The best way to organize Git for React learning is by creating separate branches for features, bugs, and experiments, ensuring a clean workflow that facilitates collaboration and version control.
Here's a basic example of how to set up your branches:
# Create a new feature branch for the React component
git checkout -b feature/react-component
# Create a bug fix branch
git checkout -b fix/login-bug
# Create an experimental branch for trying out new ideas
git checkout -b experiment/new-design
Setting Up Your Git Repository
Creating a New Repository
To get started with the best way to organize git for React learning, you first need to create a Git repository. This process can be done easily using the command line interface. Simply navigate to your desired project directory and run the following commands:
git init my-react-app
cd my-react-app
This initializes a new Git repository in a folder called `my-react-app`. Choosing a clean and descriptive repository name will help not just you but also others who may work on the project in the future.
Using GitHub or GitLab
Using cloud-based Git services like GitHub or GitLab offers numerous benefits, including collaborative features, issue tracking, and version history browsing.
To create a new repository on GitHub, follow these steps:
- Log in to your GitHub account.
- Click on the “New” button under your repositories section.
- Fill in the repository name and description, ensuring it represents the project well.
- Make sure to choose whether it will be public or private.
Once the repository is created, you can connect your local repo to the remote one. This allows you to push and pull changes easily:
git remote add origin https://github.com/username/my-react-app.git
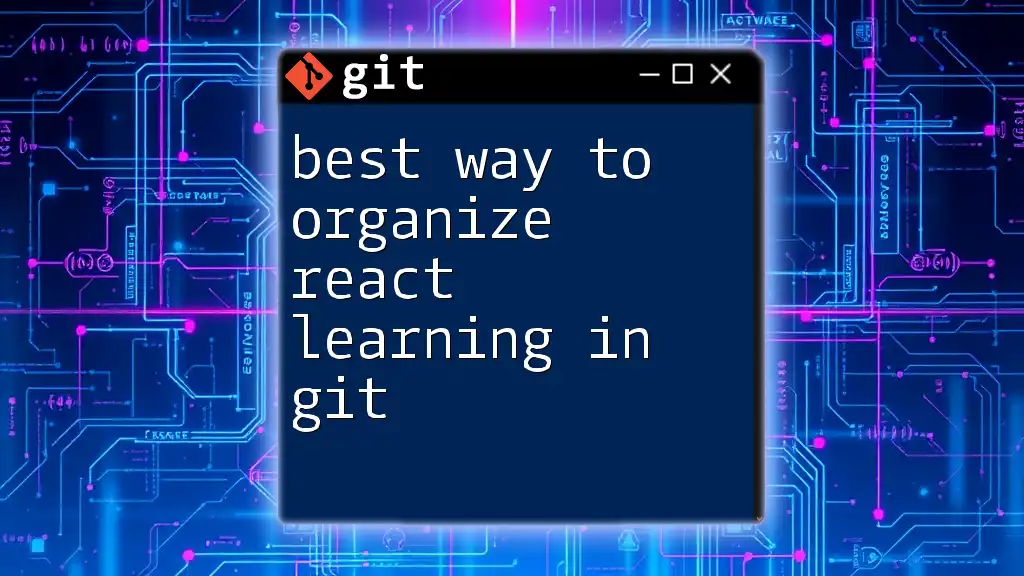
Organizing Your Git Workflow
Branching Strategy
Branches in Git allow you to work on different features or fixes in isolation. This is crucial for managing your work effectively when learning React.
Importance of Branches
Branches let you keep your codebase organized, especially when navigating through multiple features at the same time. Use them to experiment with new ideas without compromising the stability of your main application.
Recommended Branching Model
A well-defined branching model enhances code organization:
- Main Branch: This is your production-ready code.
- Development Branch: This serves as the base for all your new developments.
- Feature Branches: Each new feature should have its own branch, using names like `feature/user-authentication` to clearly identify its purpose.
To create and switch to a new branch, you can run:
git checkout -b development
Commit Messages Best Practices
Clear and concise commit messages are vital for effective collaboration and understanding your project's progress.
A good format to consider includes:
- Use the imperative mood (e.g., "Add" instead of "Added").
- If applicable, include related issue numbers for tracking.
Example Commit Messages
- Good: `Add user authentication feature`
- Poor: `Fixed stuff`
These examples illustrate the need for clarity and specificity in commit messages. Each entry should provide enough context for others (or yourself) to understand the changes made without having to read the whole diff.
Using Tags for Versioning
Tags in Git are akin to version markers. They are essential for marking release points, simplifying the identification of specific versions of your project.
To tag a release version, you could use the following command:
git tag -a v1.0 -m "Release version 1.0"
Committing Your React App
Having a clear understanding of what files to commit is essential for an organized repository. In general, ensure to commit all source files relevant to your project while avoiding unnecessary clutter.
Remember: It’s best practice to exclude certain directories or files, like `node_modules` or build artifacts, from being tracked by Git. You can accomplish this with a `.gitignore` file:
node_modules/
build/
.env
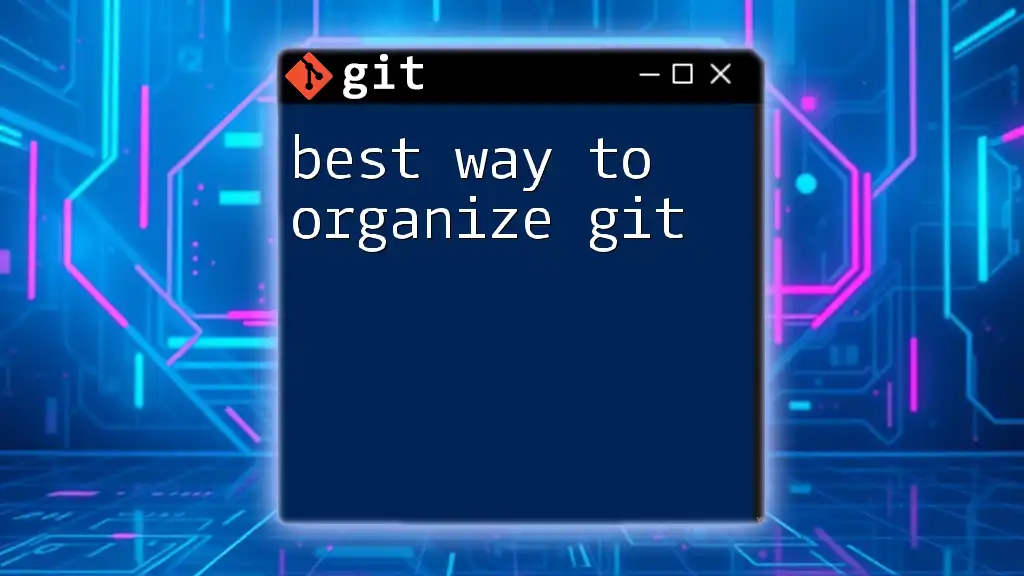
Structuring Your React Project with Git
Directory Structure
To facilitate efficient development, especially when learning React, maintaining a standardized directory structure is key. Here is a recommended layout for your React app:
my-react-app/
├── public/
├── src/
│ ├── components/
│ ├── hooks/
│ ├── utils/
│ └── App.js
├── .gitignore
├── package.json
└── README.md
This structure helps in separating different aspects of your application, making it easier for others to navigate the codebase and for you to maintain it over time.
Collaborating with Others on React Projects
Collaboration is often a critical part of the learning process, especially in coding.
Pull Requests
Pull requests (PRs) are essential for collaborative work. They not only facilitate discussions about the changes made but also serve as a review process before merging code.
To create a PR on GitHub:
- Push your feature branch to the remote repository.
- Go to the GitHub repository page and you will usually see an option to create a pull request from your branch.
Code Reviews
The code review process is crucial for maintaining code quality. During a code review, team members can examine changes, share insights, and suggest improvements.
Using GitHub's review features can streamline this process, allowing easy commenting directly on the changes made.
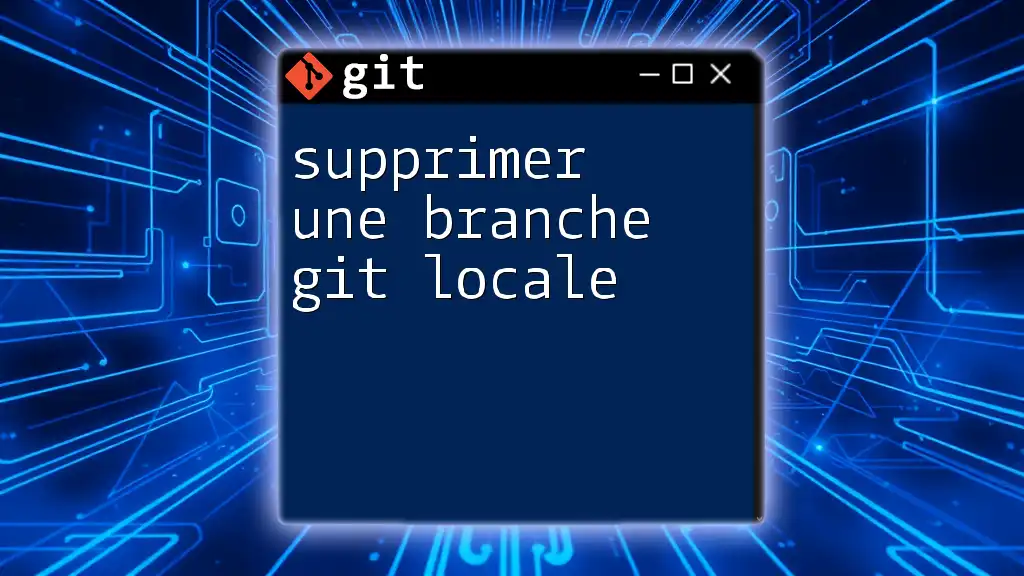
Advanced Git Techniques for React Developers
Stashing Changes
Sometimes, you may find yourself in the middle of work and need to switch branches or tasks. The `stash` command in Git lets you temporarily save your changes so you can work on something else without committing broken code.
To stash your changes, simply run:
git stash
You can retrieve those stashed changes later using:
git stash apply
Rebasing vs Merging
Understanding the differences between merging and rebasing is essential for maintaining a clean project history:
- Merging combines changes from one branch to another but retains the individual branch history.
- Rebasing integrates the changes in a linear fashion, creating a cleaner project history.
Example of Merging:
git merge development
Example of Rebasing:
git rebase development
Both methods have their pros and cons, so it’s beneficial to choose the one that aligns with your team's workflow preferences.
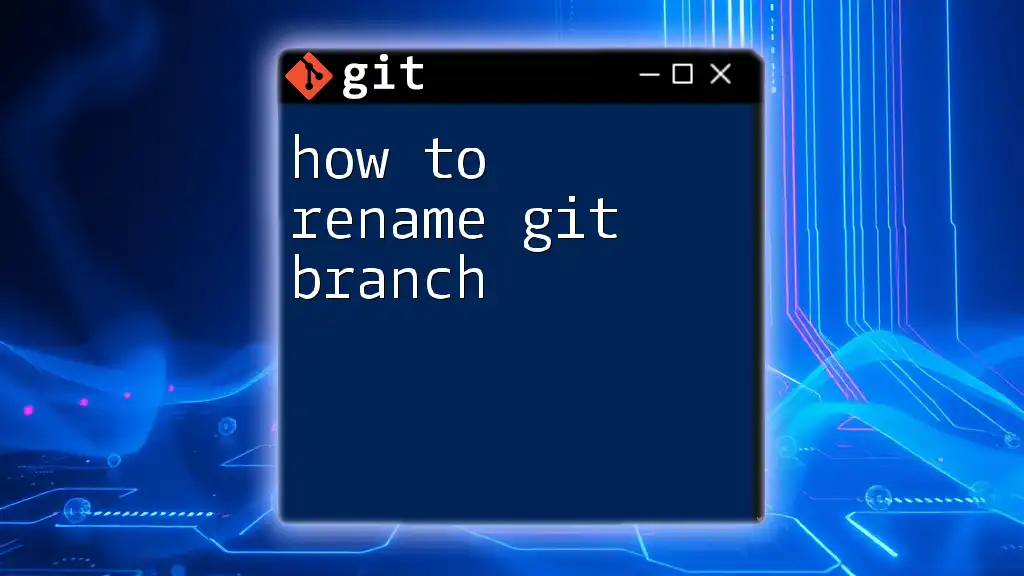
Conclusion
Organizing Git for your React learning experience is essential for improving both your individual and collaborative coding practices. By following the practices outlined — from setting up your repository correctly to adopting effective branching strategies — you will pave the way for a productive coding environment.
Remember, consistency is key. As you grow in your React journey, maintaining this organized structure will not only enhance your learning but also prepare you for real-world development scenarios. Happy coding!
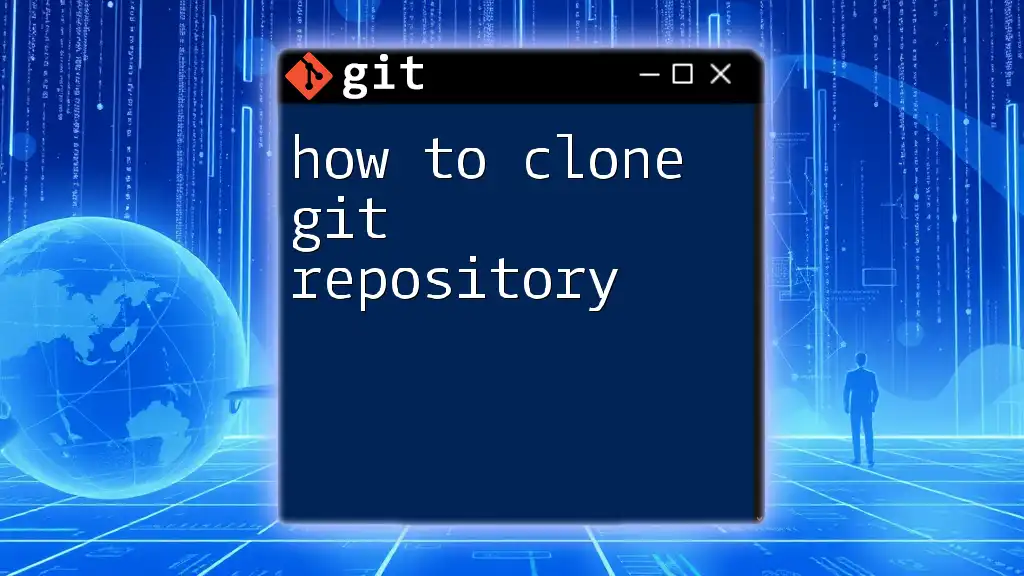
Additional Resources
For further reading and tools to perfect your Git use, refer to the official Git documentation and explore various online courses dedicated to learning Git with React. Understanding these concepts deeply will enrich your programming skills and make the process significantly more efficient.