To fetch a specific branch from a remote repository in Git, use the command `git fetch` followed by the remote name and the branch name. Here's how you can do it:
git fetch origin feature-branch
Understanding Git Fetch
What is `git fetch`?
`git fetch` is a command used to download commits, files, and references from a remote repository into your local repository without modifying your working files. When you fetch, Git retrieves updates from the remote server for branches that are tracked remotely but it doesn’t automatically merge any of this new data into your current branches. This gives you the opportunity to review changes before integrating them into your codebase.
It's essential to differentiate `git fetch` from `git pull`. While `git fetch` only retrieves new data, `git pull` combines the actions of fetching and merging. Thus, using `git fetch` allows you to see what others have been working on without altering your own files, which is particularly advantageous for collaborative projects.
When to Use `git fetch`
You might want to use `git fetch specific branch` when collaborating with a team. For instance, before you start working on your feature or bug fix, you can fetch the upstream changes to ensure your current work is based on the latest version of the branch. This can help prevent merge conflicts later on. Here are some scenarios where fetching specific branches is beneficial:
- Updating your local repository without integrating changes immediately.
- Inspecting changes made in the remote branch before merging.
- Preparedness for code reviews or other collaborative tasks.
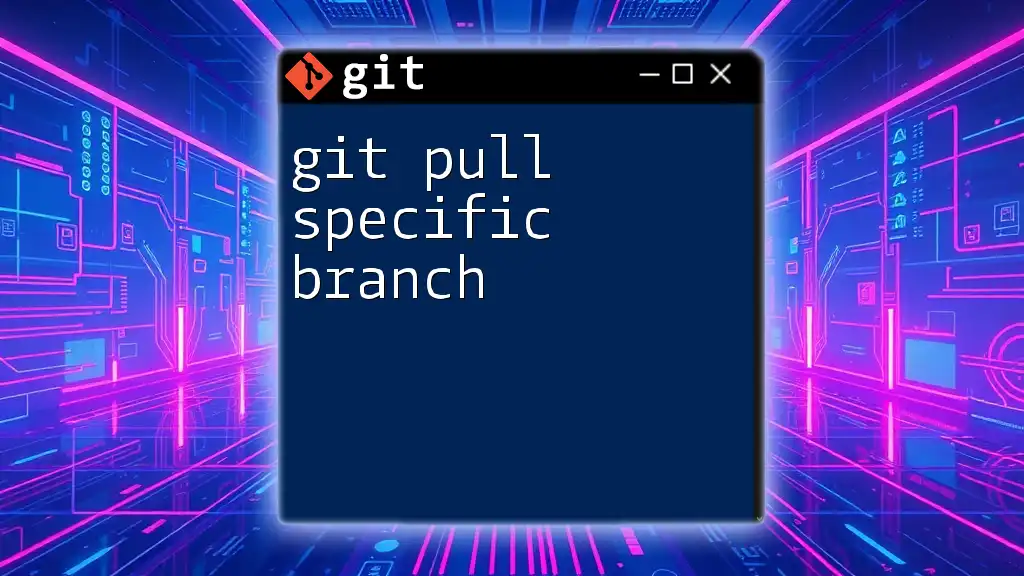
How to Fetch a Specific Branch
Basic Command Syntax
To fetch a specific branch, the command syntax is fairly straightforward:
git fetch <remote> <branch>
In this context:
- `<remote>` typically refers to the name of the remote repository (e.g., `origin`).
- `<branch>` specifies the name of the branch you want to fetch.
Fetching from a Remote Repository
Step-by-Step Process
Identifying the remote: Before fetching a specific branch, you need to confirm the name of your remote repository. You can do this with:
git remote -v
This command will list all configured remotes, typically showing `origin` as the default name pointing to your Git repository.
Fetching the branch: Once you know the name of your remote, fetching a specific branch can be done using the following command:
git fetch origin feature-branch
In this command, `origin` is the remote repository, and `feature-branch` is the name of the branch you intend to fetch. This command pulls down the latest commits from `feature-branch` on `origin` without merging any changes into your local branch.
Verifying the Fetch Operation
Checking Fetched Branches
After executing the `git fetch` command, you can check which remote branches were fetched using:
git branch -r
This command displays a list of all remote branches, allowing you to verify the presence (and updates) of your specific branch. If `origin/feature-branch` appears in the list, you have successfully fetched the branch.
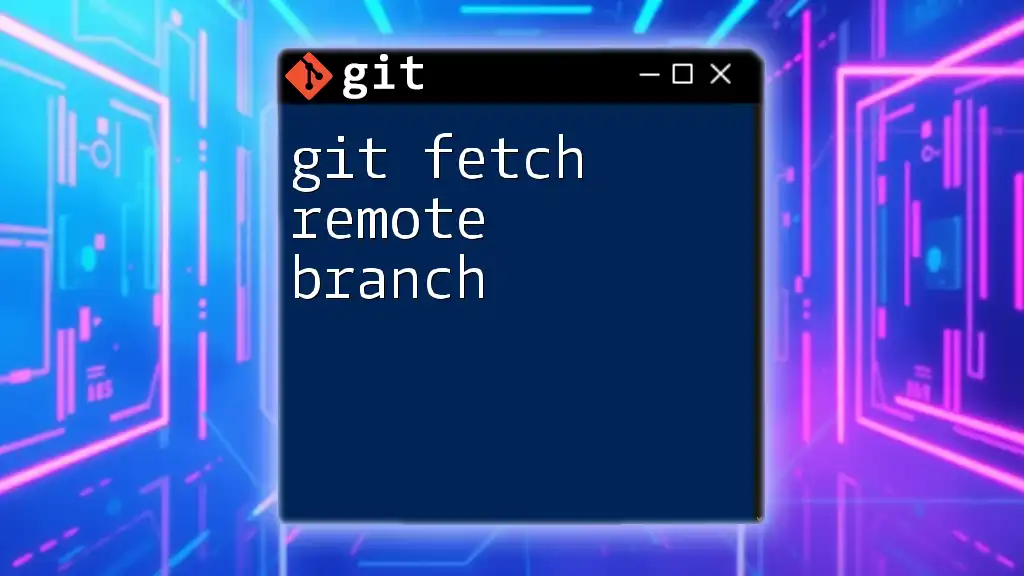
Working with Fetched Branches
Merging or Checking Out the Branch
Merging the Fetched Branch
Once you have fetched the branch, you might want to merge it into your current working branch. This can be done using:
git merge origin/feature-branch
When you execute this command, Git integrates changes from `origin/feature-branch` into your current branch. It’s essential to resolve any potential merge conflicts that may arise during this process to ensure a seamless integration.
Checking Out the Fetched Branch
Alternatively, if you want to switch to the fetched branch, you can simply check it out with:
git checkout feature-branch
This command changes your working context to `feature-branch`, allowing you to work with the most recent updates from that branch. Note that this requires that the branch exists in your local repository as a remote-tracking branch.
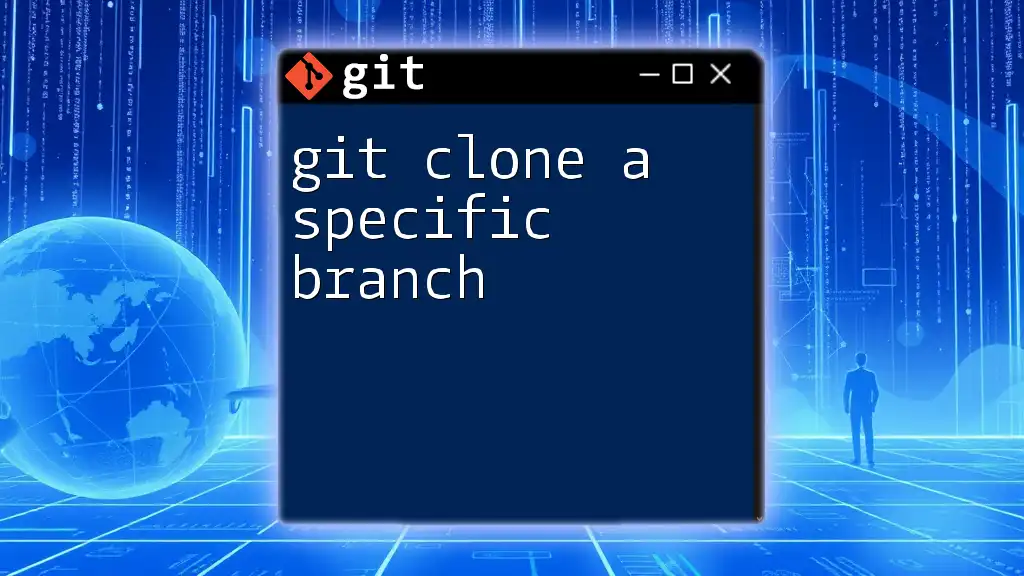
Common Issues and Troubleshooting
What to Do If the Branch Doesn’t Exist
If you attempt to fetch a branch and find that it doesn’t exist, it’s possible it was deleted or renamed. To troubleshoot, check whether the branch you’re trying to fetch has been updated or removed in the remote repository. Use:
git fetch --prune
This cleans up any stale references in your local repository.
Dealing with Fetch Failures
Sometimes you may encounter errors while trying to fetch a specific branch. Common errors might include authentication issues or referring to branches that do not exist. Always pay attention to the error messages provided by Git, as they can guide you toward resolving the problem—whether it’s ensuring proper access rights or double-checking branch names.
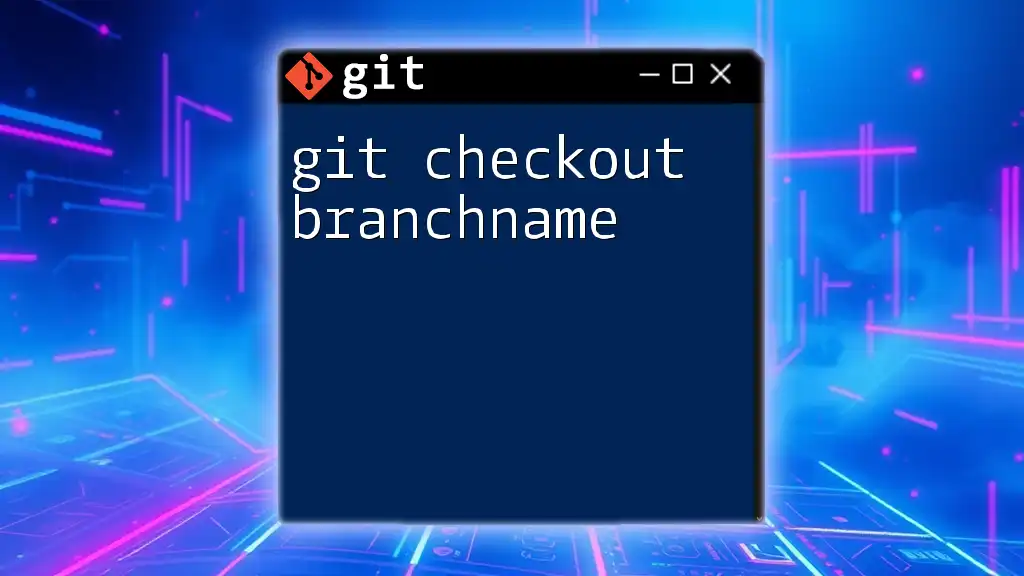
Conclusion
In summary, `git fetch specific branch` is a fundamental command for anyone looking to maintain a clean and up-to-date working environment in a collaborative setting. By utilizing `git fetch`, developers can keep their repositories synchronized without altering their files prematurely. Always remember to inspect changes before merging to minimize conflicts and maintain a smooth workflow.
With practice, mastering this command will significantly enhance your Git proficiency and your ability to work effectively in team projects.
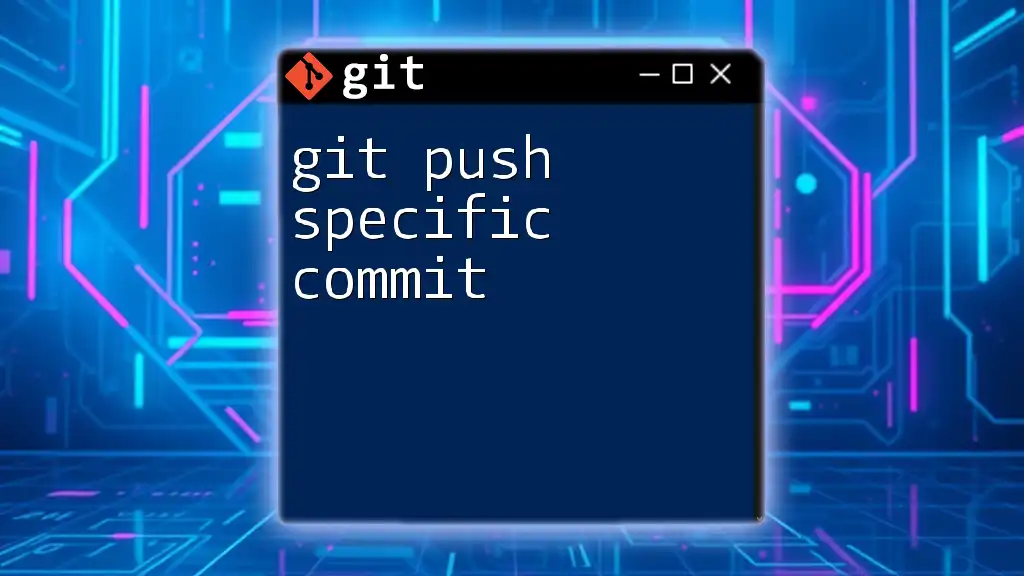
Further Learning Resources
For those eager to deepen their understanding of Git, consider exploring comprehensive online tutorials, the official Git documentation, or connecting with community forums and Git user groups for real-world problem-solving and discussions.