The Git user API allows users to configure their identity in Git by setting their name and email, which are used in commit messages.
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
What is the Git User API?
The Git User API is a powerful interface that allows developers to automate and manage Git repositories through programmatic commands. This API provides various functionalities essential for handling repository activities, such as creating, modifying, and managing repositories with ease. Understanding the Git User API is crucial for anyone looking to streamline their workflow and enhance productivity in collaborative environments.
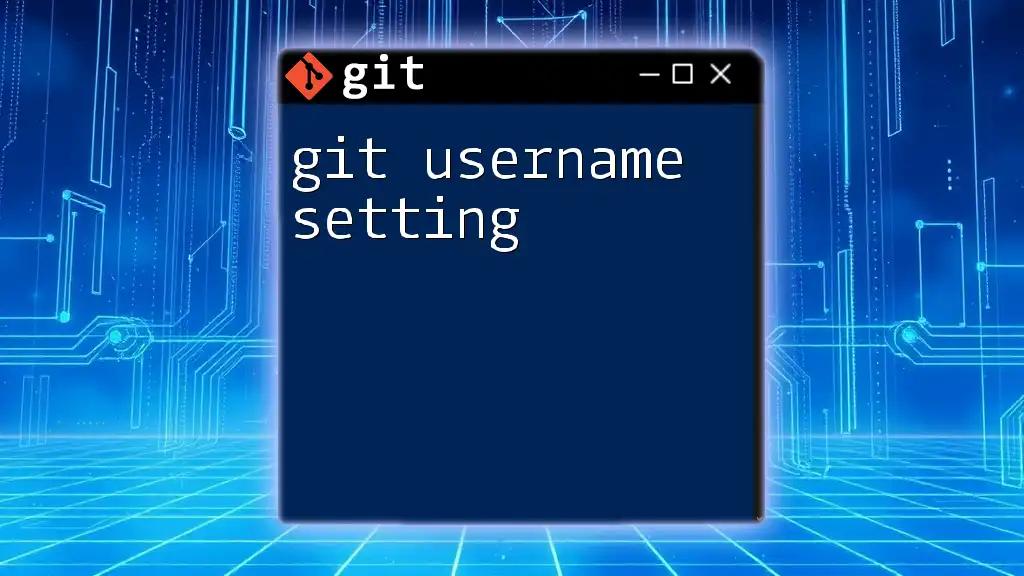
Setting Up to Use the Git User API
Prerequisites
Before diving into the Git User API, ensure that you have the required software installed on your system. A working installation of Git is essential, as well as any potential development environments or languages (like Node.js) that you may use for scripting.
Authentication Methods
To interact with the Git User API securely, proper authentication is crucial. There are two primary methods of authentication:
Username and Password: This straightforward approach entails using your Git username and password to authenticate API requests. It's essential to remember that this method may expose your credentials, so consider using SSH Keys instead.
SSH Keys: SSH keys enable secure communication between your machine and the Git server. Generating and using SSH keys can be beneficial for managing multiple repositories or for collaborative projects. Follow these steps to set up SSH keys:
- Generate the SSH Key:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
- Add Your SSH Key to the SSH Agent:
eval "$(ssh-agent -s)" ssh-add ~/.ssh/id_rsa
- Copy the SSH Key to Your Clipboard:
cat ~/.ssh/id_rsa.pub
Finally, add this key to your Git hosting service to complete the authentication setup.
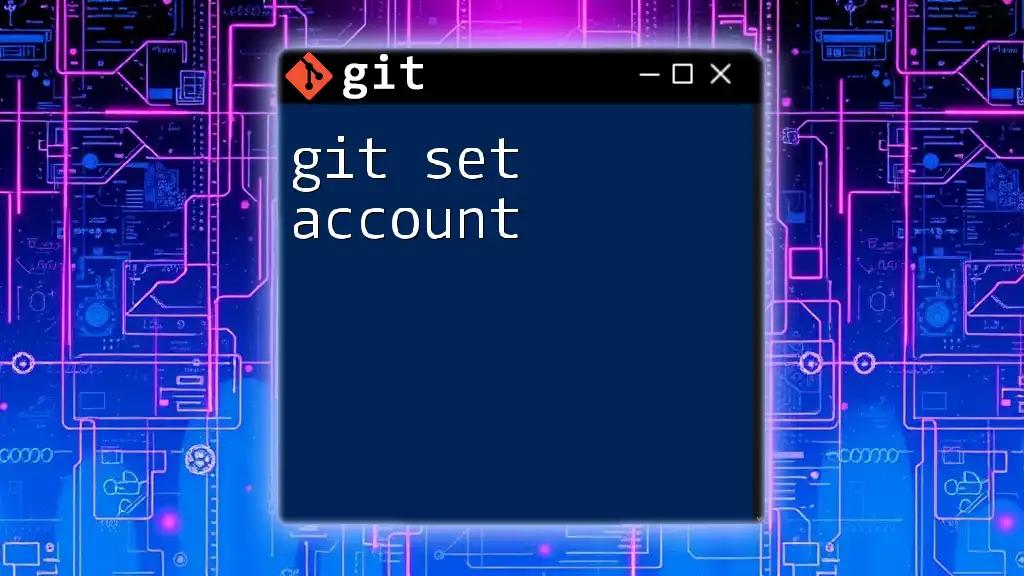
Key Features of the Git User API
Repository Management
Managing repositories effectively is crucial in any development environment. The Git User API facilitates key repository functions such as:
Creating a Repository: To initiate a new repository, you can utilize the following command:
git init <repository-name>
This command creates a new directory with the specified name where you can start adding files and commits.
Cloning a Repository: When you need to contribute to an existing repository, cloning it locally is often the first step. You can achieve this using:
git clone <repository-url>
This command creates a copy of the remote repository on your local machine, allowing you to make changes and push them back to the source.
Branch Management
Branches are a fundamental concept in Git, enabling multiple lines of development. Understanding how to manage branches using the Git User API is essential.
Creating a Branch: Use the following command to create a new branch for your development needs:
git branch <branch-name>
Switching and Merging Branches: To switch between branches effortlessly, use:
git checkout <branch-name>
Once you have completed your changes in the new branch, merging them back to the main branch can be done by executing:
git merge <branch-name>
It is essential to be aware of potential conflicts when merging, which can occur if changes are made to the same lines in different branches.
Committing Changes
Every change in a Git repository needs to be captured through a commit. Not committing your changes correctly can lead to loss of work.
Making a Commit: To save modifications to your repository, first stage the changes, then commit them using these commands:
git add <file-name>
git commit -m "Commit message"
The commit message should be clear and concise, summarizing the changes made.
Pushing Changes
After local modifications are committed, it’s time to share those changes with the remote repository. Pushing your changes ensures that others can see and collaborate on the latest code. Use the following command to push changes to the specified branch:
git push origin <branch-name>
Understanding the components of the command will empower you to customize your pushes effectively.
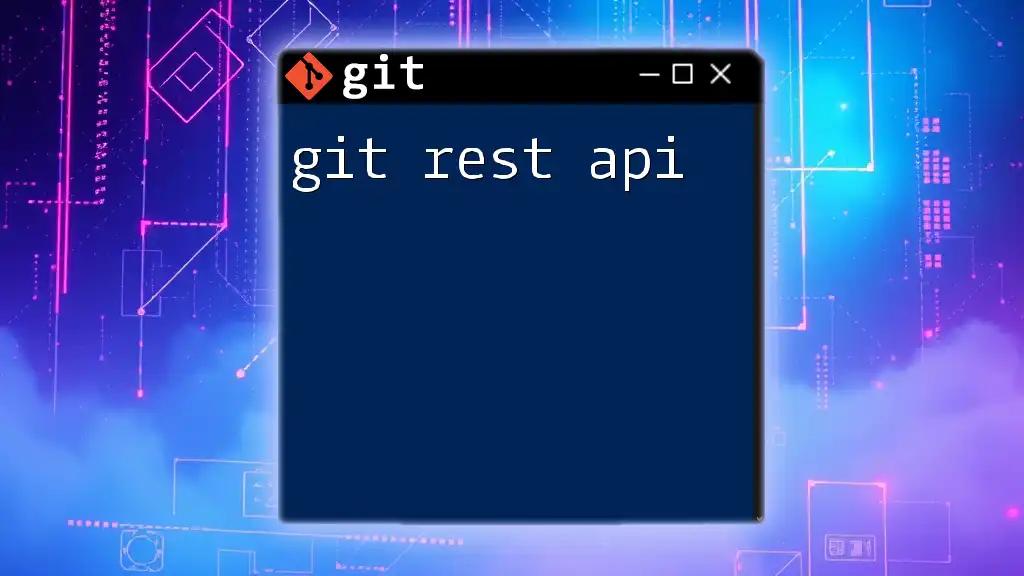
Working with the Git User API in Code
Writing Scripts
One of the significant advantages of the Git User API is the ability to automate processes through scripts. Here’s an example of a straightforward shell script that clones a repository, makes updates to a file, stages, commits, and pushes changes automatically:
#!/bin/bash
git clone <repository-url>
cd <repository-name>
echo "New changes" >> file.txt
git add file.txt
git commit -m "Added a new file"
git push origin main
This script helps eliminate the repetitive tasks of Git operations, allowing developers to focus on building features instead.
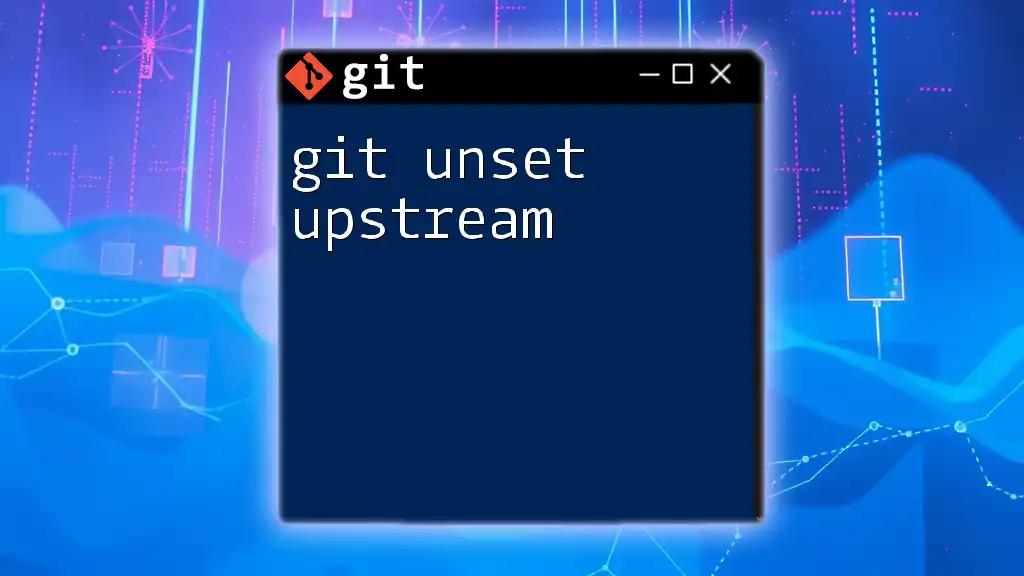
Best Practices for Using the Git User API
Organizing and Naming Repositories
Maintain a clear and consistent naming convention for your repositories. This practice aids in navigating and managing projects efficiently.
Commit Messages
Effective commit messages enhance collaboration. Be concise but descriptive enough that others (and you, in the future) will understand the changes made with just a glance.
Managing Permissions
For larger teams, managing user permissions is paramount. Ensure roles and access rights are clearly defined to maintain security and integrity in collaborative environments.
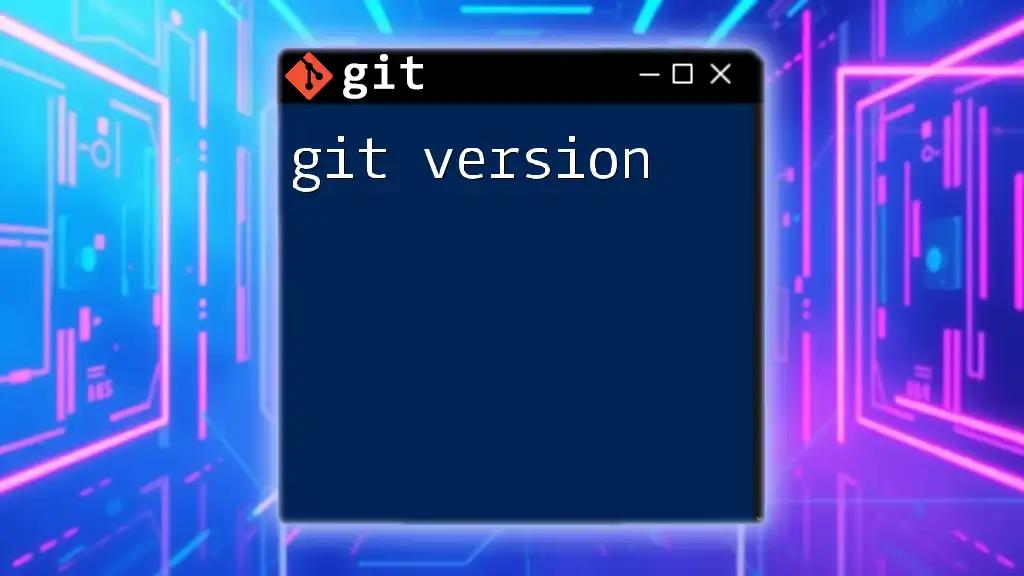
Troubleshooting Common Issues
API Response Errors
When using the Git User API, you may encounter errors such as "authorization failed" or "repository not found." Understanding how to interpret these messages is vital. Often, simply checking your authentication credentials will resolve these issues.
Debugging Checkout Issues
Errors can also arise when checking out branches. Common issues include "path not found" or "unable to switch branches." When this occurs, checking your repository status and ensuring you are on a clean branch can often help resolve these errors.
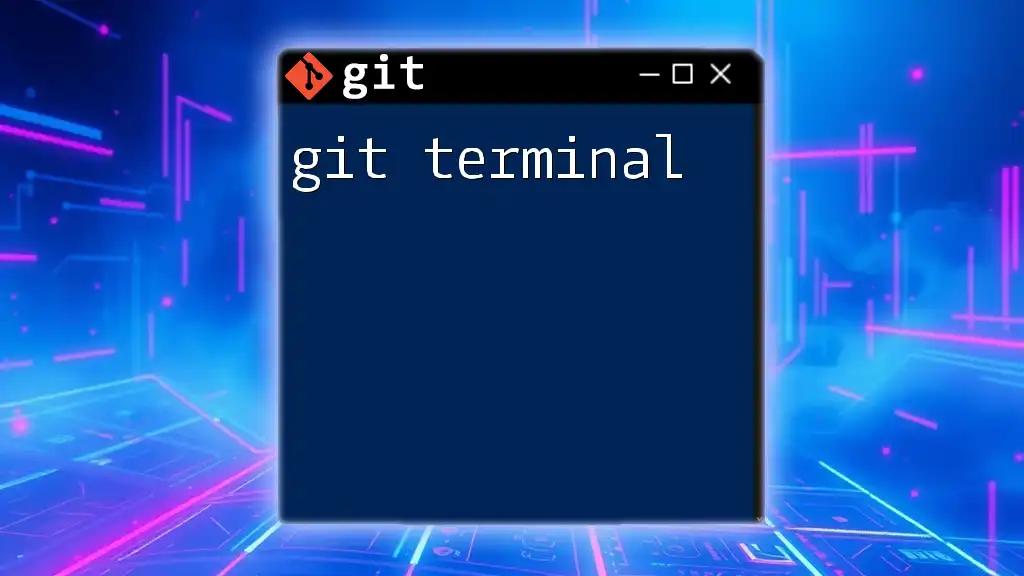
Conclusion
Mastering the Git User API is integral for developing efficient workflows in today’s collaborative coding environments. By understanding how to utilize its capabilities for repository management, branch automation, and effective scripting, you can significantly enhance your productivity and collaboration with others. As you continue to explore Git, consider diving deeper into its advanced functionalities for an even more robust development experience.
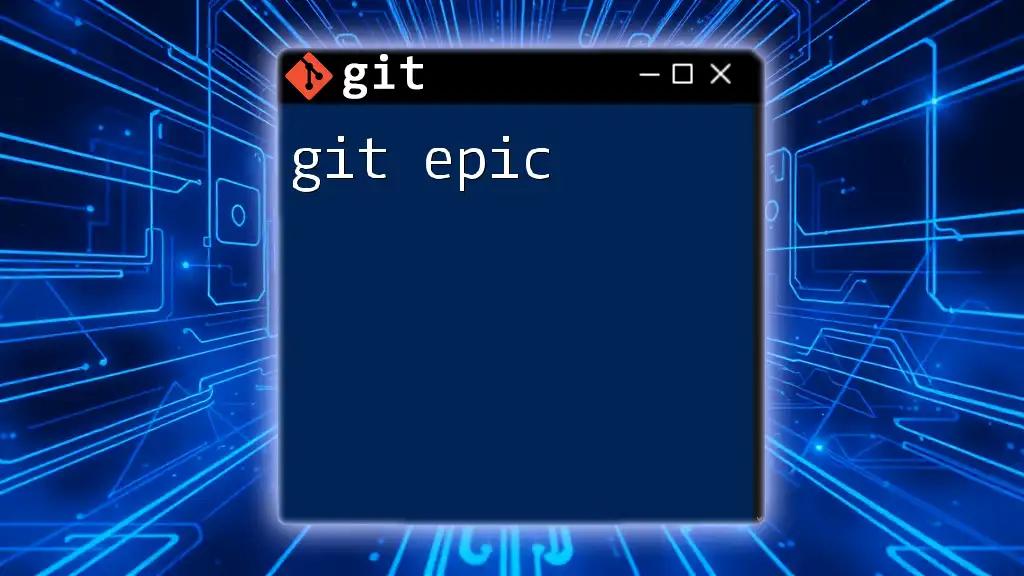
Additional Resources
For further insights and learning materials, refer to the official Git documentation, recommended tutorials, and join community forums where you can connect with other developers to share knowledge and experiences.