To ignore Java files in a Git repository, you can add a specific entry to your `.gitignore` file using the following syntax:
# Ignore all .java files
*.java
Understanding Git Ignoring Mechanisms
What is a `.gitignore` file?
A `.gitignore` file is a fundamental aspect of Git that defines which files or directories should be ignored by version control. By specifying these files, you can prevent unnecessary files—such as build artifacts, temporary files, and other non-essential items—from being tracked by Git, helping you maintain a clean and organized repository.
How Does `.gitignore` Work?
Git reads the `.gitignore` file at the root of your project to determine which files should be excluded from tracking. It employs a set of pattern-matching rules that allow you to define which files to ignore based on their names and extensions. For example, using `*.class` tells Git to ignore all files with the `.class` extension.
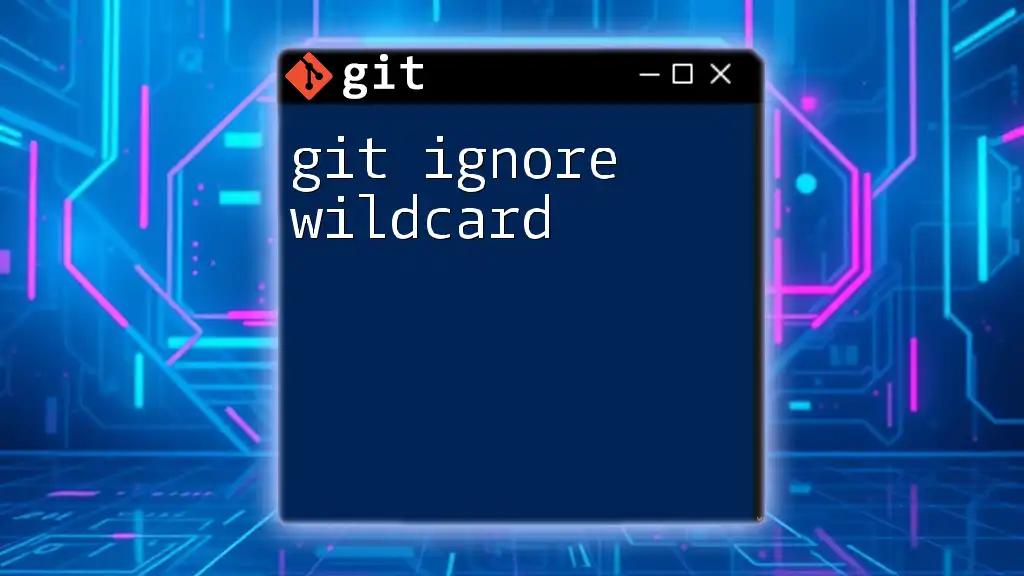
Setting Up Your `.gitignore` File
Creating a `.gitignore` File
To get started, you need to create a `.gitignore` file in your Java project. This can be accomplished simply by running the following command in your terminal:
touch .gitignore
Once you have created the `.gitignore` file, it will reside in the root directory of your repository, ready for you to specify which files and directories to ignore.
Common Patterns for Java Projects
When working on Java projects, there are several common file patterns that should be included in your `.gitignore` file. These patterns typically cover files generated during compilation and packaging processes:
- `*.class`: Compiled Java bytecode files, which are automatically generated and not needed in version control.
- `*.jar`: Java Archive files, used for packaging multiple Java classes and resources into a single file.
- `*.war`: Web Application Archive files, which are similar to JAR files but specifically meant for web applications.
Here is how you would include these entries in your `.gitignore` file:
*.class
*.jar
*.war
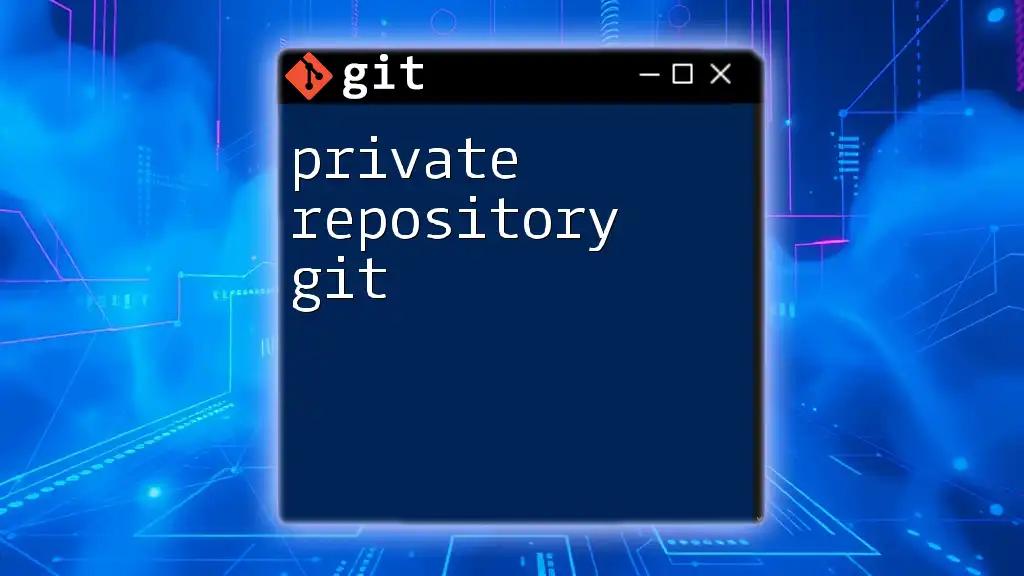
Ignoring Specific Java Files
Ignoring Build and Dependency Files
Java projects commonly use build tools like Gradle and Maven, which generate their specific files and directories during the build process. It’s crucial to exclude these from your Git repository to keep it clean:
- Gradle: You might want to ignore the `.gradle/` directory and any build-related outputs. Here’s an example of what your `.gitignore` might look like for a Gradle project:
/.gradle/
/build/
- Maven: Maven projects generate a `target/` directory where compiled files and other build artifacts are stored. To ignore this, add the following line to your `.gitignore`:
/target/
Excluding Temporary and Configuration Files
When using IDEs such as IntelliJ IDEA or Eclipse, numerous temporary files and project configuration files are automatically generated. These files should also be ignored to prevent clutter in your repository:
.idea/
*.iml
.classpath
.project
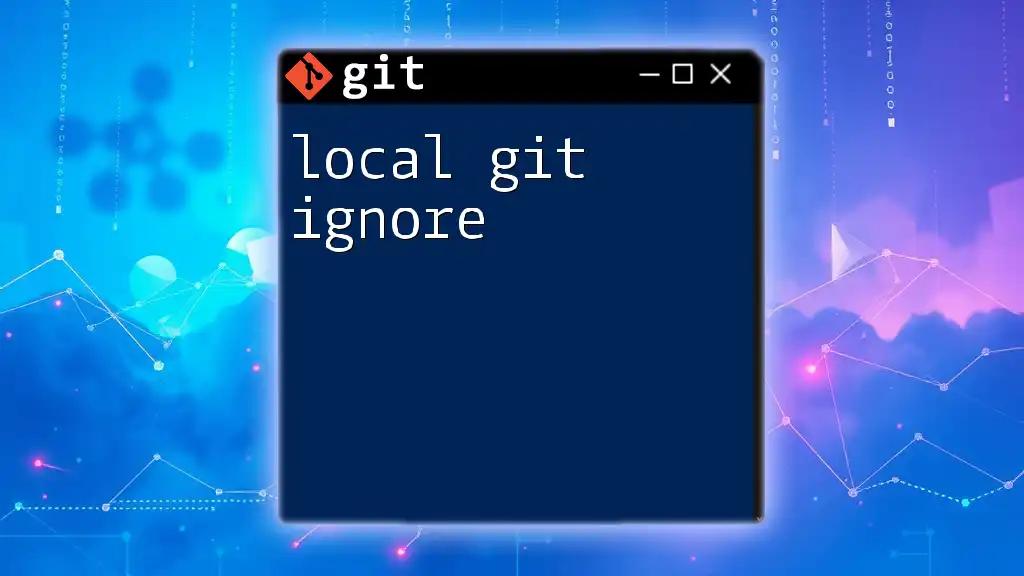
Best Practices for Writing `.gitignore`
Keeping Your `.gitignore` Organized
A well-organized `.gitignore` file enhances readability and usability. Consider grouping related patterns together, and use comments to separate different sections. For instance, you might have a section for build files, another for IDE files, and so on:
# Build Files
*.class
/*.gradle/
/build/
/target/
# IDE Files
.idea/
*.iml
.classpath
.project
Regularly Updating Your `.gitignore`
As your project grows and evolves, it’s important to keep the `.gitignore` file updated. Regularly reviewing and modifying this file allows you to adapt to new file types or patterns that should be ignored, ensuring that your repository remains clean.
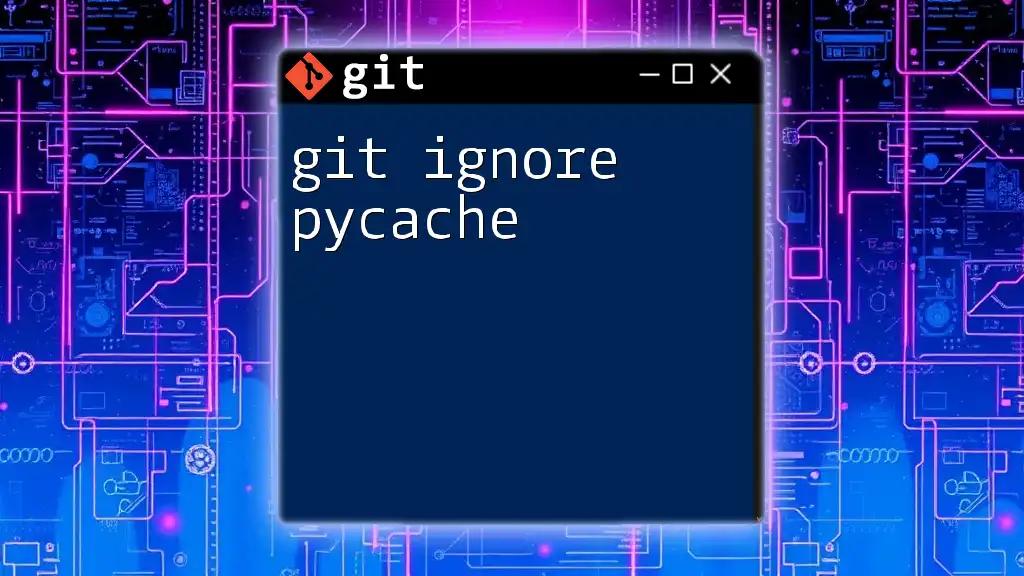
Advanced Usage of `.gitignore`
Conditional Ignoring
Advanced pattern matching allows you to specify exceptions, so you can ignore a certain type of file but still include a specific one. This is done using negation patterns. For example:
# Ignore everything
*
# Except for the important file
!important-file.java
This configuration will ignore all files except for `important-file.java`, which will be tracked by Git.
Ignoring Files in Specific Directories
If you want to ignore files within a specific directory while still tracking files elsewhere, you can specify directory-specific rules. For example, to ignore all `.log` files in a directory named `logs`, you would add:
# Ignore all .log files in the logs directory
logs/*.log
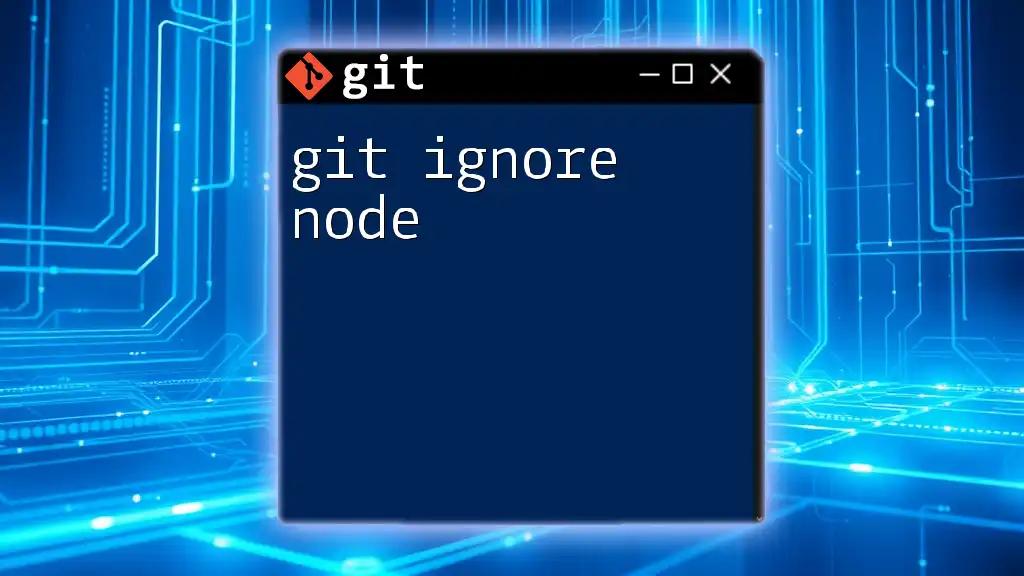
Troubleshooting `.gitignore` Issues
Files Not Ignored
Sometimes, files that you expect Git to ignore still show up in the staging area. This often happens because those files were tracked by Git before you added them to your `.gitignore` file. To resolve this, you must untrack the file by running:
git rm --cached <file>
This command removes the file from the index while keeping it in your working directory.
Validating `.gitignore` Properly
To ensure your `.gitignore` file works as expected, consider using tools like `git check-ignore`, which can help you test and validate that your rules are functioning correctly.
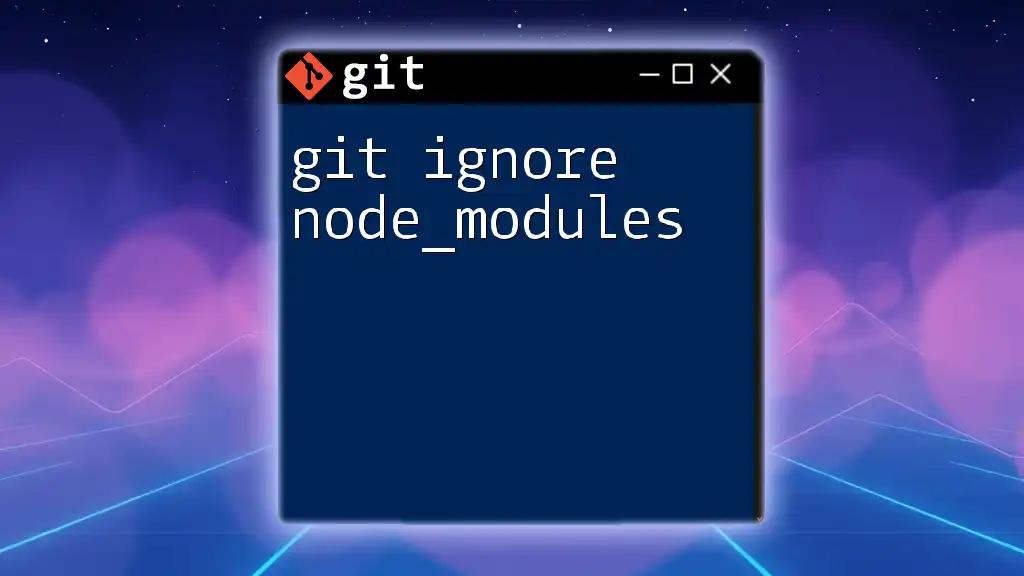
Conclusion
The Importance of a Well-Maintained `.gitignore`
Having a robust and well-organized `.gitignore` file is essential for any Java project. It not only keeps your repository clean but also maximizes developer efficiency by minimizing unnecessary clutter.
Call to Action
Take the time to tailor your `.gitignore` file to meet your project's specific needs. Share your experiences and tips in the comments, and let's create a helpful community around mastering Git!