"Git puppet" typically refers to leveraging Git hooks to automate deployments or other actions in a repository, enabling you to streamline development workflows.
Here’s a simple example of using a Git post-commit hook to automatically deploy changes:
#!/bin/sh
git push production master
To use this, place the script in the `.git/hooks/post-commit` file and make it executable.
What is Git Puppet?
Git Puppet is a powerful combination of Git, a version control system, and Puppet, a configuration management tool, that allows teams to manage their infrastructure as code efficiently. Using Git Puppet, developers can track changes in their Puppet configuration files, enabling teams to collaborate seamlessly and maintain a history of modifications.
The benefits of using Git with Puppet include:
- Version Control: Git allows you to keep track of every change made to your Puppet manifests, ensuring you can revert to previous versions if necessary. This is essential for maintaining system stability.
- Collaboration: Multiple team members can work on Puppet manifests simultaneously, thanks to Git's branching and merging capabilities, which facilitate efficient collaboration.
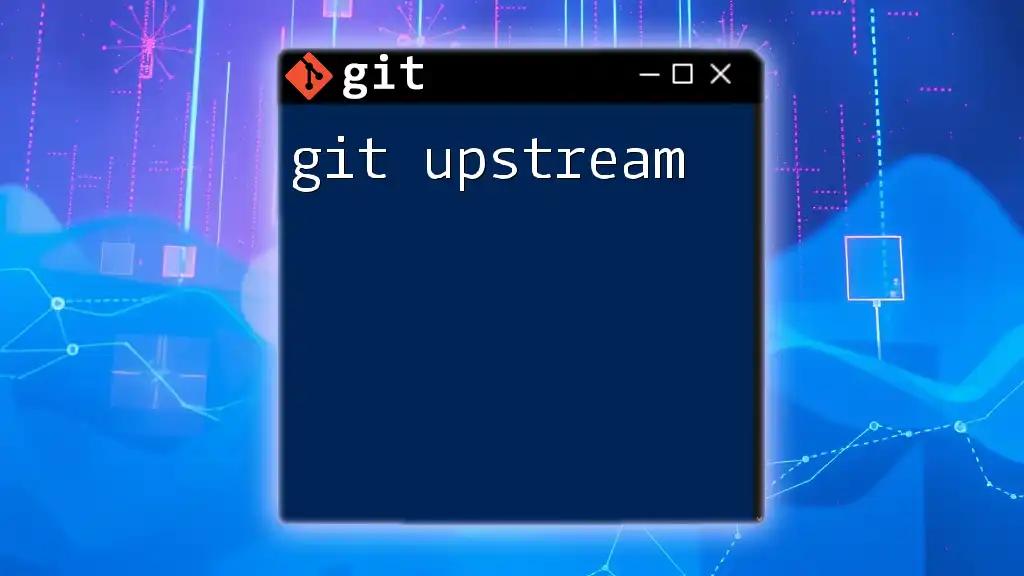
Setting Up Git Puppet Environment
Prerequisites
Before delving into Git Puppet, ensure you have a functional development environment set up:
- Git Installation: You’ll need to install Git on your system. You can find instructions for your particular operating system on the [official Git website](https://git-scm.com/downloads).
- Puppet Installation: Follow the guidelines on the [Puppet documentation](https://puppet.com/docs/puppet/latest/installing_and_upgrading.html) to install Puppet and any dependencies required for your project.
- Basic Familiarity: Familiarize yourself with essential Git and Puppet commands. Understanding how these tools work individually will make their integration much smoother.
Creating Your First Git Repository
Creating a Git repository is the first step to managing your Puppet configurations effectively. Here’s how to set it up:
Initial Setup
- Create a new directory for your Puppet project.
- Initialize a Git repository in that directory.
mkdir my_puppet_repo
cd my_puppet_repo
git init
This command creates a new Git repository, allowing you to start tracking your Puppet manifests.
Cloning Existing Repositories
If you want to work on an existing Puppet project, you can clone its repository. This is done using the `git clone` command:
git clone https://github.com/username/my_puppet_repo.git
This command creates a local copy of the repository, including all its history.
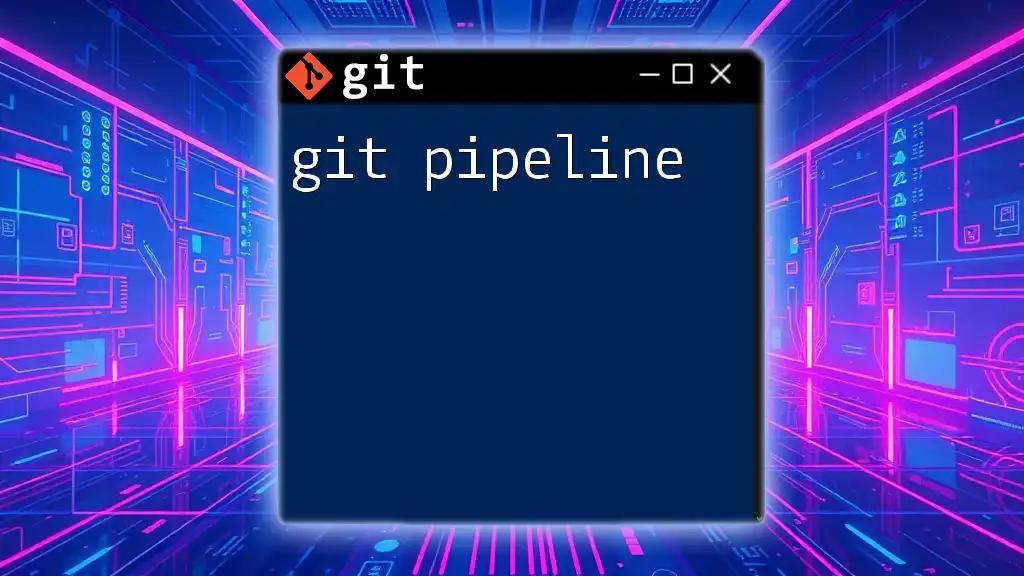
Managing Puppet Manifests with Git
Best Practices for Structuring Your Repository
A well-structured repository is vital for managing your Puppet manifests effectively. Here are some recommended practices:
- Directory Structure: Organize your repository to separate modules, manifests, and hiera data. A common structure is as follows:
my_puppet_repo/ ├── modules/ │ └── myapp/ │ ├── manifests/ │ │ └── init.pp │ └── templates/ ├── hiera.yaml └── Puppetfile
This organization promotes clarity and ease of navigation within your project.
Making Changes to Puppet Manifests
As you work with Puppet, you will frequently need to edit manifests. Here’s how to effectively make changes:
Editing Manifests
Using a text editor, add or modify Puppet manifests to reflect the desired state of your systems. For example, here’s a simple Puppet class for installing `httpd`:
class myapp {
package { 'httpd':
ensure => installed,
}
}
Staging and Committing Changes
Once you've made your changes, stage and commit them using the following commands:
git add manifests/myapp.pp
git commit -m "Added myapp manifest for HTTPD installation"
This records your changes in the project’s history.
Viewing History and Tracking Changes
To track changes over time, use the `git log` command, which provides a chronological list of all commits:
git log
Additionally, `git diff` allows you to compare the current changes against previous commits to see what has been modified.
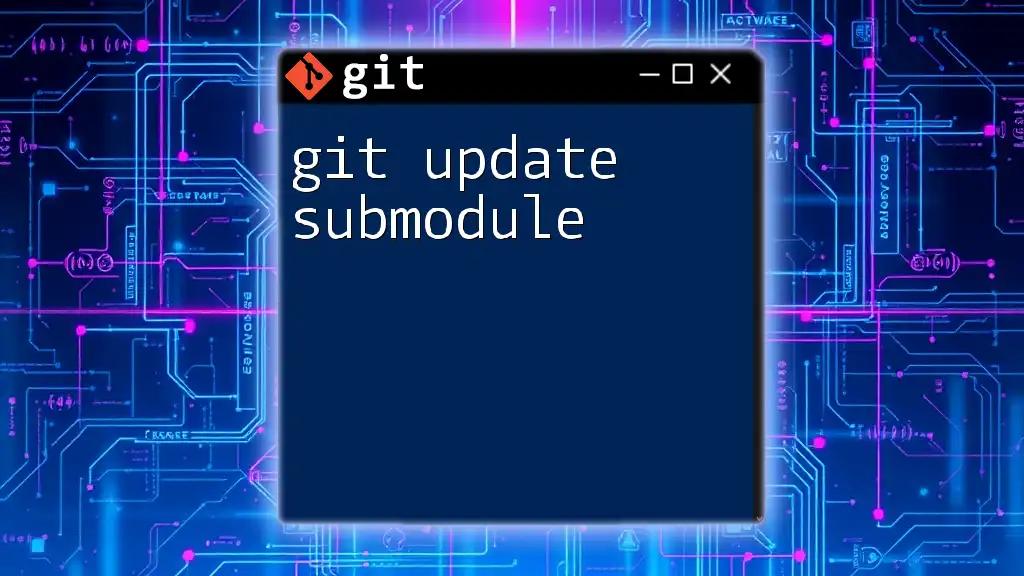
Collaborating with Teams Using Git Puppet
Branching Strategies
When collaborating with teams, branching strategies become vital. Branching facilitates multiple developers working on adjustments without affecting the main codebase. Here’s how to create a new branch:
git checkout -b feature/new-manifest
This command creates a new branch called `feature/new-manifest` and switches your working directory to it.
Merging Changes
Once changes are ready to be integrated back into the main project, merging is the next step. Use the following commands to merge a branch back into the main branch:
git checkout main
git merge feature/new-manifest
If there are conflicts, Git will notify you, and you can resolve them manually.
Using Pull Requests
Utilizing pull requests enhances team collaboration by allowing for reviews and discussions before merging changes. Platforms like GitHub and GitLab support creating pull requests, ensuring that each change is reviewed, enhancing code quality.
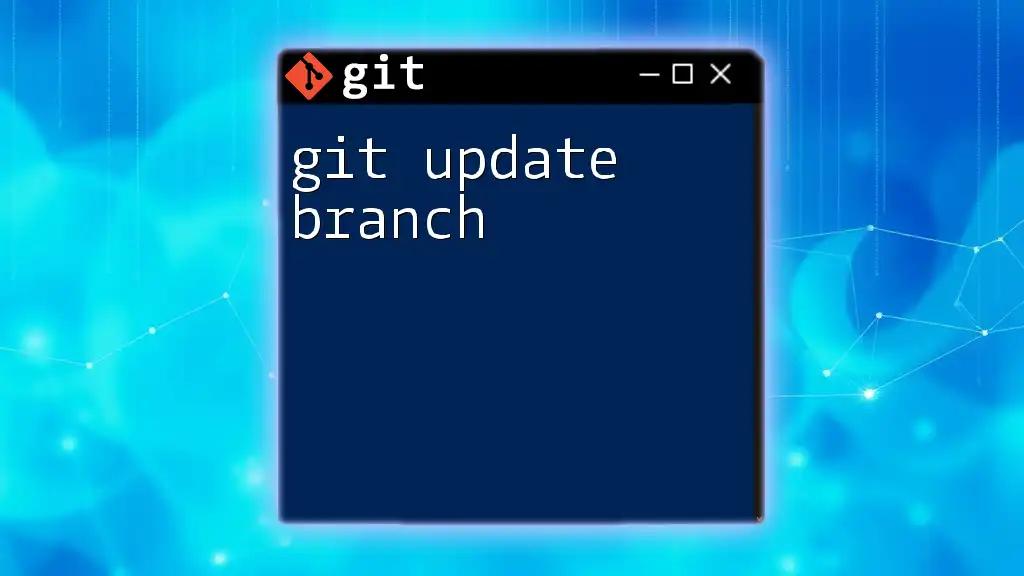
Troubleshooting Common Issues
Git Conflicts
Merge conflicts can occur when two branches have competing changes. When this happens, Git will mark the conflict in the affected files. You’ll need to manually resolve these conflicts by editing the conflicting files and then staging the resolved files using:
git add path/to/resolved_file
Rollbacks and Undoing Changes
Mistakes happen! If you need to roll back changes, you can use the `git revert` command to create a new commit that undoes the last commit or reset to a previous state using:
git reset --hard HEAD~1
This command resets your repository to the state it was in before the last commit.
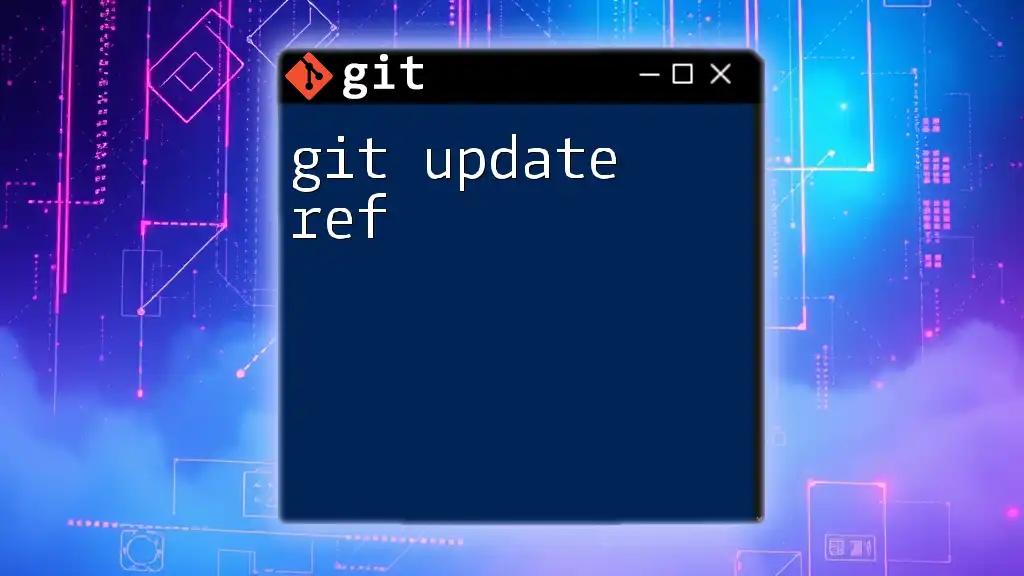
Advanced Git Puppet Techniques
Integrating CI/CD Pipelines
Integrating Continuous Integration (CI) and Continuous Deployment (CD) practices with Git Puppet allows for automated testing and deployment of your configuration changes, leading to faster and more reliable infrastructure updates. By configuring CI/CD tools like Jenkins or GitHub Actions, you can set up pipelines to automate these processes.
Using Hooks with Git
Git hooks are scripts that run automatically at specific points in the Git workflow, which can help automate tasks such as code linting or running tests before commits. Setting up a pre-commit hook ensures that code adheres to defined standards before submission.
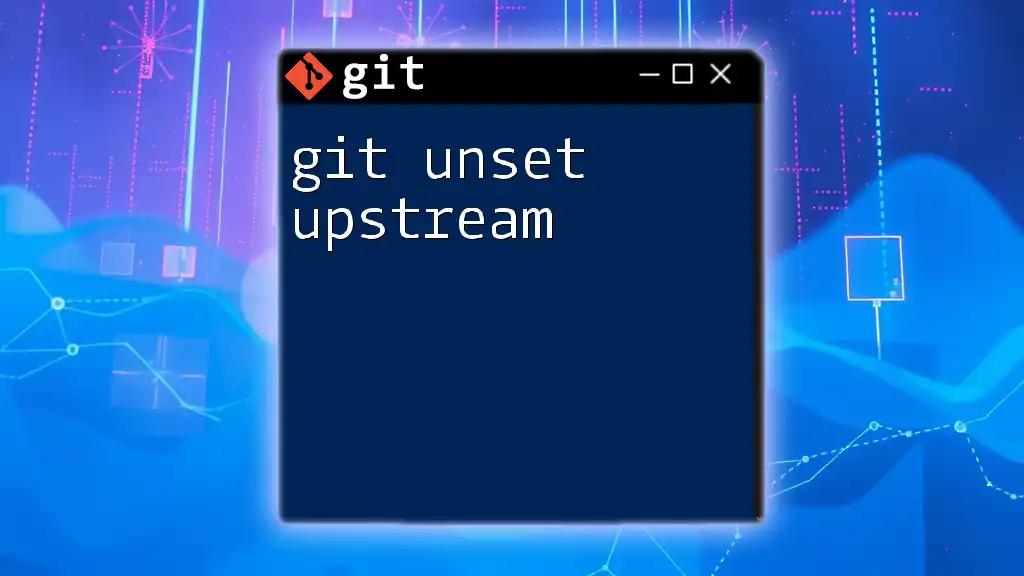
Conclusion
Throughout this guide, we’ve explored the synergies between Git and Puppet. By implementing Git Puppet into your development workflow, you embrace version control and enhance collaboration, significantly improving your ability to manage infrastructure as code.
As you embark on your Git Puppet journey, consider starting with a small project where you can apply all these principles, fostering both your understanding and skills in using these powerful tools effectively.
For those eager to delve deeper, numerous resources are available, including official documentation and community tutorials, to further enhance your expertise in Git Puppet.