The `git -a` command is often a shorthand used with `git commit` that automatically stages the changes to tracked files before committing them.
Here’s how you can use it:
git commit -a -m "Your commit message"
Understanding the Basics of Git
What is Git?
Git is a powerful version control system that enables developers to manage changes to their code effectively. It tracks modifications, allows multiple contributors to work on the same project simultaneously, and helps maintain a history of changes made over time. Utilizing Git ensures that development teams can collaborate efficiently without overwriting one another’s work.
Version Control and its Importance
Version control systems (VCS) are essential tools for managing changes in projects, especially in software development. They provide several benefits:
- Tracks Changes: Every modification is logged, allowing for easy review and restoration of previous versions.
- Collaboration: Multiple contributors can work on the same codebase without interfering with one another's changes.
- Backups: Code is stored in a repository, creating a backup that can be revived if needed.
Git is a widely used VCS due to its speed, flexibility, and ability to handle projects of all sizes.
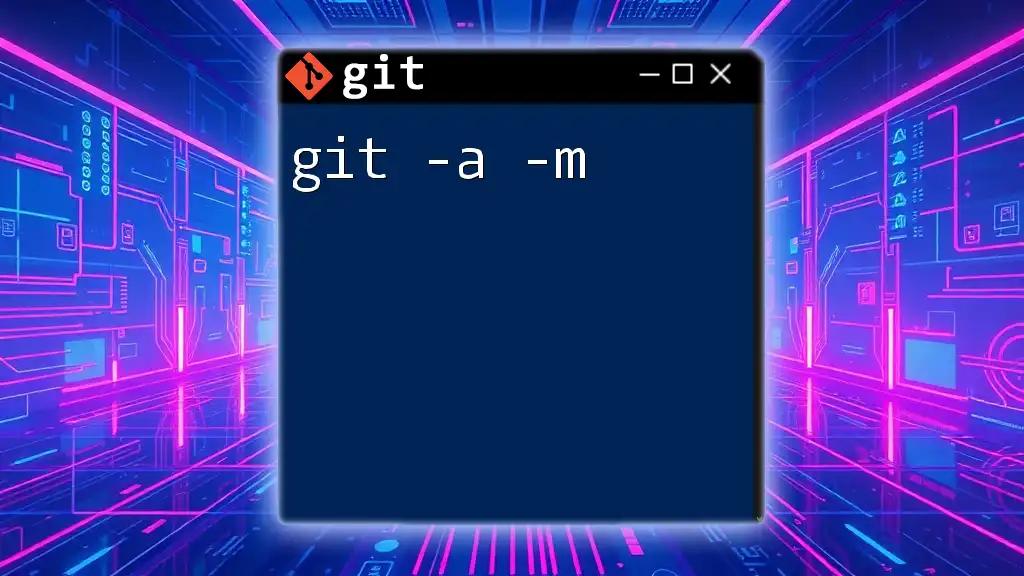
The `git add` Command
Overview of `git add`
The `git add` command is used to add changes in your working directory to the staging area. This staging area is a crucial step before committing changes, ensuring that only the intended modifications are included in a commit.
The Use of `-a` in Context
The `-a` flag stands for "all" and is often used with the `git commit` command rather than `git add`. Here’s how it fits into the picture:
- `git add`: Adds specified changes to the staging area.
- `git commit -a`: Automatically stages all modified files and commits them together. This is particularly useful for quickly committing changes without explicitly adding each modified file.
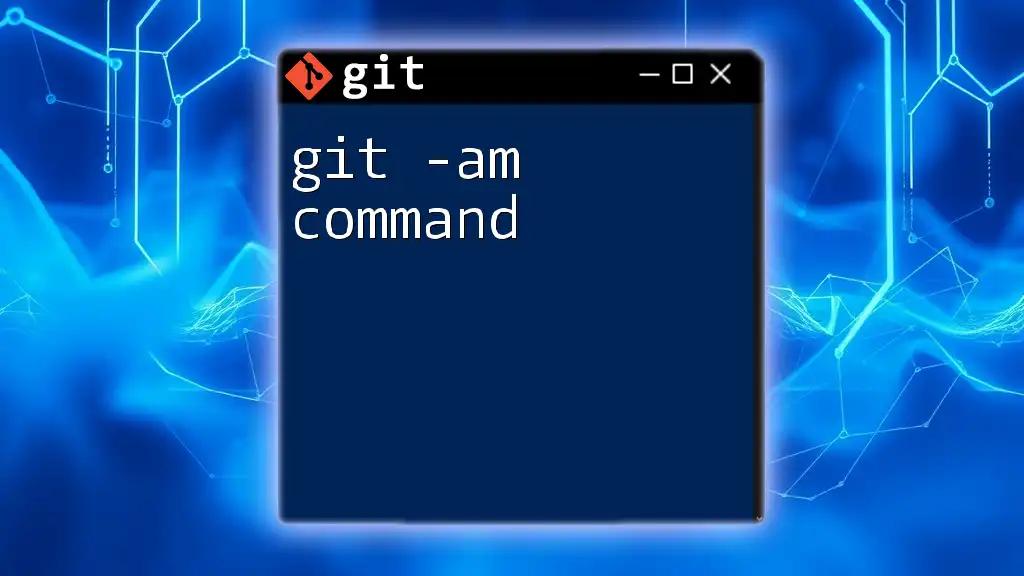
How to Use Git -a: Step-by-Step Instructions
Basic Syntax of Git -a
To effectively use the `git -a`, you typically combine it with the `commit` command, like this:
git commit -a -m "Your commit message"
Detailed Explanation of the Command
- `commit`: This command finalizes changes that have been staged, saving them to the repository history.
- `-a`: Automatically stages all modified files (but not new, untracked files) before committing. This greatly simplifies the process of committing changes, but should be used with caution.
- `-m`: Following this flag, you provide a commit message that describes the changes. Clear and concise commit messages are critical for maintaining a well-documented project history.
Practical Scenario of Using `git commit -a`
Imagine you are working on a project and have modified several files. After making changes to `file1.txt` and `file2.txt`, you want to commit those changes without having to use the `git add` command explicitly. You can run:
git commit -a -m "Updated file1 and file2 with new features"
This command will stage changes to both files and commit them in one step, streamlining your workflow.

Common Mistakes When Using Git -a
Overusing the `-a` Flag
While `git commit -a` can be a time-saver, over-relying on it may lead to unintended consequences. One common mistake is including changes that should not be in the same commit. For instance, if you have modified unrelated files, you may inadvertently lock these changes together, complicating future revisions.
Forgetting to Review Changes
Before committing with `-a`, always review your changes. Use the `git status` command to see what files are staged for commit and the `git diff` command to review the actual changes made. This step is crucial to avoid committing unintentional or erroneous modifications.
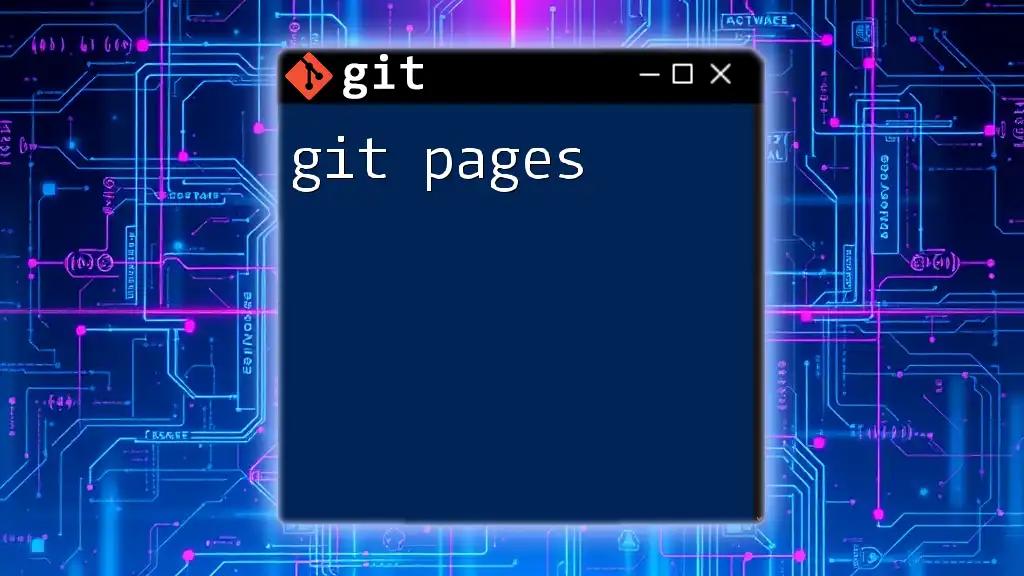
Advanced Techniques with Git -a
Combining with Other Git Commands
One of the strengths of Git is the ability to combine commands for more powerful workflows.
Using `git commit -a --amend`
This command allows you to modify the last commit, which can be useful for making small corrections. For example:
git commit -a --amend -m "Corrected commit message"
Utilizing `git commit -a -p`
Another powerful feature is the interactive patching option, which can help you choose parts of modified files to commit. This is done using:
git commit -a -p
This command enters an interactive mode where you can decide which changes to include in the commit, offering refined control over your commits.
Workflow Integrations
Integrating `git commit -a` into your daily workflow can lead to increased efficiency. It can be useful in casual coding sessions where rapid iterations are common. However, balance it with best practices related to commit structure and clarity.
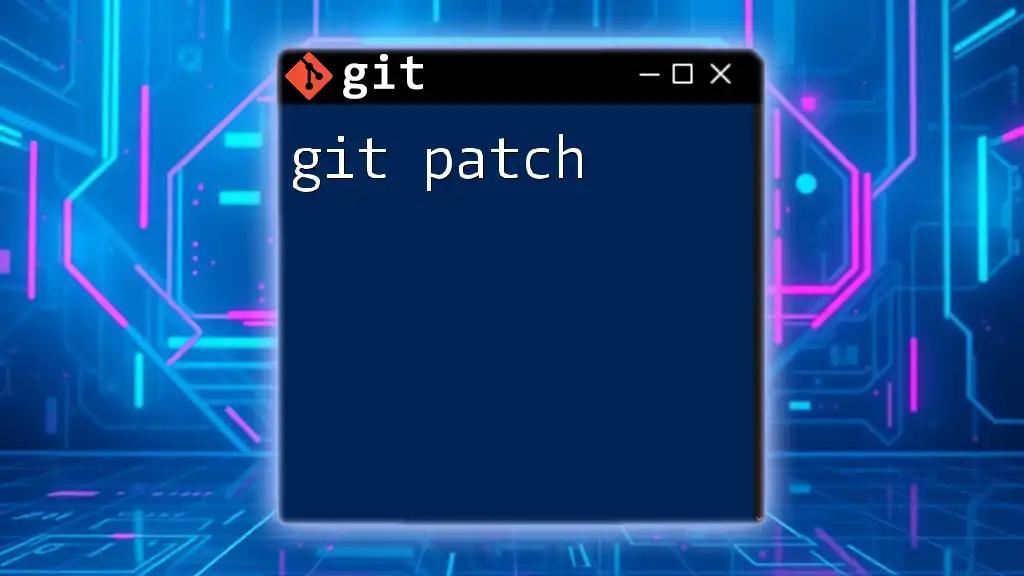
Troubleshooting Common Issues with Git -a
Unknown Errors
Sometimes, users encounter errors while using Git commands. If you receive an unknown error, check your command syntax and ensure your working directory is clean before running the commit command. Git commands often require a certain context (e.g., being in a Git-managed directory).
Dealing with Merge Conflicts
When using `git commit -a` during conflict resolution, Git will stop the commit and prompt you to resolve conflicts manually. It is crucial to address these conflicts before committing to maintain a clean history. Use tools like `git status` to identify conflicting files and resolve them accordingly.
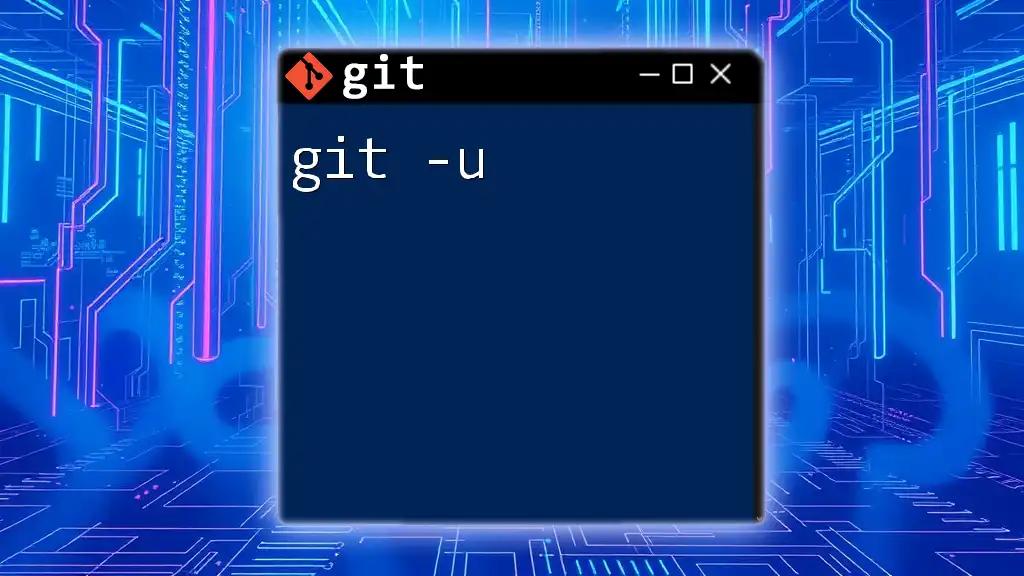
Conclusion
Mastering `git -a` is essential for efficient version control. This command streamlines the commit process, allowing you to quickly stage and commit changes. However, it is crucial to understand its implications and approach it with caution for a well-maintained project history. The ability to navigate the intricacies of Git will empower you as a developer, ensuring smooth collaboration and effective code management.
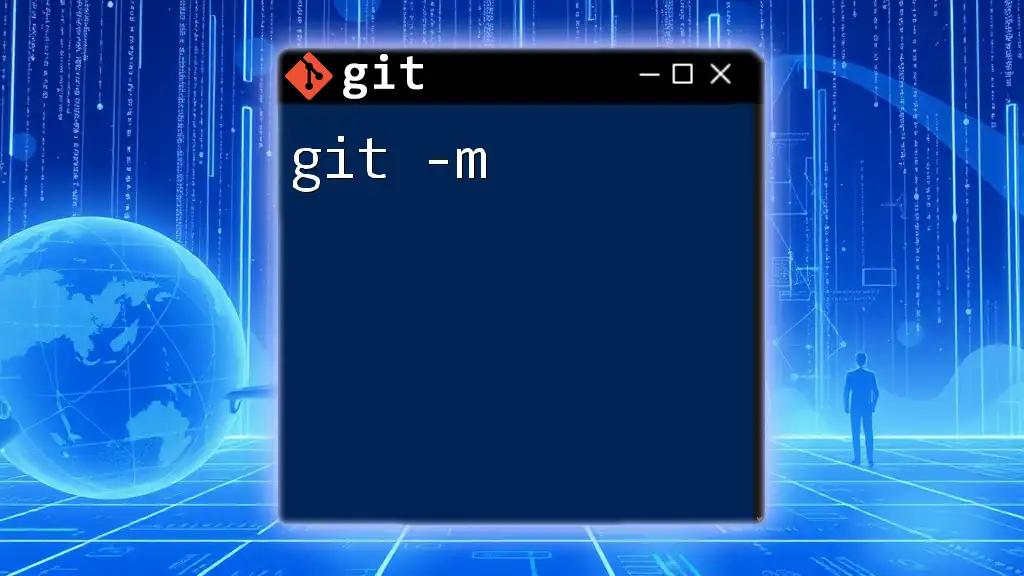
Additional Resources
For those eager to deepen their understanding of Git and the `-a` flag, plenty of resources are available. The official Git documentation is a rich treasure trove of information. Furthermore, numerous books and online courses offer structured learning paths, and community forums can provide invaluable support as you navigate your Git journey.