The command `git fetch --tags` retrieves all tags from the remote repository without modifying your working files.
git fetch --tags
Understanding Git Tags
What are Git Tags?
In Git, tags serve as a means to mark specific points in your project's history, usually aligned with release versions. Unlike branches, which are meant for ongoing development, tags signify milestones — they represent a point in time that is important or noteworthy, such as a version release.
There are two main types of tags in Git:
-
Light-weight tags: Essentially a bookmark for a specific commit, these tags do not contain additional metadata. They are created with a simple command and do not provide any information beyond the commit they point to.
-
Annotated tags: These are stored as full objects in the Git database. They include the tagger's name, email, date, and an optional message. Annotated tags are recommended for releases because the additional information offers context.
Why Use Tags?
Using tags in Git offers numerous benefits, particularly for version control in collaborative environments. Tags help maintain a clear and traceable record of what versions of a project have been released and when.
Consider a software project that undergoes a significant update every quarter. By tagging each release, developers can easily navigate to any version in the project's history. For instance, if a critical bug is identified in version 2.0.1, developers can quickly return to that tagged commit for debugging, testing, and patching, streamlining the recovery process.
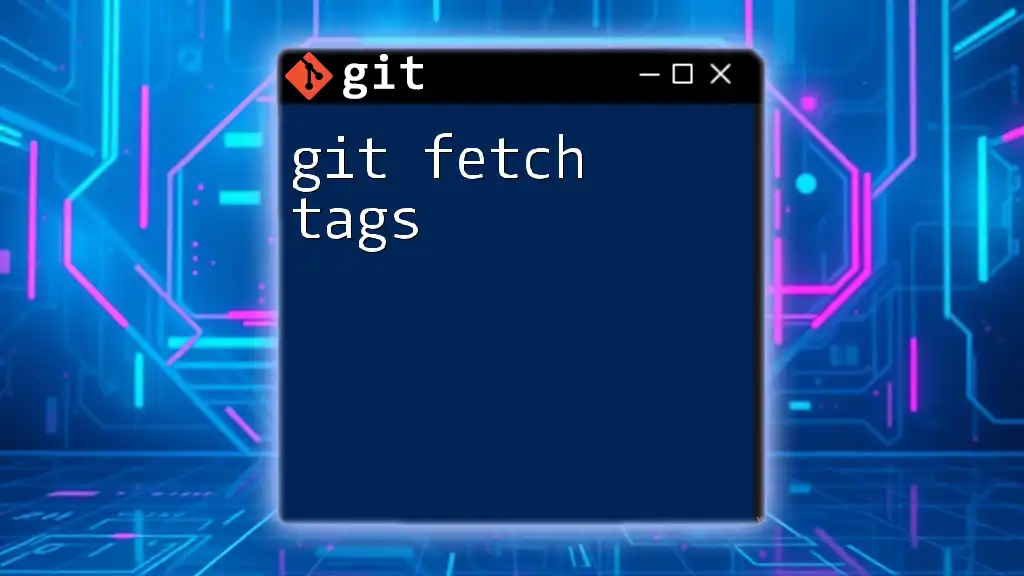
What is `git fetch`?
Definition and Purpose
The `git fetch` command is vital for keeping your local repository updated. It allows you to download commits, files, and references from a remote repository into your local repository without merging those changes. This means you get the latest updates without affecting your current working state.
One common point of confusion is the difference between `git fetch` and `git pull`. While `git fetch` merely retrieves the updates, `git pull` goes a step further by merging those updates into your current working branch. The separation ensures you can review what’s changed before integrating any modifications into your workflow.
Common Use Cases
Usually, it's best to run `git fetch` regularly to stay in sync with your team’s contributions, especially during collaborative projects. When you fetch updates, you can examine changes without risking your current work, helping to avoid conflicts down the line. Best practices suggest fetching updates after a teammate has pushed significant changes, allowing you to assess what has been altered before pushing your own.
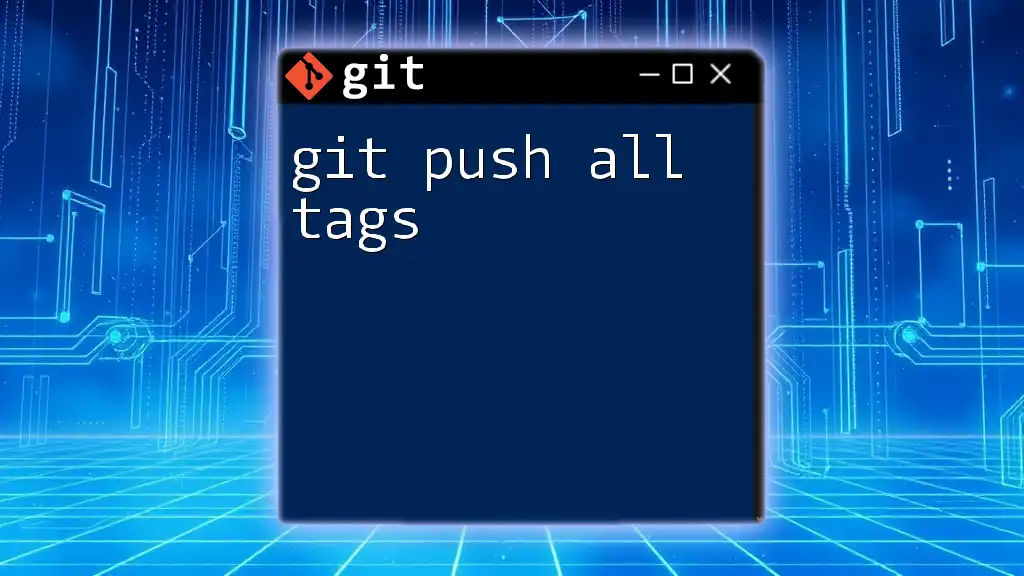
The Command: `git fetch --tags`
Syntax and Basic Usage
The command to retrieve all tags from a remote repository is straightforward:
git fetch --tags [remote-name]
This command serves two purposes: it fetches all tags from the specified remote and updates your local repository so that those tags are now accessible.
Fetching All Tags from a Remote Repository
Consider the following command to fetch all tags from the default remote repository called 'origin':
git fetch origin --tags
When executed, this command will check the remote repository for any tags that are not present locally and will retrieve them, allowing you to seamlessly synchronize your local tagging structure with that of the remote.
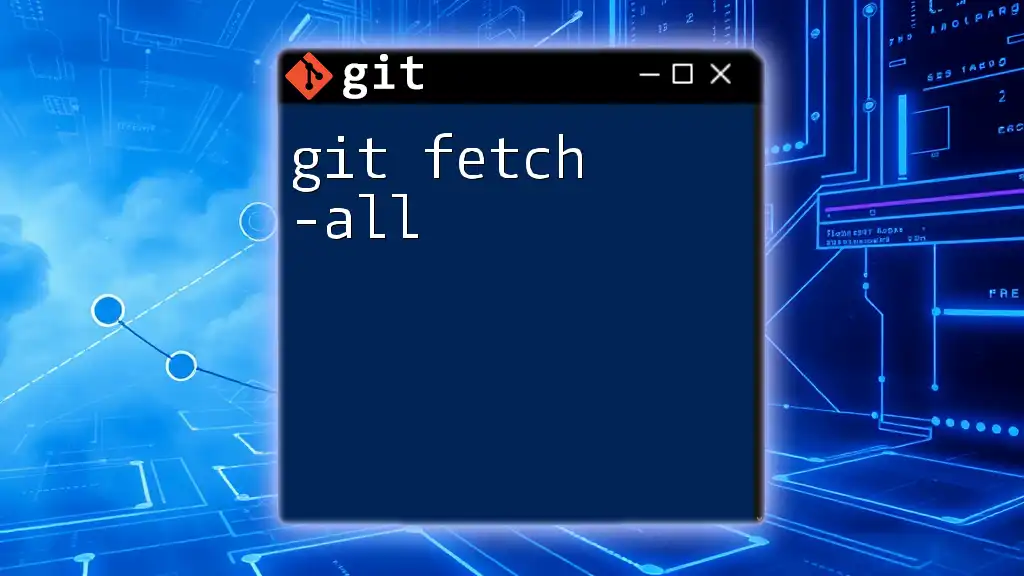
How to Verify Fetched Tags
Displaying Tags in Your Local Repository
To see all the tags stored in your local repository after fetching, you can use:
git tag
The output will be a simple list of all the tags, allowing you to verify that the fetch command executed successfully. It's essential to ensure that all required tags are now part of your local repository.
Checking Out a Tag
If you want to examine a specific version marked by a tag, you can check it out using:
git checkout [tag-name]
Keep in mind that checking out a tag puts your repository in a detached HEAD state, meaning you are not on a branch. You can look around and make changes, but if you want to keep any modifications, you'll need to create a new branch from that tag.
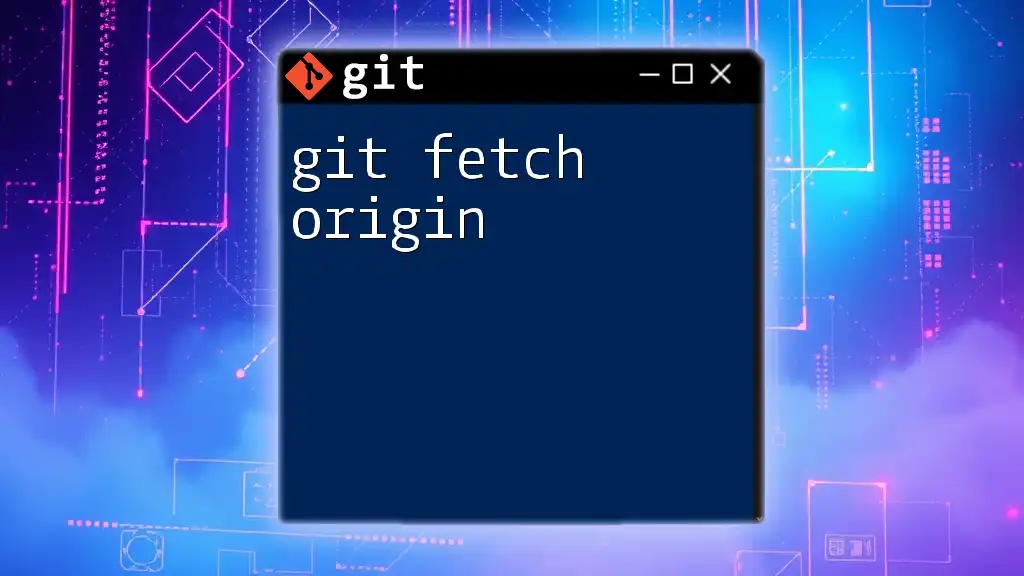
Practical Scenarios
Keeping Your Local Tags Updated
It's crucial to keep your local tags up-to-date with the remote repository, especially when multiple collaborators are pushing new tags. Regularly fetching tags can save you significant frustration later on.
For a typical workflow, consider running the following commands at the start of your work session:
git fetch --tags
git tag
This routine helps ensure you have the latest versions available and can proceed with development without missing critical updates.
Collaborating with Team Members
Using tags allows teams to communicate effectively and maintain a shared understanding of software versions. For instance, if one team member releases version 3.1, they can tag the commit in the remote repository. Other team members can then quickly fetch this tag and base their further development on it, ensuring everyone is aligned and avoiding "version drift."
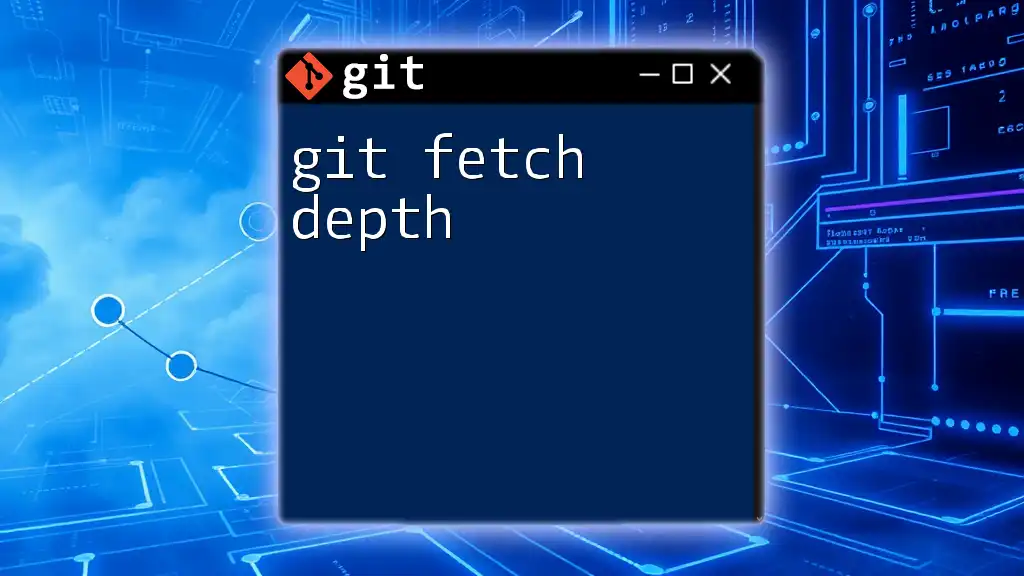
Advanced Usage
Fetching Specific Tags
If you only want a particular tag rather than all tags, you can manually fetch a specific tag using:
git fetch origin tag [tag-name]
This might be handy if the repository includes many tags but you're solely interested in one for a bug fix or feature misunderstanding.
Automation and Scripting
For those looking to automate their workflows, scripts can be incredibly useful. For example, utilizing cron jobs, you can periodically run `git fetch --tags` to ensure your local repository remains up-to-date without manual intervention. The following is a simple example of a script you could use:
#!/bin/bash
cd /path/to/your/repo
git fetch --tags
Set this script to run daily or weekly, depending on your collaboration needs.
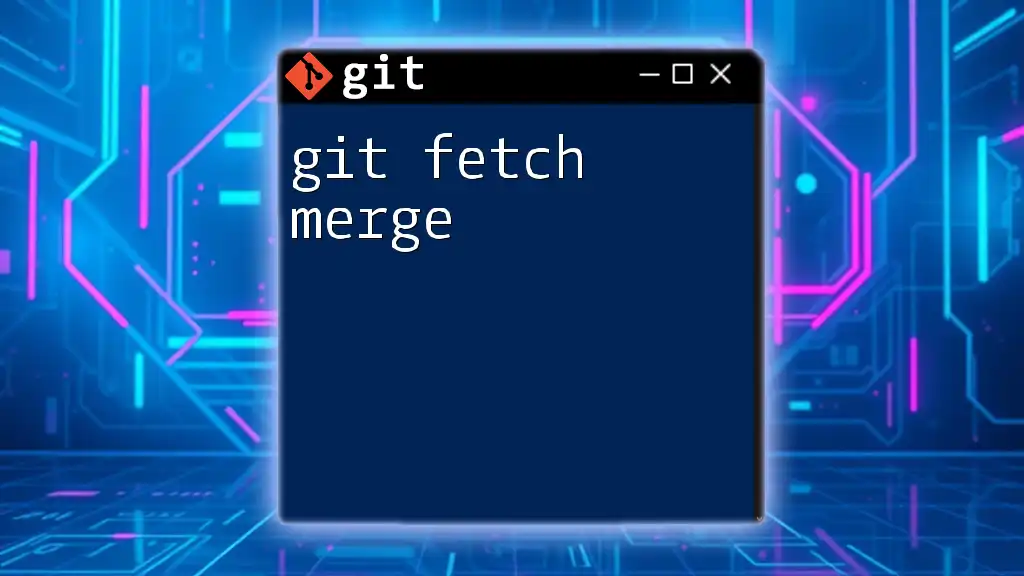
Troubleshooting Common Issues
Problems You Might Encounter
While fetching tags is relatively straightforward, occasional problems can arise:
-
Missing Tags: If you find that certain tags are not appearing, ensure you're fetching from the correct remote and that the tags actually exist on that remote.
-
Tag Conflicts: Some scenarios might lead to conflicts if local tags bear the same name as remote tags that have changed. If this is the case, you might need to delete or rename local tags before fetching.
To diagnose issues effectively, the command `git fetch --tags` usually provides output that can guide your next steps.
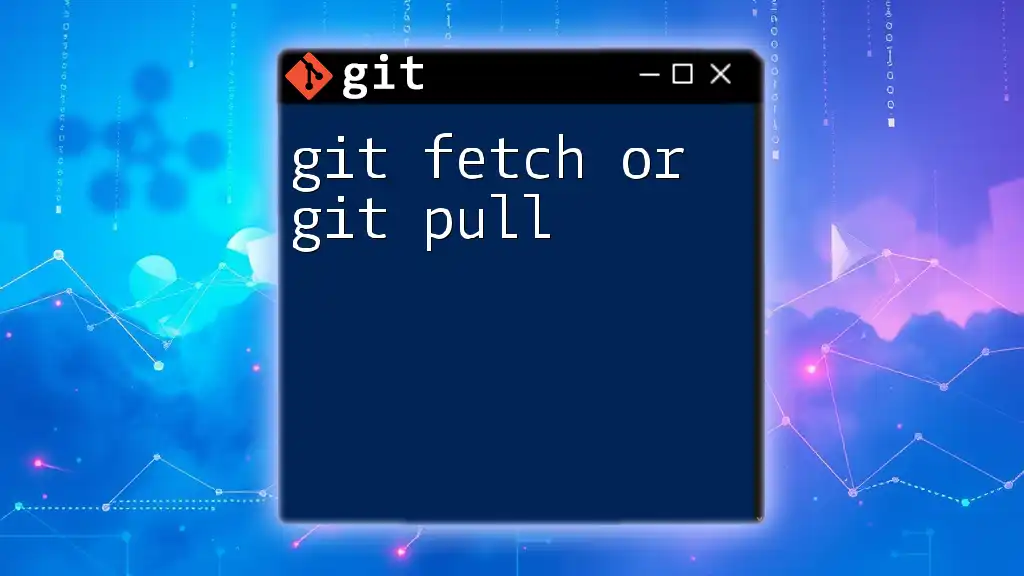
Conclusion
Mastering the use of `git fetch --tags` is a powerful tool in your Git toolkit. It allows you to stay in sync with remote repositories and effectively manage your project’s versioning. By understanding how tags work, when to use `git fetch`, and how to properly maintain your tag structure, you’re setting yourself up for a smoother development experience.
Take the plunge! Start experimenting with tags and enhance your Git capabilities to improve version control and collaboration.