The `git review` command is used to submit changes from your local branch to a corresponding code review system, often integrated with platforms like Gerrit.
Here's a simple example of how you might use it:
git review
What is Git Review?
Git Review refers to the structured process of evaluating and providing feedback on code changes made in a Git repository. Conducting a code review is essential in software development, as it ensures code quality, promotes collaboration, and facilitates knowledge sharing among team members. By leveraging Git's features, teams can efficiently manage their code review workflow.
Concept of Code Review
Code review is the practice of systematically examining code written by other developers. This process aims to identify errors, improve code quality, and enforce coding standards. Consider the following benefits of conducting code reviews:
- Improved Code Quality: Peer scrutiny often uncovers issues that the original author might overlook.
- Knowledge Sharing: Team members can learn from each other, which enhances the overall skill set within the team.
- Consistency in Code: Code reviews help maintain uniform coding standards across the project.
How Git Enhances the Review Process
Git enhances the review process through its powerful version control capabilities. With Git, developers can easily track changes, revert to previous versions, and collaborate on code through branches. The collaboration features enable teams to merge contributions seamlessly while keeping the main codebase stable.
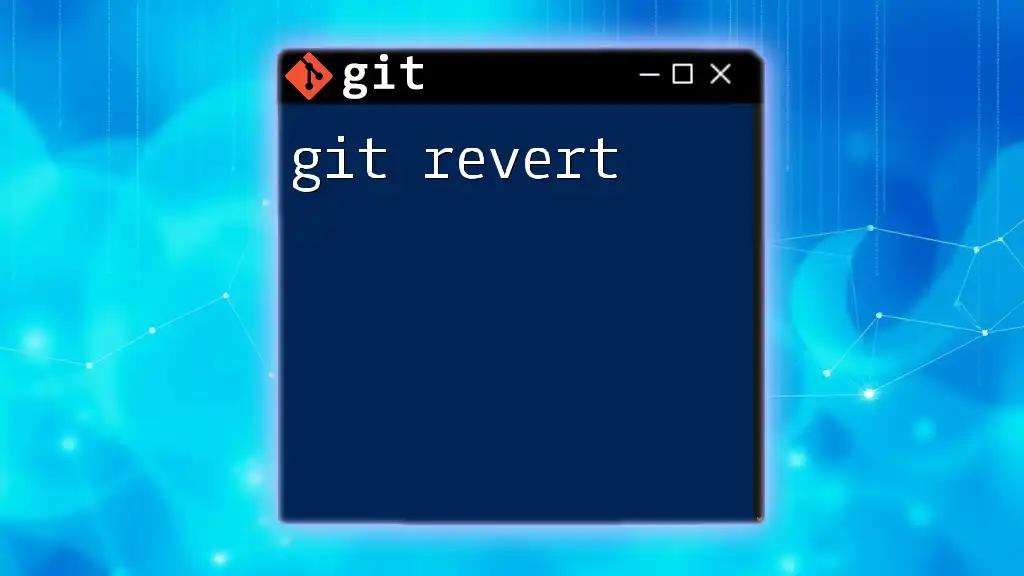
Setting Up a Git Review Environment
Prerequisites for a Git Review
Before diving into Git review, ensure you have a proper environment set up. This includes:
-
Installing Git: Depending on your operating system, you can install Git by following these steps:
- Windows: Download the installer from the [official Git website](https://git-scm.com/downloads) and run it.
- macOS: Install via Homebrew by running the command:
brew install git
- Linux: Use your distribution's package manager, for example, on Ubuntu, use:
sudo apt install git
-
Creating a Git Hosting Account: Choose a platform such as GitHub, GitLab, or Bitbucket and set up your account.
Creating a Repository for Review
Once Git is installed and your hosting account is ready, it's time to create a repository:
-
Initialize a new repository using:
git init
-
Alternatively, clone an existing repository using this command:
git clone <repository-url>
This command creates a local copy of the repository, allowing you to start contributing immediately.
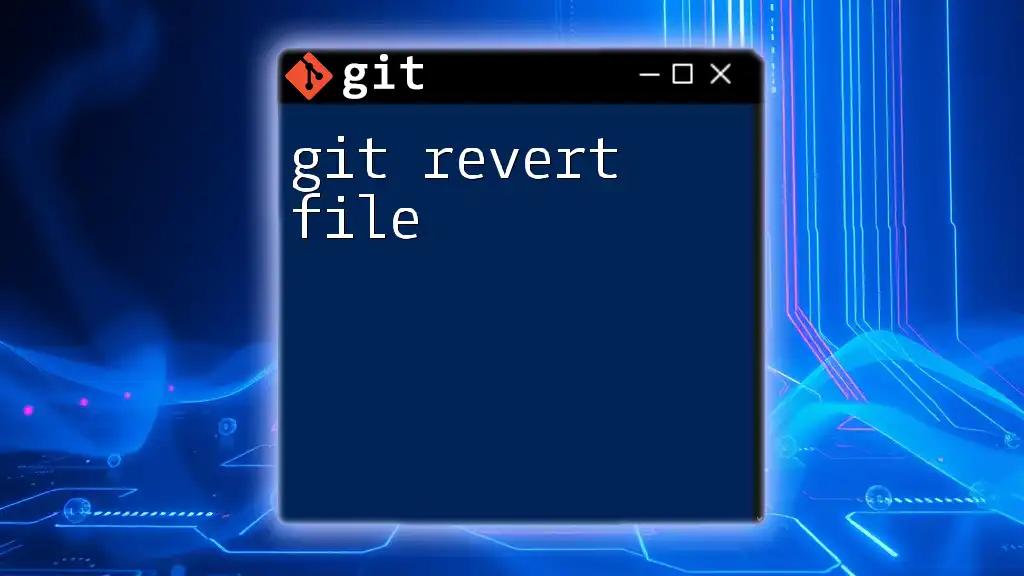
Git Review Workflow
Creating a Feature Branch
To facilitate an effective git review process, it’s critical to create feature branches for specific changes. This keeps different functionalities separate and helps prevent conflicts.
To create a new branch, use:
git branch <feature-branch-name>
Switch to your new branch using:
git checkout <feature-branch-name>
Or, you can combine these two steps with:
git checkout -b <feature-branch-name>
Branches help in isolating your development work from the main codebase, thereby simplifying the review process.
Making Changes to Your Code
When making changes, adhere to coding standards and keep your commits concise and meaningful. Use descriptive commit messages to explain the purpose of the changes. To commit changes, use:
git commit -m "A brief description of the changes"
For additional suggestions, you might consider using the `--amend` flag for making adjustments to your last commit:
git commit --amend -m "Updated commit message"
Effective commit messages are critical for reviewers to understand the intent behind changes.
Pushing Branches for Review
Once your changes are ready, you’ll need to push your branch to the remote repository to initiate the review process. Use:
git push origin <feature-branch-name>
Make sure your remote repository is set up correctly. You might need to tweak visibility settings to ensure the appropriate team members have access to the pull request.
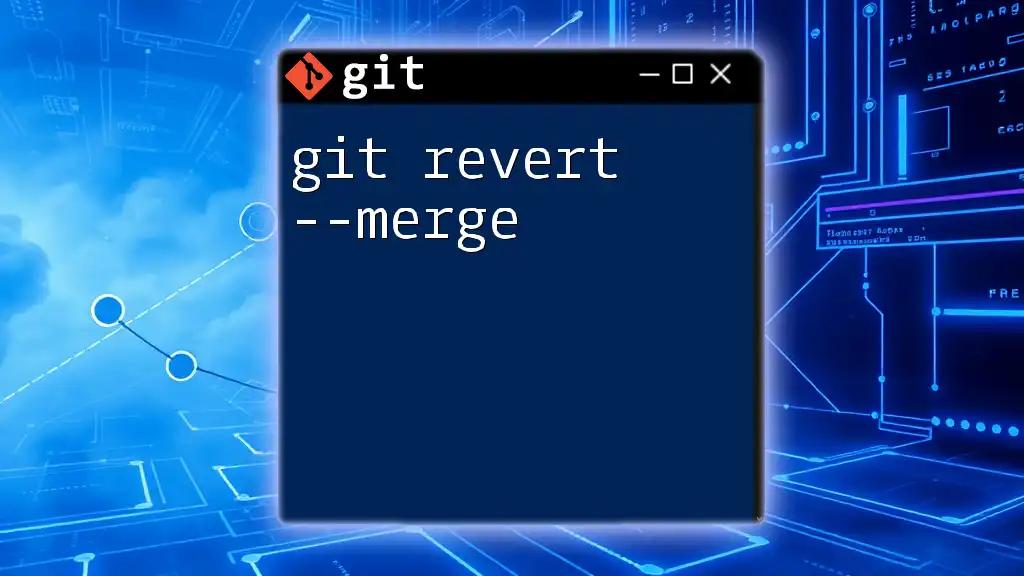
Conducting a Git Review
Pull Requests Explained
A Pull Request (PR) is a request to merge your changes from your feature branch into the main branch. This serves as the focal point for discussion about the changes.
Creating a PR typically involves navigating to your repository on your hosting platform and selecting the option for "Create Pull Request." Be sure to provide a compelling description of the changes for clarity.
Reviewing Code in a Pull Request
During the review phase, reviewers can inspect the changes directly. Most platforms provide features such as clickable diffs, inline comments, and code suggestions. Reviewers should focus on aspects like:
- Code quality and style
- Function and variable naming
- Test coverage and performance considerations
Providing effective feedback is crucial for the improvement of code quality.
Addressing Review Comments
Once feedback is received, it’s essential to iterate on the code. Resolve comments by making changes in your local repository, and push your updates again. For example, if you need to amend previous commits, ensure you follow the best practices to keep the history clean.
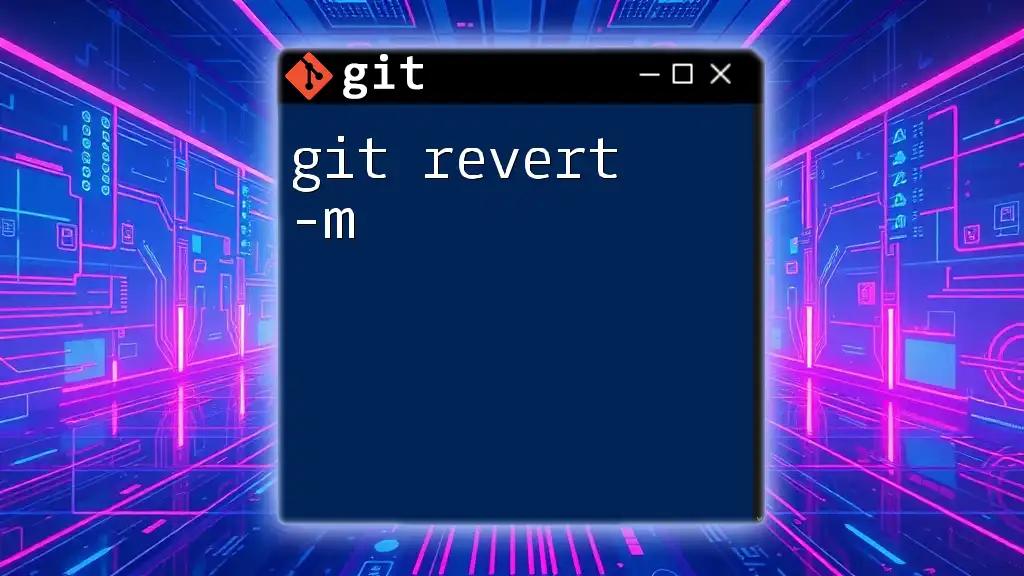
Advanced Git Review Practices
Squashing Commits
Squashing commits allows you to combine multiple commit entries into a single commit, keeping the commit history cleaner. This is particularly useful for feature branches where multiple small commits have been made.
You can squash commits through interactive rebasing with:
git rebase -i HEAD~<number-of-commits>
Follow the on-screen instructions to mark commits for squashing. This practice makes the final PR easier to read and review.
Using Git Hooks for Custom Reviews
Git hooks are scripts that execute automatically at certain points in Git's lifecycle. For example, a pre-commit hook can run automatic checks before a commit is finalized. You can set up a simple pre-commit hook to enforce code linting:
Navigate to the hooks directory of your repository:
cd .git/hooks
Create a file named `pre-commit` and write your script in it. Don't forget to make it executable:
chmod +x pre-commit
This ensures that your code meets certain standards before it's committed to the repository.
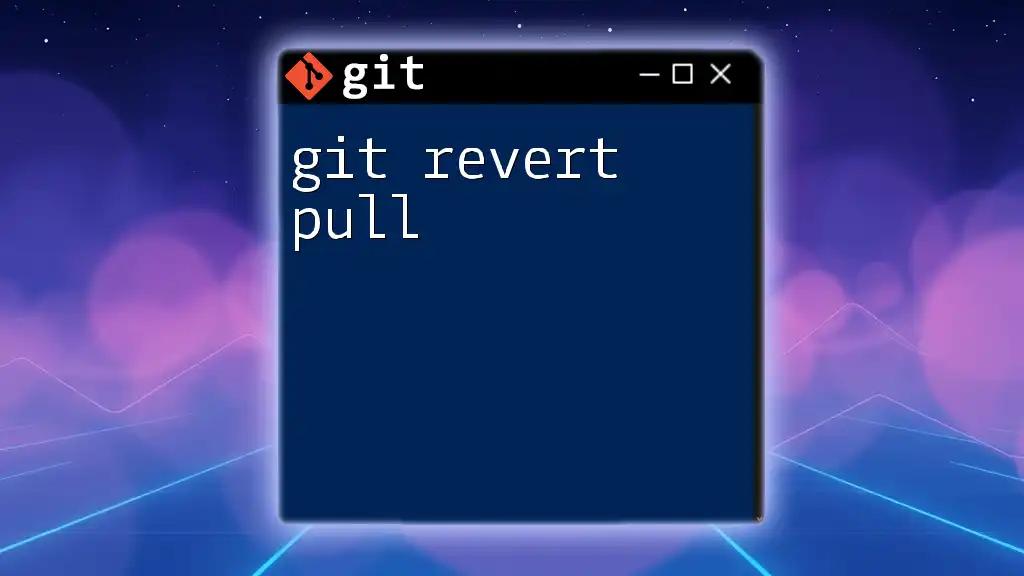
Tools and Resources for Git Review
Popular Code Review Tools
Various platforms offer unique features for managing git reviews effectively. Here’s a brief overview:
- GitHub: Robust PR system with inline commenting, review branching, and CI/CD integration.
- GitLab: Comprehensive tools for project management with merge requests, code quality checks, and more.
- Bitbucket: Support for PRs and integration with Jira for enhanced project tracking.
Additional Learning Resources
To deepen your understanding of Git review practices, consider exploring the following materials:
- Recommended books on Git fundamentals.
- Online courses that cover Git workflows and best practices.
- The official Git documentation for in-depth technical details.
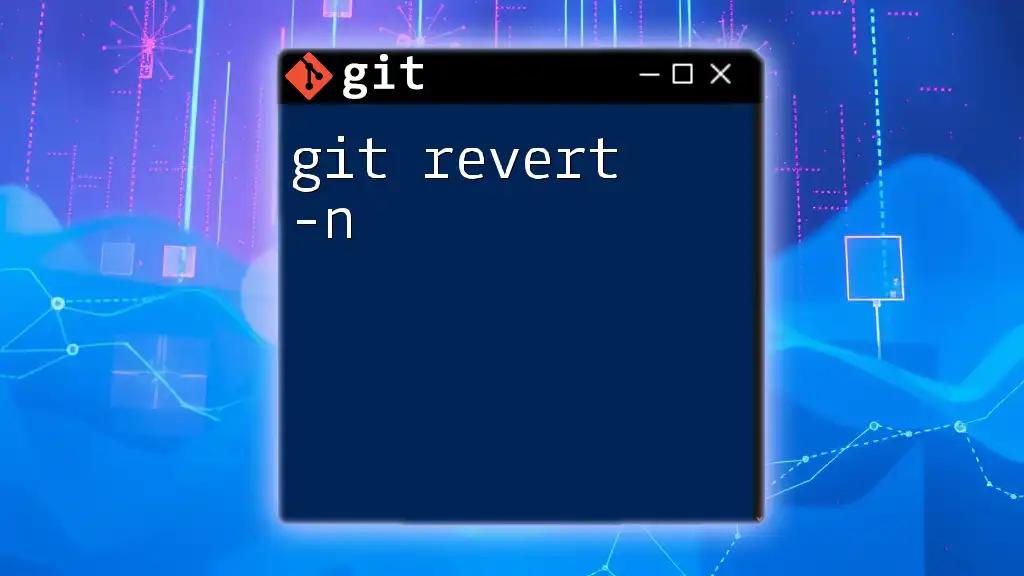
Common Mistakes to Avoid During Git Review
While engaging in git review, avoid these common pitfalls:
- Neglecting to Write Descriptive Commit Messages: Clarity in commit history aids reviewers and future developers.
- Ignoring Feedback from Reviewers: Treat reviews as learning opportunities to enhance your coding skills.
- Failing to Test Changes Before Pull Request: Rigorous testing ensures you submit code that functions as expected, reducing the back-and-forth during reviews.
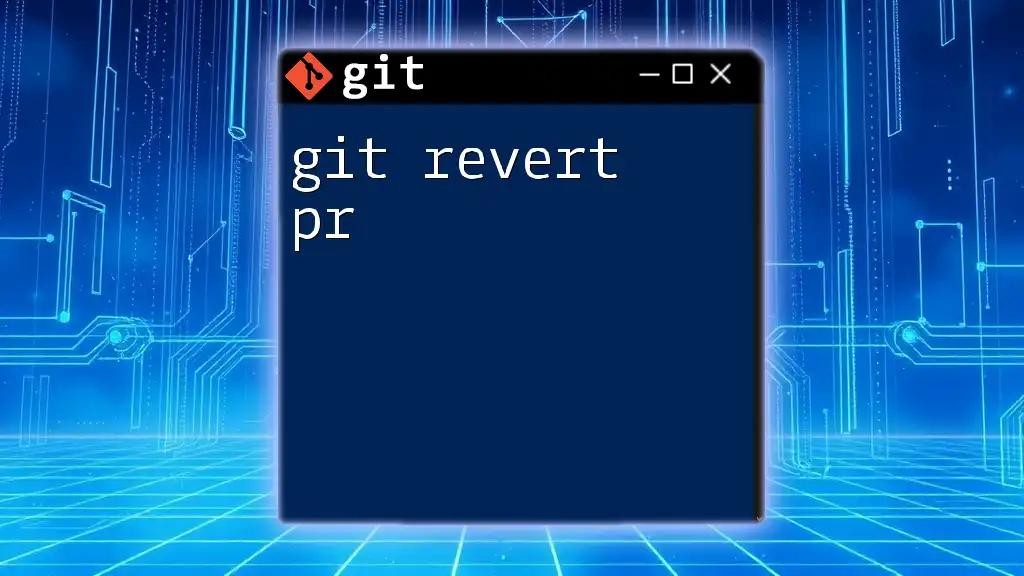
Conclusion
Incorporating a well-structured git review process can significantly improve code quality and team collaboration. By understanding and utilizing Git's powerful features, developers can streamline their workflow, enhance communication, and foster an environment of continuous improvement. Embrace this opportunity for growth and implement effective git review practices in your projects!
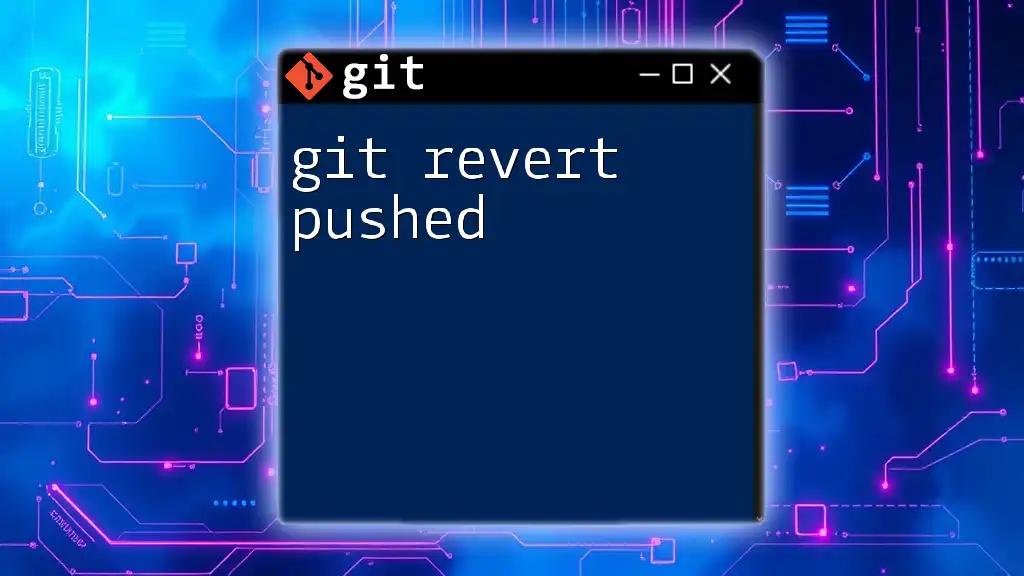
FAQs about Git Review
Q: How long should code reviews take?
A: The duration of code reviews can vary. However, aim for reviews that are concise and focused, usually not exceeding a couple of hours or days, depending on the complexity of the changes.
Q: Can I review my own code?
A: While self-reviewing is beneficial, having another developer review your code provides a fresh perspective and can catch issues you may have missed.
Q: What if disagreements arise during a review?
A: Foster open discussions to come to a consensus. Aim for constructive dialogue centered around code quality and best practices.
By following these guidelines, you'll be well-prepared to introduce git review into your development routine effectively!