The anatomy of Git involves understanding its fundamental components—such as repositories, commits, branches, and staging—which work together to manage and track changes in your code effectively.
Here's an example command to create a new repository and make your first commit:
git init my-repo
cd my-repo
echo "Hello, Git!" > README.md
git add README.md
git commit -m "Initial commit"
What is Git?
Git is a distributed version control system that allows multiple developers to work on the same codebase simultaneously without overwriting each other’s changes. Unlike centralized version control systems, where there is a single central repository, Git allows every developer to have a full copy of the project on their local machine. This decentralized nature enhances collaboration and enables better management of project histories.
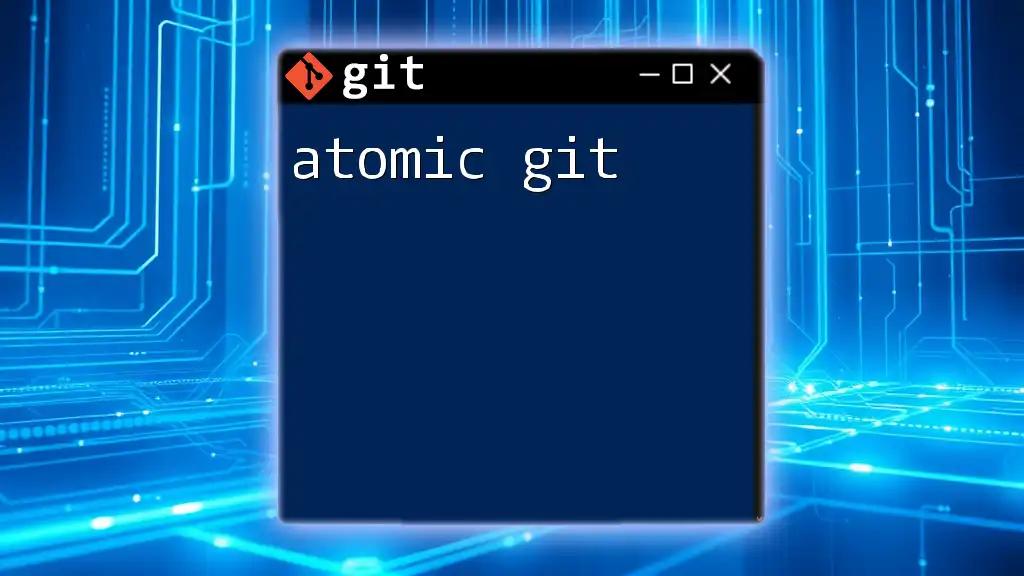
Core Concepts of Git
Repository
A repository is the foundational structure where a Git project resides. It contains the complete history of changes made to files in the project. Repositories can be divided into two main types: Local and Remote.
- Local Repository: This is located on your own machine and allows you to make changes without affecting the central codebase immediately.
- Remote Repository: This is hosted on a server accessible to multiple users, acting as a centralized place for project collaboration.
To set up a local repository, you can execute the following command:
git init
Commit
A commit represents a snapshot of your project at a particular moment in time. Each commit records changes—that is, it captures the current state of your files along with a message describing the changes made. Creating meaningful commits is essential for tracking project progress and understanding the history of the codebase.
To create a new commit, follow this syntax:
git commit -m "Your commit message here"
Best practices suggest writing clear and descriptive commit messages. For example, instead of simply stating "fixed bug," a better message would be "corrected off-by-one error in user authentication."
Branch
A branch allows developers to isolate their work without affecting the main codebase (often called the `main` or `master` branch). Working on branches is a powerful way to develop features, fix bugs, or experiment with new ideas without disturbing the production code.
To create a new branch:
git branch <branch-name>
To switch to that newly created branch, use the command:
git checkout <branch-name>
Merge
Merging is the process of integrating changes from one branch into another. This is particularly useful once a feature is complete and ready to be included in the main codebase.
To merge changes from one branch into the current branch, you would use:
git merge <branch-name>
However, merging can sometimes lead to merge conflicts when the same lines of code are altered in different branches. If this occurs, Git will prompt you to manually resolve the conflict before proceeding.
Remote
The concept of remote repositories is crucial when working collaboratively. A remote repository enables team members to share their code and collaborate effectively. Popular services for hosting Git remote repositories include GitHub, GitLab, and Bitbucket.
To connect your local repository to a remote repository, use:
git remote add origin <repository-url>
This command links your local repo to the designated remote location, enabling push and pull operations.
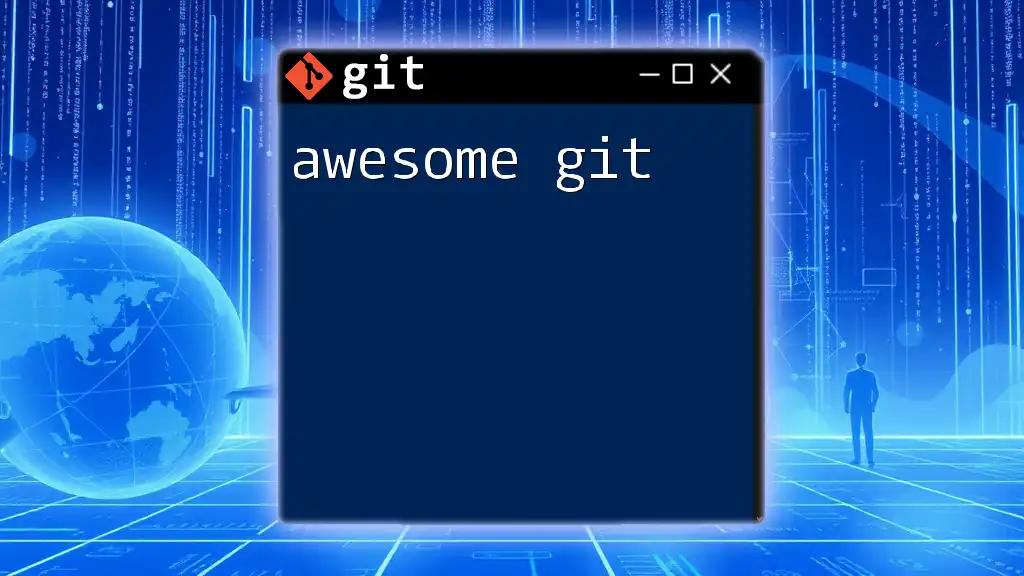
The Git Workflow
Cloning a Repository
When you want to contribute to an existing project hosted on a remote server, you first need to clone the repository. Cloning creates a copy of the remote repository on your local machine.
To clone a repository, you would execute:
git clone <repository-url>
Committing Changes
Once you make changes to files, you need to track those changes before they can be shared. The steps to commit your edits involve adding files to the staging area, followed by committing them to your local repository.
Add changed files:
git add <file-name>
Then commit those changes:
git commit -m "Commit message"
Pushing Changes
After you have made and committed your changes locally, you need to upload these changes to the remote repository to share them with your team.
To push your changes, use:
git push origin <branch-name>
Pulling Changes
To ensure that your local repository is up to date with the latest changes made by your peers, you should frequently pull updates from the remote repository.
To pull the latest changes from a remote repository, you would use:
git pull origin <branch-name>
This command fetches changes and automatically merges them into your current branch.
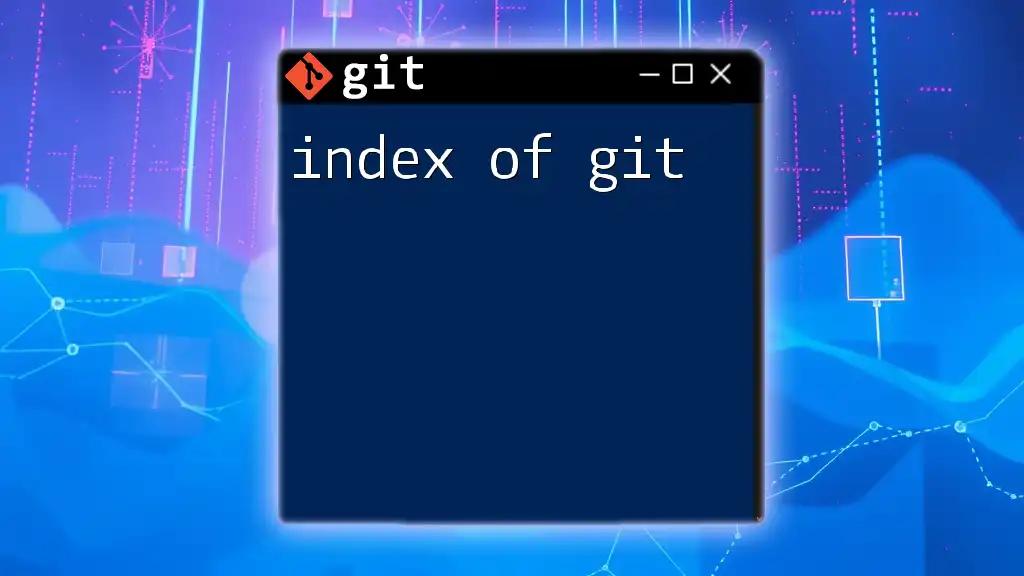
Git Configuration
Global vs Local Configuration
Setting your identity in Git is crucial for keeping track of who made changes. Git allows for both global and local configurations.
A global configuration applies to all repositories on your local machine. To set your name and email globally, you can use the following commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Local configuration, on the other hand, applies only to the current repository. It is useful when you want to contribute to a project under a different identity.
Checking Configuration
To see the current configuration settings you have applied, you can run:
git config --list
This command displays all your configuration settings, giving you an overview of your current setup.
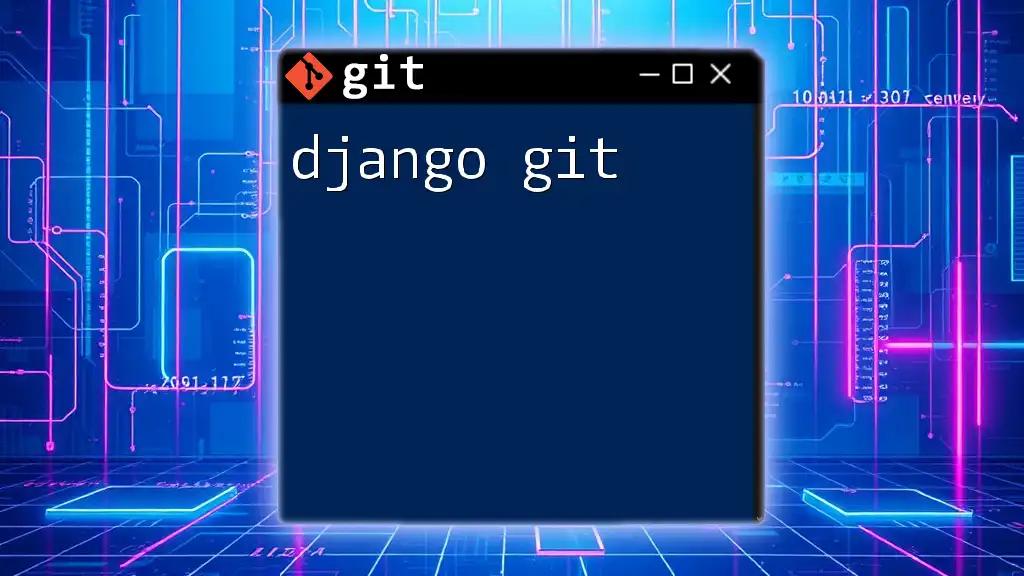
Advanced Concepts in Git
Stashing Changes
Sometimes, you may want to save your current changes temporarily without committing them. Stashing allows you to do just that, letting you switch branches or pull updates without losing your uncommitted work.
To stash your changes, run:
git stash
When you're ready to apply back those changes, use:
git stash apply
Rebase
Rebasing is a more advanced technique that allows you to transfer the base of your branch to a new commit. This helps keep a clean project history by avoiding merge commits.
The command for rebasing a branch looks like:
git rebase <branch-name>
This can often simplify the history of your project by creating a straight line of commits rather than a branching diagram.
Tags
Tags are a way to mark specific points in history as important or notable, often used to mark releases (e.g., version 1.0).
To create a tag, you would execute:
git tag v1.0
Tags make it easier to reference specific versions of your project in the future.
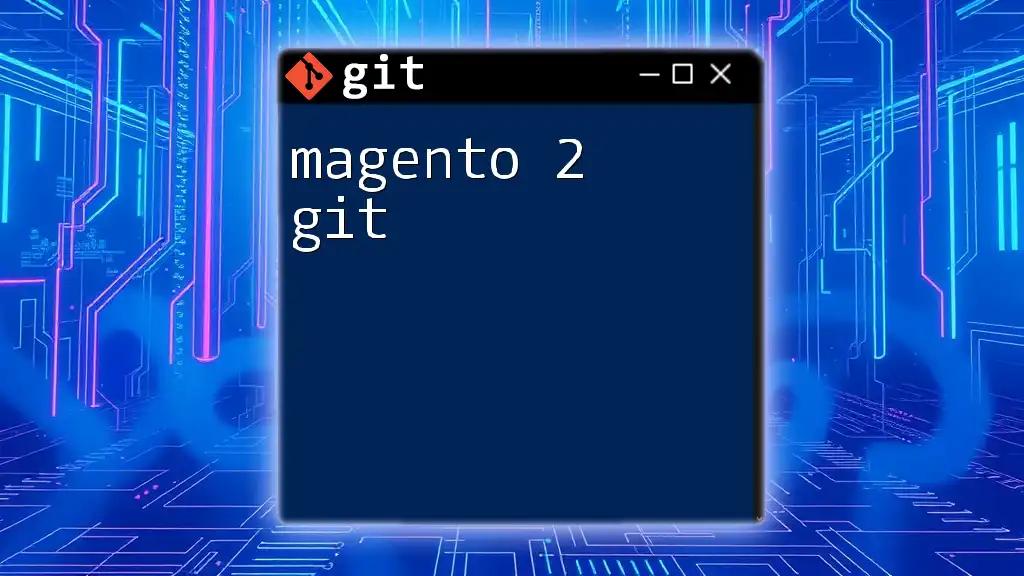
Common Git Commands Reference
A concise reference to essential Git commands is invaluable for quick look-up:
Command | Description |
---|---|
`git init` | Create a new local repository |
`git clone <url>` | Clone a remote repository |
`git add <file>` | Stage changes for commit |
`git commit -m "message"` | Commit staged changes |
`git push origin <branch>` | Push local changes to remote repository |
`git pull origin <branch>` | Fetch and merge changes from remote |
`git branch <branch-name>` | Create a new branch |
`git checkout <branch-name>` | Switch to the specified branch |
`git merge <branch-name>` | Merge specified branch into the current branch |
`git stash` | Stash uncommitted changes |
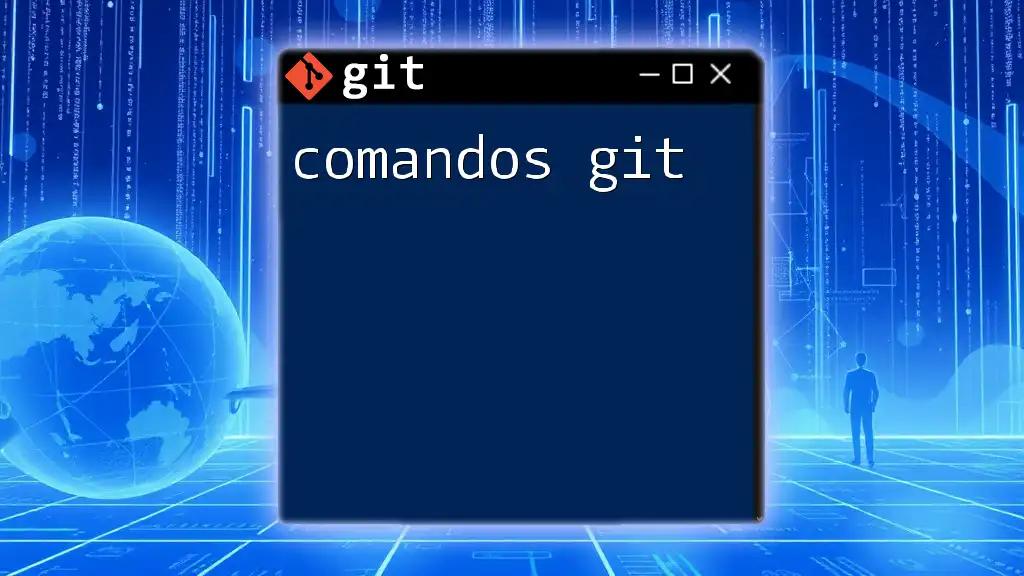
Conclusion
Understanding the anatomy of Git is essential for anyone looking to work efficiently in collaborative environments. By mastering the foundational elements of Git—repositories, commits, branches, merging, and remotes—developers can enhance their workflow, make informed contributions, and maintain order in their projects.
As you continue to practice the commands and concepts outlined in this guide, remember that Git offers powerful features for version control. Don’t hesitate to experiment and explore more advanced topics to elevate your programming skills. Whether you're part of a team or working solo, Git leads you toward seamless development experiences.
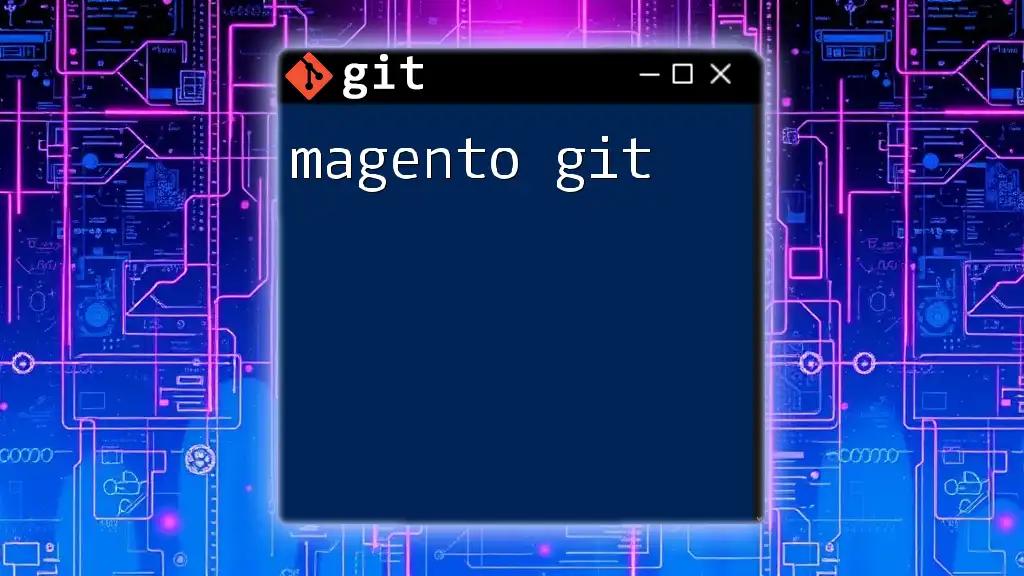
Additional Resources
For further learning, consider visiting the official [Git documentation](https://git-scm.com/doc) or exploring more specialized books and online courses designed to sharpen your Git proficiency.