Before you can switch branches or perform other Git operations, you need to resolve any changes or conflicts in your current index by either staging, committing, or stashing them.
To view the status of your current index, use the following command:
git status
What is a Conflict in Git?
A conflict in Git occurs when changes from two or more branches cannot be automatically merged due to overlapping modifications. This situation arises when you attempt to combine branches that have diverged, introducing competing changes to the same lines of code or even entire files.
For example, consider the following scenario:
- You have `master` and `feature` branches.
- You make changes to the same line of a file in both branches.
- When you try to merge `feature` into `master`, Git is unable to determine which change to keep, resulting in a conflict.
When Do Conflicts Occur?
Conflicts can arise during several common actions in Git:
-
Merging branches: When you merge two branches with diverging changes, Git tries to combine them. If it encounters conflicting changes, it raises a conflict.
-
Rebasing branches: When you rebase a branch onto another, Git rewrites commits. If any commits conflict with the parent branch, they need to be resolved.
-
Pulling changes from a remote repository: If your local branch has uncommitted changes and you try to pull updates from the remote, conflicts can occur.
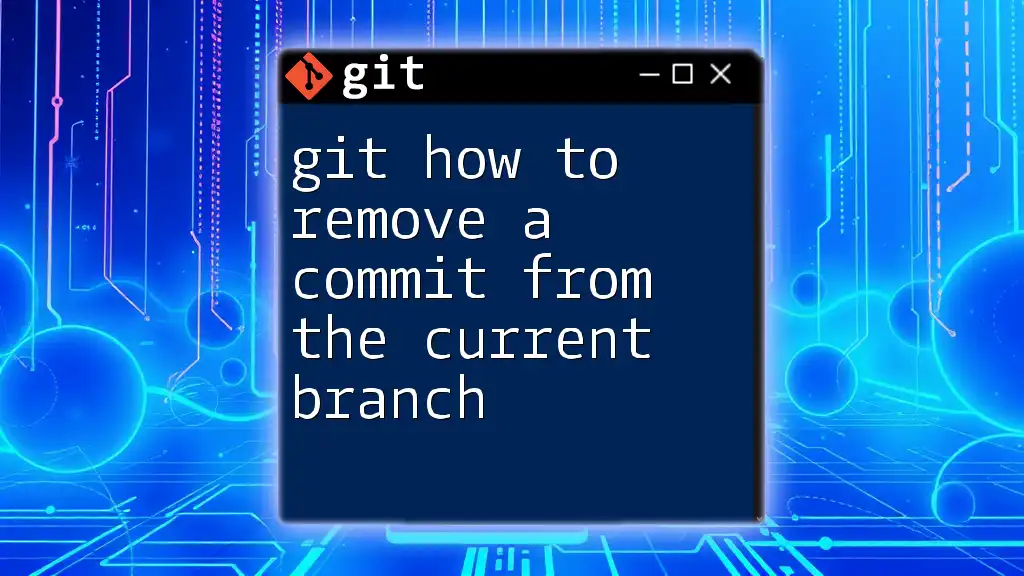
Resolving Conflicts
Importance of Resolving Conflicts
Resolving conflicts is critical before moving forward with your workflow. Ignoring these conflicts can lead to inconsistent states in your project, errors during the build process, and long-term maintenance nightmares. By taking the time to resolve conflicts right away, you maintain a clean and functional codebase.
Steps to Resolve Conflicts
Identify
The first step in resolving conflicts is to identify which files are affected. You can do this by running:
git status
This command will display a list of files that have conflicts marked as "unmerged."
Manual Conflict Resolution
When you open a file with conflicts, Git marks the conflicting sections with specific markers:
<<<<<<< HEAD
Your changes
=======
Changes from the other branch
>>>>>>> feature-branch
To resolve the conflict, you must edit the file:
- Choose which changes to keep.
- Merge changes from both branches if necessary.
- Remove the conflict markers.
Utilizing a text editor, either a basic one like Notepad or an Integrated Development Environment (IDE), can help you navigate and resolve these conflicts smoothly.
Using Git's Merge Tools
For more complicated conflicts, using a merge tool can simplify the process. Many IDEs come with built-in merge tools, but you can also configure your favorite tool. For example:
git config --global merge.tool meld
Once configured, run:
git mergetool
This launches the merge tool, allowing you to visually resolve conflicts more easily.
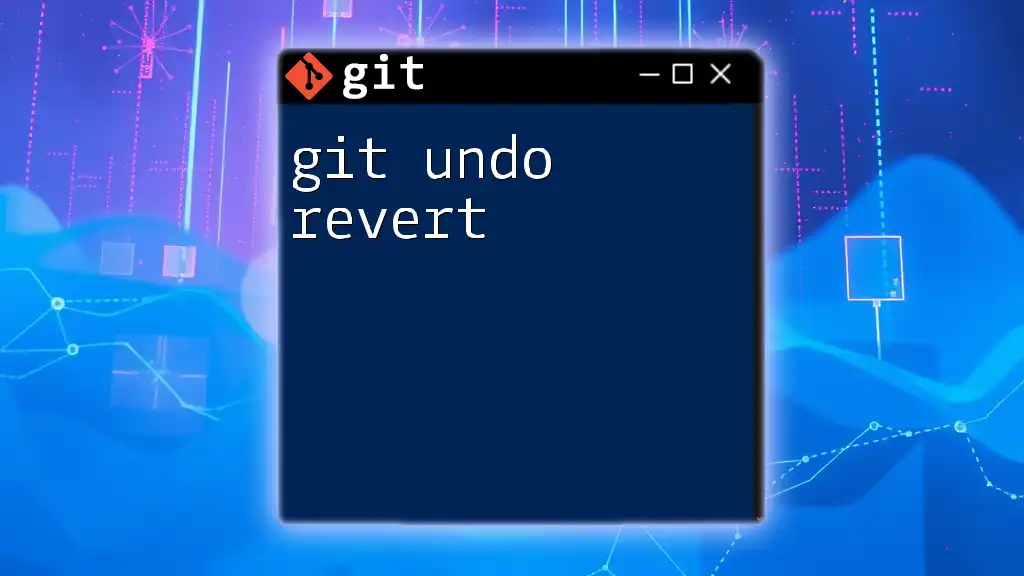
Committing Resolved Changes
Once you've successfully resolved conflicts, the next step is to stage the resolved files. Use the following command to add them to the staging area:
git add <file>
Now that your changes are staged, it's time to commit them:
git commit -m "Resolved merge conflict in <file>"
This commits your changes, including the conflict resolutions, effectively closing the merge and updating your history.
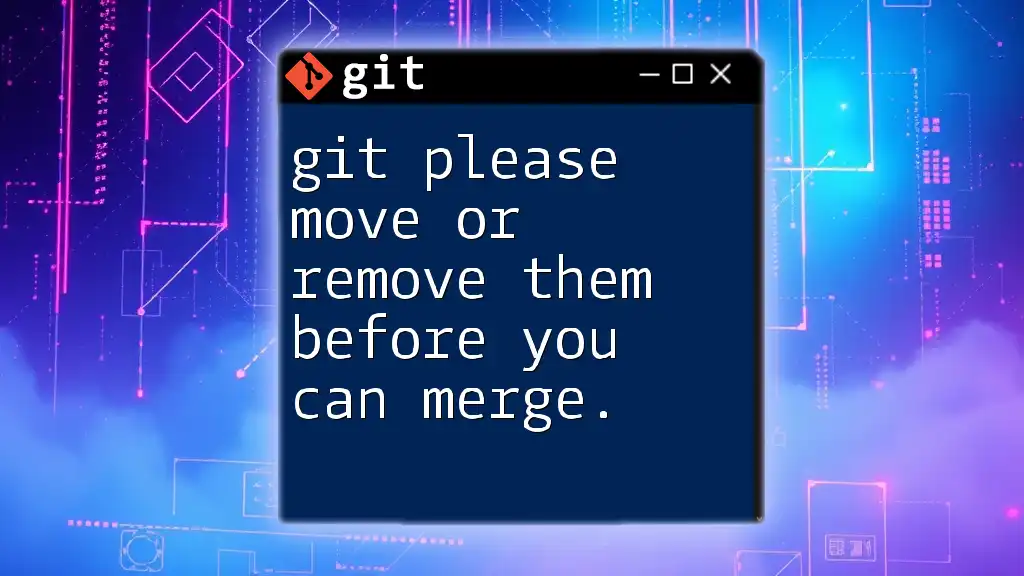
What If You Need to Abort?
Sometimes, resolving conflicts can become complex, and you may decide it's best to abort the merge altogether. If you find yourself in this situation, you can use the following command:
git merge --abort
This command will revert your working directory and index to the state before the merge attempt, allowing you to reassess your approach. It's an essential command to know when the merge process becomes unmanageable.
Understanding the Implications
Using `git merge --abort` means that any changes you made during the conflict resolution process will be lost. However, it allows you to reset your progress and start over without the complications introduced by unresolved conflicts.
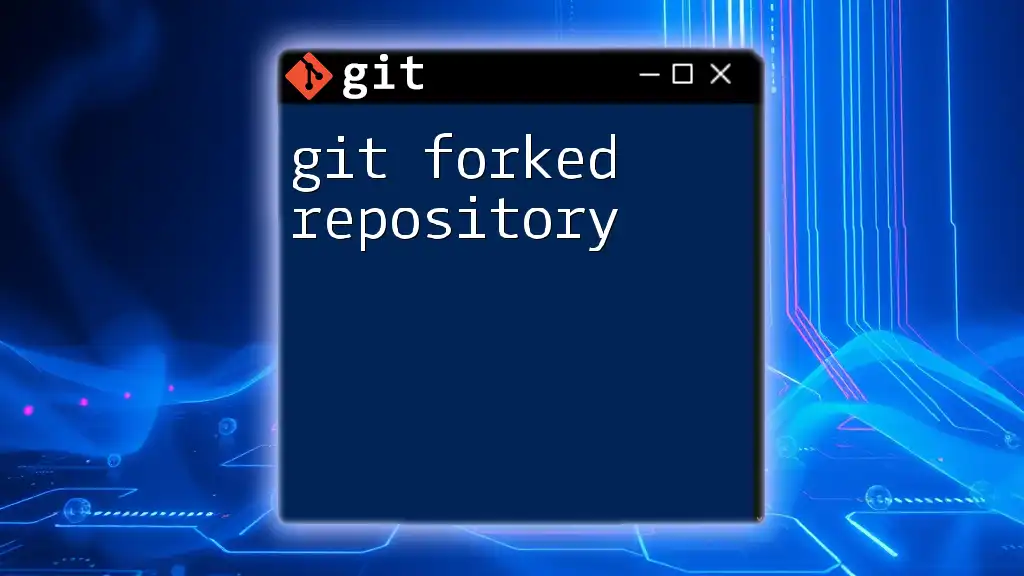
Preventing Future Conflicts
Best Practices
To minimize the likelihood of future conflicts, consider the following best practices:
-
Frequent commits and pulls: Regularly committing code changes and pulling updates from the remote repository reduces the risk of significant divergences.
-
Keeping your branches up-to-date: Regularly fetching and merging changes from the base branch helps you stay in sync with the rest of the team.
Use of Feature Branches
Utilizing feature branches can aid in avoiding conflicts. By encapsulating changes related to a single feature or bug fix in its own branch, you can work independently from the main code line. This approach not only helps keep the code organized but also reduces the risk of running into conflicts when merging back into the main branch.
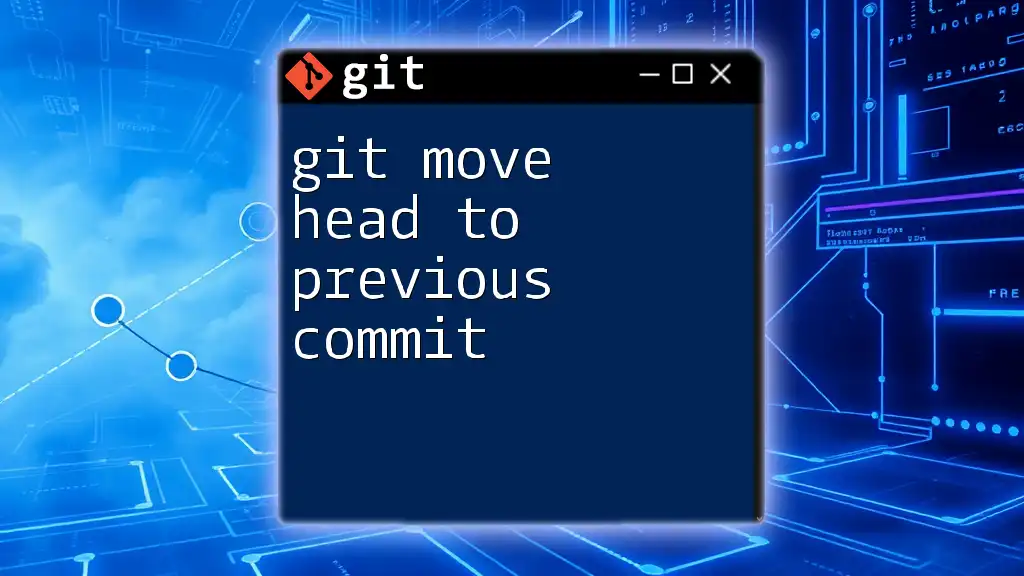
Conclusion
In summary, understanding how to address conflicts in Git is crucial for maintaining a smooth and efficient workflow. By following best practices and resolving conflicts thoughtfully when they arise, you foster a more manageable codebase. Practicing these techniques will help you become more proficient with Git and empower you to work collaboratively without the disruption of unresolved conflicts.
For additional resources and further reading on Git commands and workflows, consider checking out the official Git documentation and online tutorials tailored to your learning style.