The error message "git: 'remote-https' is not a git command" typically occurs when trying to execute a Git command related to the remote HTTPS protocol without having the necessary Git components installed or configured properly.
Here's a code snippet that demonstrates how to clone a repository using HTTPS, which is usually the context in which you might encounter this error:
git clone https://github.com/username/repository.git
Understanding the Git Command-Line Interface
What is the Git CLI?
The Git Command-Line Interface (CLI) is a powerful tool that allows users to interact with their Git repositories directly through terminal commands. This interface is essential for developers who seek to manage source code efficiently, track changes, and collaborate with others seamlessly.
One of the main benefits of using the Git CLI over graphical user interfaces (GUIs) is that it offers comprehensive control and flexibility. As a developer, you can perform complex version control tasks with simple commands, automating workflows and speeding up development processes.
Common Git Commands
In the realm of Git, a few commands are frequently used and essential for daily operations. Here’s a brief overview of these commands:
- `git init`: Initializes a new Git repository.
- `git clone [repository]`: Clones an existing repository into a new directory.
- `git commit -m "[message]"`: Saves changes to the local repository with a descriptive message.
- `git push [remote] [branch]`: Uploads local repository content to a remote repository.
Familiarity with these basic commands lays the foundation for more complex operations and will come in handy when dealing with error messages like "git: 'remote-https' is not a git command".
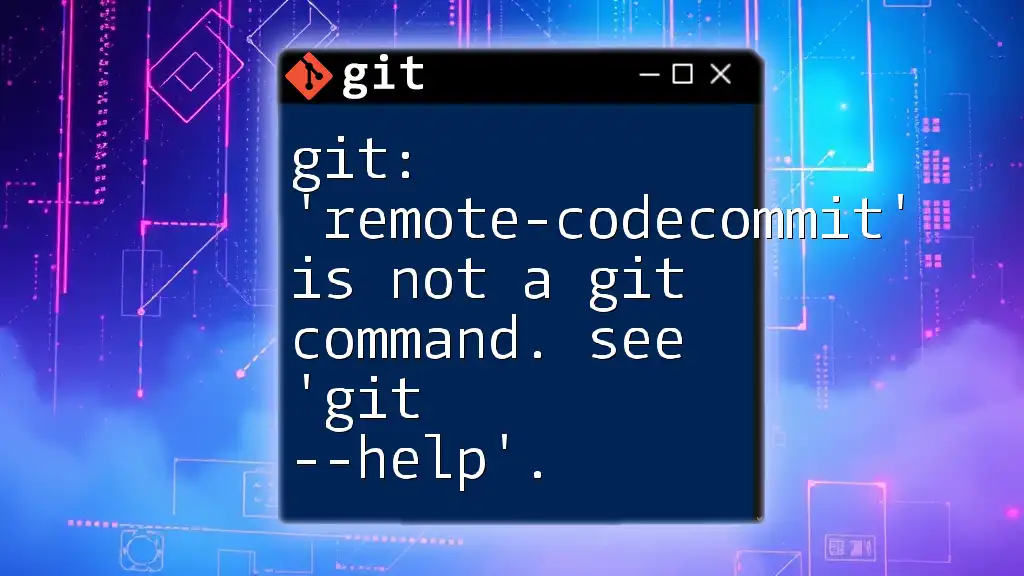
Decoding the Error Message
What Does the Error Mean?
When you encounter the error “remote-https is not a git command”, it signifies that Git is interpreting your input incorrectly. Specifically, "remote-https" refers to a protocol for transferring data, not a standalone command within Git itself. Instead, Git uses `remote` as a command to manage connections to remote repositories.
Situations Leading to the Error
Several scenarios may lead to this error:
- Typing Mistakes: Simple typos can change the intended command. For instance, omitting a crucial space or word can lead to this confusion.
- Incorrect Command Structure: Using the wrong command form can also trigger this error, especially if you’re trying to perform a remote action.
Being aware of these common pitfalls can significantly reduce the frequency of encountering this error.
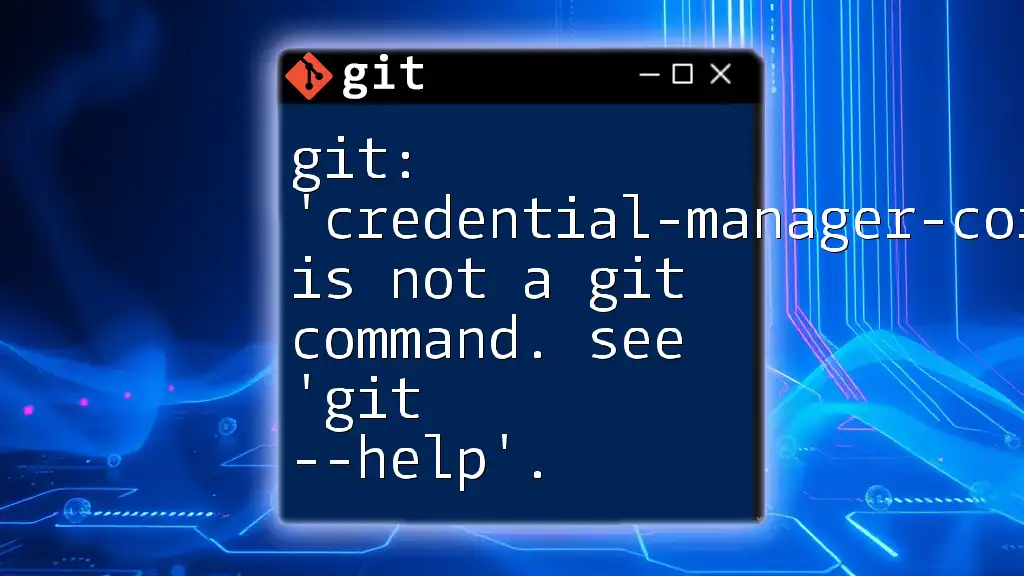
Troubleshooting the Error
Basic Checks
Before diving deep into troubleshooting, it's crucial to verify that Git is correctly installed. You can check your Git version by using:
git --version
This command helps confirm that your installation is good and functioning as expected.
Correcting Potential Mistakes
When faced with the error, your first line of action should be to review the command you entered. Understanding the correct command format is particularly important. For example, if you mistakenly typed:
git remote-https add origin https://github.com/user/repo.git
You received an error because `remote-https` is not a valid command. The correct command format is:
git remote add origin https://github.com/user/repo.git
Here, you specify the remote repository's URL correctly, avoiding the confusion of the protocol and command structure.
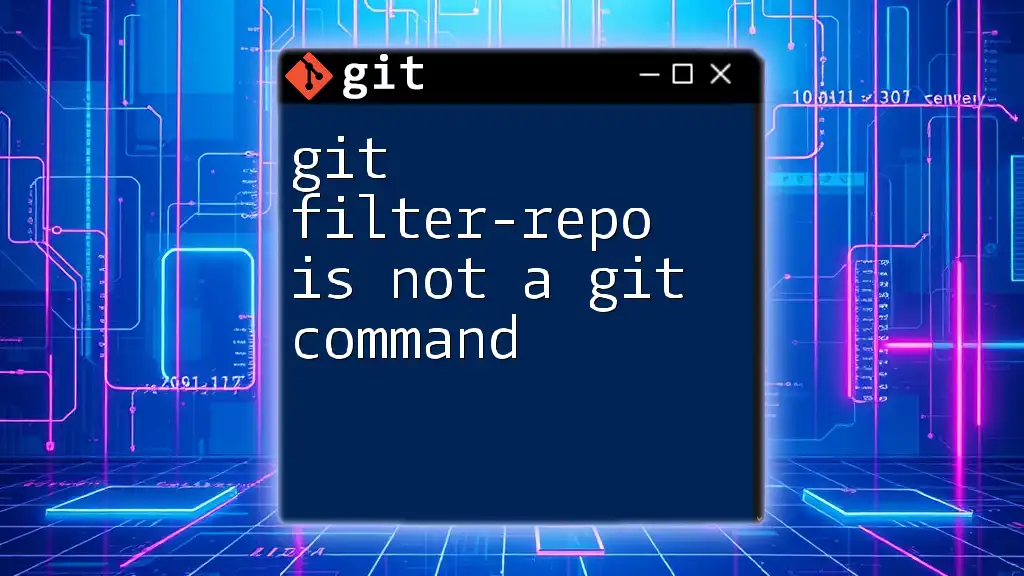
Configuring Git Remotes Properly
What are Git Remotes?
Git remotes are versions of your repository that are hosted on the internet or another network. They serve as a collaborative tool, allowing developers to work together on various projects seamlessly. Understanding remotes is crucial for effective version control across team projects.
Adding a Remote Correctly
Adding a remote repository is a pivotal step in collaboration. Here’s how to do it properly:
git remote add origin https://github.com/user/repo.git
This command establishes a connection between your local repository and the specified remote repository at the URL provided.
Checking Existing Remotes
When you want to view the remotes already associated with your repository, use the following command:
git remote -v
This command will display all remotes, providing a quick way to verify that your addition was successful and is pointed to the correct location.
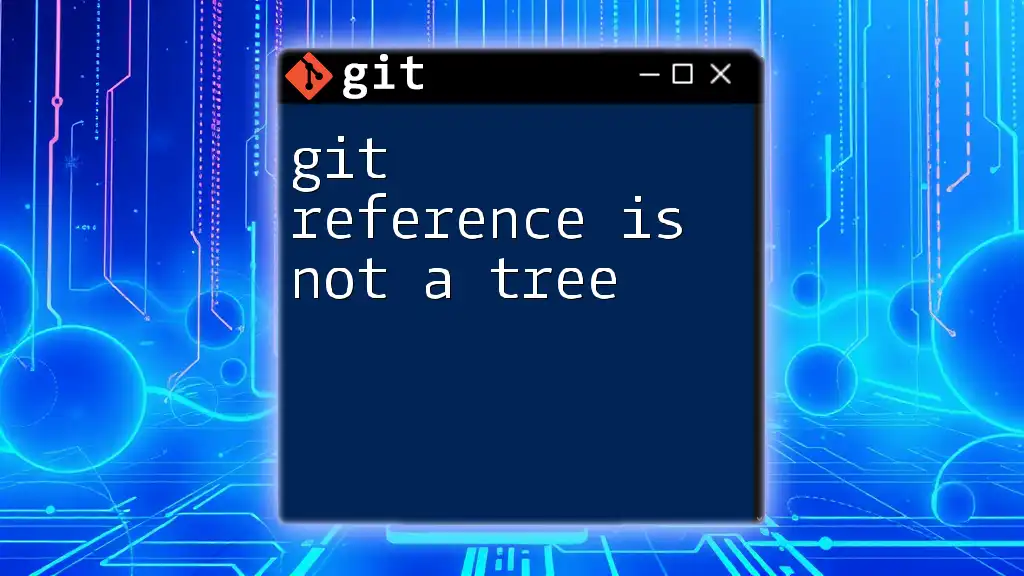
Alternative Protocols
Overview of Different Git Protocols
Git supports various protocols to interact with repositories, including:
- SSH: Offers secure communication and is often preferred for private repositories.
- HTTP/HTTPS: Commonly used for public repositories, often easier for beginners as it does not require SSH key setup.
- Git Protocol: The simplest but less secure, best for local use.
Each protocol has its unique benefits, and understanding these can help you choose the right one for your project needs.
When to Use HTTPS
Using HTTPS is advisable in scenarios where ease of access and convenience are paramount, especially for users less familiar with SSH keys. HTTPS can be particularly beneficial when working in environments with strict firewall rules, where SSH connections may be blocked.
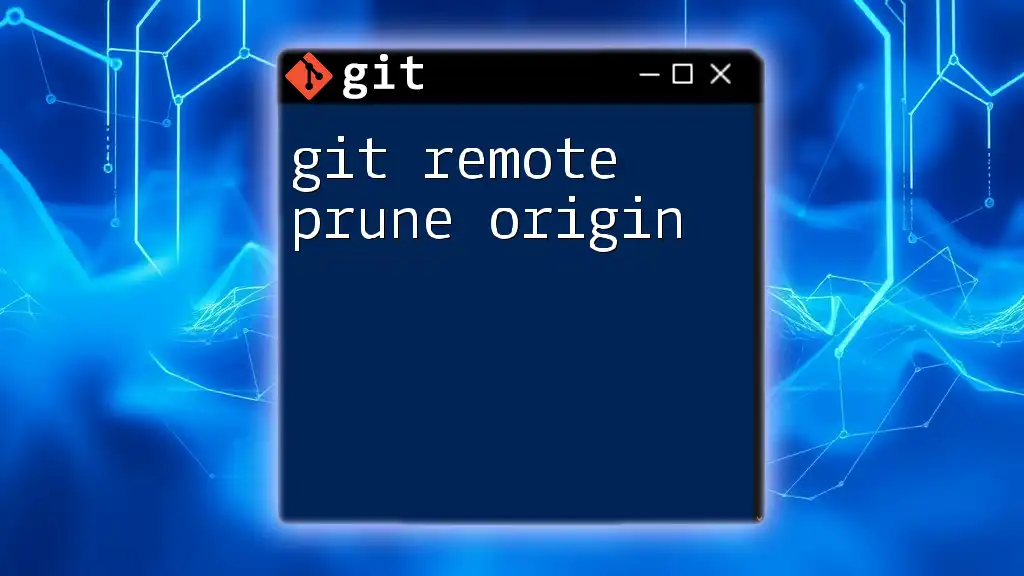
Seeking Help with Git Commands
Using Git’s Help System
When you are unsure about a command or need clarification, Git has a built-in help system that is invaluable. You can invoke help for various commands by using:
git remote --help
This command will provide you with detailed documentation about how to use the `remote` command and its various options, helping you to troubleshoot issues effectively.
Accessing Official Documentation
In addition to the built-in help command, extensive Git documentation is available online. The [official Git documentation](https://git-scm.com/doc) provides comprehensive resources for understanding all Git commands and concepts.
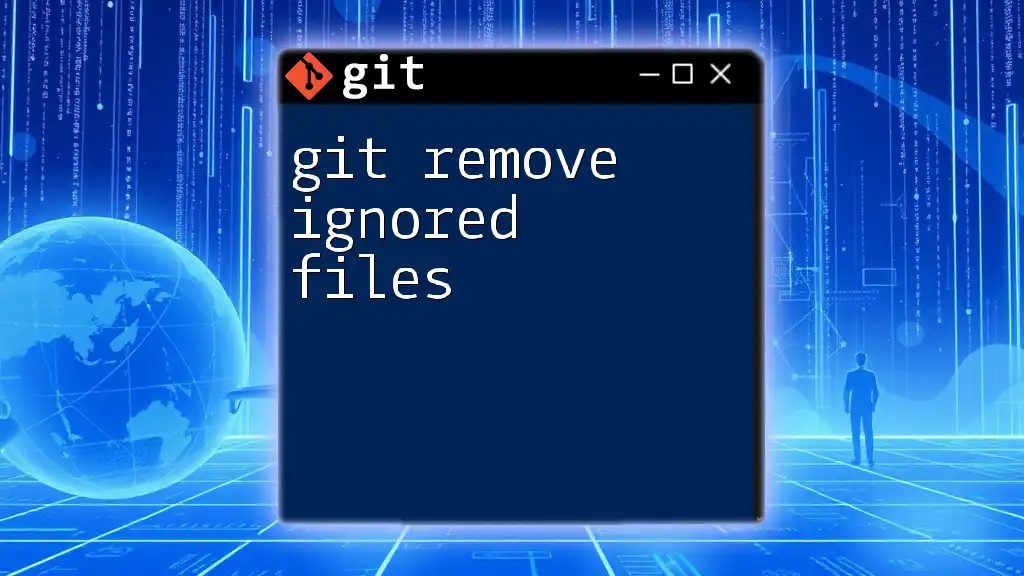
Conclusion
Understanding the nuances of Git commands is crucial for navigating potential errors like "git: 'remote-https' is not a git command" effectively. By familiarizing yourself with correct command usage, troubleshooting processes, and the configuration of Git remotes, you can enhance your version control skills significantly.
For those looking to delve deeper into Git or wish to lean on an expert guide, consider joining one of our Git training courses. Embrace the challenge of mastering Git, and elevate your software development skills to new heights!
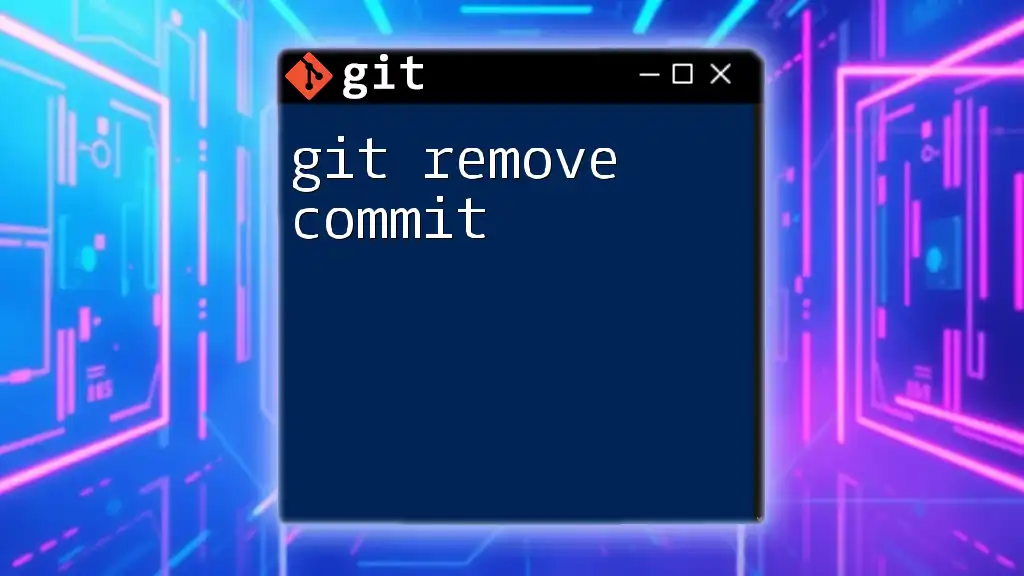
Additional Resources
For further exploration, check out our Git Cheat Sheet, which offers quick references and tips. Additionally, consider reading popular Git-related books or taking online courses to solidify your understanding and command of using Git in your development projects.