The `git blame -e -M -C` command allows you to view the author information for each line of a file while ignoring specific revisions, which can help focus on relevant contributions; you can specify the revisions to ignore with the `-e` and `--ignore-revs-file` options.
Here's an example code snippet in markdown format:
git blame --ignore-revs-file=<path_to_ignore_file> <file_name>
In this command, replace `<path_to_ignore_file>` with the path to a file containing the commit hashes you want to ignore and `<file_name>` with the name of the file you're inspecting.
What is `git blame`?
`git blame` is a powerful command in Git that is used to track changes in a file by showing the author, commit hash, and the date of the last modification for each line of the file. This allows developers to understand the history and evolution of the code, highlighting who made specific changes and when.
Functionality
When you run `git blame` on a file, it outputs each line in context with the relevant information on who modified it last. This information can be incredibly useful for:
- Understanding Code Ownership: Discovering who is responsible for a particular section of code.
- Debugging: Tracing the introduction of bugs by identifying related commits.
- Learning: Exploring how code evolves over time to better grasp coding practices and decisions.
Use Cases
There are several scenarios in which `git blame` is particularly useful, including during code reviews, onboarding new developers, and general maintenance of a codebase. Each of these situations benefits from knowing the history behind changes.
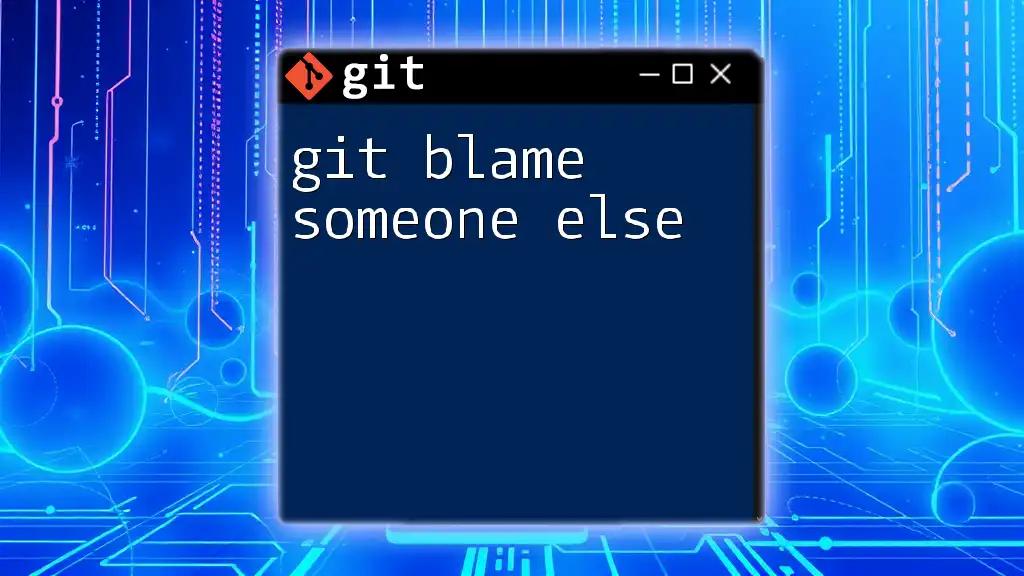
The Problem with `git blame`
Despite its usefulness, `git blame` has its drawbacks. As your codebase grows and becomes more complex, the history of changes can become cluttered.
Complex History
In many projects, developers frequently perform merges or apply various formatting fixes over time. This can complicate the output of `git blame`, making it difficult to interpret which changes are genuinely relevant.
Irrelevant Commits
Committing formatting changes or commits that merely refactor code can make it challenging to read the blame output meaningfully. These irrelevant commits can obscure the essential code contributions, making it harder to identify the original intent behind the code.
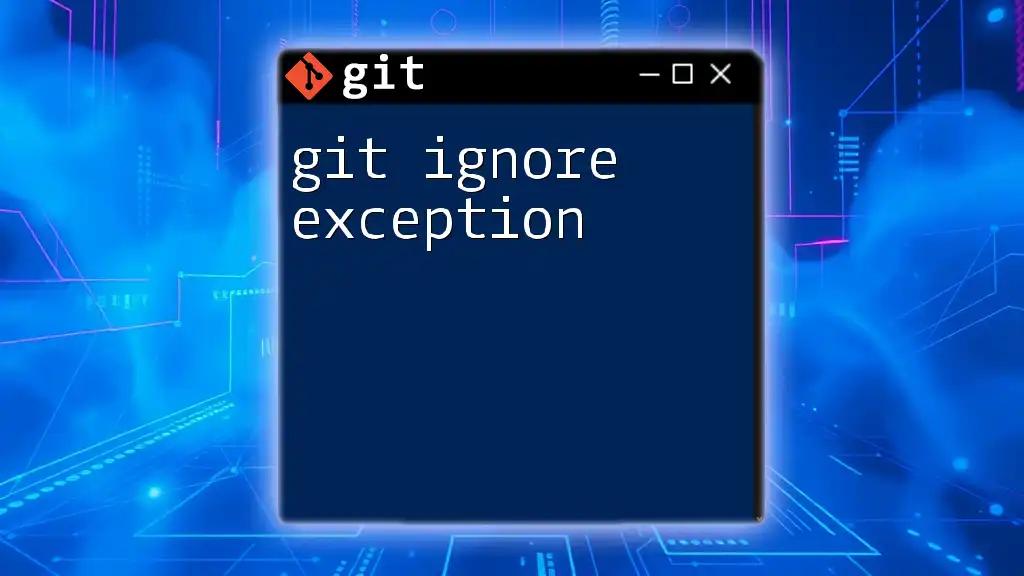
Introducing the `--ignore-revs` Option
Git provides a solution for these challenges through the `--ignore-revs` option, which enables users to ignore specific commits when running `git blame`. This feature can significantly streamline the output by focusing exclusively on relevant contributions.
How it Works
When you use the `--ignore-revs` option, you supply a commit hash or hashes that you want Git to exclude from the output. This allows you to filter out unnecessary noise, improving the clarity and usability of the blame information.
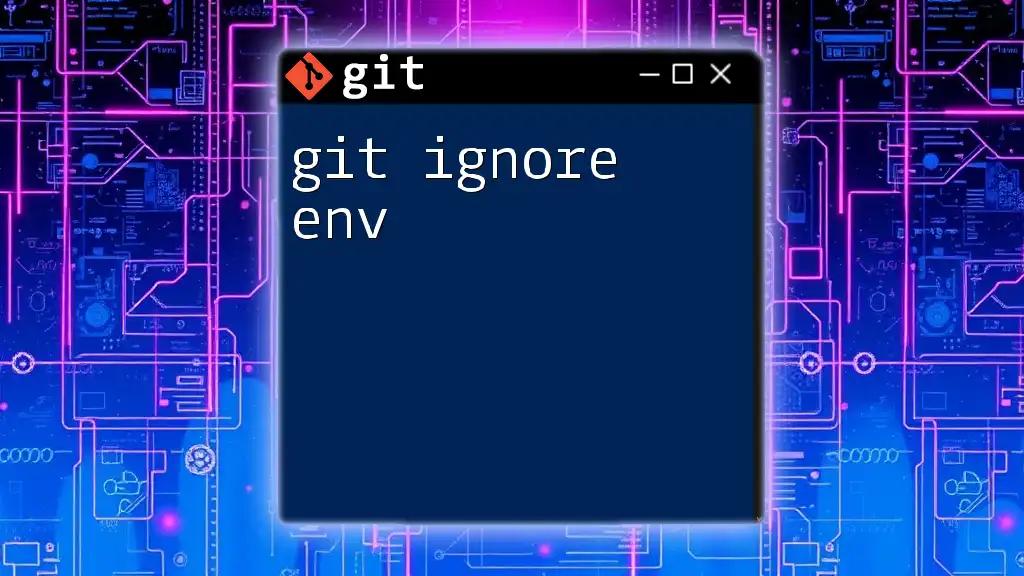
How to Use `git blame --ignore-revs`
Basic Syntax
The basic syntax for using the `--ignore-revs` option is straightforward:
git blame --ignore-revs <commit_hash> <file>
Example Command
For example, if you wish to ignore a specific commit identified by its hash `abcdef123456` for a file named `myfile.py`, you can use the command:
git blame --ignore-revs abcdef123456 -- myfile.py
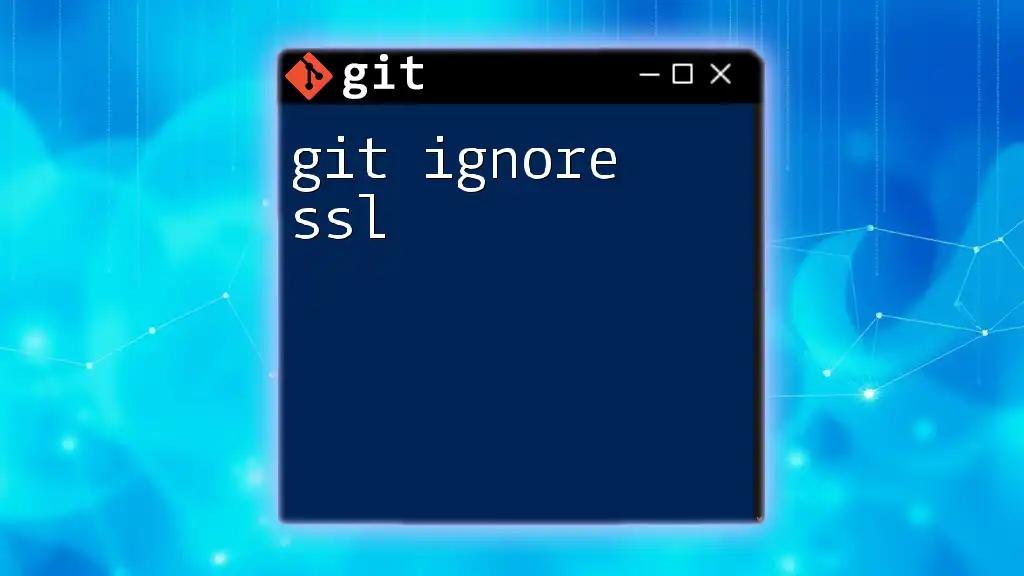
Creating `.git-blame-ignore-revs` File
Purpose
If you have multiple commits that need ignoring, creating a designated file for this purpose can be beneficial. The `.git-blame-ignore-revs` file lists the hashes of commits that you'd like Git to ignore during blame operations.
Format
The structure of the `.git-blame-ignore-revs` file is simple, with each line containing a commit hash followed by an optional reason for the exclusion:
abcdef123456 Merge commit to reformat code
123456abcdef Fix typo in documentation
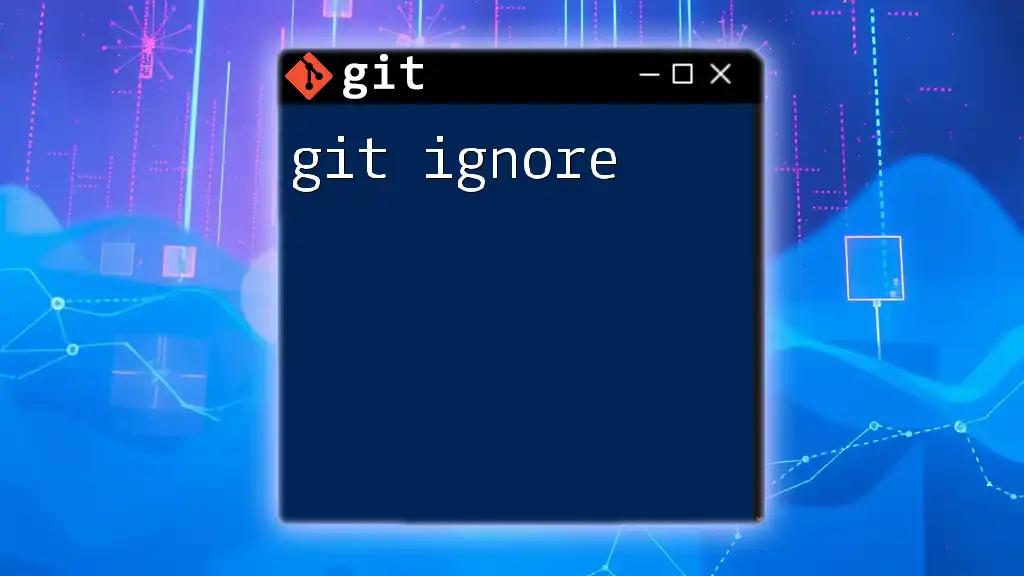
Implementing the Ignore File in Git
Step-by-Step Instructions
Create the File: To set up the `.git-blame-ignore-revs` file in your repository, first create it:
touch .git-blame-ignore-revs
Add Commits to the File: Open the file in your preferred text editor and add the commit hashes you wish to ignore:
nano .git-blame-ignore-revs
Configure Git to Recognize the File: Use the following command to tell Git to use this ignore file:
git config blame.ignoreRevsFile .git-blame-ignore-revs
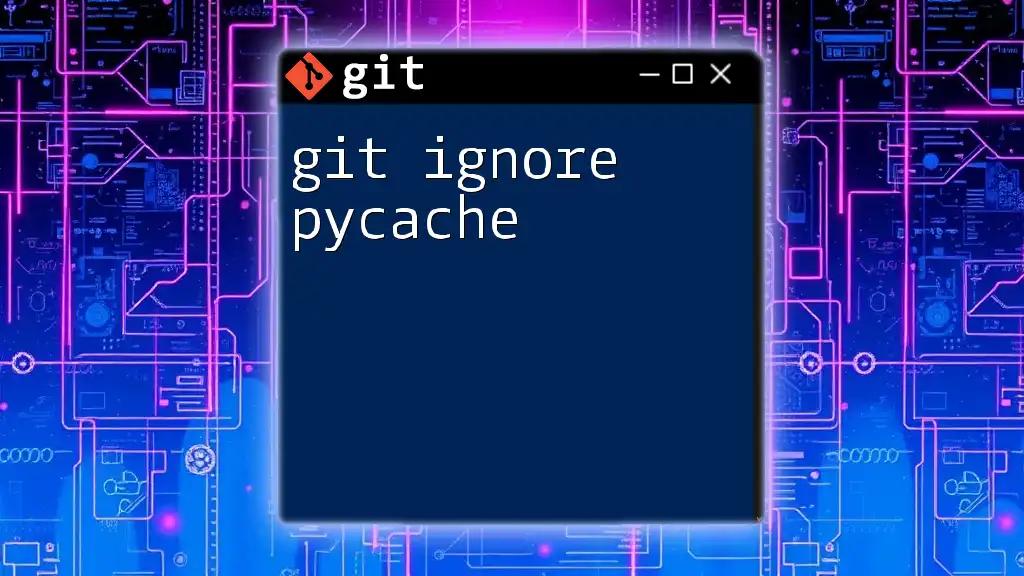
Combining `--ignore-revs` with Other Options
`--ignore-revs` can be combined with other useful `git blame` options, enhancing its utility.
Other Useful Flags
- `-L` for Line Range: Use this option to limit blame output to a specific range of lines in the file, making it even easier to focus on relevant changes.
- `-C` for Change Copies: This option helps track changes made to lines that have been copied, adding depth to your investigation of code history.
Example Combining Options
For instance, if you want to only see blame information for lines 10 to 20 while ignoring certain revisions, you can do so with:
git blame -L 10,20 --ignore-revs myfile.py
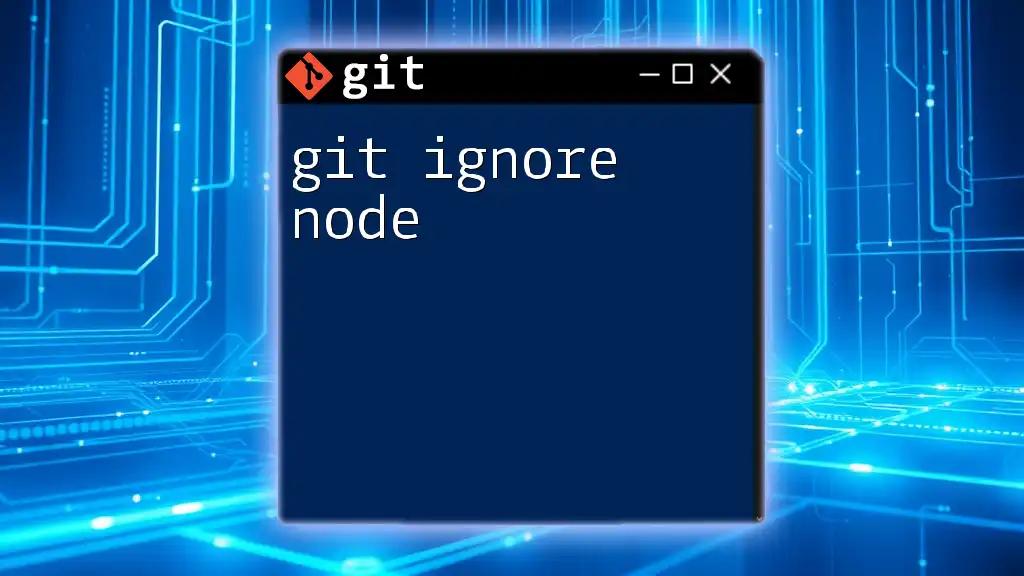
Common Use Cases and Scenarios
Refactoring
One of the primary scenarios for using `git blame ignore-revs` is during code refactoring. When developers refactor code, they may commit many changes that aren't meaningful to the history. By using the ignore feature, the remaining lines reflect only the actual contributions, preserving the intent of the original code.
Temporary Changes
Sometimes, temporary changes might clutter blame output. For instance, a commit may involve update dependencies or similar tasks that don’t directly impact the code itself. Ignoring these revisions keeps the focus on significant code contributions.
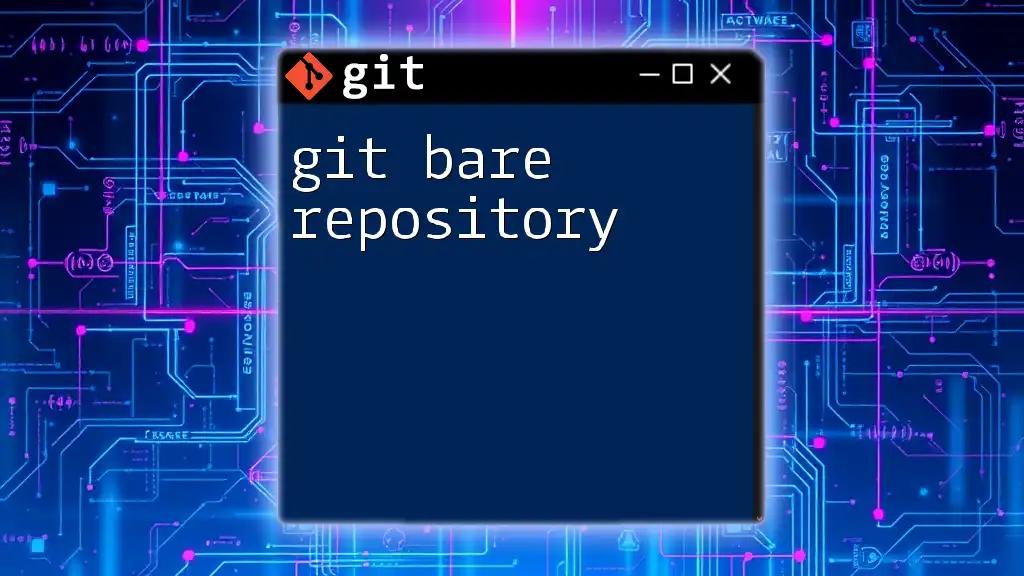
Troubleshooting Common Issues
No Output with Ignore Revs
If you run `git blame` with `--ignore-revs` and don't see any output, there could be several reasons behind this. Check to ensure that the commit hashes listed in your `.git-blame-ignore-revs` file are accurate and belong to the commits you intended to ignore.
Incorrect Commit Ignoring
If you find that Git is not ignoring the expected commits, be sure to revisit your `.git-blame-ignore-revs` file for typos or formatting errors. Each commit hash should be precise, or the ignore functionality may not work as intended.
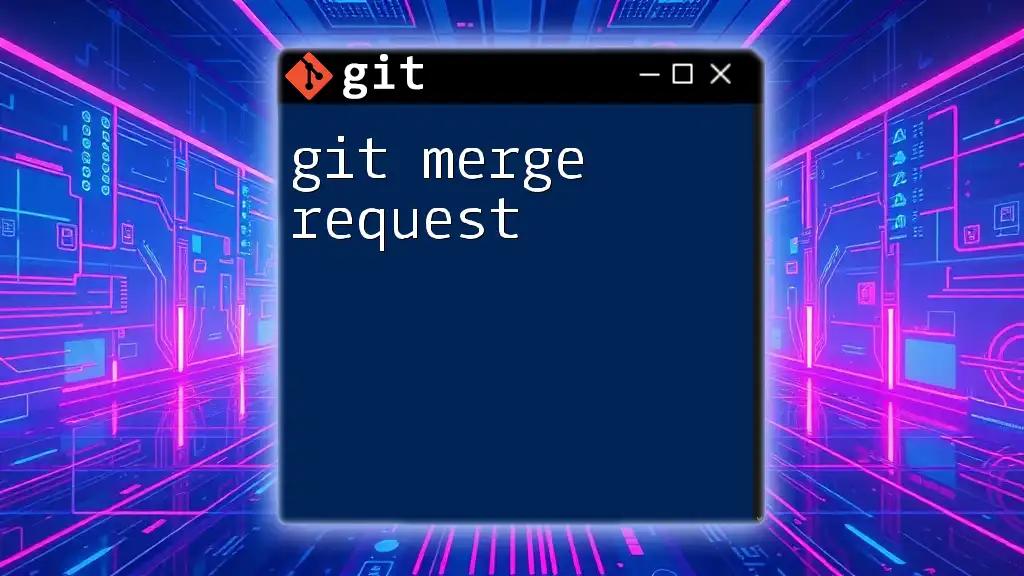
Conclusion
In summary, mastering the `git blame ignore-revs` command can enhance your workflow by allowing you to filter out irrelevant changes that obscure the contributions that truly matter. By leveraging these capabilities, you can achieve a clearer and more understandable revision history in your projects.
Call to Action
We encourage you to practice implementing `git blame --ignore-revs` and share your experiences. Engage with your peers by discussing what you’ve learned and how it has made your development process smoother. Don’t forget to subscribe for more Git tips and tutorials!