A Git deployment key is an SSH key that provides secure, read-only access to a single repository, allowing you to automate deployments without requiring a full user account.
# Adding a deployment key to your repository
ssh-keygen -t rsa -b 4096 -C "your_email@example.com" # Generate SSH key
cat ~/.ssh/id_rsa.pub # Display the public key to copy
# Go to your Git repository settings and add the public key under "Deployment keys"
What is a Git Deployment Key?
A Git deployment key is an SSH key that grants access to a specific repository. Unlike user-specific SSH keys, these deployment keys are associated with a particular repository and can provide either read-only or read/write access.
The primary purpose of a deployment key is to automate deployments without requiring interaction from a user. Team members, automated scripts, or CI/CD systems can use these keys, allowing for a smooth workflow while maintaining security protocols.
Common use cases for deployment keys include:
- Automating Continuous Integration/Continuous Deployment (CI/CD) processes.
- Enabling read-only access for servers that need to pull code.
- Supporting microservice architectures where different services may require access to specific repositories.
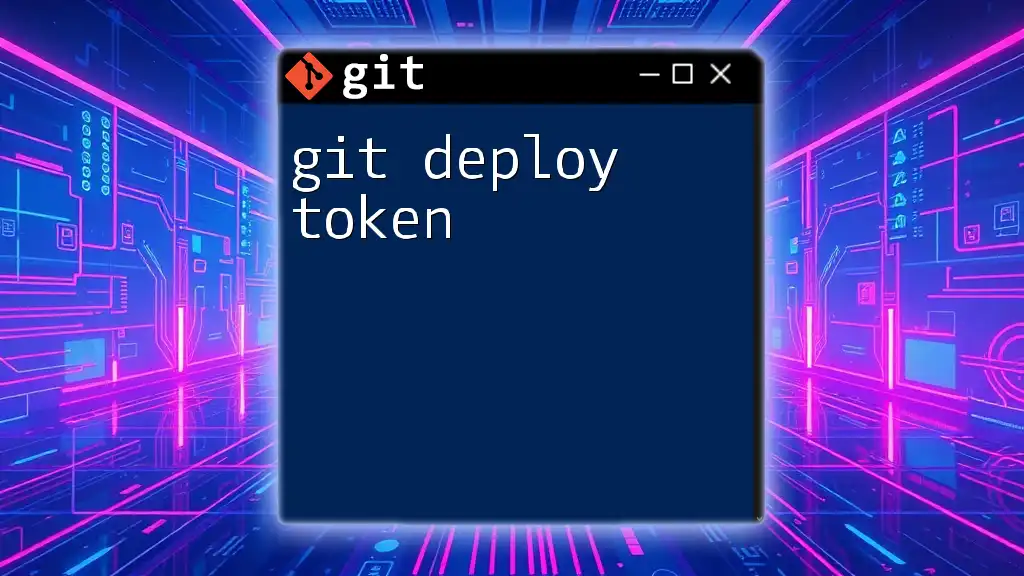
How Deployment Keys Work
Deployment keys utilize SSH (Secure Shell) for authentication, which is considered more secure than using HTTPS for deployment. Using SSH ensures that communication between the server and the repository is encrypted.
During the authentication process, when a server attempts to access a repository using a deployment key:
- The server presents the associated private key.
- The repository checks if the corresponding public key is associated with it.
- If a match is found, access is granted based on the permissions tied to that key.
By using deployment keys, systems avoid the need for manually entering credentials, streamlining automated deployment and operations.
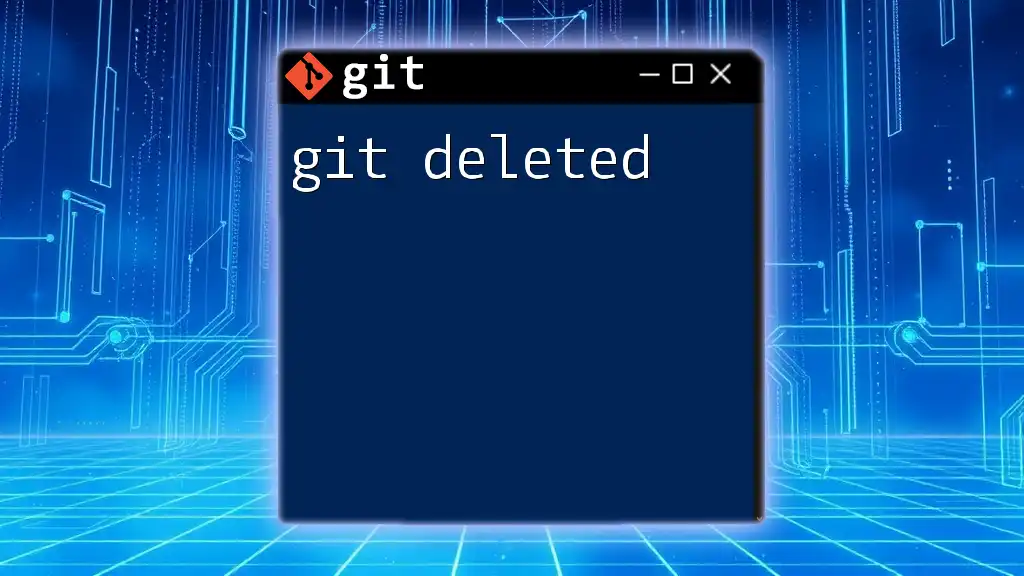
Creating a Git Deployment Key
Step-by-Step Guide
Step 1: Generate an SSH Key Pair
To create a deployment key, first, generate an SSH key pair using the following command:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This command creates a new SSH key, using the provided email as a label. During this step, you will be prompted to specify a file location to save the key. You can either press Enter to use the default location or specify a different one.
Step 2: Locate the Public Key
Once your SSH key pair is generated, you will need to locate the public key. This key is stored in your specified file location. Use the following command to display the public key:
cat ~/.ssh/id_rsa.pub
Make sure to copy this public key, as it will be used to configure your Git deployment.
Step 3: Add the Deployment Key to Your Git Repository
To add the deployment key to your repository on platforms such as GitHub or GitLab:
- Navigate to the repository settings.
- Look for the section labeled "Deploy keys" (on GitHub) or "Repository settings" > "Deploy keys" (on GitLab).
- Click "Add deploy key" and paste your public key into the provided field.
- Specify the access level (read-only or read/write) based on your requirements.
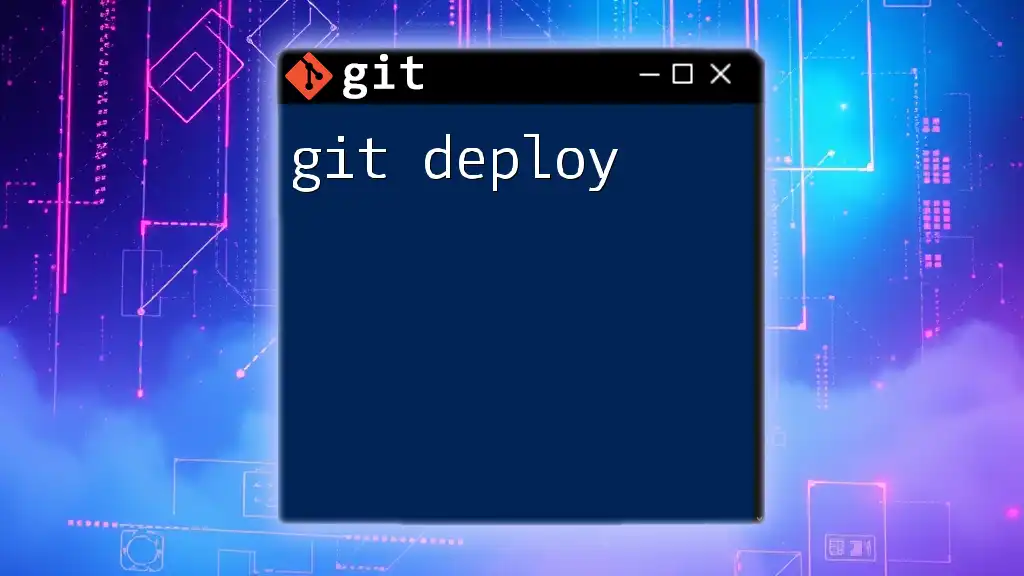
Configuring the Deployment Key
When configuring the deployment key, it's essential to understand how permissions work. Deployment keys can be set to either:
- Read-only: Access the repository to fetch code but not to make changes.
- Read/Write: Ability to fetch code and push changes to the repository.
In CI/CD environments, you may need to leverage environment variables to securely use deployment keys. For instance, in a GitHub Actions workflow, you can securely integrate your deployment key as follows:
# Example for a GitHub Actions workflow
steps:
- name: Checkout code
uses: actions/checkout@v2
with:
ssh-key: ${{ secrets.SSH_PRIVATE_KEY }}
In this example, `${{ secrets.SSH_PRIVATE_KEY }}` refers to a securely stored secret containing your private key.
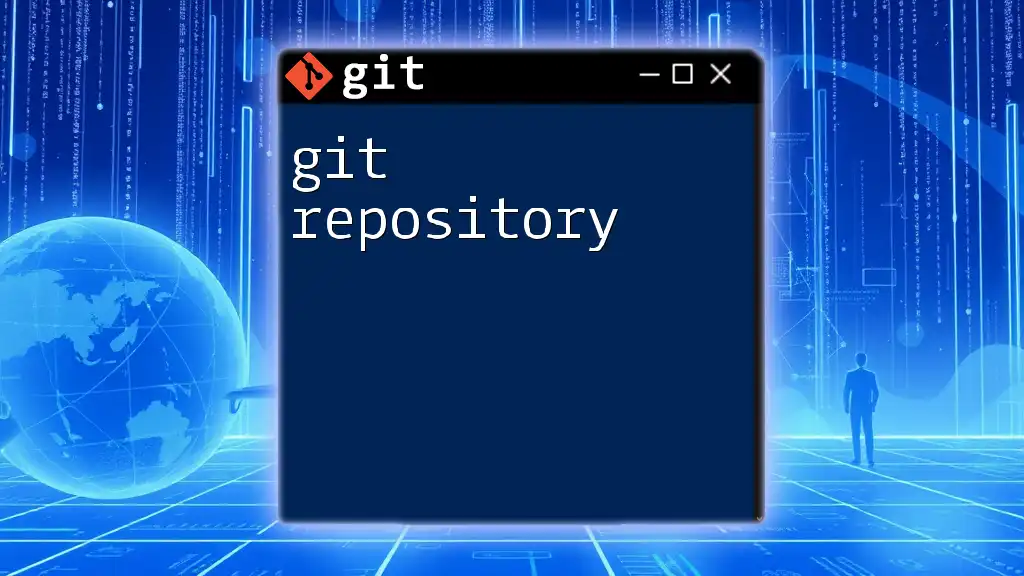
Using the Deployment Key
Once the deployment key is configured, you can begin using it to access the repository. To clone a repository using the deployment key, utilize the SSH URL as follows:
git clone git@github.com:username/repo.git
This command will clone the specified repository onto your local machine, using the deployment key for authentication.
When working with deployment keys, it’s crucial to follow best practices to ensure their security:
- Regularly rotate keys to limit the impact of any potential exposure.
- Restrict access to the minimal necessary permissions.
- Audit the usage of deployment keys periodically to detect any unauthorized access.
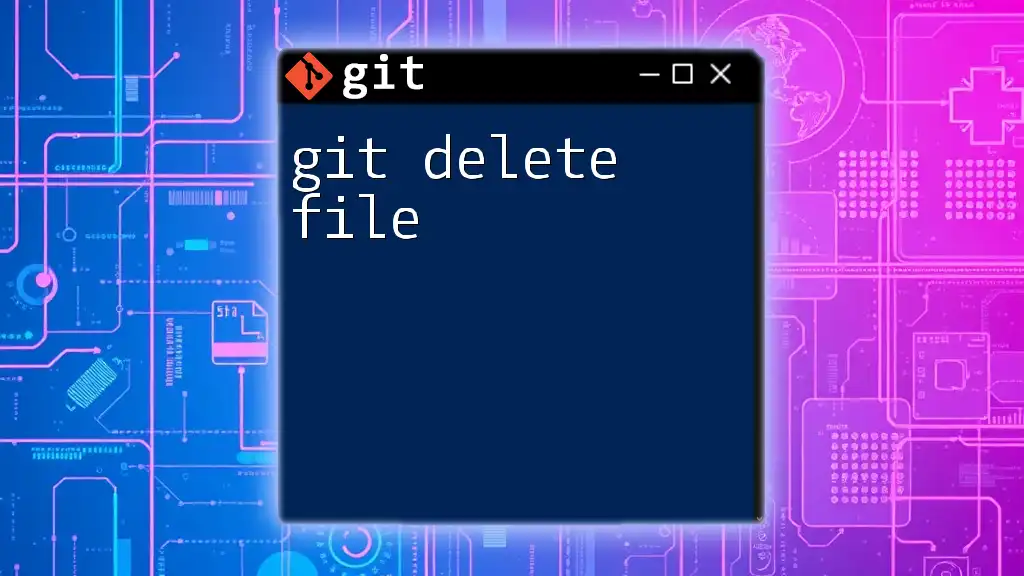
Managing Deployment Keys
Updating Keys
Over time, you may need to update or regenerate your deployment keys. Follow these steps:
- Generate a new SSH key pair using the `ssh-keygen` command.
- Replace the old public key in the repository settings with the new one.
- Save the corresponding private key securely on your server.
Revoking Keys
If a deployment key is no longer needed or may have been compromised, you should quickly revoke it:
- Navigate to the repository settings.
- Find the "Deploy keys" section.
- Locate the key you wish to remove and click the "Delete" or "Revoke" button.
Taking timely action to manage deployment keys is essential for maintaining the security of your repositories.
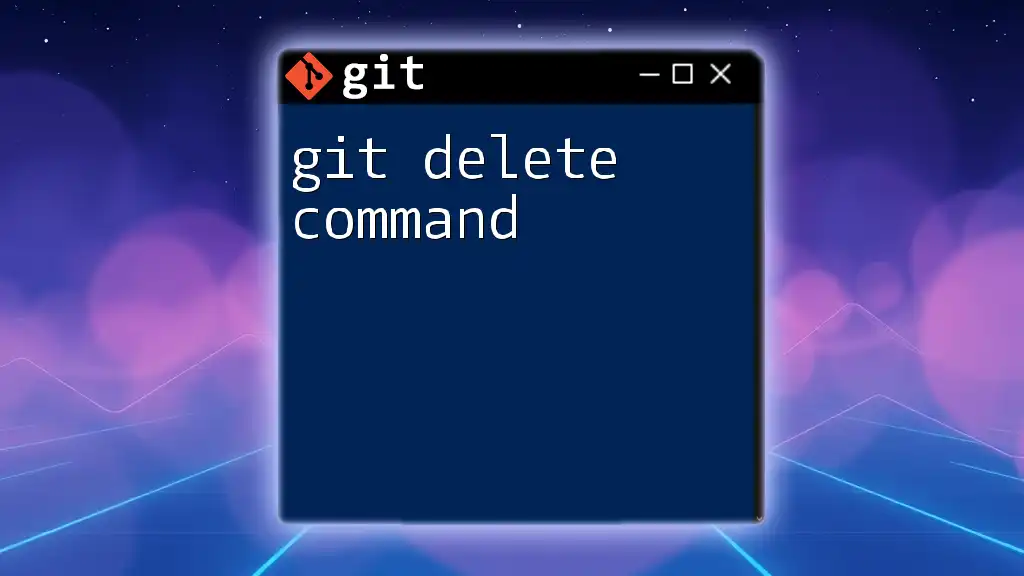
Common Issues and Troubleshooting
When working with deployment keys, users may encounter several issues. Here are some common problems and their solutions:
SSH Agent Issues
Sometimes the SSH agent may not be running or may not have the key loaded. To troubleshoot, run:
ssh-add ~/.ssh/id_rsa
This command adds the specified private key to your SSH agent, enabling it for authentication.
Permission Denied Errors
If you encounter a "Permission denied" error message, verify that the public key has been correctly configured in the repository and that the private key is present on your server.
Checking Configuration
To verify that your SSH configuration is correct, try testing your connection with the command:
ssh -T git@github.com
If the configuration is correct, you should see a welcome message or confirmation that authentication was successful.
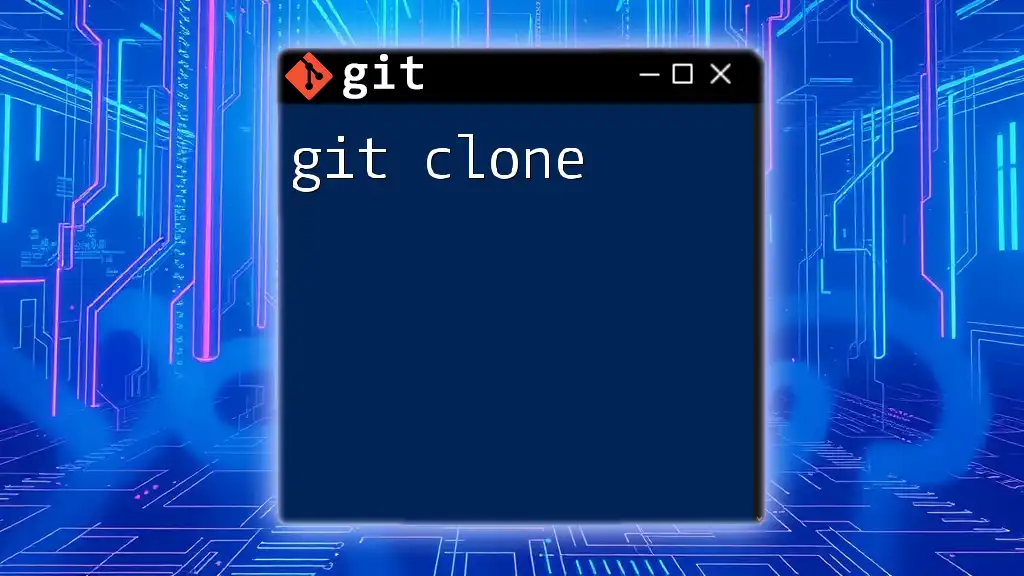
Conclusion
In summary, Git deployment keys simplify the process of automating workflows, especially in CI/CD contexts, while providing a secure means of accessing repositories. By following best practices in their management, such as key rotation, permission restrictions, and regular auditing, you can ensure that your deployment process remains secure and efficient.
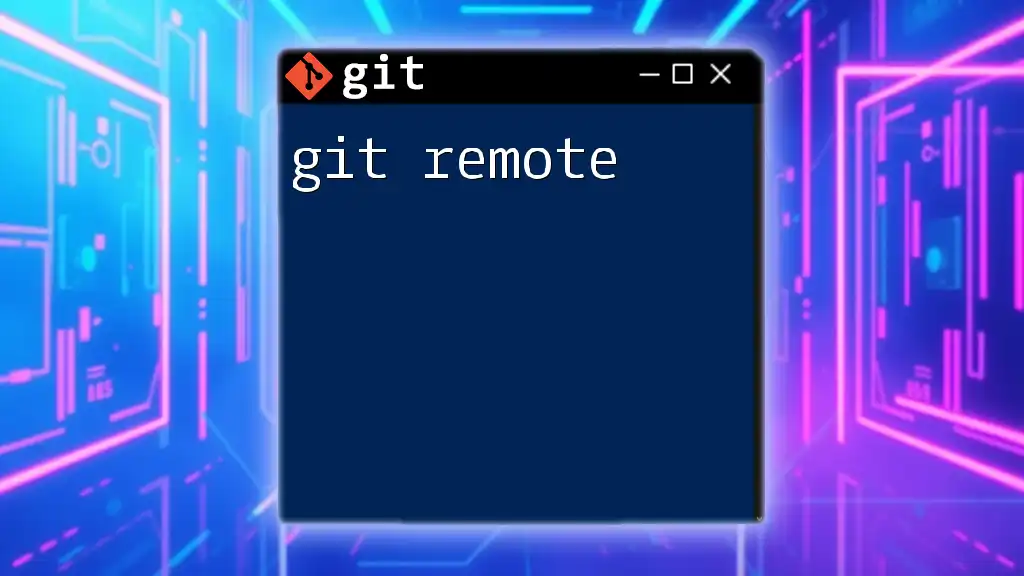
FAQ
What is the difference between a deployment key and a personal access token?
Deployment keys are SSH keys specific to a repository that allow access without a user's intervention. In contrast, personal access tokens are user-generated tokens that allow broader access to Git repositories and operations.
Can I use deployment keys for multiple repositories?
Each deployment key is typically associated with a single repository. However, you can generate multiple keys for different repositories or use the same public key across multiple repositories, keeping in mind the security implications.
What happens if a deployment key gets compromised?
If a deployment key is compromised, you should revoke it immediately. Generate a new key pair and update the repository with the new public key to restore secure access.