Git offers superior performance, flexibility, and decentralized collaboration compared to SVN, allowing developers to work offline and manage their code efficiently.
Here's a code snippet to demonstrate how to clone a repository in Git:
git clone https://github.com/username/repository.git
Understanding Version Control Systems
What is a Version Control System?
A version control system (VCS) is a software tool that helps individuals and teams manage changes to code and documents over time. It enables developers to track revisions and collaborate efficiently. Common examples of version control systems include Git, SVN (Subversion), Mercurial, and Perforce.
Overview of SVN (Subversion)
SVN, or Subversion, was developed in 2000 as a centralized version control system. Key features of SVN include:
- Central Repository: All versions are stored in a single central location.
- File Locking: Prevents simultaneous edits by multiple users on the same file.
- Binary File Support: Handles binary files well, which can be an advantage in certain contexts.
While SVN suits many workflows, it has limitations that Git addresses effectively.
Overview of Git
Git, created by Linus Torvalds in 2005, is a distributed version control system that allows for a more flexible and robust approach to managing code. Key features include:
- Distributed Architecture: Each user has a complete copy of the repository on their local machine.
- Powerful Branching and Merging: Enables flexible development workflows.
- Lightweight Commits: Fast and efficient commit operations, with self-contained history tracking.
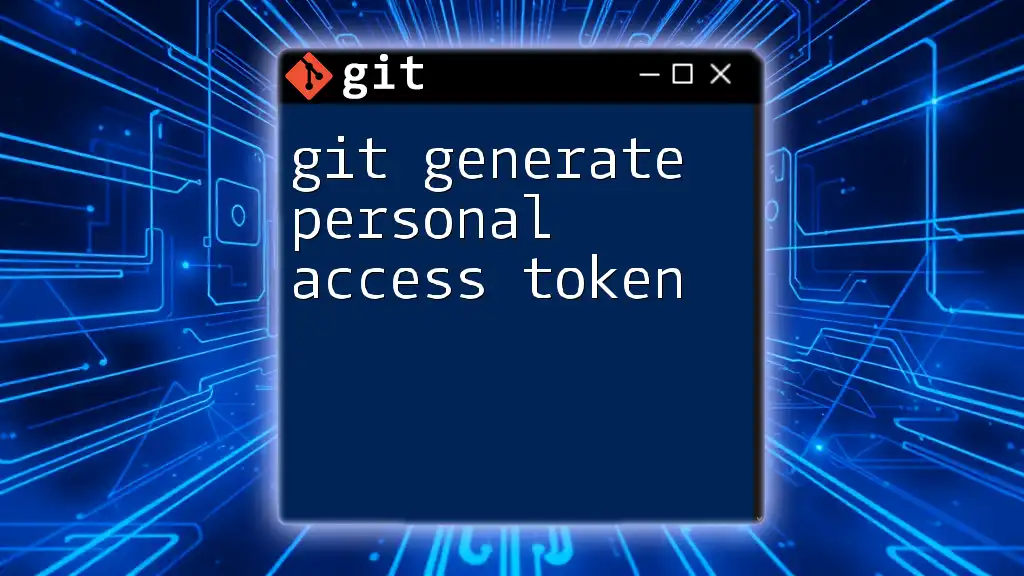
Key Benefits of Git Over SVN
Distributed Version Control
One of the most significant advantages of Git over SVN is its distributed nature. In SVN, developers rely on a central repository, which can lead to bottlenecks and accessibility issues. In contrast, Git allows each user to have their own local repository. This means:
- Users can work offline and commit changes locally, which promotes productivity without a constant internet connection.
- Everyone can experiment freely with features and changes in their local copy until they decide to push to the shared repository.
Code Snippet: Cloning a repository
git clone <repository-url>
With this command, users create a local copy of the repository, allowing them to work without impacting others until they choose to share their changes.
Speed and Performance
Git excels in speed due to its decentralized model. Many operations are performed locally rather than requiring a network call to a central server. This results in:
- Faster Operations: Actions such as branching, merging, and committing are significantly quicker in Git. Operations that would involve frequent communication with the server in SVN can be executed almost instantly in Git.
Examples of Speed Gains Imagine running a commit in Git versus SVN; the former completes nearly instantly since it operates on local data, while the latter may require communicating with the server, which can be time-consuming.
Code Snippet: Speed test comparison
time git commit -m "example commit"
By timing the performance of a commit, one can appreciate the speed that Git provides.
Branching and Merging
In Git, branching is not just encouraged; it’s a core feature designed for efficient workflows. Unlike SVN, where branching can be cumbersome and slow, Git allows for:
- Flexible Branching Model: Developers can quickly create, manage, and delete branches without considerable overhead. This encourages experimentation without affecting the main codebase.
- Seamless Merging: With powerful built-in tools, merging branches in Git is often simpler and can be automated to resolve conflicts.
Code Snippet: Creating and switching branches
git checkout -b new-feature
git checkout master
This command illustrates how simple it is to create a new feature branch and then switch back to the master branch, significantly enhancing productivity.
Data Integrity with Git
Git places a strong emphasis on maintaining data integrity. It utilizes a mechanism called SHA-1 hashing to ensure that every change to the repository is trackable and secure. Each commit creates a unique identifier based on the contents, meaning:
- Data Integrity Checks: Changes can be verified against this identifier, preventing data corruption.
- Revisitable History: Since the commit data is immutable and securely tracked, reverting to previous versions is straightforward and safe.
Better Support for Non-linear Development
Git fosters non-linear workflows which are essential for modern development practices. Developers may find themselves needing to implement new features, fix bugs, and work on different versions of the product simultaneously. In Git, this is easily managed because:
- Feature Branches: Developers can create isolated branches for feature development or bug fixes, which can later be merged back into the main codebase.
Visualization of Commit Graphs Git allows you to visualize commit history in a branching fashion, highlighting contributions from multiple developers. This is particularly beneficial in teams where multiple features are developed simultaneously.
Code Snippet: Viewing commit history
git log --graph --oneline
This command generates a visually appealing representation of your project's commit history, making it easy to track progress.
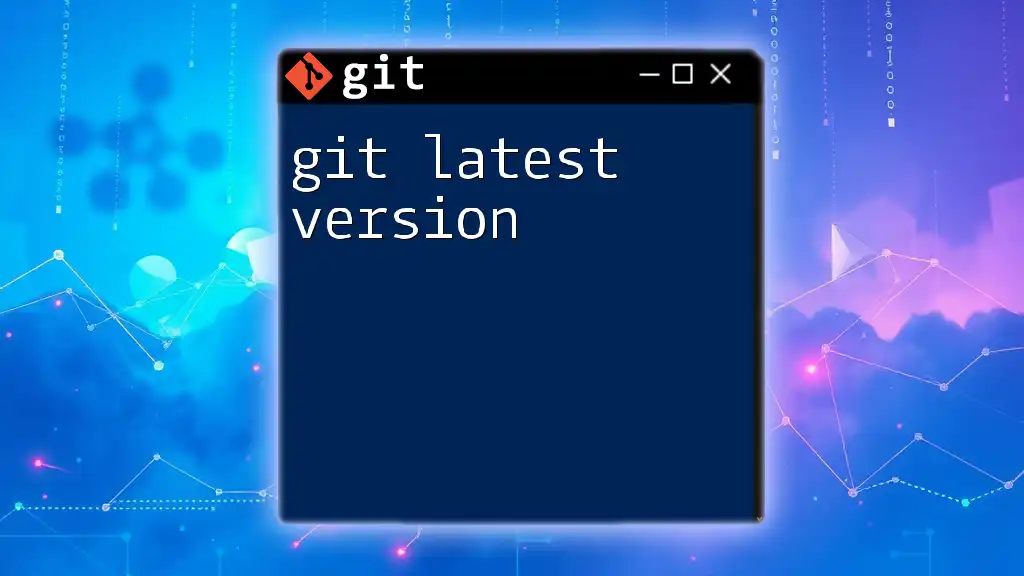
Enhanced Collaboration Features
Pull Requests and Code Reviews
A cornerstone of Git's collaborative features is the concept of pull requests. Pull requests facilitate smooth code reviews by allowing team members to propose changes, discuss them, and request feedback before integration. This process ensures:
- Quality Control: Code gets reviewed by peers before being merged, leading to fewer bugs and cleaner code.
- Transparent Collaboration: Everyone in the team can see proposed changes and provide insights.
Code Snippet: Creating a pull request on GitHub
Click on "New Pull Request" on your repository page
This simple action initiates a collaborative discussion around code changes and fosters teamwork.
Easier Conflict Resolution
Git comes with robust tools for handling merge conflicts that can often arise when multiple developers are working on the same files. The built-in conflict resolution tools in Git help:
- Identify and Manage Conflicts: When there are conflicting changes, Git presents the differences clearly, allowing developers to choose which changes to keep.
- Integrated Sorting Tools: These tools can help resolve conflicts smoothly, allowing both editors' changes to be preserved if desired.
Code Snippet: Example of resolving a conflict
git mergetool
This command activates Git's merge tools, providing developers with a graphical or command-line interface to resolve conflicts effectively.
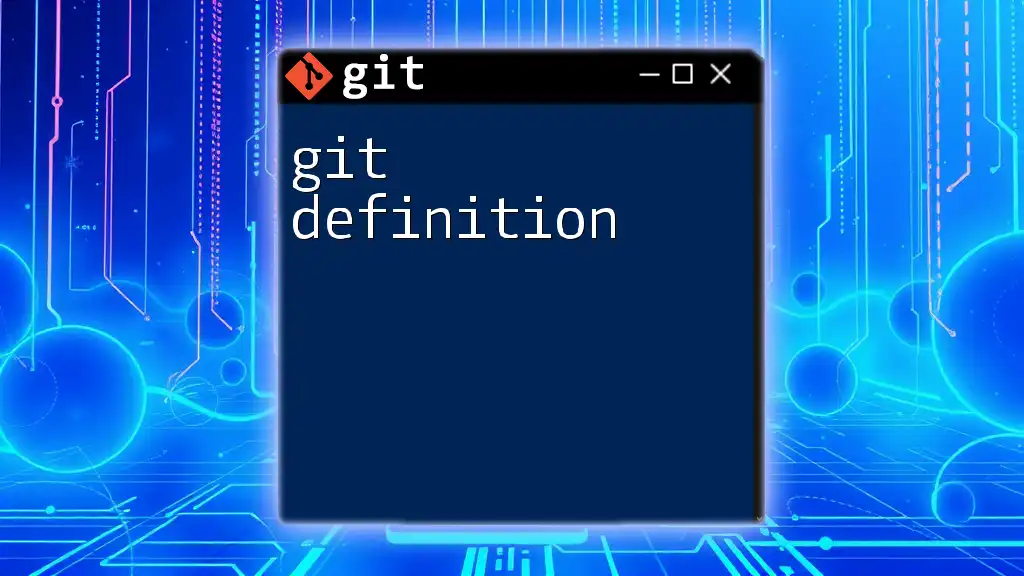
Conclusion
The git benefits over svn are substantial, providing developers with a flexible, fast, and reliable version control experience. From distributed repositories and efficient branching to powerful collaboration features, Git encompasses everything modern development needs. Transitioning from SVN to Git may seem daunting, but the advantages in speed, efficiency, and collaborative potential make it a worthwhile endeavor. Consider taking the leap into Git and embrace its robust features for your development projects.
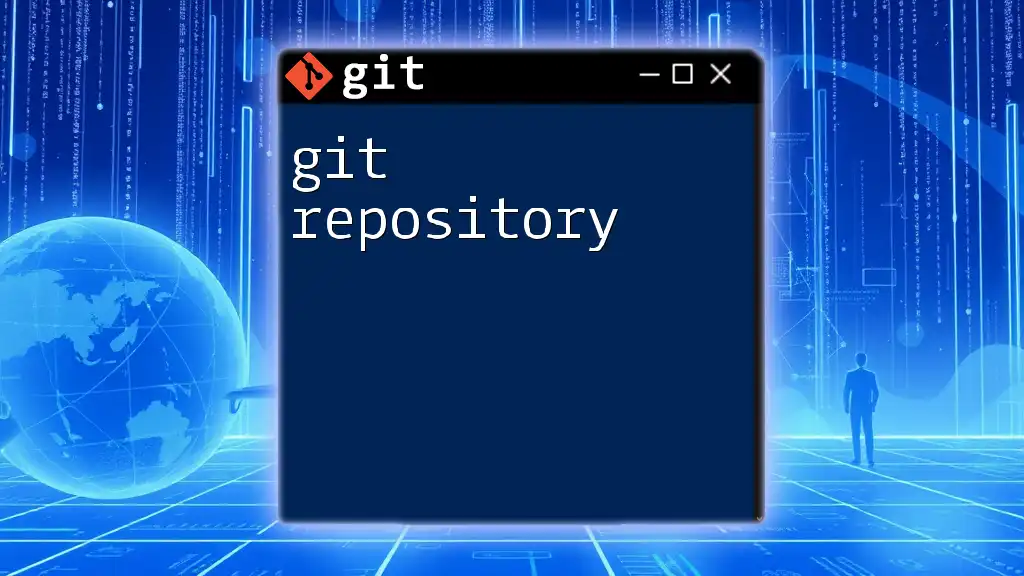
Additional Resources
For further learning about Git, consult the official Git documentation and consider diving into tutorials that enhance your understanding of its complex features. Additionally, keep an eye out for upcoming workshops or courses designed to teach Git effectively, ensuring you master this invaluable tool for version control.