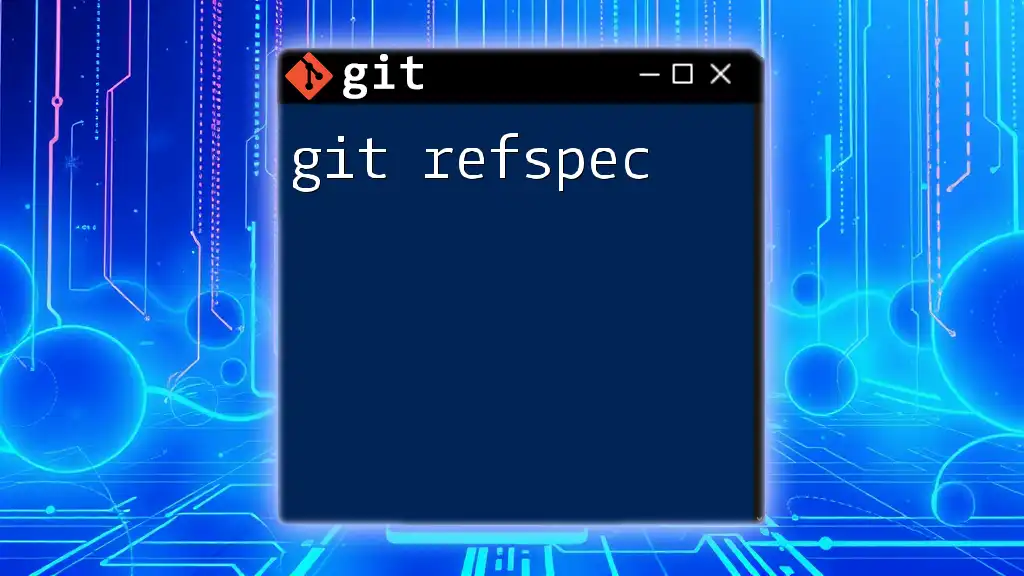
Common Issues with Git Refs
Conflicts and Merge Issues
It’s common to encounter merge conflicts when working with branches. Refs can sometimes lead to these conflicts, especially when multiple developers work on the same code section simultaneously.
Resolving Conflicts: When a conflict arises, Git will provide guidance on which files conflict and where. It’s essential to address these issues cautiously, ensuring that you understand the code’s intentions before resolving the discrepancy.
git merge <branch-name>
Before merging, always ensure your working directory is clean to avoid complications.
Tracking Remote Refs
Understanding the distinction between local and remote refs is crucial in a collaborative environment. When working with a team, remote refs need to be clear to maintain synchronization.
To keep your local refs up to date with remote branches, frequently fetch updates using:
git fetch
git branch -r
This practice ensures you are aware of any changes made by teammates on the remote repository.
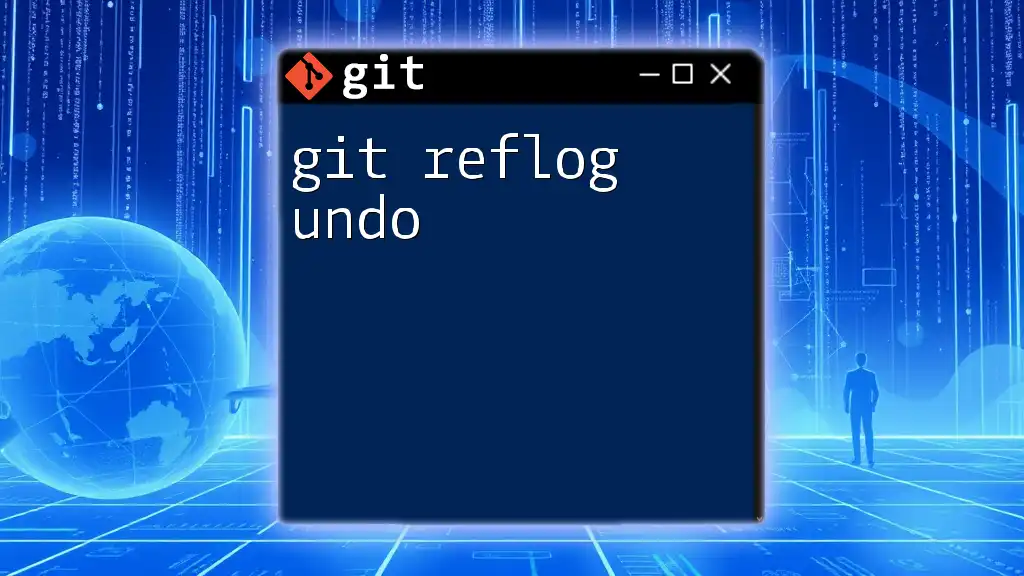
Conclusion
In this guide, we discussed the fundamentals of git refs, which serve as essential components in managing your repository's history. From branches to tags and the significance of HEAD, understanding refs empowers you to leverage the full capabilities of Git effectively. Explore and practice the commands covered, as mastering these concepts will enhance your version control skills.
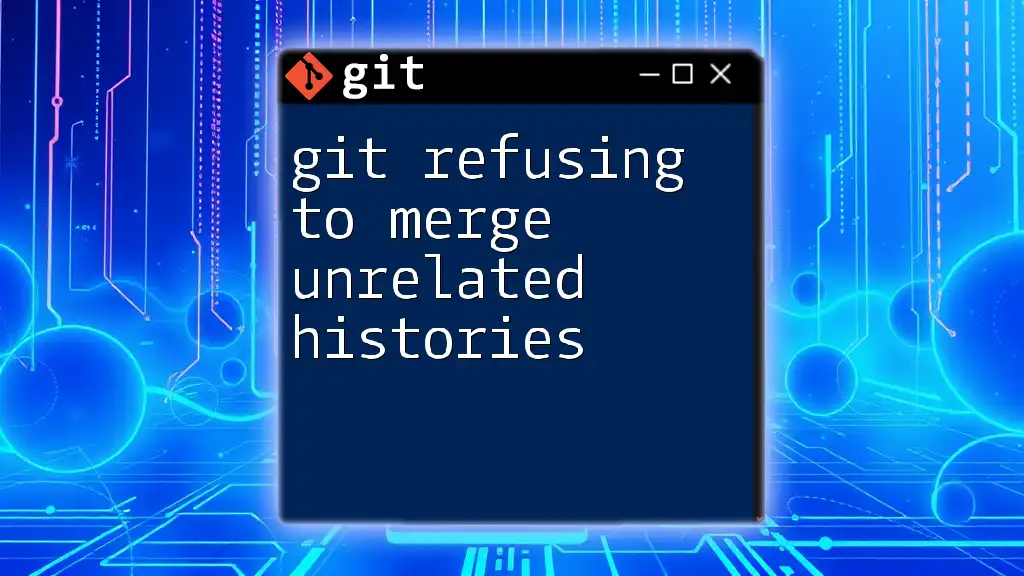
FAQs
What is a ref in Git?
A ref is a pointer to a specific commit in your repository’s history, allowing you to navigate between branches, tags, and commits.
How do I create a new branch?
You can create a new branch using `git branch <branch-name>` and switch to it with `git checkout <branch-name>`.
What is the difference between a tag and a branch?
Tags are fixed points in history used to mark releases, while branches represent ongoing lines of development that can change with new commits.
What happens if I delete a ref?
Deleting a branch means you lose that line of development, while deleting a tag removes the snapshot reference, although the commits themselves remain intact.