You can count the number of lines of code in your Git repository using the following command, which combines `git` and `wc` (word count) to get the total line count across all tracked files.
git ls-files | xargs wc -l
Understanding Code Lines
When embarking on the journey to count how many lines of code in Git, it's essential first to define what a line of code actually means. Typically, it can be categorized into two types: physical lines and logical lines of code.
- Physical Lines: These represent each line in the source file regardless of whether it contains code, comments, or whitespace.
- Logical Lines: These represent actual lines of code that contribute to the functionality of the program.
It’s crucial to consider how comments and blank lines are treated in your counting strategy, as they might inflate the total line count without adding functional value.
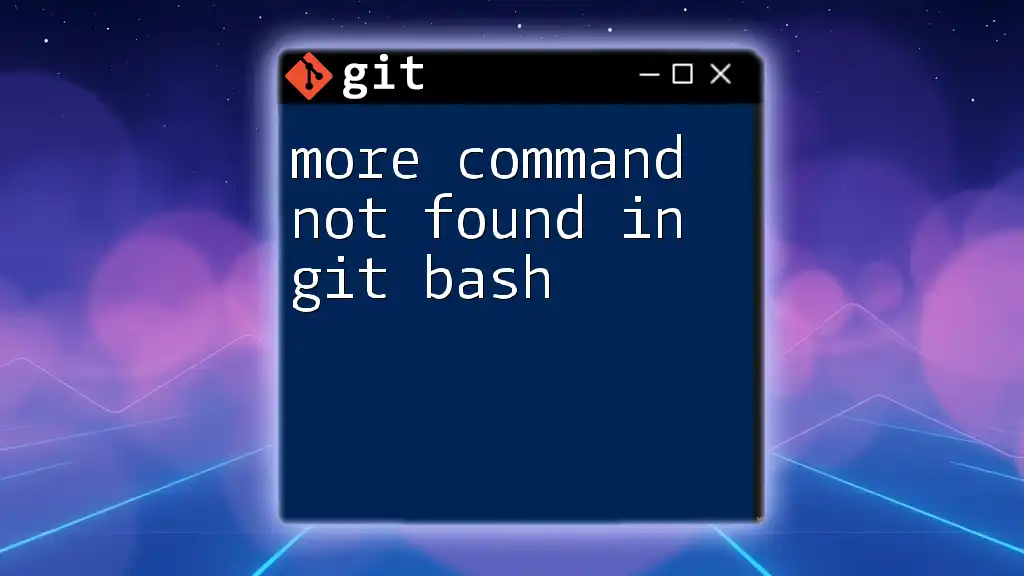
Overview of Git Commands for Code Measurement
To effectively count how many lines of code in Git, understanding the right commands and their contexts is essential. Key Git commands such as `git diff`, and `git log` will become your friends for measuring lines of code.
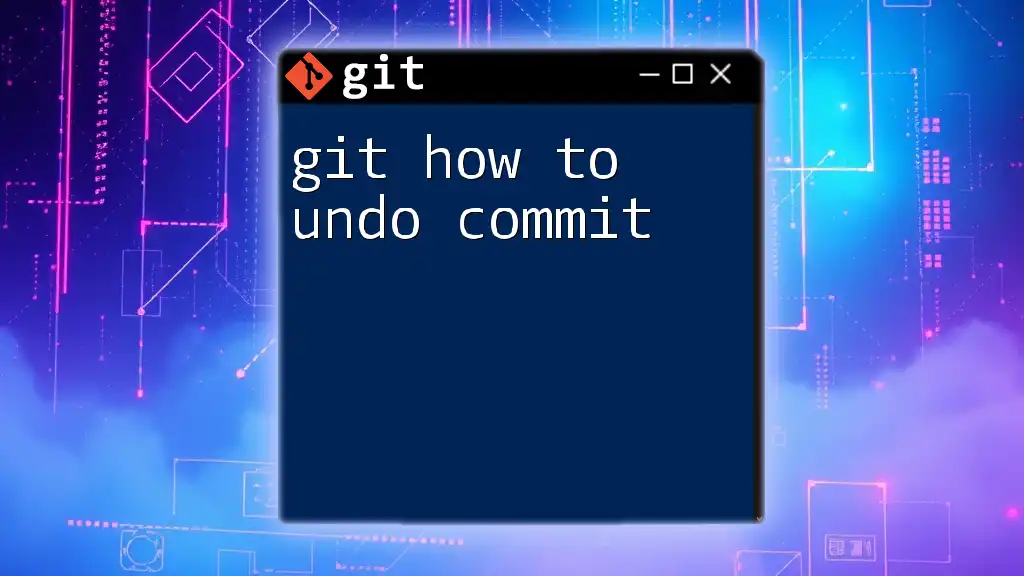
Counting Lines of Code in Local and Remote Repositories
Counting Lines in the Working Directory
The working directory often contains the files being actively developed. You can utilize the `git ls-files` command to list all tracked files and subsequently count the lines.
git ls-files | xargs wc -l
In this command:
- `git ls-files` retrieves all tracked file paths in the repository.
- `xargs wc -l` pipes these file paths into the `wc` command, which counts lines.
The output will display the number of lines per file followed by a total line count.
Counting Lines in a Specific Commit
To see how many lines were added or removed in a particular commit, use the `git show` command. Here’s how you can do that:
git show COMMIT_HASH | wc -l
Replace `COMMIT_HASH` with the actual hash of the commit. This command outputs the number of lines in the diff output of that commit.
Understanding this output allows you to view the scale of changes made at a particular point in time, which can inform your future development strategies.
Counting Lines in the Entire Repository
To analyze the total changes made across your repository, the `git diff` command can provide valuable insights:
git diff --shortstat HEAD^ HEAD
This command compares the last commit (`HEAD`) with its previous state (`HEAD^`). The `--shortstat` option gives a summary of added and deleted lines.
While this method is effective in revealing the dynamics of your project over time, remember that counting lines across multiple commits can become complex as histories grow.
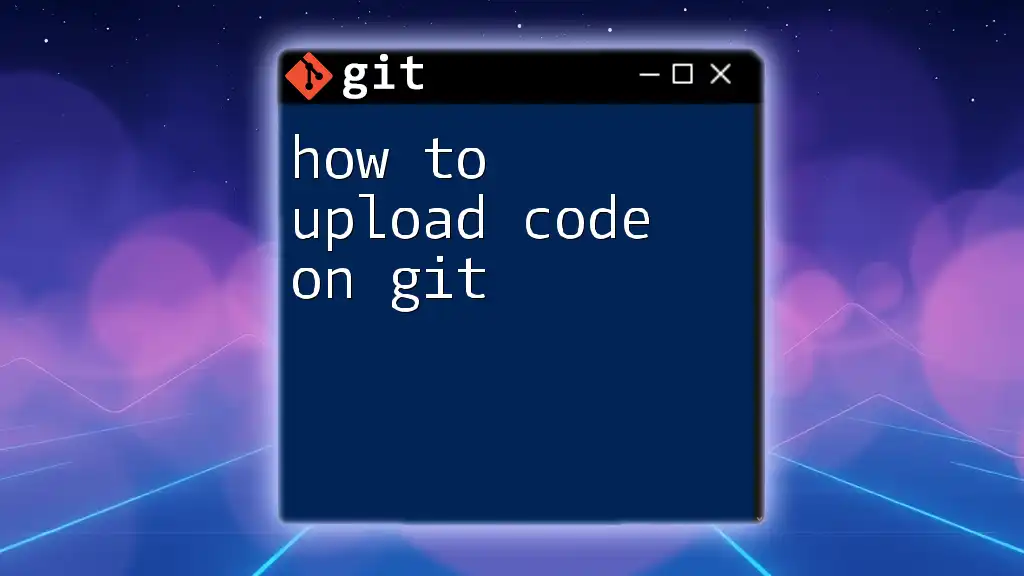
Advanced Techniques for Counting Lines
Using `git log` with `--stat`
To summarize total line additions/removals over a series of commits, the `git log` command with the `--stat` option is highly beneficial:
git log --pretty=tformat: --numstat
This command provides a detailed view of how many lines were added or deleted in each commit, allowing you to understand trends in your codebase over time. The numeric output can be interpreted as:
- Lines added
- Lines deleted
- File names
This analysis is particularly helpful when evaluating the activity of contributors in a collaborative environment.
Integrating External Tools for Code Analysis
While Git provides powerful built-in commands for counting lines of code, there are also excellent external tools like cloc (Count Lines of Code) that can offer more granular metrics.
To use `cloc`, simply execute:
cloc .
This command will yield a comprehensive report detailing not just the number of lines of code, but also break down counts by language, comments, blanks, and more. Leveraging this level of detail can dramatically enhance your understanding of your codebase.
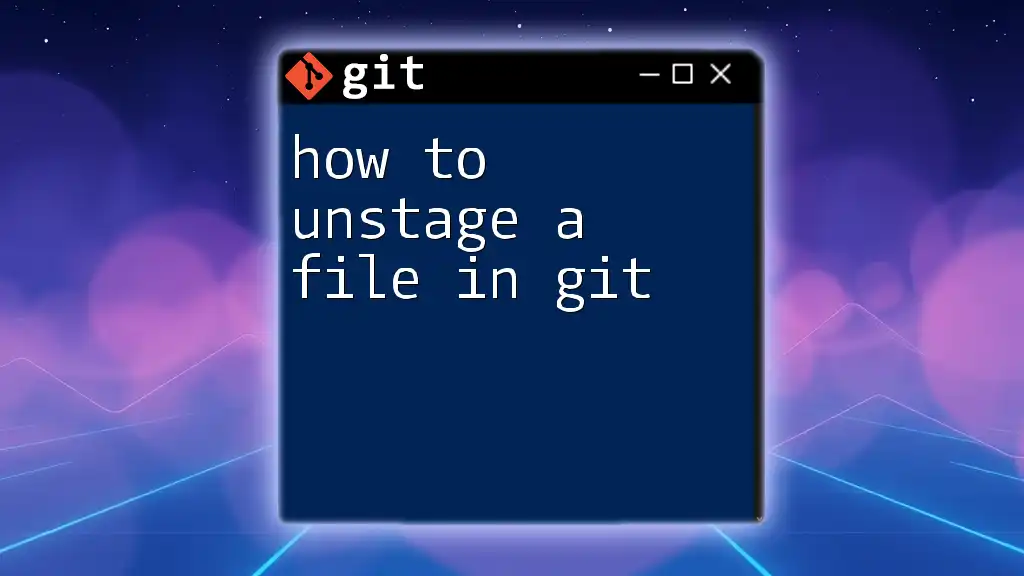
Best Practices for Tracking Lines of Code
To maximize the benefits of line counting, consider incorporating regular reporting and tracking into your workflow. Regularly checking your line counts can help manage project scope, workload, and identify potential areas for improvement.
Using these line counts strategically can yield insights into team performance. For example, correlating line counts to completed features can provide a metric that informs sprint planning and overall progress.
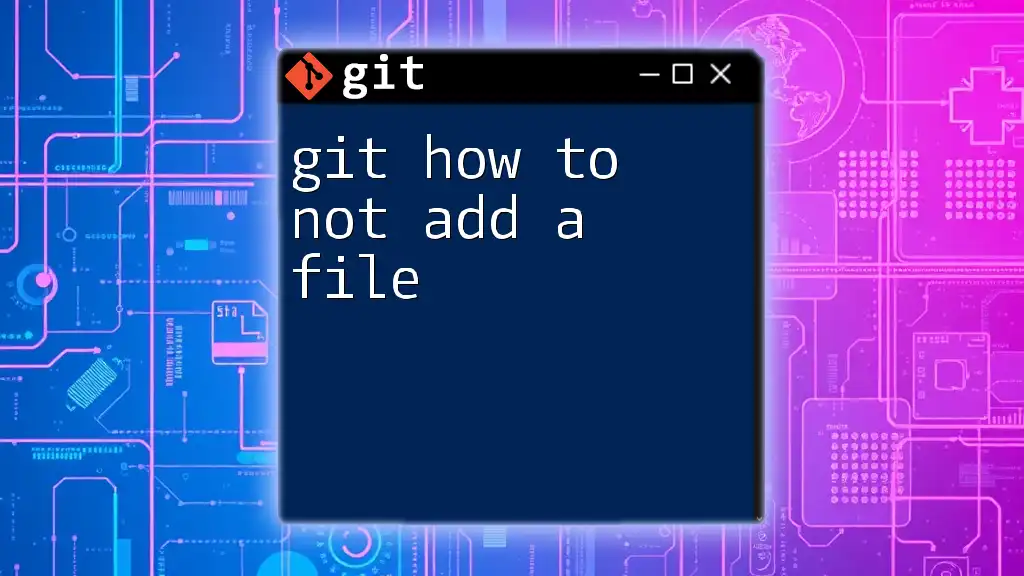
Conclusion
Knowing how to count how many lines of code in Git not only enhances your technical ability but can significantly inform project management strategies, team performance evaluation, and overall software quality. By leveraging the techniques and commands outlined here, you can efficiently monitor and sustain the health of your codebase.
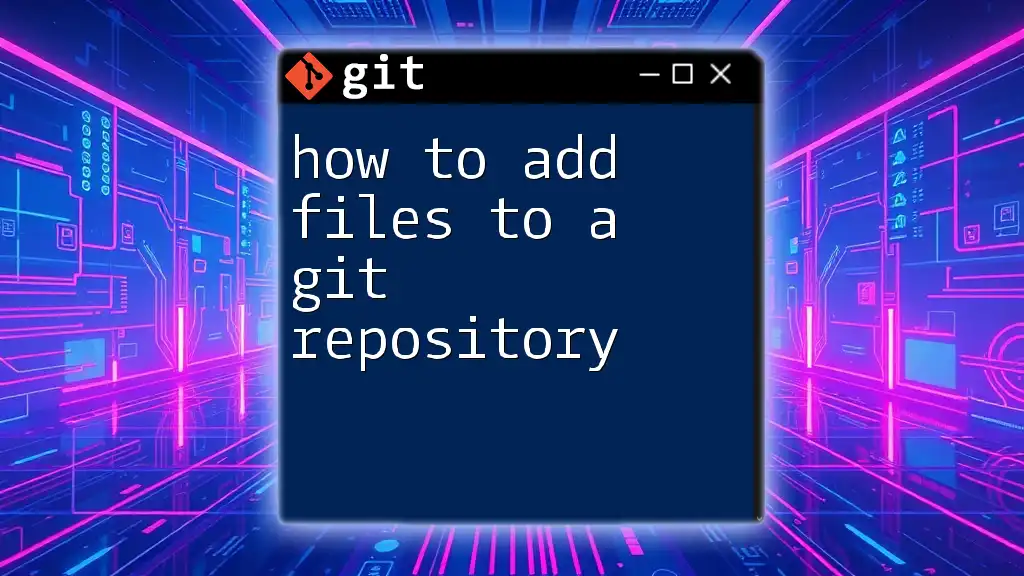
Additional Resources
For further reading on Git commands and best practices, refer to the [official Git documentation](https://git-scm.com/doc) or explore community forums and online tutorials that delve deeper into advanced Git techniques.
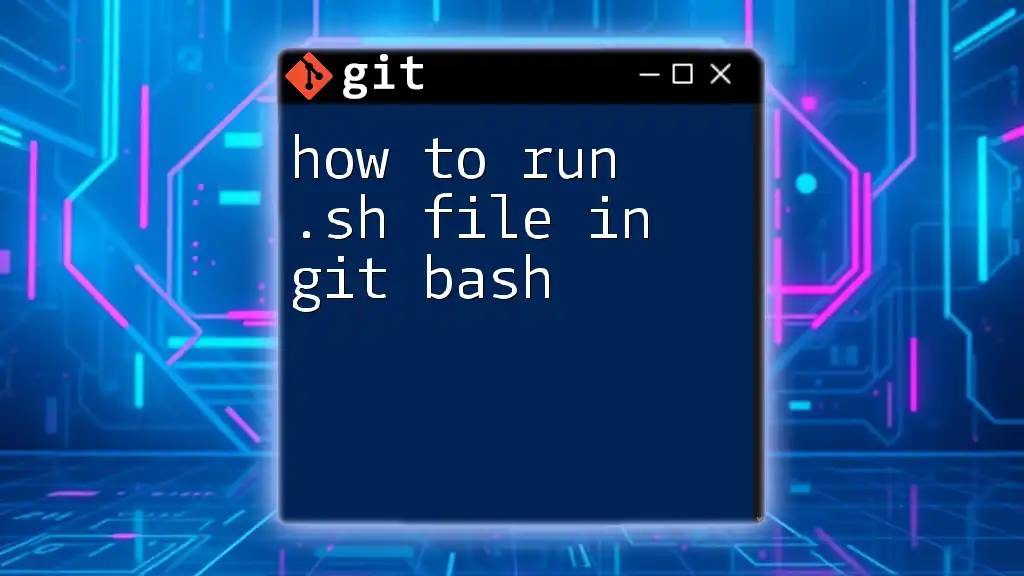
Call to Action
If you found this guide helpful, consider subscribing to our updates. Stay tuned for more tutorials, tips, and tricks to enhance your Git proficiency and take control of your coding practices!