In Git, a rebase is not a restore; rather, it is a process that allows you to apply your changes onto a different base commit, effectively rewriting your project’s history.
git rebase <base-commit>
What is Rebase in Git?
Understanding Git Rebase
Rebasing in Git is a method of integrating changes from one branch into another. Essentially, it allows you to move or combine a sequence of commits to a new base commit. This is particularly useful for maintaining a clean and linear commit history, making it easier for collaborators to understand the project's evolution.
Key Features of Rebase
One of the main advantages of rebasing is its ability to create a linear commit history, which enhances clarity. Unlike a merge which results in a branching history, rebasing integrates the changes linearly, making it appear as though changes were made in a single stream.
Another key feature involves conflict resolution. When you rebase, if there are conflicts between the two branches, you will be prompted to resolve them one at a time, ensuring you can handle each conflict carefully.
In contrast to merging, which combines the histories of both branches, rebasing rewrites the commit history. This changes the commit hashes, which is a critical element to understand when using rebase.
Example of a Git Rebase
To illustrate how to use rebase, consider the following basic rebase command:
git checkout feature-branch
git rebase main
Here, `feature-branch` is the branch you're working on, and `main` is the branch you want to rebase onto. By executing these commands, Git will replay the changes made in `feature-branch` on top of the commits on `main`, allowing you to keep your branch updated with the latest changes in the mainline.
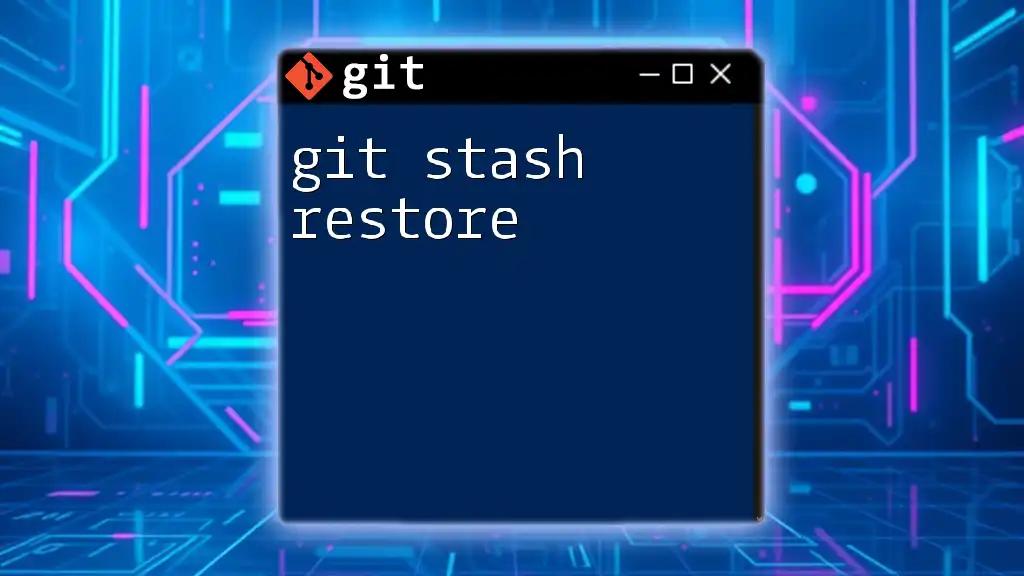
When to Use Rebase
Use Cases for Rebase
You should consider using rebase when you want to keep your feature branches up to date with the main branch. By regularly rebasing, you minimize the chances of running into significant conflicts when it's time to merge your changes back into the main branch.
Another typical use case is to streamline your commit history. A clear and orderly commit history can significantly enhance the project’s maintainability and makes the review process smoother for your team.
Risks of Using Rebase
While rebasing is a powerful tool, it is not without risks. One major concern is the danger of history rewriting. If you rebase commits that have already been pushed to a shared repository, it can create confusion among team members as it alters the project history. It’s advisable to only rebase local branches that haven’t been pushed or shared yet.
Additionally, there are scenarios where using rebasing can lead to instability or data loss, especially when resolving conflicts improperly. Always ensure you have backups or check if the changes are truly suitable for rebasing.
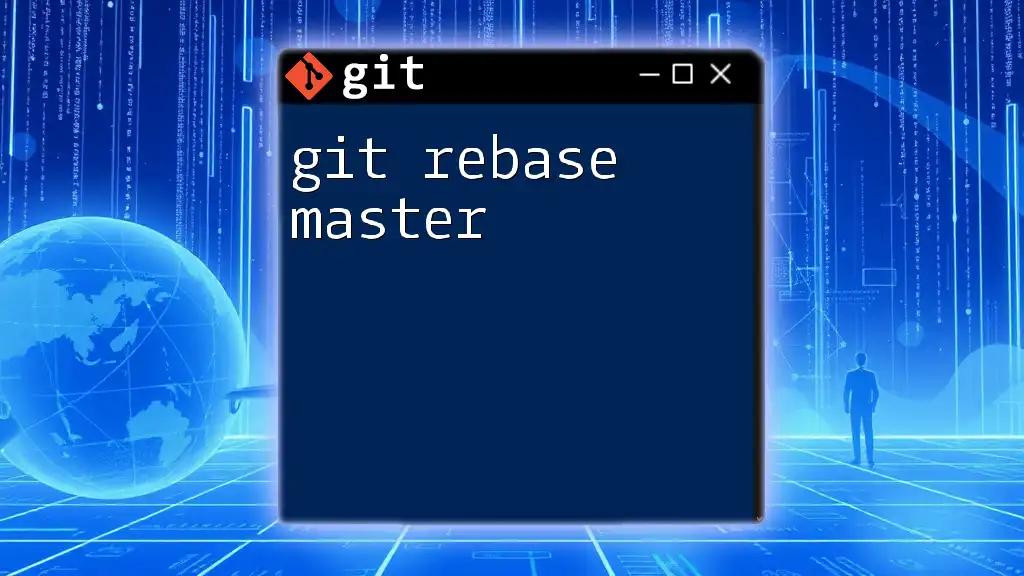
What is Restore in Git?
Understanding Git Restore
In contrast to rebase, the Git restore command is primarily used for managing changes in files within the repository. It helps you revert files to a previous state, whether that's discarding changes in your working directory or resetting files to the state of a specific commit.
Key Features of Restore
The restore command offers a versatile way to recover files and changes. Its primary advantage is that it focuses specifically on file restoration, which allows you to cherry-pick changes or recover files from a past commit without affecting your commit history.
What sets `git restore` apart from other commands such as `checkout` or `reset` is its focused approach. While `git checkout` can be used to change branches as well as restore files, `git restore` is built explicitly for restoration purposes. This specialized focus can help you avoid unintended consequences that may arise from using more generalized commands.
Example of a Git Restore
Here’s how to use the restore command in practice:
git restore filename.txt
This command is straightforward: it will revert `filename.txt` to its last committed state. You can narrow this down even further with the following command:
git restore --source=HEAD filename.txt
This restores the file to the state it was in at the last commit, giving you precise control over your file's history.
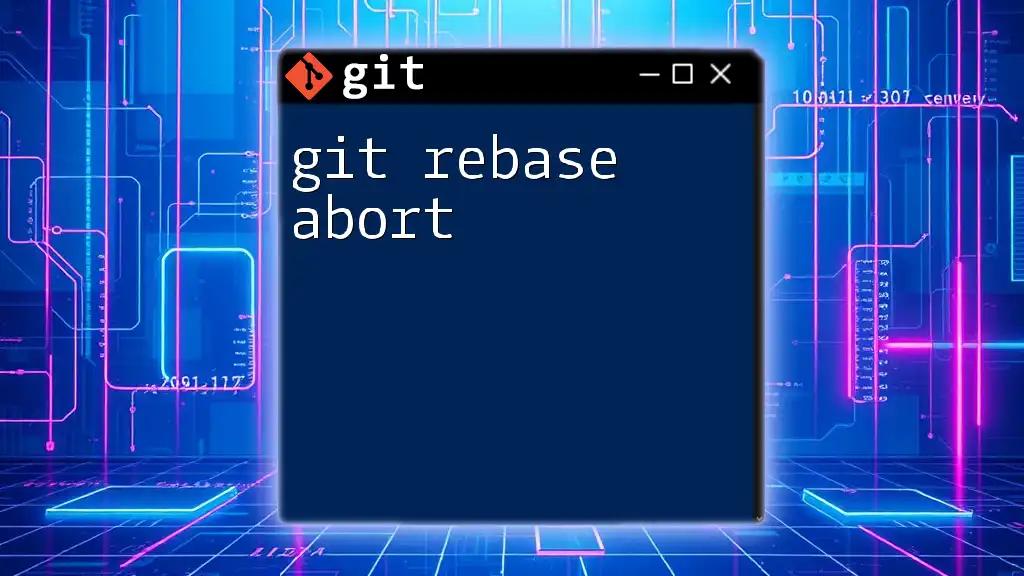
Differences Between Rebase and Restore
Conceptual Differences
At their core, rebase and restore serve different purposes. Rebase is about modifying how commits relate to each other within a branch, helping streamline project history. On the other hand, restore deals specifically with file states, allowing for recovery of changes without altering commit history.
Situational Application
Understanding when to use rebase or restore is vital for effective Git management. For example, if you're working on a feature branch and need to incorporate the latest changes from the main branch, then rebase is the appropriate choice. Conversely, if you've made changes to a file that you want to discard or revert, using `git restore` is the way to go.
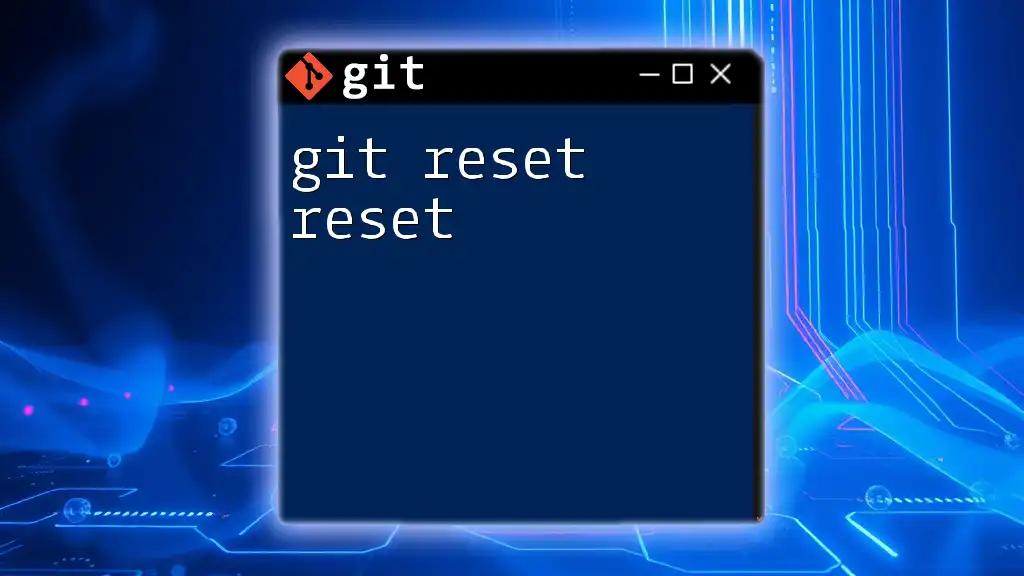
How to Effectively Use Rebase and Restore Together
Workflow Integration
For optimal Git usage, it's beneficial to integrate both rebase and restore in your workflow. As an example, before pushing your branch to the shared repository, you might want to ensure you have the latest updates from the main branch. Using rebase can clean up the history prior to including your changes.
If, during this process, you discover an error in one particular file, you can use the restore command to revert that file to a known good state without affecting other changes.
Real-World Example
Consider this scenario: You are working on a feature branch but realize there are changes in the main branch that you need to include. You might start with:
git checkout feature-branch
git rebase main
After resolving any conflicts that arise, you notice a mistake in one of your files. Instead of resetting your entire branch, a targeted restoration can be performed:
git restore src/someFile.js
Using both rebase and restore in tandem allows you to keep a clean and manageable project history while addressing issues as they arise.
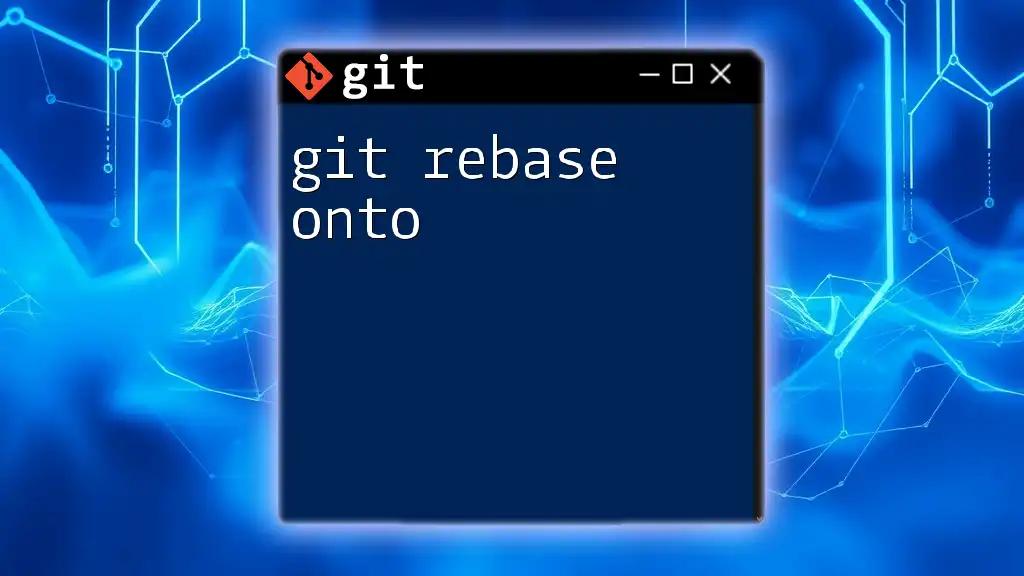
Conclusion
In summary, Git rebase and restore serve distinct yet complementary functions within version control. Rebase is about managing commit history while restore focuses on file management. Understanding these differences and knowing when to use each command can significantly enhance your efficacy and clarity in using Git.
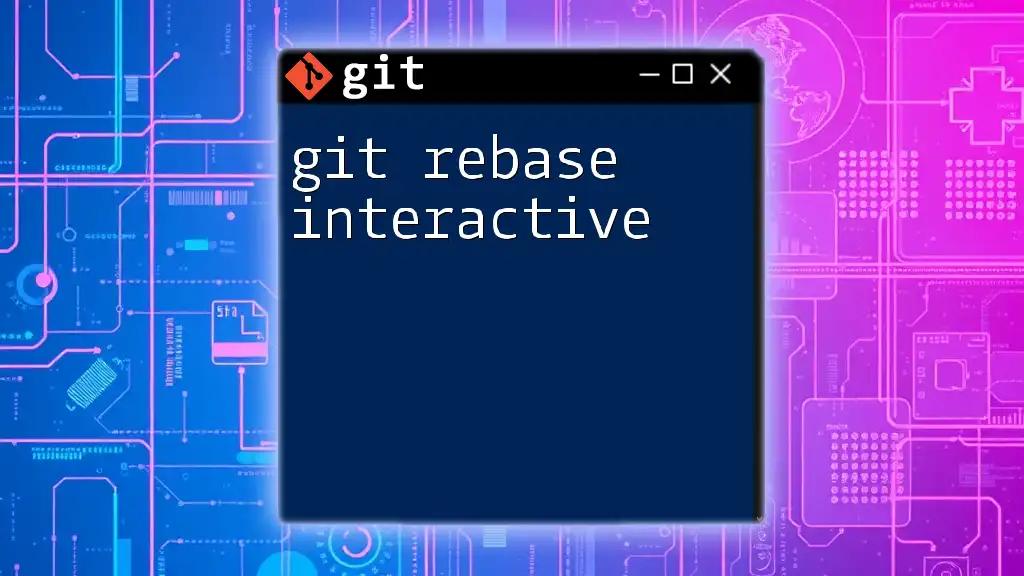
Additional Resources
For those looking to deepen their understanding of Git, check out the [official Git documentation](https://git-scm.com/doc). Online tutorials and courses focusing on Git best practices can also provide more context. Additionally, tools like SourceTree or GitKraken can give you a visual representation of your Git workflow, making it easier to grasp complex operations.
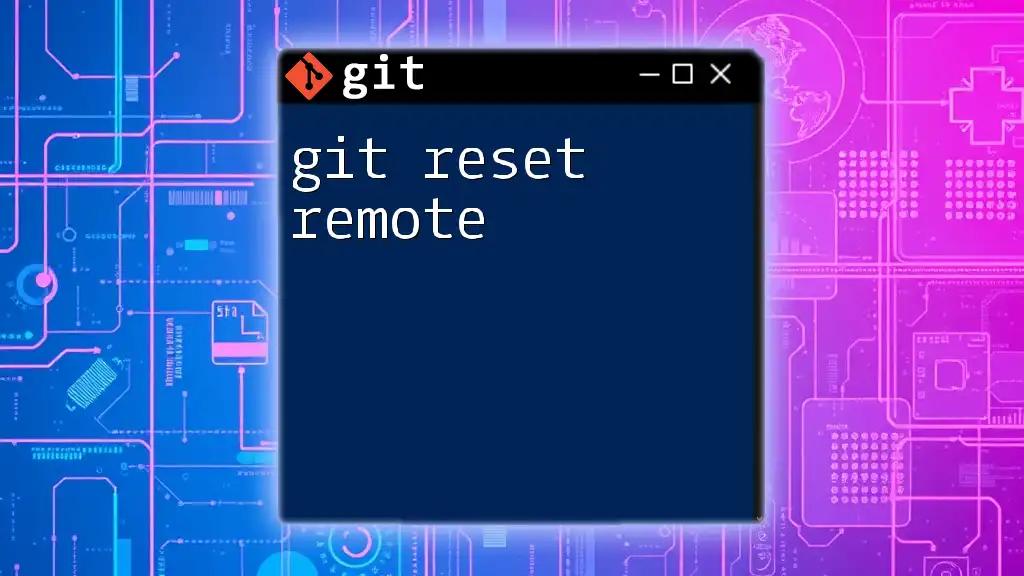
FAQs
Common Misconceptions about Rebase and Restore
One common misconception is that rebasing is always the better choice over merging. While rebasing has its advantages, it's essential to evaluate your project’s needs to make an informed decision.
Further Reading Suggestions
For more advanced Git topics, consider reading books like "Pro Git" by Scott Chacon and Ben Straub or accessing further online content to expand your knowledge on version control and teamwork dynamics in software development.