The best way to organize Git is to use clear naming conventions for branches, commits, and tags, ensuring that each one reflects its purpose and keeps the repository structured.
git checkout -b feature/add-user-authentication
git commit -m "Add user authentication feature with JWT"
git tag -a v1.0.0 -m "Release version 1.0.0 with user authentication"
Understanding Git Repository Structure
What is a Git Repository?
A Git repository is where Git tracks your project. It serves as a container that holds all your project files, along with the history of changes made to those files. Understanding the key components of a Git repository is essential for effective organization.
Every Git repository consists of two main directories:
- The `.git` directory, which contains all the metadata and object database for the repository (like commit history, branches, etc.).
- The working directory, where files are modified and staged for commits.
Visualizing these components helps highlight the organization needed for an efficient workflow.
Key Concepts in Git Organization
Branches and Tags
Branches are fundamental to Git’s workflow; they allow multiple versions of a project to be developed simultaneously. When creating branches, adopt descriptive naming conventions that reflect their purpose. For instance, use:
- `feature/new-user-auth` for new feature development
- `bugfix/login-issue` for bug fixes
Creating a new branch is simple:
git checkout -b feature/new-user-auth
Tags serve a different purpose: they mark specific points in the commit history, usually a release. Tags provide a convenient way to reference significant changes or milestones in your project.
Commits and History
A clean commit history is essential for future reference. Ensure that each commit message is descriptive, explaining what changes were made and why. Following formats such as:
- `feat: add user authentication`
- `fix: correct spelling in README`
helps maintain clarity. Avoid vague messages like "fixed stuff" or "update", as they do not provide context for future developers.
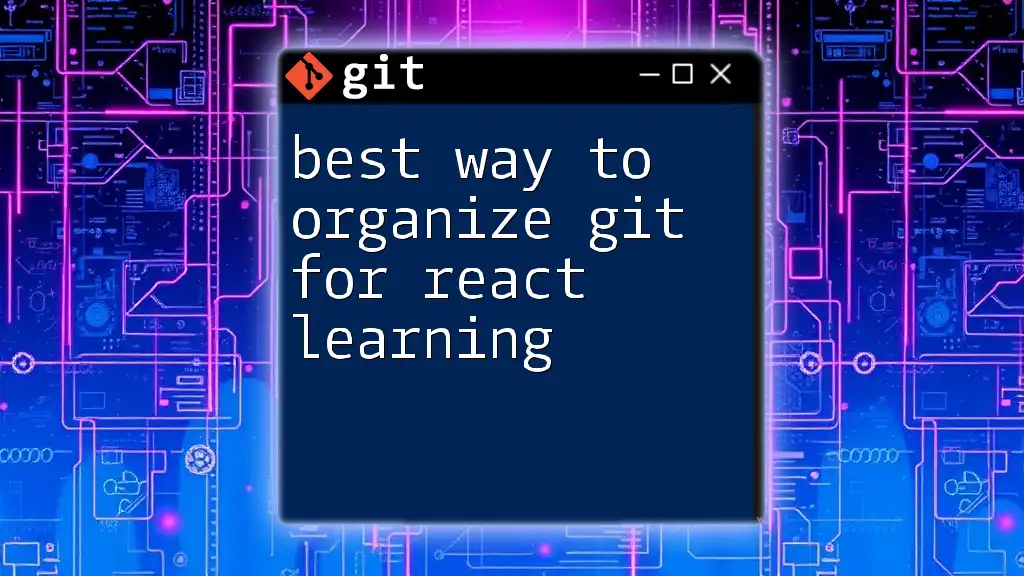
Folder Structure Best Practices
Organizing Files and Directories
A well-organized folder structure contributes significantly to project maintainability. Here is a recommended structure for a typical web application:
/my-web-app
|-- /src
| |-- /components
| |-- /styles
| |-- /views
|-- /public
|-- /tests
|-- package.json
|-- README.md
Organizing files based on their functions makes it easier for developers to locate and manage parts of the application.
Using .gitignore
The `.gitignore` file plays a crucial role in keeping the repository clean by preventing unnecessary files from being tracked. Common entries include:
# Ignore node_modules
node_modules/
# Ignore build artifacts
dist/
Including files that don't need version control reduces clutter and confusion.
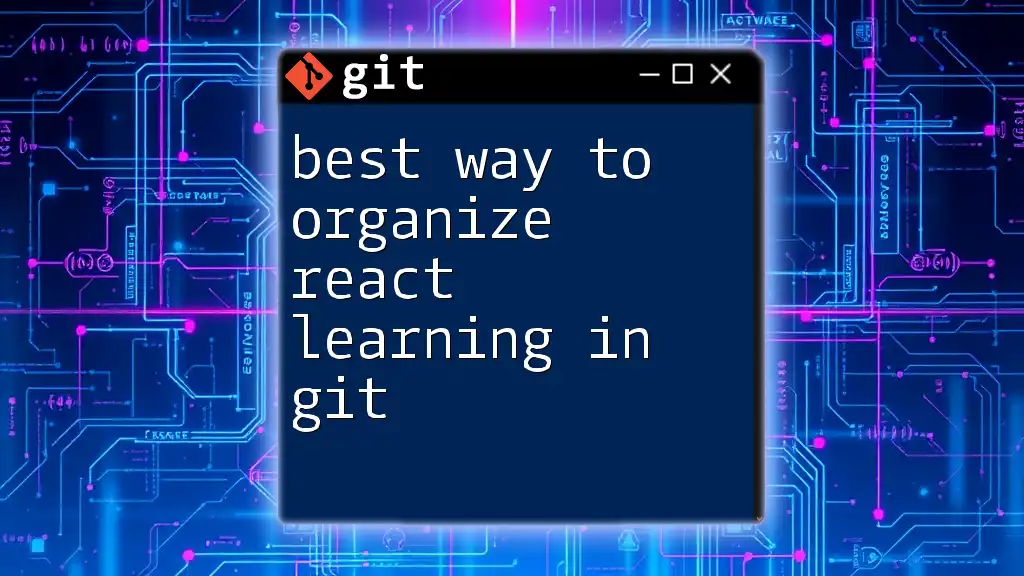
Managing Branches Effectively
Creating Branches
Branching strategy is vital for effective collaboration in Git. Create a new branch for every feature or bug fix to keep your work isolated. This practice makes merging and testing much easier. After creating a branch, ensure you switch to it promptly to start your work.
Merging and Rebasing
Understanding the difference between merging and rebasing is crucial for maintaining a clean project history. Merging combines changes from different branches, while rebasing integrates changes from one branch into another without a merge commit.
Use merging when you want to keep the history of how branches diverged:
git checkout main
git merge feature/new-user-auth
With rebasing, the goal is to create a linear project history:
git checkout feature/new-user-auth
git rebase main
Choose the method based on your team’s workflow and desired project history clarity.
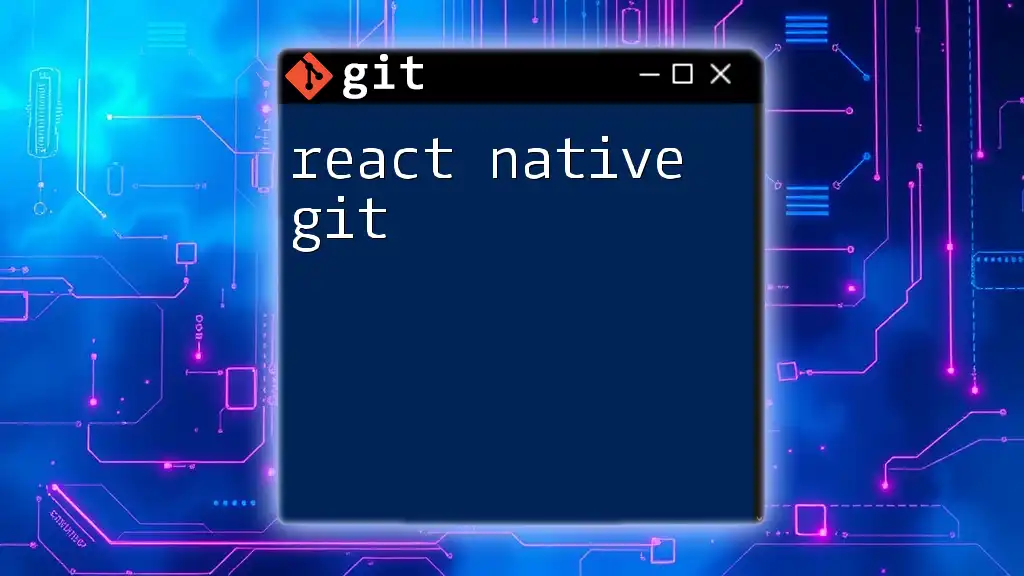
Collaboration in Git
Working with Remote Repositories
Collaborative work often occurs on remote repositories. A remote is simply a version of the repository hosted elsewhere, such as on GitHub or GitLab. Using remotes allows teams to collaborate seamlessly.
Adding a remote repository is straightforward:
git remote add origin https://github.com/username/repo.git
To share your local changes, simply push:
git push origin main
Pull Requests and Code Review
Pull requests (PRs) are vital in maintaining code quality through peer review. When opening a PR, accompany it with a descriptive summary of the changes made. This practice not only clarifies your intentions but also enhances team collaboration.
Before merging, the PR should undergo an extensive review to identify potential issues or improvements, ensuring robust and stable integration.
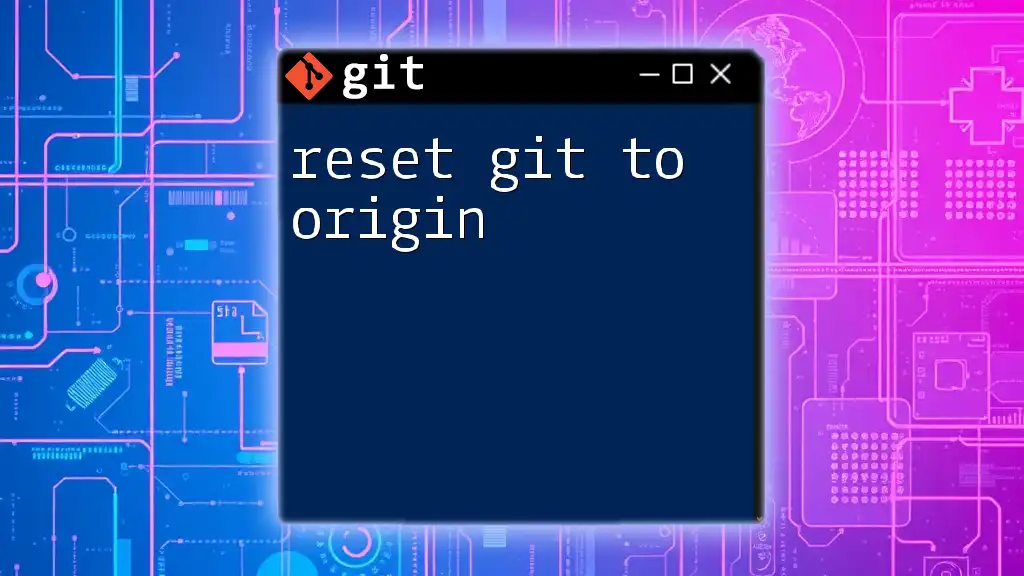
Using Git Workflows
Git Flow
The Git Flow branching model offers a structured approach to managing changes. In this model, there are defined roles for various branches:
- `main`: reflects production-ready code.
- `develop`: a staging area for features.
- `feature`: used to develop new features.
- `release`: prepares versioned releases.
This organized structure helps coordinate development and streamline releases.
Feature Branch Workflow
The feature branch workflow focuses on creating isolated branches for each feature under development. Each newly created feature branch begins from `develop` and integrates back into it once the feature is complete. This method enhances both team collaboration and version control.
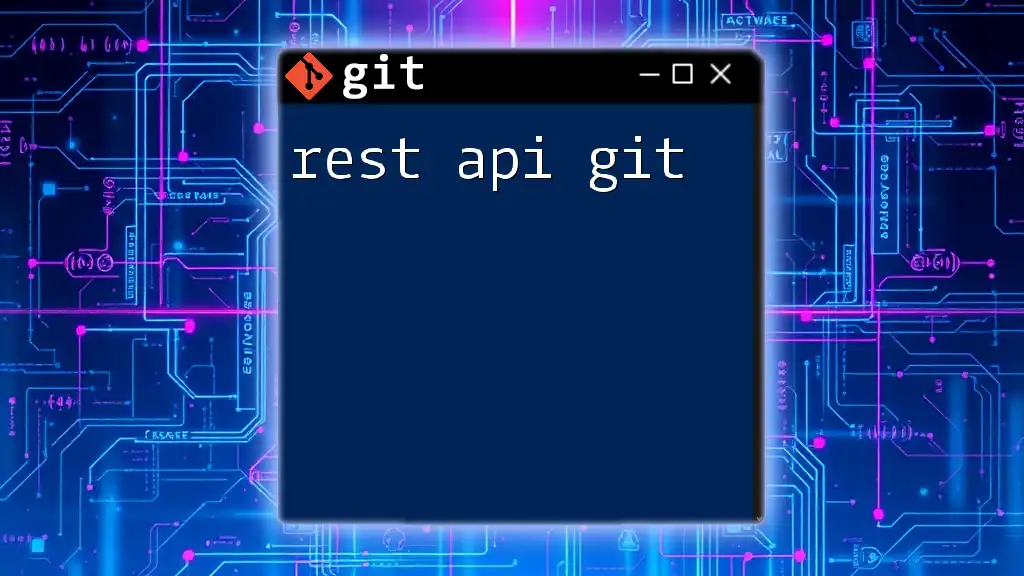
Tools and Resources
Integrating Git with Development Tools
Many development environments seamlessly integrate with Git, making version control easier. Popular IDEs such as Visual Studio Code and JetBrains products provide built-in Git tools. Learning to use these features can streamline your workflow.
Learning Resources
To further improve your Git skills, consider leveraging tutorials and courses. Comprehensive resources, including the [Git documentation](https://git-scm.com/doc) and platforms like Coursera or Udemy, can greatly enhance your understanding.
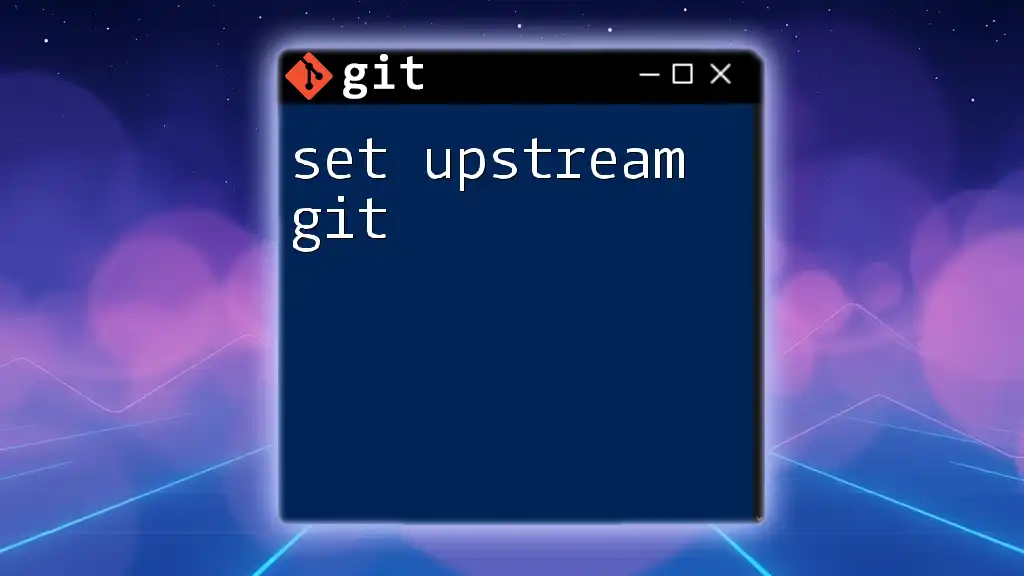
Conclusion
Organizing a Git repository correctly is crucial for any developer or team aiming to increase productivity and efficiency. From structuring files to adopting workflows and utilizing branches effectively, implementing best practices creates a smooth collaborative experience.
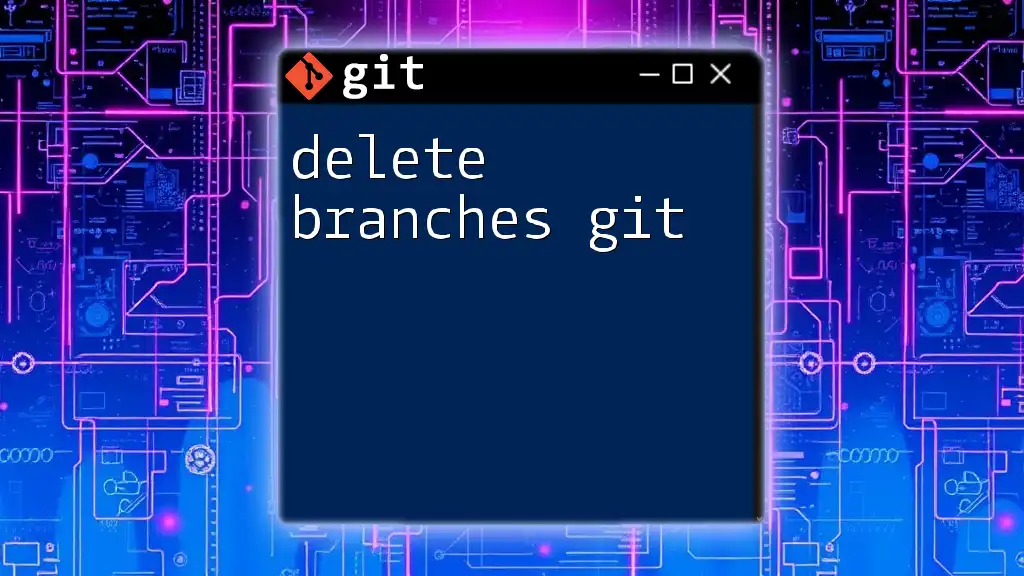
Call to Action
Feel free to comment with your thoughts, questions, or tips on the best way to organize Git! If you’re interested in mastering Git commands, check out our upcoming courses designed to boost your competencies and accelerate your development journey.